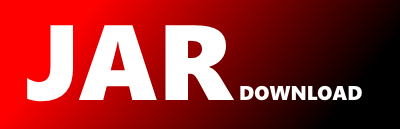
org.sonar.python.cfg.fixpoint.CfgBlockState Maven / Gradle / Ivy
The newest version!
/*
* SonarQube Python Plugin
* Copyright (C) 2011-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.python.cfg.fixpoint;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.sonar.plugins.python.api.cfg.CfgBlock;
import org.sonar.plugins.python.api.tree.Tree;
import org.sonar.python.cfg.fixpoint.ReadWriteVisitor.SymbolReadWrite;
import org.sonar.plugins.python.api.symbols.Symbol;
public abstract class CfgBlockState {
protected final CfgBlock block;
protected final Map> variableReadWritesPerElement;
/**
* variables that are being read in the block
*/
protected final Set gen = new HashSet<>();
/**
* variables that are being written in the block
*/
protected final Set kill = new HashSet<>();
CfgBlockState(CfgBlock block) {
this.block = block;
this.variableReadWritesPerElement = new HashMap<>();
}
public Map getSymbolReadWrites(Tree tree) {
return variableReadWritesPerElement.get(tree);
}
protected void init(CfgBlock block) {
// 'writtenOnly' has variables that are WRITE-ONLY inside at least one element
// (as opposed to 'kill' which can have a variable that inside an element is both READ and WRITTEN)
Set writtenOnly = new HashSet<>();
for (Tree element : block.elements()) {
ReadWriteVisitor readWriteVisitor = new ReadWriteVisitor();
element.accept(readWriteVisitor);
variableReadWritesPerElement.put(element, readWriteVisitor.symbolToUsages());
computeGenAndKill(writtenOnly, readWriteVisitor.symbolToUsages());
}
}
/**
* This has side effects on 'writtenOnly'
*/
private void computeGenAndKill(Set writtenOnly, Map symbolToUsages) {
for (Map.Entry symbolListEntry : symbolToUsages.entrySet()) {
Symbol symbol = symbolListEntry.getKey();
SymbolReadWrite usage = symbolListEntry.getValue();
if (usage.isRead() && !writtenOnly.contains(symbol)) {
gen.add(symbol);
}
if (usage.isWrite()) {
kill.add(symbol);
if (!usage.isRead()) {
writtenOnly.add(symbol);
}
}
}
}
public Set getGen() {
return gen;
}
public Set getKill() {
return kill;
}
public boolean isSymbolUsedInBlock(Symbol symbol) {
return variableReadWritesPerElement.values().stream()
.flatMap(m -> m.keySet().stream())
.anyMatch(s -> s == symbol);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy