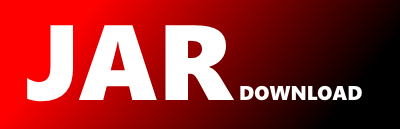
org.sonar.db.permission.PermissionDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-db Show documentation
Show all versions of sonar-db Show documentation
Create and request SonarQube schema
/*
* SonarQube
* Copyright (C) 2009-2016 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.db.permission;
import com.google.common.base.Function;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.apache.ibatis.session.ResultHandler;
import org.apache.ibatis.session.RowBounds;
import org.apache.ibatis.session.SqlSession;
import org.sonar.api.security.DefaultGroups;
import org.sonar.db.Dao;
import org.sonar.db.DatabaseUtils;
import org.sonar.db.DbSession;
import org.sonar.db.MyBatis;
import static com.google.common.collect.Maps.newHashMap;
public class PermissionDao implements Dao {
private static final String QUERY_PARAMETER = "query";
private static final String COMPONENT_ID_PARAMETER = "componentId";
private static final String ANYONE_GROUP_PARAMETER = "anyoneGroup";
private final MyBatis myBatis;
public PermissionDao(MyBatis myBatis) {
this.myBatis = myBatis;
}
/**
* @return a paginated list of users.
*/
public List selectUsers(DbSession session, PermissionQuery query, @Nullable Long componentId, int offset, int limit) {
Map params = usersParameters(query, componentId);
return mapper(session).selectUsers(params, new RowBounds(offset, limit));
}
public int countUsers(DbSession session, PermissionQuery query, @Nullable Long componentId) {
Map params = usersParameters(query, componentId);
return mapper(session).countUsers(params);
}
private static Map usersParameters(PermissionQuery query, @Nullable Long componentId) {
Map params = newHashMap();
params.put(QUERY_PARAMETER, query);
params.put(COMPONENT_ID_PARAMETER, componentId);
return params;
}
/**
* 'Anyone' group is not returned when it has not the asked permission.
* Membership parameter from query is not taking into account in order to deal more easily with the 'Anyone' group
*
* @return a non paginated list of groups.
*/
public List selectGroups(DbSession session, PermissionQuery query, @Nullable Long componentId) {
Map params = groupsParameters(query, componentId);
return mapper(session).selectGroups(params);
}
public List selectGroups(PermissionQuery query, @Nullable Long componentId) {
DbSession session = myBatis.openSession(false);
try {
return selectGroups(session, query, componentId);
} finally {
MyBatis.closeQuietly(session);
}
}
public int countGroups(DbSession session, String permission, @Nullable Long componentId) {
Map parameters = new HashMap<>();
parameters.put("permission", permission);
parameters.put(ANYONE_GROUP_PARAMETER, DefaultGroups.ANYONE);
parameters.put(COMPONENT_ID_PARAMETER, componentId);
return mapper(session).countGroups(parameters);
}
/**
* Each row returns a CountByProjectAndPermissionDto
*/
public void usersCountByComponentIdAndPermission(final DbSession dbSession, List componentIds, final ResultHandler resultHandler) {
final Map parameters = new HashMap<>();
DatabaseUtils.executeLargeInputsWithoutOutput(componentIds, new Function, Void>() {
@Override
public Void apply(@Nonnull List partitionedComponentIds) {
parameters.put("componentIds", partitionedComponentIds);
mapper(dbSession).usersCountByProjectIdAndPermission(parameters, resultHandler);
return null;
}
});
}
/**
* Each row returns a CountByProjectAndPermissionDto
*/
public void groupsCountByComponentIdAndPermission(final DbSession dbSession, final List componentIds, final ResultHandler resultHandler) {
final Map parameters = new HashMap<>();
parameters.put(ANYONE_GROUP_PARAMETER, DefaultGroups.ANYONE);
DatabaseUtils.executeLargeInputsWithoutOutput(componentIds, new Function, Void>() {
@Override
public Void apply(@Nonnull List partitionedComponentIds) {
parameters.put("componentIds", partitionedComponentIds);
mapper(dbSession).groupsCountByProjectIdAndPermission(parameters, resultHandler);
return null;
}
});
}
private static Map groupsParameters(PermissionQuery query, @Nullable Long componentId) {
Map params = newHashMap();
params.put(QUERY_PARAMETER, query);
params.put(COMPONENT_ID_PARAMETER, componentId);
params.put(ANYONE_GROUP_PARAMETER, DefaultGroups.ANYONE);
return params;
}
private static PermissionMapper mapper(SqlSession session) {
return session.getMapper(PermissionMapper.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy