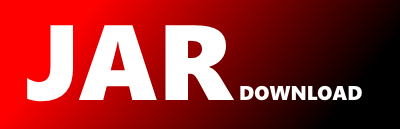
org.sonar.python.index.FunctionDescriptor Maven / Gradle / Ivy
The newest version!
/*
* SonarQube Python Plugin
* Copyright (C) 2011-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Sonar Source-Available License Version 1, as published by SonarSource SA.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the Sonar Source-Available License for more details.
*
* You should have received a copy of the Sonar Source-Available License
* along with this program; if not, see https://sonarsource.com/license/ssal/
*/
package org.sonar.python.index;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.sonar.plugins.python.api.LocationInFile;
public class FunctionDescriptor implements Descriptor {
private final String name;
private final String fullyQualifiedName;
private final List parameters;
private final boolean isAsynchronous;
private final boolean isInstanceMethod;
private final List decorators;
private final boolean hasDecorators;
@Nullable
private final LocationInFile definitionLocation;
@Nullable
private final String annotatedReturnTypeName;
@Nullable
private final TypeAnnotationDescriptor typeAnnotationDescriptor;
public FunctionDescriptor(String name, String fullyQualifiedName, List parameters, boolean isAsynchronous,
boolean isInstanceMethod, List decorators, boolean hasDecorators, @Nullable LocationInFile definitionLocation, @Nullable String annotatedReturnTypeName) {
this(name, fullyQualifiedName, parameters, isAsynchronous, isInstanceMethod, decorators, hasDecorators, definitionLocation, annotatedReturnTypeName, null);
}
public FunctionDescriptor(String name, String fullyQualifiedName, List parameters, boolean isAsynchronous,
boolean isInstanceMethod, List decorators, boolean hasDecorators, @Nullable LocationInFile definitionLocation,
@Nullable String annotatedReturnTypeName, @Nullable TypeAnnotationDescriptor typeAnnotationDescriptor) {
this.name = name;
this.fullyQualifiedName = fullyQualifiedName;
this.parameters = parameters;
this.isAsynchronous = isAsynchronous;
this.isInstanceMethod = isInstanceMethod;
this.decorators = decorators;
this.hasDecorators = hasDecorators;
this.definitionLocation = definitionLocation;
this.annotatedReturnTypeName = annotatedReturnTypeName;
this.typeAnnotationDescriptor = typeAnnotationDescriptor;
}
@Override
public String name() {
return name;
}
@Override
@Nonnull
public String fullyQualifiedName() {
return fullyQualifiedName;
}
@Override
public Kind kind() {
return Kind.FUNCTION;
}
public List parameters() {
return parameters;
}
public boolean isAsynchronous() {
return isAsynchronous;
}
public boolean isInstanceMethod() {
return isInstanceMethod;
}
public List decorators() {
return decorators;
}
public boolean hasDecorators() {
return hasDecorators;
}
@CheckForNull
public LocationInFile definitionLocation() {
return definitionLocation;
}
@CheckForNull
public String annotatedReturnTypeName() {
return annotatedReturnTypeName;
}
@CheckForNull
public TypeAnnotationDescriptor typeAnnotationDescriptor() {
return typeAnnotationDescriptor;
}
public static class Parameter {
private final String name;
private final String annotatedType;
private final boolean hasDefaultValue;
private final boolean isKeywordVariadic;
private final boolean isPositionalVariadic;
private final boolean isKeywordOnly;
private final boolean isPositionalOnly;
private final LocationInFile location;
public Parameter(@Nullable String name, @Nullable String annotatedType, boolean hasDefaultValue,
boolean isKeywordOnly, boolean isPositionalOnly, boolean isPositionalVariadic, boolean isKeywordVariadic, @Nullable LocationInFile location) {
this.name = name;
this.annotatedType = annotatedType;
this.hasDefaultValue = hasDefaultValue;
this.isKeywordVariadic = isKeywordVariadic;
this.isPositionalVariadic = isPositionalVariadic;
this.isKeywordOnly = isKeywordOnly;
this.isPositionalOnly = isPositionalOnly;
this.location = location;
}
@CheckForNull
public String name() {
return name;
}
public String annotatedType() {
return annotatedType;
}
public boolean hasDefaultValue() {
return hasDefaultValue;
}
public boolean isVariadic() {
return isKeywordVariadic || isPositionalVariadic;
}
public boolean isKeywordOnly() {
return isKeywordOnly;
}
public boolean isPositionalOnly() {
return isPositionalOnly;
}
public boolean isKeywordVariadic() {
return isKeywordVariadic;
}
public boolean isPositionalVariadic() {
return isPositionalVariadic;
}
@CheckForNull
public LocationInFile location() {
return location;
}
}
public static class FunctionDescriptorBuilder {
private String name;
private String fullyQualifiedName;
private List parameters = new ArrayList<>();
private boolean isAsynchronous = false;
private boolean isInstanceMethod = false;
private List decorators = new ArrayList<>();
private boolean hasDecorators = false;
private LocationInFile definitionLocation = null;
private String annotatedReturnTypeName = null;
private TypeAnnotationDescriptor typeAnnotationDescriptor = null;
public FunctionDescriptorBuilder withName(String name) {
this.name = name;
return this;
}
public FunctionDescriptorBuilder withFullyQualifiedName(String fullyQualifiedName) {
this.fullyQualifiedName = fullyQualifiedName;
return this;
}
public FunctionDescriptorBuilder withParameters(List parameters) {
this.parameters = parameters;
return this;
}
public FunctionDescriptorBuilder withIsAsynchronous(boolean isAsynchronous) {
this.isAsynchronous = isAsynchronous;
return this;
}
public FunctionDescriptorBuilder withIsInstanceMethod(boolean isInstanceMethod) {
this.isInstanceMethod = isInstanceMethod;
return this;
}
public FunctionDescriptorBuilder withDecorators(List decorators) {
this.decorators = decorators;
return this;
}
public FunctionDescriptorBuilder withHasDecorators(boolean hasDecorators) {
this.hasDecorators = hasDecorators;
return this;
}
public FunctionDescriptorBuilder withDefinitionLocation(@Nullable LocationInFile definitionLocation) {
this.definitionLocation = definitionLocation;
return this;
}
public FunctionDescriptorBuilder withAnnotatedReturnTypeName(@Nullable String annotatedReturnTypeName) {
this.annotatedReturnTypeName = annotatedReturnTypeName;
return this;
}
public FunctionDescriptorBuilder withTypeAnnotationDescriptor(@Nullable TypeAnnotationDescriptor typeAnnotationDescriptor) {
this.typeAnnotationDescriptor = typeAnnotationDescriptor;
return this;
}
public FunctionDescriptor build() {
return new FunctionDescriptor(name, fullyQualifiedName, parameters, isAsynchronous, isInstanceMethod, decorators,
hasDecorators, definitionLocation, annotatedReturnTypeName, typeAnnotationDescriptor);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy