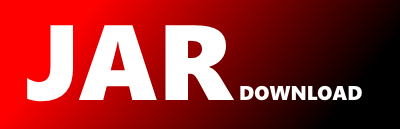
org.sonar.db.measure.MeasureDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-db Show documentation
Show all versions of sonar-db Show documentation
Create and request SonarQube schema
/*
* SonarQube
* Copyright (C) 2009-2016 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.db.measure;
import com.google.common.base.Function;
import com.google.common.collect.Lists;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import org.sonar.db.Dao;
import org.sonar.db.DatabaseUtils;
import org.sonar.db.DbSession;
import org.sonar.db.component.SnapshotDto;
import static com.google.common.collect.FluentIterable.from;
public class MeasureDao implements Dao {
public boolean existsByKey(DbSession session, String componentKey, String metricKey) {
return mapper(session).countByComponentAndMetric(componentKey, metricKey) > 0;
}
@CheckForNull
public MeasureDto selectByComponentKeyAndMetricKey(DbSession session, String componentKey, String metricKey) {
return mapper(session).selectByComponentAndMetric(componentKey, metricKey);
}
public List selectByComponentKeyAndMetricKeys(final DbSession session, final String componentKey, List metricKeys) {
return DatabaseUtils.executeLargeInputs(metricKeys, new Function, List>() {
@Override
public List apply(List keys) {
return mapper(session).selectByComponentAndMetrics(componentKey, keys);
}
});
}
/**
* Selects all measures of a specific snapshot for the specified metric keys.
*
* Uses by Views.
*/
public List selectBySnapshotIdAndMetricKeys(final long snapshotId, Set metricKeys, final DbSession dbSession) {
return DatabaseUtils.executeLargeInputs(from(metricKeys).toSortedList(String.CASE_INSENSITIVE_ORDER),
new Function, List>() {
@Override
public List apply(List keys) {
return mapper(dbSession).selectBySnapshotAndMetricKeys(snapshotId, keys);
}
});
}
public List selectByComponentUuidAndProjectSnapshotIdAndMetricIds(final DbSession session, final String componentUuid, final long projectSnapshotId,
Set metricIds) {
return DatabaseUtils.executeLargeInputs(metricIds, new Function, List>() {
@Override
public List apply(List ids) {
return mapper(session).selectByComponentUuidAndProjectSnapshotIdAndStatusAndMetricIds(componentUuid, projectSnapshotId, ids,
SnapshotDto.STATUS_PROCESSED);
}
});
}
/**
* Used by plugin Developer Cockpit
*/
public List selectByDeveloperForSnapshotAndMetrics(final DbSession dbSession, final long developerId, final long snapshotId, Collection metricIds) {
return DatabaseUtils.executeLargeInputs(metricIds, new Function, List>() {
@Override
@Nonnull
public List apply(@Nonnull List input) {
return mapper(dbSession).selectByDeveloperForSnapshotAndMetrics(developerId, snapshotId, input);
}
});
}
/**
* Used by plugin Developer Cockpit
*/
public List selectBySnapshotAndMetrics(final DbSession dbSession, final long snapshotId, Collection metricIds) {
return DatabaseUtils.executeLargeInputs(metricIds, new Function, List>() {
@Override
@Nonnull
public List apply(@Nonnull List input) {
return mapper(dbSession).selectBySnapshotAndMetrics(snapshotId, input);
}
});
}
/**
* Used by plugin Developer Cockpit
*/
public List selectBySnapshotIdsAndMetricIds(final DbSession dbSession, List snapshotIds, final List metricIds) {
return DatabaseUtils.executeLargeInputs(snapshotIds, new Function, List>() {
@Override
@Nonnull
public List apply(@Nonnull List input) {
return mapper(dbSession).selectBySnapshotIdsAndMetricIds(input, metricIds);
}
});
}
/**
* Retrieves all measures associated to a specific developer and to the last snapshot of any project.
* property {@link MeasureDto#componentId} of the returned objects is populated
*
* Used by Developer Cockpit
*/
public List selectProjectMeasuresByDeveloperForMetrics(DbSession dbSession, long developerId, Collection metricIds) {
return mapper(dbSession).selectProjectMeasuresByDeveloperForMetrics(developerId, metricIds);
}
public void insert(DbSession session, MeasureDto measureDto) {
mapper(session).insert(measureDto);
}
public void insert(DbSession session, Collection items) {
for (MeasureDto item : items) {
insert(session, item);
}
}
public void insert(DbSession session, MeasureDto item, MeasureDto... others) {
insert(session, Lists.asList(item, others));
}
public List selectMetricKeysForSnapshot(DbSession session, long snapshotId) {
return mapper(session).selectMetricKeysForSnapshot(snapshotId);
}
private MeasureMapper mapper(DbSession session) {
return session.getMapper(MeasureMapper.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy