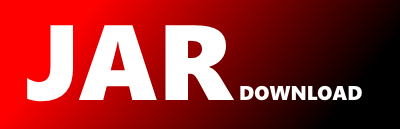
org.sonarsource.sonarlint.ls.connected.notifications.TaintVulnerabilityRaisedNotification Maven / Gradle / Ivy
/*
* SonarLint Language Server
* Copyright (C) 2009-2023 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonarsource.sonarlint.ls.connected.notifications;
import com.google.gson.JsonPrimitive;
import java.util.List;
import org.eclipse.lsp4j.ExecuteCommandParams;
import org.eclipse.lsp4j.MessageActionItem;
import org.eclipse.lsp4j.MessageType;
import org.eclipse.lsp4j.ShowMessageRequestParams;
import org.eclipse.lsp4j.jsonrpc.CompletableFutures;
import org.sonarsource.sonarlint.core.serverapi.push.TaintVulnerabilityRaisedEvent;
import org.sonarsource.sonarlint.ls.CommandManager;
import org.sonarsource.sonarlint.ls.SonarLintExtendedLanguageClient;
public class TaintVulnerabilityRaisedNotification {
private final SonarLintExtendedLanguageClient client;
private final CommandManager commandManager;
public static final MessageActionItem SETTINGS_ACTION = new MessageActionItem("Open Settings");
public static final MessageActionItem SHOW_ISSUE_ACTION = new MessageActionItem("Show Issue");
static final String SONARLINT_SHOW_TAINT_VULNERABILITY_FLOWS = "SonarLint.ShowTaintVulnerabilityFlows";
public TaintVulnerabilityRaisedNotification(SonarLintExtendedLanguageClient client, CommandManager commandManager) {
this.client = client;
this.commandManager = commandManager;
}
public void showTaintVulnerabilityNotification(TaintVulnerabilityRaisedEvent event, String connectionId, boolean isSonarCloud) {
var notificationParams = new ShowMessageRequestParams();
notificationParams.setType(MessageType.Info);
notificationParams.setMessage(String.format("SonarQube Notification: new injection vulnerability detected on project '%s' on branch '%s'." +
" Apply a fix and re-trigger remote analysis." +
" [Learn More](https://docs.sonarsource.com/sonarlint/vs-code/getting-started/requirements/#injection-vulnerabilities)", event.getProjectKey(), event.getBranchName()));
notificationParams.setActions(List.of(SHOW_ISSUE_ACTION, SETTINGS_ACTION));
var showIssueCommandParams = new ExecuteCommandParams(SONARLINT_SHOW_TAINT_VULNERABILITY_FLOWS, List.of(new JsonPrimitive(event.getKey()), new JsonPrimitive(connectionId)));
client.showMessageRequest(notificationParams).thenAccept(action -> {
if (SHOW_ISSUE_ACTION.equals(action)) {
CompletableFutures.computeAsync(cancelToken -> {
cancelToken.checkCanceled();
commandManager.executeCommand(showIssueCommandParams, cancelToken);
return null;
});
} else if (SETTINGS_ACTION.equals(action)) {
client.openConnectionSettings(isSonarCloud);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy