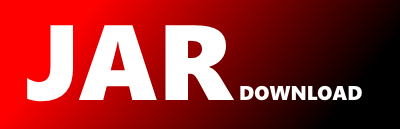
org.sonar.l10n.py.rules.python.S6978.html Maven / Gradle / Ivy
This rule raises an issue when a class is a Pytorch module and does not call the super().__init__()
method in its constructor.
Why is this an issue?
To provide the AutoGrad functionality, the Pytorch library needs to set up the necessary data structures in the base class. If the
super().__init__()
method is not called, the module will not be able to keep track of its parameters and other attributes.
For example, when trying to instantiate a module like nn.Linear
without calling the super().__init__()
method, the
instantiation will fail when it tries to register it as a submodule of the parent module.
import torch.nn as nn
class MyCustomModule(nn.Module):
def __init__(self, input_size, output_size):
self.fc = nn.Linear(input_size, output_size)
model = MyCustomModule(10, 5) # AttributeError: cannot assign module before Module.__init__() call
How to fix it
Add a call to super().__init__()
at the beginning of the constructor of the class.
Code examples
Noncompliant code example
import torch.nn as nn
class MyCustomModule(nn.Module):
def __init__(self, input_size, output_size):
self.fc = nn.Linear(input_size, output_size) # Noncompliant: creating an nn.Linear without calling super().__init__()
Compliant solution
import torch.nn as nn
class MyCustomModule(nn.Module):
def __init__(self, input_size, output_size):
super().__init__()
self.fc = nn.Linear(input_size, output_size)
Resources
Documentation
- Pytorch documentation - torch.nn.Module
© 2015 - 2025 Weber Informatics LLC | Privacy Policy