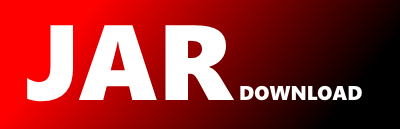
org.sonar.runner.cache.PersistentCacheBuilder Maven / Gradle / Ivy
/*
* SonarQube Runner - API
* Copyright (C) 2011 SonarSource
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02
*/
package org.sonar.runner.cache;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.concurrent.TimeUnit;
import javax.annotation.Nullable;
/**
* Cache files will be placed in 3 areas:
*
* <sonar_home>/ws_cache/<server_url>-<version>/projects/<project>/
* <sonar_home>/ws_cache/<server_url>-<version>/global/
* <sonar_home>/ws_cache/<server_url>-<version>/local/
*
*/
public class PersistentCacheBuilder {
private static final long DEFAULT_EXPIRE_DURATION = TimeUnit.MILLISECONDS.convert(9999L, TimeUnit.DAYS);
private static final String DIR_NAME = "ws_cache";
private Path cacheBasePath;
private Path relativePath;
private final Logger logger;
public PersistentCacheBuilder(Logger logger) {
this.logger = logger;
}
public PersistentCacheBuilder setAreaForProject(String serverUrl, String serverVersion, String projectKey) {
relativePath = Paths.get(sanitizeFilename(serverUrl))
.resolve(sanitizeFilename(serverVersion))
.resolve("projects")
.resolve(sanitizeFilename(projectKey));
return this;
}
public PersistentCacheBuilder setAreaForGlobal(String serverUrl) {
relativePath = Paths.get(sanitizeFilename(serverUrl))
.resolve("global");
return this;
}
public PersistentCacheBuilder setAreaForLocalProject(String serverUrl, String serverVersion) {
relativePath = Paths.get(sanitizeFilename(serverUrl))
.resolve(sanitizeFilename(serverVersion))
.resolve("local");
return this;
}
public PersistentCacheBuilder setSonarHome(@Nullable Path p) {
if (p != null) {
this.cacheBasePath = p.resolve(DIR_NAME);
}
return this;
}
public PersistentCache build() {
if (relativePath == null) {
throw new IllegalStateException("area must be set before building");
}
if (cacheBasePath == null) {
setSonarHome(findHome());
}
Path cachePath = cacheBasePath.resolve(relativePath);
DirectoryLock lock = new DirectoryLock(cacheBasePath, logger);
PersistentCacheInvalidation criteria = new TTLCacheInvalidation(DEFAULT_EXPIRE_DURATION);
return new PersistentCache(cachePath, criteria, logger, lock);
}
private static Path findHome() {
String home = System.getenv("SONAR_USER_HOME");
if (home != null) {
return Paths.get(home);
}
home = System.getProperty("user.home");
return Paths.get(home, ".sonar");
}
private static String sanitizeFilename(String name) {
try {
return URLEncoder.encode(name, StandardCharsets.UTF_8.name());
} catch (UnsupportedEncodingException e) {
throw new IllegalStateException("Couldn't sanitize filename: " + name, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy