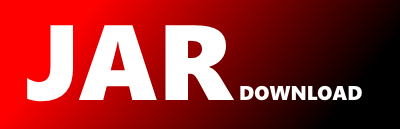
org.sonarsource.ruby.converter.RubyConverter Maven / Gradle / Ivy
/*
* SonarSource Ruby
* Copyright (C) 2018-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonarsource.ruby.converter;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
import org.jruby.Ruby;
import org.jruby.RubyRuntimeAdapter;
import org.jruby.exceptions.NoMethodError;
import org.jruby.exceptions.StandardError;
import org.jruby.javasupport.JavaEmbedUtils;
import org.jruby.runtime.builtin.IRubyObject;
import org.jruby.specialized.RubyArrayTwoObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.sonarsource.ruby.converter.adapter.CommentAdapter;
import org.sonarsource.ruby.converter.adapter.RangeAdapter;
import org.sonarsource.ruby.converter.adapter.TokenAdapter;
import org.sonarsource.slang.api.ASTConverter;
import org.sonarsource.slang.api.Comment;
import org.sonarsource.slang.api.ParseException;
import org.sonarsource.slang.api.TextPointer;
import org.sonarsource.slang.api.TextRange;
import org.sonarsource.slang.api.Token;
import org.sonarsource.slang.api.Tree;
import org.sonarsource.slang.api.TreeMetaData;
import org.sonarsource.slang.impl.TextRanges;
import org.sonarsource.slang.impl.TopLevelTreeImpl;
import org.sonarsource.slang.impl.TreeMetaDataProvider;
import static java.nio.charset.StandardCharsets.UTF_8;
import static java.util.Collections.emptyList;
import static java.util.Collections.singletonList;
public class RubyConverter implements ASTConverter {
private static final Logger LOG = LoggerFactory.getLogger(RubyConverter.class);
private static final String SETUP_SCRIPT_PATH = "whitequark_parser_init.rb";
private static final String RACC_RUBYGEM_PATH = "racc-1.8.1-java/lib";
private static final String AST_RUBYGEM_PATH = "ast-2.4.2/lib";
private static final String PARSER_RUBYGEM_PATH = "parser-3.3.4.2/lib";
private static final String COMMENT_TOKEN_TYPE = "tCOMMENT";
static final String FILENAME = "(Analysis of Ruby)";
private final RubyRuntimeAdapter rubyRuntimeAdapter;
private final Ruby runtime;
RubyConverter(RubyRuntimeAdapter rubyRuntimeAdapter) {
this.rubyRuntimeAdapter = rubyRuntimeAdapter;
try {
runtime = initializeRubyRuntime();
} catch (IOException e) {
throw new IllegalStateException("Failed to initialized ruby runtime", e);
}
}
public RubyConverter() {
this(JavaEmbedUtils.newRuntimeAdapter());
}
@Override
public void terminate() {
// Shutdown and terminate ruby instance
if (runtime != null) {
JavaEmbedUtils.terminate(runtime);
}
}
@Override
public Tree parse(String content) {
try {
return parseContent(content);
} catch (StandardError e) {
throw new ParseException(e.getMessage(), getErrorLocation(e), e);
} catch (Exception e) {
throw new ParseException(e.getMessage(), null, e);
}
}
TextPointer getErrorLocation(StandardError e) {
try {
IRubyObject diagnostic = (IRubyObject) invokeMethod(e.getException(), "diagnostic", null);
IRubyObject location = (IRubyObject) invokeMethod(diagnostic, "location", null);
if (location != null) {
return new RangeAdapter(runtime, location).toTextRange().start();
}
} catch (NoMethodError nme) {
// location information could not be retrieved from ruby object
}
LOG.warn("No location information available for parse error");
return null;
}
Tree parseContent(String content) {
Object[] parameters = {content, FILENAME};
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy