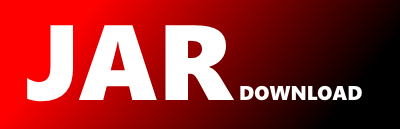
org.sonar.l10n.py.rules.python.S6781.html Maven / Gradle / Ivy
Secret leaks often occur when a sensitive piece of authentication data is stored with the source code of an application. Considering the source
code is intended to be deployed across multiple assets, including source code repositories or application hosting servers, the secrets might get
exposed to an unintended audience.
Why is this an issue?
In most cases, trust boundaries are violated when a secret is exposed in a source code repository or an uncontrolled deployment environment.
Unintended people who don’t need to know the secret might get access to it. They might then be able to use it to gain unwanted access to associated
services or resources.
The trust issue can be more or less severe depending on the people’s role and entitlement.
What is the potential impact?
If a JWT secret key leaks to an unintended audience, it can have serious security implications for the corresponding application. The secret key is
used to encode and decode JWTs when using a symmetric signing algorithm, and an attacker could potentially use it to perform malicious actions.
For example, an attacker could use the secret key to create their own authentication tokens that appear to be legitimate, allowing them to bypass
authentication and gain access to sensitive data or functionality.
In the worst-case scenario, an attacker could be able to execute arbitrary code on the application by abusing administrative features, and take
over its hosting server.
How to fix it in Flask
Revoke the secret
Revoke any leaked secrets and remove them from the application source code.
Before revoking the secret, ensure that no other applications or processes are using it. Other usages of the secret will also be impacted when the
secret is revoked.
Changing the secret value is sufficient to invalidate any data that it protected.
Code examples
Noncompliant code example
The following noncompliant code contains a hard-coded secret that can be exposed unintentionally.
from flask import Flask
app = Flask(__name__)
app.config['JWT_SECRET_KEY'] = secret_key # Noncompliant
Compliant solution
A solution is to set this secret in an environment string.
from flask import Flask
import os
app = Flask(__name__)
app.config['JWT_SECRET_KEY'] = os.environ["JWT_SECRET_KEY"]
Going the extra mile
Use a secret vault
A secret vault should be used to generate and store the new secret. This will ensure the secret’s security and prevent any further unexpected
disclosure.
Depending on the development platform and the leaked secret type, multiple solutions are currently available.
How to fix it in python-jose
Code examples
Noncompliant code example
The following noncompliant FastAPI application contains a hard-coded secret that can be exposed unintentionally.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
secret_key = 'secret'
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, secret_key, algorithm="HS256") # Noncompliant
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Compliant solution
A solution is to set this secret in an environment variable.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import jwt
import os
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
secret_key = os.environ["JWT_SECRET_KEY"]
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, secret_key, algorithm="HS256")
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Noncompliant code example
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
private_key = '''-----BEGIN PRIVATE KEY-----
MIIEvgIBADANBgkqhkiG9w0BAQEFAASCBKgwggSkAgEAAoIBAQDAK5Lsx5Ow0N+d
...
-----END PRIVATE KEY-----'''
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, private_key, algorithm="RS256") # Noncompliant
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Compliant solution
When using an asymmetric encryption algorithm, the keys can be loaded from a file instead of being hardcoded.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
private_key = ''
with open('resources/rs256.pem', 'r') as f:
private_key = f.read()
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, private_key, algorithm="RS256")
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Going the extra mile
Use a secret vault
A secret vault should be used to generate and store the new secret. This will ensure the secret’s security and prevent any further unexpected
disclosure.
Depending on the development platform and the leaked secret type, multiple solutions are currently available.
How to fix it in PyJWT
Code examples
Noncompliant code example
The following noncompliant FastAPI application contains a hard-coded secret that can be exposed unintentionally.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
secret_key = 'secret'
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, secret_key, algorithm="HS256") # Noncompliant
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Compliant solution
A solution is to set this secret in an environment string.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
import jwt
import os
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
secret_key = os.environ["JWT_SECRET_KEY"]
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, secret_key, algorithm="HS256")
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Noncompliant code example
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
private_key = '''-----BEGIN PRIVATE KEY-----
MIIEvgIBADANBgkqhkiG9w0BAQEFAASCBKgwggSkAgEAAoIBAQDAK5Lsx5Ow0N+d
...
-----END PRIVATE KEY-----'''
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, private_key, algorithm="RS256") # Noncompliant
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Compliant solution
When using an asymmetric encryption algorithm, the keys can be loaded from a file instead of being hardcoded.
from typing import Dict
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
import jwt
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="login")
private_key = ''
with open('resources/rs256.pem', 'r') as f:
private_key = f.read()
def create_access_token(data: dict):
to_encode = data.copy()
to_encode.update({"exp": datetime.now(timezone.utc) +
timedelta(minutes=15)})
return jwt.encode(to_encode, private_key, algorithm="RS256")
def validate_login(username: str, password: str) -> None:
...
@app.post("/login")
async def login(
form_data: OAuth2PasswordRequestForm = Depends()
) -> Dict[str, str]:
validate_login(form_data.username, form_data.password)
return dict(access_token=create_access_token(data={"sub": form_data.username}))
Going the extra mile
Use a secret vault
A secret vault should be used to generate and store the new secret. This will ensure the secret’s security and prevent any further unexpected
disclosure.
Depending on the development platform and the leaked secret type, multiple solutions are currently available.
How to fix it in Django
Code examples
Noncompliant code example
The following noncompliant Django REST framework application contains a hard-coded secret that can be exposed unintentionally.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
]
REST_FRAMEWORK = {
'DEFAULT_PERMISSION_CLASSES': [
'rest_framework.permissions.DjangoModelPermissionsOrAnonReadOnly',
],
'DEFAULT_AUTHENTICATION_CLASSES': (
'rest_framework_simplejwt.authentication.JWTAuthentication',
)
}
SIMPLE_JWT = {
"ALGORITHM": "HS256",
"SIGNING_KEY": "secret" # Noncompliant
}
Compliant solution
A solution is to set this secret in an environment variable.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
]
REST_FRAMEWORK = {
'DEFAULT_PERMISSION_CLASSES': [
'rest_framework.permissions.DjangoModelPermissionsOrAnonReadOnly',
],
'DEFAULT_AUTHENTICATION_CLASSES': (
'rest_framework_simplejwt.authentication.JWTAuthentication',
)
}
SIMPLE_JWT = {
"ALGORITHM": "HS256",
"SIGNING_KEY": os.environ["SIGNING_KEY"]
}
Going the extra mile
Use a secret vault
A secret vault should be used to generate and store the new secret. This will ensure the secret’s security and prevent any further unexpected
disclosure.
Depending on the development platform and the leaked secret type, multiple solutions are currently available.
Resources
Standards
- OWASP - Top 10 2021 Category A7 - Identification and
Authentication Failures
- OWASP - Top 10 2017 Category A3 - Sensitive Data
Exposure
- CWE - CWE-798 - Use of Hard-coded Credentials
- CWE - CWE-259 - Use of Hard-coded Password
- STIG Viewer - Application Security and
Development: V-222642 - The application must not contain embedded authentication data.
Documentation
- Flask JWT documentation - Config - JWT_SECRET_KEY
- Python-Jose documentation - JSON Web Token
- PyJWT documentation - API Reference
- Simple JWT documentation - SIGNING_KEY