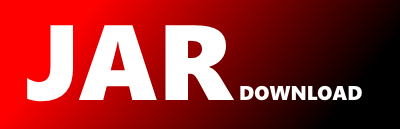
org.sonar.python.semantic.DependencyGraph Maven / Gradle / Ivy
The newest version!
/*
* SonarQube Python Plugin
* Copyright (C) 2011-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.python.semantic;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class DependencyGraph {
private final Map> dependentModules;
private DependencyGraph(Map> dependentModules) {
this.dependentModules = dependentModules;
}
public Map> dependentModules() {
return Collections.unmodifiableMap(dependentModules);
}
public static DependencyGraph from(Map> importsByModule, Set projectModulesFQN) {
Map> dependentModules = computeDependentModules(importsByModule, projectModulesFQN);
return new DependencyGraph(dependentModules);
}
private static Map> computeDependentModules(Map> importsByModule, Set projectModulesFQN) {
Map> result = new HashMap<>();
for (var entry : importsByModule.entrySet()) {
entry.getValue().forEach(importedModuleFQN -> {
String dependentModule = entry.getKey();
if (projectModulesFQN.contains(importedModuleFQN)) {
result.computeIfAbsent(importedModuleFQN, x -> new HashSet<>()).add(dependentModule);
return;
}
int endIndex = importedModuleFQN.lastIndexOf(".");
if (endIndex < 0) {
return;
}
String substring = importedModuleFQN.substring(0, endIndex);
if (projectModulesFQN.contains(substring)) {
result.computeIfAbsent(substring, x -> new HashSet<>()).add(dependentModule);
}
});
}
return result;
}
public Set impactedModules(List modifiedModules) {
Set impactedModules = new HashSet<>();
for (String modifiedModuleFQN : modifiedModules) {
recursivelyComputeImpactedModules(modifiedModuleFQN, impactedModules);
}
return impactedModules;
}
private void recursivelyComputeImpactedModules(String changedModule, Set impactedModules) {
if (!impactedModules.contains(changedModule)) {
impactedModules.add(changedModule);
Set transitivelyImpactedModules = dependentModules.get(changedModule);
if (transitivelyImpactedModules == null) {
return;
}
for (String transitivelyImpacted : transitivelyImpactedModules) {
recursivelyComputeImpactedModules(transitivelyImpacted, impactedModules);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy