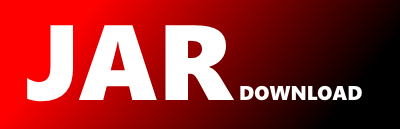
org.sonar.scanner.protocol.output.ScannerReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-scanner-protocol Show documentation
Show all versions of sonar-scanner-protocol Show documentation
Classes used for communication between scanner and server
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: scanner_report.proto
package org.sonar.scanner.protocol.output;
public final class ScannerReport {
private ScannerReport() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface MetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:Metadata)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 analysis_date = 1;
*/
long getAnalysisDate();
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
java.lang.String getProjectKey();
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
com.google.protobuf.ByteString
getProjectKeyBytes();
/**
* optional string branch = 3;
*/
java.lang.String getBranch();
/**
* optional string branch = 3;
*/
com.google.protobuf.ByteString
getBranchBytes();
/**
* optional int32 root_component_ref = 4;
*/
int getRootComponentRef();
/**
* optional bool cross_project_duplication_activated = 5;
*/
boolean getCrossProjectDuplicationActivated();
/**
* map<string, .Metadata.QProfile> qprofiles_per_language = 6;
*/
java.util.Map
getQprofilesPerLanguage();
}
/**
* Protobuf type {@code Metadata}
*/
public static final class Metadata extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Metadata)
MetadataOrBuilder {
// Use Metadata.newBuilder() to construct.
private Metadata(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Metadata() {
analysisDate_ = 0L;
projectKey_ = "";
branch_ = "";
rootComponentRef_ = 0;
crossProjectDuplicationActivated_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Metadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
analysisDate_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
projectKey_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
branch_ = s;
break;
}
case 32: {
rootComponentRef_ = input.readInt32();
break;
}
case 40: {
crossProjectDuplicationActivated_ = input.readBool();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
qprofilesPerLanguage_ = com.google.protobuf.MapField.newMapField(
QprofilesPerLanguageDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000020;
}
com.google.protobuf.MapEntry
qprofilesPerLanguage = input.readMessage(
QprofilesPerLanguageDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
qprofilesPerLanguage_.getMutableMap().put(qprofilesPerLanguage.getKey(), qprofilesPerLanguage.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetQprofilesPerLanguage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Metadata.class, org.sonar.scanner.protocol.output.ScannerReport.Metadata.Builder.class);
}
public interface QProfileOrBuilder extends
// @@protoc_insertion_point(interface_extends:Metadata.QProfile)
com.google.protobuf.MessageOrBuilder {
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string language = 3;
*/
java.lang.String getLanguage();
/**
* optional string language = 3;
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
* optional int64 rulesUpdatedAt = 4;
*/
long getRulesUpdatedAt();
}
/**
* Protobuf type {@code Metadata.QProfile}
*/
public static final class QProfile extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Metadata.QProfile)
QProfileOrBuilder {
// Use QProfile.newBuilder() to construct.
private QProfile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private QProfile() {
key_ = "";
name_ = "";
language_ = "";
rulesUpdatedAt_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private QProfile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
language_ = s;
break;
}
case 32: {
rulesUpdatedAt_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QProfile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.class, org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANGUAGE_FIELD_NUMBER = 3;
private volatile java.lang.Object language_;
/**
* optional string language = 3;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
}
}
/**
* optional string language = 3;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RULESUPDATEDAT_FIELD_NUMBER = 4;
private long rulesUpdatedAt_;
/**
* optional int64 rulesUpdatedAt = 4;
*/
public long getRulesUpdatedAt() {
return rulesUpdatedAt_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, key_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (!getLanguageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, language_);
}
if (rulesUpdatedAt_ != 0L) {
output.writeInt64(4, rulesUpdatedAt_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, key_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (!getLanguageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, language_);
}
if (rulesUpdatedAt_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, rulesUpdatedAt_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Metadata.QProfile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Metadata.QProfile)
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QProfile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.class, org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = "";
name_ = "";
language_ = "";
rulesUpdatedAt_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QProfile_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile build() {
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile result = new org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile(this);
result.key_ = key_;
result.name_ = name_;
result.language_ = language_;
result.rulesUpdatedAt_ = rulesUpdatedAt_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.getDefaultInstance()) return this;
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getLanguage().isEmpty()) {
language_ = other.language_;
onChanged();
}
if (other.getRulesUpdatedAt() != 0L) {
setRulesUpdatedAt(other.getRulesUpdatedAt());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object language_ = "";
/**
* optional string language = 3;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language = 3;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language = 3;
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
language_ = value;
onChanged();
return this;
}
/**
* optional string language = 3;
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* optional string language = 3;
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
language_ = value;
onChanged();
return this;
}
private long rulesUpdatedAt_ ;
/**
* optional int64 rulesUpdatedAt = 4;
*/
public long getRulesUpdatedAt() {
return rulesUpdatedAt_;
}
/**
* optional int64 rulesUpdatedAt = 4;
*/
public Builder setRulesUpdatedAt(long value) {
rulesUpdatedAt_ = value;
onChanged();
return this;
}
/**
* optional int64 rulesUpdatedAt = 4;
*/
public Builder clearRulesUpdatedAt() {
rulesUpdatedAt_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Metadata.QProfile)
}
// @@protoc_insertion_point(class_scope:Metadata.QProfile)
private static final org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public QProfile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new QProfile(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ANALYSIS_DATE_FIELD_NUMBER = 1;
private long analysisDate_;
/**
* optional int64 analysis_date = 1;
*/
public long getAnalysisDate() {
return analysisDate_;
}
public static final int PROJECT_KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object projectKey_;
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public java.lang.String getProjectKey() {
java.lang.Object ref = projectKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
projectKey_ = s;
return s;
}
}
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public com.google.protobuf.ByteString
getProjectKeyBytes() {
java.lang.Object ref = projectKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BRANCH_FIELD_NUMBER = 3;
private volatile java.lang.Object branch_;
/**
* optional string branch = 3;
*/
public java.lang.String getBranch() {
java.lang.Object ref = branch_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
branch_ = s;
return s;
}
}
/**
* optional string branch = 3;
*/
public com.google.protobuf.ByteString
getBranchBytes() {
java.lang.Object ref = branch_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
branch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROOT_COMPONENT_REF_FIELD_NUMBER = 4;
private int rootComponentRef_;
/**
* optional int32 root_component_ref = 4;
*/
public int getRootComponentRef() {
return rootComponentRef_;
}
public static final int CROSS_PROJECT_DUPLICATION_ACTIVATED_FIELD_NUMBER = 5;
private boolean crossProjectDuplicationActivated_;
/**
* optional bool cross_project_duplication_activated = 5;
*/
public boolean getCrossProjectDuplicationActivated() {
return crossProjectDuplicationActivated_;
}
public static final int QPROFILES_PER_LANGUAGE_FIELD_NUMBER = 6;
private static final class QprofilesPerLanguageDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_QprofilesPerLanguageEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile> qprofilesPerLanguage_;
private com.google.protobuf.MapField
internalGetQprofilesPerLanguage() {
if (qprofilesPerLanguage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
QprofilesPerLanguageDefaultEntryHolder.defaultEntry);
}
return qprofilesPerLanguage_;
}
/**
* map<string, .Metadata.QProfile> qprofiles_per_language = 6;
*/
public java.util.Map getQprofilesPerLanguage() {
return internalGetQprofilesPerLanguage().getMap();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (analysisDate_ != 0L) {
output.writeInt64(1, analysisDate_);
}
if (!getProjectKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, projectKey_);
}
if (!getBranchBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, branch_);
}
if (rootComponentRef_ != 0) {
output.writeInt32(4, rootComponentRef_);
}
if (crossProjectDuplicationActivated_ != false) {
output.writeBool(5, crossProjectDuplicationActivated_);
}
for (java.util.Map.Entry entry
: internalGetQprofilesPerLanguage().getMap().entrySet()) {
com.google.protobuf.MapEntry
qprofilesPerLanguage = QprofilesPerLanguageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
output.writeMessage(6, qprofilesPerLanguage);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (analysisDate_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, analysisDate_);
}
if (!getProjectKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, projectKey_);
}
if (!getBranchBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, branch_);
}
if (rootComponentRef_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, rootComponentRef_);
}
if (crossProjectDuplicationActivated_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, crossProjectDuplicationActivated_);
}
for (java.util.Map.Entry entry
: internalGetQprofilesPerLanguage().getMap().entrySet()) {
com.google.protobuf.MapEntry
qprofilesPerLanguage = QprofilesPerLanguageDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, qprofilesPerLanguage);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Metadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Metadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Metadata)
org.sonar.scanner.protocol.output.ScannerReport.MetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetQprofilesPerLanguage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 6:
return internalGetMutableQprofilesPerLanguage();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Metadata.class, org.sonar.scanner.protocol.output.ScannerReport.Metadata.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Metadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
analysisDate_ = 0L;
projectKey_ = "";
branch_ = "";
rootComponentRef_ = 0;
crossProjectDuplicationActivated_ = false;
internalGetMutableQprofilesPerLanguage().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Metadata_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Metadata.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata build() {
org.sonar.scanner.protocol.output.ScannerReport.Metadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Metadata result = new org.sonar.scanner.protocol.output.ScannerReport.Metadata(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.analysisDate_ = analysisDate_;
result.projectKey_ = projectKey_;
result.branch_ = branch_;
result.rootComponentRef_ = rootComponentRef_;
result.crossProjectDuplicationActivated_ = crossProjectDuplicationActivated_;
result.qprofilesPerLanguage_ = internalGetQprofilesPerLanguage();
result.qprofilesPerLanguage_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Metadata) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Metadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Metadata other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Metadata.getDefaultInstance()) return this;
if (other.getAnalysisDate() != 0L) {
setAnalysisDate(other.getAnalysisDate());
}
if (!other.getProjectKey().isEmpty()) {
projectKey_ = other.projectKey_;
onChanged();
}
if (!other.getBranch().isEmpty()) {
branch_ = other.branch_;
onChanged();
}
if (other.getRootComponentRef() != 0) {
setRootComponentRef(other.getRootComponentRef());
}
if (other.getCrossProjectDuplicationActivated() != false) {
setCrossProjectDuplicationActivated(other.getCrossProjectDuplicationActivated());
}
internalGetMutableQprofilesPerLanguage().mergeFrom(
other.internalGetQprofilesPerLanguage());
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Metadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Metadata) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long analysisDate_ ;
/**
* optional int64 analysis_date = 1;
*/
public long getAnalysisDate() {
return analysisDate_;
}
/**
* optional int64 analysis_date = 1;
*/
public Builder setAnalysisDate(long value) {
analysisDate_ = value;
onChanged();
return this;
}
/**
* optional int64 analysis_date = 1;
*/
public Builder clearAnalysisDate() {
analysisDate_ = 0L;
onChanged();
return this;
}
private java.lang.Object projectKey_ = "";
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public java.lang.String getProjectKey() {
java.lang.Object ref = projectKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
projectKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public com.google.protobuf.ByteString
getProjectKeyBytes() {
java.lang.Object ref = projectKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public Builder setProjectKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
projectKey_ = value;
onChanged();
return this;
}
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public Builder clearProjectKey() {
projectKey_ = getDefaultInstance().getProjectKey();
onChanged();
return this;
}
/**
* optional string project_key = 2;
*
*
* TODO should we keep this project_key here or not ? Because it's a duplication of Component.key
*
*/
public Builder setProjectKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
projectKey_ = value;
onChanged();
return this;
}
private java.lang.Object branch_ = "";
/**
* optional string branch = 3;
*/
public java.lang.String getBranch() {
java.lang.Object ref = branch_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
branch_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string branch = 3;
*/
public com.google.protobuf.ByteString
getBranchBytes() {
java.lang.Object ref = branch_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
branch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string branch = 3;
*/
public Builder setBranch(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
branch_ = value;
onChanged();
return this;
}
/**
* optional string branch = 3;
*/
public Builder clearBranch() {
branch_ = getDefaultInstance().getBranch();
onChanged();
return this;
}
/**
* optional string branch = 3;
*/
public Builder setBranchBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
branch_ = value;
onChanged();
return this;
}
private int rootComponentRef_ ;
/**
* optional int32 root_component_ref = 4;
*/
public int getRootComponentRef() {
return rootComponentRef_;
}
/**
* optional int32 root_component_ref = 4;
*/
public Builder setRootComponentRef(int value) {
rootComponentRef_ = value;
onChanged();
return this;
}
/**
* optional int32 root_component_ref = 4;
*/
public Builder clearRootComponentRef() {
rootComponentRef_ = 0;
onChanged();
return this;
}
private boolean crossProjectDuplicationActivated_ ;
/**
* optional bool cross_project_duplication_activated = 5;
*/
public boolean getCrossProjectDuplicationActivated() {
return crossProjectDuplicationActivated_;
}
/**
* optional bool cross_project_duplication_activated = 5;
*/
public Builder setCrossProjectDuplicationActivated(boolean value) {
crossProjectDuplicationActivated_ = value;
onChanged();
return this;
}
/**
* optional bool cross_project_duplication_activated = 5;
*/
public Builder clearCrossProjectDuplicationActivated() {
crossProjectDuplicationActivated_ = false;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, org.sonar.scanner.protocol.output.ScannerReport.Metadata.QProfile> qprofilesPerLanguage_;
private com.google.protobuf.MapField
internalGetQprofilesPerLanguage() {
if (qprofilesPerLanguage_ == null) {
return com.google.protobuf.MapField.emptyMapField(
QprofilesPerLanguageDefaultEntryHolder.defaultEntry);
}
return qprofilesPerLanguage_;
}
private com.google.protobuf.MapField
internalGetMutableQprofilesPerLanguage() {
onChanged();;
if (qprofilesPerLanguage_ == null) {
qprofilesPerLanguage_ = com.google.protobuf.MapField.newMapField(
QprofilesPerLanguageDefaultEntryHolder.defaultEntry);
}
if (!qprofilesPerLanguage_.isMutable()) {
qprofilesPerLanguage_ = qprofilesPerLanguage_.copy();
}
return qprofilesPerLanguage_;
}
/**
* map<string, .Metadata.QProfile> qprofiles_per_language = 6;
*/
public java.util.Map getQprofilesPerLanguage() {
return internalGetQprofilesPerLanguage().getMap();
}
/**
* map<string, .Metadata.QProfile> qprofiles_per_language = 6;
*/
public java.util.Map
getMutableQprofilesPerLanguage() {
return internalGetMutableQprofilesPerLanguage().getMutableMap();
}
/**
* map<string, .Metadata.QProfile> qprofiles_per_language = 6;
*/
public Builder putAllQprofilesPerLanguage(
java.util.Map values) {
getMutableQprofilesPerLanguage().putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Metadata)
}
// @@protoc_insertion_point(class_scope:Metadata)
private static final org.sonar.scanner.protocol.output.ScannerReport.Metadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Metadata();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Metadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Metadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Metadata(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Metadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ActiveRuleOrBuilder extends
// @@protoc_insertion_point(interface_extends:ActiveRule)
com.google.protobuf.MessageOrBuilder {
/**
* optional string rule_repository = 1;
*/
java.lang.String getRuleRepository();
/**
* optional string rule_repository = 1;
*/
com.google.protobuf.ByteString
getRuleRepositoryBytes();
/**
* optional string rule_key = 2;
*/
java.lang.String getRuleKey();
/**
* optional string rule_key = 2;
*/
com.google.protobuf.ByteString
getRuleKeyBytes();
/**
* optional .Severity severity = 3;
*/
int getSeverityValue();
/**
* optional .Severity severity = 3;
*/
org.sonar.scanner.protocol.Constants.Severity getSeverity();
/**
* map<string, string> params_by_key = 4;
*/
java.util.Map
getParamsByKey();
}
/**
* Protobuf type {@code ActiveRule}
*/
public static final class ActiveRule extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:ActiveRule)
ActiveRuleOrBuilder {
// Use ActiveRule.newBuilder() to construct.
private ActiveRule(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ActiveRule() {
ruleRepository_ = "";
ruleKey_ = "";
severity_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ActiveRule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ruleRepository_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
ruleKey_ = s;
break;
}
case 24: {
int rawValue = input.readEnum();
severity_ = rawValue;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
paramsByKey_ = com.google.protobuf.MapField.newMapField(
ParamsByKeyDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000008;
}
com.google.protobuf.MapEntry
paramsByKey = input.readMessage(
ParamsByKeyDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
paramsByKey_.getMutableMap().put(paramsByKey.getKey(), paramsByKey.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetParamsByKey();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.class, org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.Builder.class);
}
private int bitField0_;
public static final int RULE_REPOSITORY_FIELD_NUMBER = 1;
private volatile java.lang.Object ruleRepository_;
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleRepository_ = s;
return s;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RULE_KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object ruleKey_;
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleKey_ = s;
return s;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEVERITY_FIELD_NUMBER = 3;
private int severity_;
/**
* optional .Severity severity = 3;
*/
public int getSeverityValue() {
return severity_;
}
/**
* optional .Severity severity = 3;
*/
public org.sonar.scanner.protocol.Constants.Severity getSeverity() {
org.sonar.scanner.protocol.Constants.Severity result = org.sonar.scanner.protocol.Constants.Severity.valueOf(severity_);
return result == null ? org.sonar.scanner.protocol.Constants.Severity.UNRECOGNIZED : result;
}
public static final int PARAMS_BY_KEY_FIELD_NUMBER = 4;
private static final class ParamsByKeyDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_ParamsByKeyEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> paramsByKey_;
private com.google.protobuf.MapField
internalGetParamsByKey() {
if (paramsByKey_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ParamsByKeyDefaultEntryHolder.defaultEntry);
}
return paramsByKey_;
}
/**
* map<string, string> params_by_key = 4;
*/
public java.util.Map getParamsByKey() {
return internalGetParamsByKey().getMap();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRuleRepositoryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, ruleRepository_);
}
if (!getRuleKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, ruleKey_);
}
if (severity_ != org.sonar.scanner.protocol.Constants.Severity.UNSET_SEVERITY.getNumber()) {
output.writeEnum(3, severity_);
}
for (java.util.Map.Entry entry
: internalGetParamsByKey().getMap().entrySet()) {
com.google.protobuf.MapEntry
paramsByKey = ParamsByKeyDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
output.writeMessage(4, paramsByKey);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRuleRepositoryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, ruleRepository_);
}
if (!getRuleKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, ruleKey_);
}
if (severity_ != org.sonar.scanner.protocol.Constants.Severity.UNSET_SEVERITY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, severity_);
}
for (java.util.Map.Entry entry
: internalGetParamsByKey().getMap().entrySet()) {
com.google.protobuf.MapEntry
paramsByKey = ParamsByKeyDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, paramsByKey);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.ActiveRule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ActiveRule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:ActiveRule)
org.sonar.scanner.protocol.output.ScannerReport.ActiveRuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetParamsByKey();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 4:
return internalGetMutableParamsByKey();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.class, org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
ruleRepository_ = "";
ruleKey_ = "";
severity_ = 0;
internalGetMutableParamsByKey().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ActiveRule_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.ActiveRule getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.ActiveRule build() {
org.sonar.scanner.protocol.output.ScannerReport.ActiveRule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.ActiveRule buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.ActiveRule result = new org.sonar.scanner.protocol.output.ScannerReport.ActiveRule(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.ruleRepository_ = ruleRepository_;
result.ruleKey_ = ruleKey_;
result.severity_ = severity_;
result.paramsByKey_ = internalGetParamsByKey();
result.paramsByKey_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.ActiveRule) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.ActiveRule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.ActiveRule other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.ActiveRule.getDefaultInstance()) return this;
if (!other.getRuleRepository().isEmpty()) {
ruleRepository_ = other.ruleRepository_;
onChanged();
}
if (!other.getRuleKey().isEmpty()) {
ruleKey_ = other.ruleKey_;
onChanged();
}
if (other.severity_ != 0) {
setSeverityValue(other.getSeverityValue());
}
internalGetMutableParamsByKey().mergeFrom(
other.internalGetParamsByKey());
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.ActiveRule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.ActiveRule) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object ruleRepository_ = "";
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleRepository_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepository(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ruleRepository_ = value;
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder clearRuleRepository() {
ruleRepository_ = getDefaultInstance().getRuleRepository();
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepositoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ruleRepository_ = value;
onChanged();
return this;
}
private java.lang.Object ruleKey_ = "";
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ruleKey_ = value;
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder clearRuleKey() {
ruleKey_ = getDefaultInstance().getRuleKey();
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ruleKey_ = value;
onChanged();
return this;
}
private int severity_ = 0;
/**
* optional .Severity severity = 3;
*/
public int getSeverityValue() {
return severity_;
}
/**
* optional .Severity severity = 3;
*/
public Builder setSeverityValue(int value) {
severity_ = value;
onChanged();
return this;
}
/**
* optional .Severity severity = 3;
*/
public org.sonar.scanner.protocol.Constants.Severity getSeverity() {
org.sonar.scanner.protocol.Constants.Severity result = org.sonar.scanner.protocol.Constants.Severity.valueOf(severity_);
return result == null ? org.sonar.scanner.protocol.Constants.Severity.UNRECOGNIZED : result;
}
/**
* optional .Severity severity = 3;
*/
public Builder setSeverity(org.sonar.scanner.protocol.Constants.Severity value) {
if (value == null) {
throw new NullPointerException();
}
severity_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .Severity severity = 3;
*/
public Builder clearSeverity() {
severity_ = 0;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> paramsByKey_;
private com.google.protobuf.MapField
internalGetParamsByKey() {
if (paramsByKey_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ParamsByKeyDefaultEntryHolder.defaultEntry);
}
return paramsByKey_;
}
private com.google.protobuf.MapField
internalGetMutableParamsByKey() {
onChanged();;
if (paramsByKey_ == null) {
paramsByKey_ = com.google.protobuf.MapField.newMapField(
ParamsByKeyDefaultEntryHolder.defaultEntry);
}
if (!paramsByKey_.isMutable()) {
paramsByKey_ = paramsByKey_.copy();
}
return paramsByKey_;
}
/**
* map<string, string> params_by_key = 4;
*/
public java.util.Map getParamsByKey() {
return internalGetParamsByKey().getMap();
}
/**
* map<string, string> params_by_key = 4;
*/
public java.util.Map
getMutableParamsByKey() {
return internalGetMutableParamsByKey().getMutableMap();
}
/**
* map<string, string> params_by_key = 4;
*/
public Builder putAllParamsByKey(
java.util.Map values) {
getMutableParamsByKey().putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:ActiveRule)
}
// @@protoc_insertion_point(class_scope:ActiveRule)
private static final org.sonar.scanner.protocol.output.ScannerReport.ActiveRule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.ActiveRule();
}
public static org.sonar.scanner.protocol.output.ScannerReport.ActiveRule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ActiveRule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ActiveRule(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.ActiveRule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ComponentLinkOrBuilder extends
// @@protoc_insertion_point(interface_extends:ComponentLink)
com.google.protobuf.MessageOrBuilder {
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
int getTypeValue();
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType getType();
/**
* optional string href = 2;
*/
java.lang.String getHref();
/**
* optional string href = 2;
*/
com.google.protobuf.ByteString
getHrefBytes();
}
/**
* Protobuf type {@code ComponentLink}
*/
public static final class ComponentLink extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:ComponentLink)
ComponentLinkOrBuilder {
// Use ComponentLink.newBuilder() to construct.
private ComponentLink(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ComponentLink() {
type_ = 0;
href_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ComponentLink(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
href_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ComponentLink_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ComponentLink_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.class, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder.class);
}
/**
* Protobuf enum {@code ComponentLink.ComponentLinkType}
*/
public enum ComponentLinkType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNSET = 0;
*/
UNSET(0, 0),
/**
* HOME = 1;
*/
HOME(1, 1),
/**
* SCM = 2;
*/
SCM(2, 2),
/**
* SCM_DEV = 3;
*/
SCM_DEV(3, 3),
/**
* ISSUE = 4;
*/
ISSUE(4, 4),
/**
* CI = 5;
*/
CI(5, 5),
UNRECOGNIZED(-1, -1),
;
/**
* UNSET = 0;
*/
public static final int UNSET_VALUE = 0;
/**
* HOME = 1;
*/
public static final int HOME_VALUE = 1;
/**
* SCM = 2;
*/
public static final int SCM_VALUE = 2;
/**
* SCM_DEV = 3;
*/
public static final int SCM_DEV_VALUE = 3;
/**
* ISSUE = 4;
*/
public static final int ISSUE_VALUE = 4;
/**
* CI = 5;
*/
public static final int CI_VALUE = 5;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
public static ComponentLinkType valueOf(int value) {
switch (value) {
case 0: return UNSET;
case 1: return HOME;
case 2: return SCM;
case 3: return SCM_DEV;
case 4: return ISSUE;
case 5: return CI;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ComponentLinkType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ComponentLinkType findValueByNumber(int number) {
return ComponentLinkType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.getDescriptor().getEnumTypes().get(0);
}
private static final ComponentLinkType[] VALUES = values();
public static ComponentLinkType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ComponentLinkType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ComponentLink.ComponentLinkType)
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType getType() {
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType result = org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.UNRECOGNIZED : result;
}
public static final int HREF_FIELD_NUMBER = 2;
private volatile java.lang.Object href_;
/**
* optional string href = 2;
*/
public java.lang.String getHref() {
java.lang.Object ref = href_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
href_ = s;
return s;
}
}
/**
* optional string href = 2;
*/
public com.google.protobuf.ByteString
getHrefBytes() {
java.lang.Object ref = href_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
href_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.UNSET.getNumber()) {
output.writeEnum(1, type_);
}
if (!getHrefBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, href_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (!getHrefBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, href_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.ComponentLink prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ComponentLink}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:ComponentLink)
org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ComponentLink_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ComponentLink_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.class, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
type_ = 0;
href_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_ComponentLink_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink build() {
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink result = new org.sonar.scanner.protocol.output.ScannerReport.ComponentLink(this);
result.type_ = type_;
result.href_ = href_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.ComponentLink) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.ComponentLink)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.ComponentLink other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (!other.getHref().isEmpty()) {
href_ = other.href_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.ComponentLink) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int type_ = 0;
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType getType() {
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType result = org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType.UNRECOGNIZED : result;
}
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public Builder setType(org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.ComponentLinkType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .ComponentLink.ComponentLinkType type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.lang.Object href_ = "";
/**
* optional string href = 2;
*/
public java.lang.String getHref() {
java.lang.Object ref = href_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
href_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string href = 2;
*/
public com.google.protobuf.ByteString
getHrefBytes() {
java.lang.Object ref = href_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
href_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string href = 2;
*/
public Builder setHref(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
href_ = value;
onChanged();
return this;
}
/**
* optional string href = 2;
*/
public Builder clearHref() {
href_ = getDefaultInstance().getHref();
onChanged();
return this;
}
/**
* optional string href = 2;
*/
public Builder setHrefBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
href_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:ComponentLink)
}
// @@protoc_insertion_point(class_scope:ComponentLink)
private static final org.sonar.scanner.protocol.output.ScannerReport.ComponentLink DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.ComponentLink();
}
public static org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ComponentLink parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ComponentLink(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ComponentOrBuilder extends
// @@protoc_insertion_point(interface_extends:Component)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 ref = 1;
*/
int getRef();
/**
* optional string path = 2;
*/
java.lang.String getPath();
/**
* optional string path = 2;
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* optional string name = 3;
*/
java.lang.String getName();
/**
* optional string name = 3;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional .Component.ComponentType type = 4;
*/
int getTypeValue();
/**
* optional .Component.ComponentType type = 4;
*/
org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType getType();
/**
* optional bool is_test = 5;
*/
boolean getIsTest();
/**
* optional string language = 6;
*/
java.lang.String getLanguage();
/**
* optional string language = 6;
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
* repeated int32 child_ref = 7 [packed = true];
*/
java.util.List getChildRefList();
/**
* repeated int32 child_ref = 7 [packed = true];
*/
int getChildRefCount();
/**
* repeated int32 child_ref = 7 [packed = true];
*/
int getChildRef(int index);
/**
* repeated .ComponentLink link = 8;
*/
java.util.List
getLinkList();
/**
* repeated .ComponentLink link = 8;
*/
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getLink(int index);
/**
* repeated .ComponentLink link = 8;
*/
int getLinkCount();
/**
* repeated .ComponentLink link = 8;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder>
getLinkOrBuilderList();
/**
* repeated .ComponentLink link = 8;
*/
org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder getLinkOrBuilder(
int index);
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
java.lang.String getVersion();
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
java.lang.String getKey();
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional int32 lines = 11;
*
*
* Only available on FILE type
*
*/
int getLines();
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
java.lang.String getDescription();
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code Component}
*/
public static final class Component extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Component)
ComponentOrBuilder {
// Use Component.newBuilder() to construct.
private Component(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Component() {
ref_ = 0;
path_ = "";
name_ = "";
type_ = 0;
isTest_ = false;
language_ = "";
childRef_ = java.util.Collections.emptyList();
link_ = java.util.Collections.emptyList();
version_ = "";
key_ = "";
lines_ = 0;
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Component(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
ref_ = input.readInt32();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 32: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 40: {
isTest_ = input.readBool();
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
language_ = s;
break;
}
case 56: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
childRef_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
childRef_.add(input.readInt32());
break;
}
case 58: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040) && input.getBytesUntilLimit() > 0) {
childRef_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
while (input.getBytesUntilLimit() > 0) {
childRef_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
link_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
link_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.parser(), extensionRegistry));
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
version_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 88: {
lines_ = input.readInt32();
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
childRef_ = java.util.Collections.unmodifiableList(childRef_);
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
link_ = java.util.Collections.unmodifiableList(link_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Component_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Component_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Component.class, org.sonar.scanner.protocol.output.ScannerReport.Component.Builder.class);
}
/**
* Protobuf enum {@code Component.ComponentType}
*/
public enum ComponentType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNSET = 0;
*/
UNSET(0, 0),
/**
* PROJECT = 1;
*/
PROJECT(1, 1),
/**
* MODULE = 2;
*/
MODULE(2, 2),
/**
* DIRECTORY = 3;
*/
DIRECTORY(3, 3),
/**
* FILE = 4;
*/
FILE(4, 4),
UNRECOGNIZED(-1, -1),
;
/**
* UNSET = 0;
*/
public static final int UNSET_VALUE = 0;
/**
* PROJECT = 1;
*/
public static final int PROJECT_VALUE = 1;
/**
* MODULE = 2;
*/
public static final int MODULE_VALUE = 2;
/**
* DIRECTORY = 3;
*/
public static final int DIRECTORY_VALUE = 3;
/**
* FILE = 4;
*/
public static final int FILE_VALUE = 4;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
public static ComponentType valueOf(int value) {
switch (value) {
case 0: return UNSET;
case 1: return PROJECT;
case 2: return MODULE;
case 3: return DIRECTORY;
case 4: return FILE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ComponentType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ComponentType findValueByNumber(int number) {
return ComponentType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.Component.getDescriptor().getEnumTypes().get(0);
}
private static final ComponentType[] VALUES = values();
public static ComponentType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ComponentType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:Component.ComponentType)
}
private int bitField0_;
public static final int REF_FIELD_NUMBER = 1;
private int ref_;
/**
* optional int32 ref = 1;
*/
public int getRef() {
return ref_;
}
public static final int PATH_FIELD_NUMBER = 2;
private volatile java.lang.Object path_;
/**
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object name_;
/**
* optional string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 4;
private int type_;
/**
* optional .Component.ComponentType type = 4;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .Component.ComponentType type = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType getType() {
org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType result = org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.UNRECOGNIZED : result;
}
public static final int IS_TEST_FIELD_NUMBER = 5;
private boolean isTest_;
/**
* optional bool is_test = 5;
*/
public boolean getIsTest() {
return isTest_;
}
public static final int LANGUAGE_FIELD_NUMBER = 6;
private volatile java.lang.Object language_;
/**
* optional string language = 6;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
}
}
/**
* optional string language = 6;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHILD_REF_FIELD_NUMBER = 7;
private java.util.List childRef_;
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public java.util.List
getChildRefList() {
return childRef_;
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public int getChildRefCount() {
return childRef_.size();
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public int getChildRef(int index) {
return childRef_.get(index);
}
private int childRefMemoizedSerializedSize = -1;
public static final int LINK_FIELD_NUMBER = 8;
private java.util.List link_;
/**
* repeated .ComponentLink link = 8;
*/
public java.util.List getLinkList() {
return link_;
}
/**
* repeated .ComponentLink link = 8;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder>
getLinkOrBuilderList() {
return link_;
}
/**
* repeated .ComponentLink link = 8;
*/
public int getLinkCount() {
return link_.size();
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getLink(int index) {
return link_.get(index);
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder getLinkOrBuilder(
int index) {
return link_.get(index);
}
public static final int VERSION_FIELD_NUMBER = 9;
private volatile java.lang.Object version_;
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_FIELD_NUMBER = 10;
private volatile java.lang.Object key_;
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LINES_FIELD_NUMBER = 11;
private int lines_;
/**
* optional int32 lines = 11;
*
*
* Only available on FILE type
*
*/
public int getLines() {
return lines_;
}
public static final int DESCRIPTION_FIELD_NUMBER = 12;
private volatile java.lang.Object description_;
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (ref_ != 0) {
output.writeInt32(1, ref_);
}
if (!getPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, path_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, name_);
}
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.UNSET.getNumber()) {
output.writeEnum(4, type_);
}
if (isTest_ != false) {
output.writeBool(5, isTest_);
}
if (!getLanguageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, language_);
}
if (getChildRefList().size() > 0) {
output.writeRawVarint32(58);
output.writeRawVarint32(childRefMemoizedSerializedSize);
}
for (int i = 0; i < childRef_.size(); i++) {
output.writeInt32NoTag(childRef_.get(i));
}
for (int i = 0; i < link_.size(); i++) {
output.writeMessage(8, link_.get(i));
}
if (!getVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, version_);
}
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, key_);
}
if (lines_ != 0) {
output.writeInt32(11, lines_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 12, description_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (ref_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, ref_);
}
if (!getPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, path_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, name_);
}
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, type_);
}
if (isTest_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isTest_);
}
if (!getLanguageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, language_);
}
{
int dataSize = 0;
for (int i = 0; i < childRef_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(childRef_.get(i));
}
size += dataSize;
if (!getChildRefList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
childRefMemoizedSerializedSize = dataSize;
}
for (int i = 0; i < link_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, link_.get(i));
}
if (!getVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(9, version_);
}
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, key_);
}
if (lines_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, lines_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(12, description_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Component prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Component}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Component)
org.sonar.scanner.protocol.output.ScannerReport.ComponentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Component_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Component_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Component.class, org.sonar.scanner.protocol.output.ScannerReport.Component.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Component.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getLinkFieldBuilder();
}
}
public Builder clear() {
super.clear();
ref_ = 0;
path_ = "";
name_ = "";
type_ = 0;
isTest_ = false;
language_ = "";
childRef_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
if (linkBuilder_ == null) {
link_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
linkBuilder_.clear();
}
version_ = "";
key_ = "";
lines_ = 0;
description_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Component_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Component getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Component.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Component build() {
org.sonar.scanner.protocol.output.ScannerReport.Component result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Component buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Component result = new org.sonar.scanner.protocol.output.ScannerReport.Component(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.ref_ = ref_;
result.path_ = path_;
result.name_ = name_;
result.type_ = type_;
result.isTest_ = isTest_;
result.language_ = language_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
childRef_ = java.util.Collections.unmodifiableList(childRef_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.childRef_ = childRef_;
if (linkBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
link_ = java.util.Collections.unmodifiableList(link_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.link_ = link_;
} else {
result.link_ = linkBuilder_.build();
}
result.version_ = version_;
result.key_ = key_;
result.lines_ = lines_;
result.description_ = description_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Component) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Component)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Component other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Component.getDefaultInstance()) return this;
if (other.getRef() != 0) {
setRef(other.getRef());
}
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getIsTest() != false) {
setIsTest(other.getIsTest());
}
if (!other.getLanguage().isEmpty()) {
language_ = other.language_;
onChanged();
}
if (!other.childRef_.isEmpty()) {
if (childRef_.isEmpty()) {
childRef_ = other.childRef_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureChildRefIsMutable();
childRef_.addAll(other.childRef_);
}
onChanged();
}
if (linkBuilder_ == null) {
if (!other.link_.isEmpty()) {
if (link_.isEmpty()) {
link_ = other.link_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureLinkIsMutable();
link_.addAll(other.link_);
}
onChanged();
}
} else {
if (!other.link_.isEmpty()) {
if (linkBuilder_.isEmpty()) {
linkBuilder_.dispose();
linkBuilder_ = null;
link_ = other.link_;
bitField0_ = (bitField0_ & ~0x00000080);
linkBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getLinkFieldBuilder() : null;
} else {
linkBuilder_.addAllMessages(other.link_);
}
}
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
onChanged();
}
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (other.getLines() != 0) {
setLines(other.getLines());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Component parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Component) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int ref_ ;
/**
* optional int32 ref = 1;
*/
public int getRef() {
return ref_;
}
/**
* optional int32 ref = 1;
*/
public Builder setRef(int value) {
ref_ = value;
onChanged();
return this;
}
/**
* optional int32 ref = 1;
*/
public Builder clearRef() {
ref_ = 0;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
* optional string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string path = 2;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* optional string path = 2;
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* optional string path = 2;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 3;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 3;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 3;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
* optional .Component.ComponentType type = 4;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .Component.ComponentType type = 4;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* optional .Component.ComponentType type = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType getType() {
org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType result = org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType.UNRECOGNIZED : result;
}
/**
* optional .Component.ComponentType type = 4;
*/
public Builder setType(org.sonar.scanner.protocol.output.ScannerReport.Component.ComponentType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .Component.ComponentType type = 4;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private boolean isTest_ ;
/**
* optional bool is_test = 5;
*/
public boolean getIsTest() {
return isTest_;
}
/**
* optional bool is_test = 5;
*/
public Builder setIsTest(boolean value) {
isTest_ = value;
onChanged();
return this;
}
/**
* optional bool is_test = 5;
*/
public Builder clearIsTest() {
isTest_ = false;
onChanged();
return this;
}
private java.lang.Object language_ = "";
/**
* optional string language = 6;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language = 6;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language = 6;
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
language_ = value;
onChanged();
return this;
}
/**
* optional string language = 6;
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* optional string language = 6;
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
language_ = value;
onChanged();
return this;
}
private java.util.List childRef_ = java.util.Collections.emptyList();
private void ensureChildRefIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
childRef_ = new java.util.ArrayList(childRef_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public java.util.List
getChildRefList() {
return java.util.Collections.unmodifiableList(childRef_);
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public int getChildRefCount() {
return childRef_.size();
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public int getChildRef(int index) {
return childRef_.get(index);
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public Builder setChildRef(
int index, int value) {
ensureChildRefIsMutable();
childRef_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public Builder addChildRef(int value) {
ensureChildRefIsMutable();
childRef_.add(value);
onChanged();
return this;
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public Builder addAllChildRef(
java.lang.Iterable extends java.lang.Integer> values) {
ensureChildRefIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, childRef_);
onChanged();
return this;
}
/**
* repeated int32 child_ref = 7 [packed = true];
*/
public Builder clearChildRef() {
childRef_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
private java.util.List link_ =
java.util.Collections.emptyList();
private void ensureLinkIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
link_ = new java.util.ArrayList(link_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder, org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder> linkBuilder_;
/**
* repeated .ComponentLink link = 8;
*/
public java.util.List getLinkList() {
if (linkBuilder_ == null) {
return java.util.Collections.unmodifiableList(link_);
} else {
return linkBuilder_.getMessageList();
}
}
/**
* repeated .ComponentLink link = 8;
*/
public int getLinkCount() {
if (linkBuilder_ == null) {
return link_.size();
} else {
return linkBuilder_.getCount();
}
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink getLink(int index) {
if (linkBuilder_ == null) {
return link_.get(index);
} else {
return linkBuilder_.getMessage(index);
}
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder setLink(
int index, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink value) {
if (linkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLinkIsMutable();
link_.set(index, value);
onChanged();
} else {
linkBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder setLink(
int index, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder builderForValue) {
if (linkBuilder_ == null) {
ensureLinkIsMutable();
link_.set(index, builderForValue.build());
onChanged();
} else {
linkBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder addLink(org.sonar.scanner.protocol.output.ScannerReport.ComponentLink value) {
if (linkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLinkIsMutable();
link_.add(value);
onChanged();
} else {
linkBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder addLink(
int index, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink value) {
if (linkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLinkIsMutable();
link_.add(index, value);
onChanged();
} else {
linkBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder addLink(
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder builderForValue) {
if (linkBuilder_ == null) {
ensureLinkIsMutable();
link_.add(builderForValue.build());
onChanged();
} else {
linkBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder addLink(
int index, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder builderForValue) {
if (linkBuilder_ == null) {
ensureLinkIsMutable();
link_.add(index, builderForValue.build());
onChanged();
} else {
linkBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder addAllLink(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.ComponentLink> values) {
if (linkBuilder_ == null) {
ensureLinkIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, link_);
onChanged();
} else {
linkBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder clearLink() {
if (linkBuilder_ == null) {
link_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
linkBuilder_.clear();
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public Builder removeLink(int index) {
if (linkBuilder_ == null) {
ensureLinkIsMutable();
link_.remove(index);
onChanged();
} else {
linkBuilder_.remove(index);
}
return this;
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder getLinkBuilder(
int index) {
return getLinkFieldBuilder().getBuilder(index);
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder getLinkOrBuilder(
int index) {
if (linkBuilder_ == null) {
return link_.get(index); } else {
return linkBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ComponentLink link = 8;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder>
getLinkOrBuilderList() {
if (linkBuilder_ != null) {
return linkBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(link_);
}
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder addLinkBuilder() {
return getLinkFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.getDefaultInstance());
}
/**
* repeated .ComponentLink link = 8;
*/
public org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder addLinkBuilder(
int index) {
return getLinkFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.getDefaultInstance());
}
/**
* repeated .ComponentLink link = 8;
*/
public java.util.List
getLinkBuilderList() {
return getLinkFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder, org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder>
getLinkFieldBuilder() {
if (linkBuilder_ == null) {
linkBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.ComponentLink, org.sonar.scanner.protocol.output.ScannerReport.ComponentLink.Builder, org.sonar.scanner.protocol.output.ScannerReport.ComponentLinkOrBuilder>(
link_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
link_ = null;
}
return linkBuilder_;
}
private java.lang.Object version_ = "";
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
return this;
}
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
* optional string version = 9;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
version_ = value;
onChanged();
return this;
}
private java.lang.Object key_ = "";
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 10;
*
*
* Only available on PROJECT and MODULE types
* TODO rename this property -> moduleKey ?
*
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private int lines_ ;
/**
* optional int32 lines = 11;
*
*
* Only available on FILE type
*
*/
public int getLines() {
return lines_;
}
/**
* optional int32 lines = 11;
*
*
* Only available on FILE type
*
*/
public Builder setLines(int value) {
lines_ = value;
onChanged();
return this;
}
/**
* optional int32 lines = 11;
*
*
* Only available on FILE type
*
*/
public Builder clearLines() {
lines_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* optional string description = 12;
*
*
* Only available on PROJECT and MODULE types
*
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Component)
}
// @@protoc_insertion_point(class_scope:Component)
private static final org.sonar.scanner.protocol.output.ScannerReport.Component DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Component();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Component getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Component parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Component(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Component getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MeasureOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure)
com.google.protobuf.MessageOrBuilder {
/**
* optional string metric_key = 1;
*/
java.lang.String getMetricKey();
/**
* optional string metric_key = 1;
*/
com.google.protobuf.ByteString
getMetricKeyBytes();
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getBooleanValue();
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder getBooleanValueOrBuilder();
/**
* optional .Measure.IntValue int_value = 3;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getIntValue();
/**
* optional .Measure.IntValue int_value = 3;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder getIntValueOrBuilder();
/**
* optional .Measure.LongValue long_value = 4;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getLongValue();
/**
* optional .Measure.LongValue long_value = 4;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder getLongValueOrBuilder();
/**
* optional .Measure.DoubleValue double_value = 5;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDoubleValue();
/**
* optional .Measure.DoubleValue double_value = 5;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder getDoubleValueOrBuilder();
/**
* optional .Measure.StringValue string_value = 6;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getStringValue();
/**
* optional .Measure.StringValue string_value = 6;
*/
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder getStringValueOrBuilder();
public org.sonar.scanner.protocol.output.ScannerReport.Measure.ValueCase getValueCase();
}
/**
* Protobuf type {@code Measure}
*/
public static final class Measure extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure)
MeasureOrBuilder {
// Use Measure.newBuilder() to construct.
private Measure(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Measure() {
metricKey_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Measure(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
metricKey_ = s;
break;
}
case 18: {
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder subBuilder = null;
if (valueCase_ == 2) {
subBuilder = ((org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_).toBuilder();
}
value_ =
input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 2;
break;
}
case 26: {
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder subBuilder = null;
if (valueCase_ == 3) {
subBuilder = ((org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_).toBuilder();
}
value_ =
input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 3;
break;
}
case 34: {
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder subBuilder = null;
if (valueCase_ == 4) {
subBuilder = ((org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_).toBuilder();
}
value_ =
input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 4;
break;
}
case 42: {
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder subBuilder = null;
if (valueCase_ == 5) {
subBuilder = ((org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_).toBuilder();
}
value_ =
input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 5;
break;
}
case 50: {
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder subBuilder = null;
if (valueCase_ == 6) {
subBuilder = ((org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_).toBuilder();
}
value_ =
input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_);
value_ = subBuilder.buildPartial();
}
valueCase_ = 6;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.Builder.class);
}
public interface BoolValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure.BoolValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional bool value = 1;
*/
boolean getValue();
/**
* optional string data = 2;
*/
java.lang.String getData();
/**
* optional string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code Measure.BoolValue}
*/
public static final class BoolValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure.BoolValue)
BoolValueOrBuilder {
// Use BoolValue.newBuilder() to construct.
private BoolValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private BoolValue() {
value_ = false;
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private BoolValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
value_ = input.readBool();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_BoolValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_BoolValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private boolean value_;
/**
* optional bool value = 1;
*/
public boolean getValue() {
return value_;
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (value_ != false) {
output.writeBool(1, value_);
}
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (value_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, value_);
}
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure.BoolValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure.BoolValue)
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_BoolValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_BoolValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = false;
data_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_BoolValue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue result = new org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue(this);
result.value_ = value_;
result.data_ = data_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance()) return this;
if (other.getValue() != false) {
setValue(other.getValue());
}
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean value_ ;
/**
* optional bool value = 1;
*/
public boolean getValue() {
return value_;
}
/**
* optional bool value = 1;
*/
public Builder setValue(boolean value) {
value_ = value;
onChanged();
return this;
}
/**
* optional bool value = 1;
*/
public Builder clearValue() {
value_ = false;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure.BoolValue)
}
// @@protoc_insertion_point(class_scope:Measure.BoolValue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BoolValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new BoolValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IntValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure.IntValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 value = 1;
*/
int getValue();
/**
* optional string data = 2;
*/
java.lang.String getData();
/**
* optional string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code Measure.IntValue}
*/
public static final class IntValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure.IntValue)
IntValueOrBuilder {
// Use IntValue.newBuilder() to construct.
private IntValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private IntValue() {
value_ = 0;
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private IntValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
value_ = input.readInt32();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_IntValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_IntValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private int value_;
/**
* optional int32 value = 1;
*/
public int getValue() {
return value_;
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (value_ != 0) {
output.writeInt32(1, value_);
}
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (value_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, value_);
}
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure.IntValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure.IntValue)
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_IntValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_IntValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = 0;
data_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_IntValue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue result = new org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue(this);
result.value_ = value_;
result.data_ = data_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance()) return this;
if (other.getValue() != 0) {
setValue(other.getValue());
}
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int value_ ;
/**
* optional int32 value = 1;
*/
public int getValue() {
return value_;
}
/**
* optional int32 value = 1;
*/
public Builder setValue(int value) {
value_ = value;
onChanged();
return this;
}
/**
* optional int32 value = 1;
*/
public Builder clearValue() {
value_ = 0;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure.IntValue)
}
// @@protoc_insertion_point(class_scope:Measure.IntValue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public IntValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new IntValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LongValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure.LongValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 value = 1;
*/
long getValue();
/**
* optional string data = 2;
*/
java.lang.String getData();
/**
* optional string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code Measure.LongValue}
*/
public static final class LongValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure.LongValue)
LongValueOrBuilder {
// Use LongValue.newBuilder() to construct.
private LongValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private LongValue() {
value_ = 0L;
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private LongValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
value_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_LongValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_LongValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private long value_;
/**
* optional int64 value = 1;
*/
public long getValue() {
return value_;
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (value_ != 0L) {
output.writeInt64(1, value_);
}
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (value_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, value_);
}
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure.LongValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure.LongValue)
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_LongValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_LongValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = 0L;
data_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_LongValue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue result = new org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue(this);
result.value_ = value_;
result.data_ = data_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance()) return this;
if (other.getValue() != 0L) {
setValue(other.getValue());
}
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long value_ ;
/**
* optional int64 value = 1;
*/
public long getValue() {
return value_;
}
/**
* optional int64 value = 1;
*/
public Builder setValue(long value) {
value_ = value;
onChanged();
return this;
}
/**
* optional int64 value = 1;
*/
public Builder clearValue() {
value_ = 0L;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure.LongValue)
}
// @@protoc_insertion_point(class_scope:Measure.LongValue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LongValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new LongValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DoubleValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure.DoubleValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional double value = 1;
*/
double getValue();
/**
* optional string data = 2;
*/
java.lang.String getData();
/**
* optional string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code Measure.DoubleValue}
*/
public static final class DoubleValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure.DoubleValue)
DoubleValueOrBuilder {
// Use DoubleValue.newBuilder() to construct.
private DoubleValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private DoubleValue() {
value_ = 0D;
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private DoubleValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 9: {
value_ = input.readDouble();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_DoubleValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_DoubleValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private double value_;
/**
* optional double value = 1;
*/
public double getValue() {
return value_;
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (value_ != 0D) {
output.writeDouble(1, value_);
}
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (value_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, value_);
}
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure.DoubleValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure.DoubleValue)
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_DoubleValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_DoubleValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = 0D;
data_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_DoubleValue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue result = new org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue(this);
result.value_ = value_;
result.data_ = data_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance()) return this;
if (other.getValue() != 0D) {
setValue(other.getValue());
}
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private double value_ ;
/**
* optional double value = 1;
*/
public double getValue() {
return value_;
}
/**
* optional double value = 1;
*/
public Builder setValue(double value) {
value_ = value;
onChanged();
return this;
}
/**
* optional double value = 1;
*/
public Builder clearValue() {
value_ = 0D;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure.DoubleValue)
}
// @@protoc_insertion_point(class_scope:Measure.DoubleValue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DoubleValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new DoubleValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StringValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Measure.StringValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional string value = 1;
*/
java.lang.String getValue();
/**
* optional string value = 1;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code Measure.StringValue}
*/
public static final class StringValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Measure.StringValue)
StringValueOrBuilder {
// Use StringValue.newBuilder() to construct.
private StringValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private StringValue() {
value_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private StringValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
value_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_StringValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_StringValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private volatile java.lang.Object value_;
/**
* optional string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* optional string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, value_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, value_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure.StringValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure.StringValue)
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_StringValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_StringValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
value_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_StringValue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue result = new org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue(this);
result.value_ = value_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance()) return this;
if (!other.getValue().isEmpty()) {
value_ = other.value_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object value_ = "";
/**
* optional string value = 1;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string value = 1;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string value = 1;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* optional string value = 1;
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* optional string value = 1;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
value_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure.StringValue)
}
// @@protoc_insertion_point(class_scope:Measure.StringValue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public StringValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new StringValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int valueCase_ = 0;
private java.lang.Object value_;
public enum ValueCase
implements com.google.protobuf.Internal.EnumLite {
BOOLEAN_VALUE(2),
INT_VALUE(3),
LONG_VALUE(4),
DOUBLE_VALUE(5),
STRING_VALUE(6),
VALUE_NOT_SET(0);
private int value = 0;
private ValueCase(int value) {
this.value = value;
}
public static ValueCase valueOf(int value) {
switch (value) {
case 2: return BOOLEAN_VALUE;
case 3: return INT_VALUE;
case 4: return LONG_VALUE;
case 5: return DOUBLE_VALUE;
case 6: return STRING_VALUE;
case 0: return VALUE_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public ValueCase
getValueCase() {
return ValueCase.valueOf(
valueCase_);
}
public static final int METRIC_KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object metricKey_;
/**
* optional string metric_key = 1;
*/
public java.lang.String getMetricKey() {
java.lang.Object ref = metricKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
metricKey_ = s;
return s;
}
}
/**
* optional string metric_key = 1;
*/
public com.google.protobuf.ByteString
getMetricKeyBytes() {
java.lang.Object ref = metricKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
metricKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BOOLEAN_VALUE_FIELD_NUMBER = 2;
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getBooleanValue() {
if (valueCase_ == 2) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder getBooleanValueOrBuilder() {
if (valueCase_ == 2) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
public static final int INT_VALUE_FIELD_NUMBER = 3;
/**
* optional .Measure.IntValue int_value = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getIntValue() {
if (valueCase_ == 3) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder getIntValueOrBuilder() {
if (valueCase_ == 3) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
public static final int LONG_VALUE_FIELD_NUMBER = 4;
/**
* optional .Measure.LongValue long_value = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getLongValue() {
if (valueCase_ == 4) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder getLongValueOrBuilder() {
if (valueCase_ == 4) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
public static final int DOUBLE_VALUE_FIELD_NUMBER = 5;
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDoubleValue() {
if (valueCase_ == 5) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder getDoubleValueOrBuilder() {
if (valueCase_ == 5) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
public static final int STRING_VALUE_FIELD_NUMBER = 6;
/**
* optional .Measure.StringValue string_value = 6;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getStringValue() {
if (valueCase_ == 6) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder getStringValueOrBuilder() {
if (valueCase_ == 6) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getMetricKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, metricKey_);
}
if (valueCase_ == 2) {
output.writeMessage(2, (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_);
}
if (valueCase_ == 3) {
output.writeMessage(3, (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_);
}
if (valueCase_ == 4) {
output.writeMessage(4, (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_);
}
if (valueCase_ == 5) {
output.writeMessage(5, (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_);
}
if (valueCase_ == 6) {
output.writeMessage(6, (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getMetricKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, metricKey_);
}
if (valueCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_);
}
if (valueCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_);
}
if (valueCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_);
}
if (valueCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_);
}
if (valueCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Measure prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Measure}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Measure)
org.sonar.scanner.protocol.output.ScannerReport.MeasureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Measure.class, org.sonar.scanner.protocol.output.ScannerReport.Measure.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Measure.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
metricKey_ = "";
valueCase_ = 0;
value_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Measure_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Measure.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure build() {
org.sonar.scanner.protocol.output.ScannerReport.Measure result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Measure result = new org.sonar.scanner.protocol.output.ScannerReport.Measure(this);
result.metricKey_ = metricKey_;
if (valueCase_ == 2) {
if (booleanValueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = booleanValueBuilder_.build();
}
}
if (valueCase_ == 3) {
if (intValueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = intValueBuilder_.build();
}
}
if (valueCase_ == 4) {
if (longValueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = longValueBuilder_.build();
}
}
if (valueCase_ == 5) {
if (doubleValueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = doubleValueBuilder_.build();
}
}
if (valueCase_ == 6) {
if (stringValueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = stringValueBuilder_.build();
}
}
result.valueCase_ = valueCase_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Measure) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Measure)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Measure other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Measure.getDefaultInstance()) return this;
if (!other.getMetricKey().isEmpty()) {
metricKey_ = other.metricKey_;
onChanged();
}
switch (other.getValueCase()) {
case BOOLEAN_VALUE: {
mergeBooleanValue(other.getBooleanValue());
break;
}
case INT_VALUE: {
mergeIntValue(other.getIntValue());
break;
}
case LONG_VALUE: {
mergeLongValue(other.getLongValue());
break;
}
case DOUBLE_VALUE: {
mergeDoubleValue(other.getDoubleValue());
break;
}
case STRING_VALUE: {
mergeStringValue(other.getStringValue());
break;
}
case VALUE_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Measure parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Measure) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int valueCase_ = 0;
private java.lang.Object value_;
public ValueCase
getValueCase() {
return ValueCase.valueOf(
valueCase_);
}
public Builder clearValue() {
valueCase_ = 0;
value_ = null;
onChanged();
return this;
}
private java.lang.Object metricKey_ = "";
/**
* optional string metric_key = 1;
*/
public java.lang.String getMetricKey() {
java.lang.Object ref = metricKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
metricKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string metric_key = 1;
*/
public com.google.protobuf.ByteString
getMetricKeyBytes() {
java.lang.Object ref = metricKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
metricKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string metric_key = 1;
*/
public Builder setMetricKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
metricKey_ = value;
onChanged();
return this;
}
/**
* optional string metric_key = 1;
*/
public Builder clearMetricKey() {
metricKey_ = getDefaultInstance().getMetricKey();
onChanged();
return this;
}
/**
* optional string metric_key = 1;
*/
public Builder setMetricKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
metricKey_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder> booleanValueBuilder_;
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue getBooleanValue() {
if (booleanValueBuilder_ == null) {
if (valueCase_ == 2) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
} else {
if (valueCase_ == 2) {
return booleanValueBuilder_.getMessage();
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public Builder setBooleanValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue value) {
if (booleanValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
booleanValueBuilder_.setMessage(value);
}
valueCase_ = 2;
return this;
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public Builder setBooleanValue(
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder builderForValue) {
if (booleanValueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
booleanValueBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 2;
return this;
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public Builder mergeBooleanValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue value) {
if (booleanValueBuilder_ == null) {
if (valueCase_ == 2 &&
value_ != org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance()) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.newBuilder((org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 2) {
booleanValueBuilder_.mergeFrom(value);
}
booleanValueBuilder_.setMessage(value);
}
valueCase_ = 2;
return this;
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public Builder clearBooleanValue() {
if (booleanValueBuilder_ == null) {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 2) {
valueCase_ = 0;
value_ = null;
}
booleanValueBuilder_.clear();
}
return this;
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder getBooleanValueBuilder() {
return getBooleanValueFieldBuilder().getBuilder();
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder getBooleanValueOrBuilder() {
if ((valueCase_ == 2) && (booleanValueBuilder_ != null)) {
return booleanValueBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 2) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
}
/**
* optional .Measure.BoolValue boolean_value = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder>
getBooleanValueFieldBuilder() {
if (booleanValueBuilder_ == null) {
if (!(valueCase_ == 2)) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.getDefaultInstance();
}
booleanValueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValueOrBuilder>(
(org.sonar.scanner.protocol.output.ScannerReport.Measure.BoolValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 2;
onChanged();;
return booleanValueBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder> intValueBuilder_;
/**
* optional .Measure.IntValue int_value = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue getIntValue() {
if (intValueBuilder_ == null) {
if (valueCase_ == 3) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
} else {
if (valueCase_ == 3) {
return intValueBuilder_.getMessage();
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public Builder setIntValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue value) {
if (intValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
intValueBuilder_.setMessage(value);
}
valueCase_ = 3;
return this;
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public Builder setIntValue(
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder builderForValue) {
if (intValueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
intValueBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 3;
return this;
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public Builder mergeIntValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue value) {
if (intValueBuilder_ == null) {
if (valueCase_ == 3 &&
value_ != org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance()) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.newBuilder((org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 3) {
intValueBuilder_.mergeFrom(value);
}
intValueBuilder_.setMessage(value);
}
valueCase_ = 3;
return this;
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public Builder clearIntValue() {
if (intValueBuilder_ == null) {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 3) {
valueCase_ = 0;
value_ = null;
}
intValueBuilder_.clear();
}
return this;
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder getIntValueBuilder() {
return getIntValueFieldBuilder().getBuilder();
}
/**
* optional .Measure.IntValue int_value = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder getIntValueOrBuilder() {
if ((valueCase_ == 3) && (intValueBuilder_ != null)) {
return intValueBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 3) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
}
/**
* optional .Measure.IntValue int_value = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder>
getIntValueFieldBuilder() {
if (intValueBuilder_ == null) {
if (!(valueCase_ == 3)) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.getDefaultInstance();
}
intValueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValueOrBuilder>(
(org.sonar.scanner.protocol.output.ScannerReport.Measure.IntValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 3;
onChanged();;
return intValueBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder> longValueBuilder_;
/**
* optional .Measure.LongValue long_value = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue getLongValue() {
if (longValueBuilder_ == null) {
if (valueCase_ == 4) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
} else {
if (valueCase_ == 4) {
return longValueBuilder_.getMessage();
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public Builder setLongValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue value) {
if (longValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
longValueBuilder_.setMessage(value);
}
valueCase_ = 4;
return this;
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public Builder setLongValue(
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder builderForValue) {
if (longValueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
longValueBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 4;
return this;
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public Builder mergeLongValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue value) {
if (longValueBuilder_ == null) {
if (valueCase_ == 4 &&
value_ != org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance()) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.newBuilder((org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 4) {
longValueBuilder_.mergeFrom(value);
}
longValueBuilder_.setMessage(value);
}
valueCase_ = 4;
return this;
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public Builder clearLongValue() {
if (longValueBuilder_ == null) {
if (valueCase_ == 4) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 4) {
valueCase_ = 0;
value_ = null;
}
longValueBuilder_.clear();
}
return this;
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder getLongValueBuilder() {
return getLongValueFieldBuilder().getBuilder();
}
/**
* optional .Measure.LongValue long_value = 4;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder getLongValueOrBuilder() {
if ((valueCase_ == 4) && (longValueBuilder_ != null)) {
return longValueBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 4) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
}
/**
* optional .Measure.LongValue long_value = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder>
getLongValueFieldBuilder() {
if (longValueBuilder_ == null) {
if (!(valueCase_ == 4)) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.getDefaultInstance();
}
longValueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValueOrBuilder>(
(org.sonar.scanner.protocol.output.ScannerReport.Measure.LongValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 4;
onChanged();;
return longValueBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder> doubleValueBuilder_;
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue getDoubleValue() {
if (doubleValueBuilder_ == null) {
if (valueCase_ == 5) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
} else {
if (valueCase_ == 5) {
return doubleValueBuilder_.getMessage();
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public Builder setDoubleValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue value) {
if (doubleValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
doubleValueBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public Builder setDoubleValue(
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder builderForValue) {
if (doubleValueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
doubleValueBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 5;
return this;
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public Builder mergeDoubleValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue value) {
if (doubleValueBuilder_ == null) {
if (valueCase_ == 5 &&
value_ != org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance()) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.newBuilder((org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 5) {
doubleValueBuilder_.mergeFrom(value);
}
doubleValueBuilder_.setMessage(value);
}
valueCase_ = 5;
return this;
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public Builder clearDoubleValue() {
if (doubleValueBuilder_ == null) {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 5) {
valueCase_ = 0;
value_ = null;
}
doubleValueBuilder_.clear();
}
return this;
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder getDoubleValueBuilder() {
return getDoubleValueFieldBuilder().getBuilder();
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder getDoubleValueOrBuilder() {
if ((valueCase_ == 5) && (doubleValueBuilder_ != null)) {
return doubleValueBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 5) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
}
/**
* optional .Measure.DoubleValue double_value = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder>
getDoubleValueFieldBuilder() {
if (doubleValueBuilder_ == null) {
if (!(valueCase_ == 5)) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.getDefaultInstance();
}
doubleValueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValueOrBuilder>(
(org.sonar.scanner.protocol.output.ScannerReport.Measure.DoubleValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 5;
onChanged();;
return doubleValueBuilder_;
}
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder> stringValueBuilder_;
/**
* optional .Measure.StringValue string_value = 6;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue getStringValue() {
if (stringValueBuilder_ == null) {
if (valueCase_ == 6) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
} else {
if (valueCase_ == 6) {
return stringValueBuilder_.getMessage();
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public Builder setStringValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue value) {
if (stringValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
stringValueBuilder_.setMessage(value);
}
valueCase_ = 6;
return this;
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public Builder setStringValue(
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder builderForValue) {
if (stringValueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
stringValueBuilder_.setMessage(builderForValue.build());
}
valueCase_ = 6;
return this;
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public Builder mergeStringValue(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue value) {
if (stringValueBuilder_ == null) {
if (valueCase_ == 6 &&
value_ != org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance()) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.newBuilder((org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_)
.mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
if (valueCase_ == 6) {
stringValueBuilder_.mergeFrom(value);
}
stringValueBuilder_.setMessage(value);
}
valueCase_ = 6;
return this;
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public Builder clearStringValue() {
if (stringValueBuilder_ == null) {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
onChanged();
}
} else {
if (valueCase_ == 6) {
valueCase_ = 0;
value_ = null;
}
stringValueBuilder_.clear();
}
return this;
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder getStringValueBuilder() {
return getStringValueFieldBuilder().getBuilder();
}
/**
* optional .Measure.StringValue string_value = 6;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder getStringValueOrBuilder() {
if ((valueCase_ == 6) && (stringValueBuilder_ != null)) {
return stringValueBuilder_.getMessageOrBuilder();
} else {
if (valueCase_ == 6) {
return (org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_;
}
return org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
}
/**
* optional .Measure.StringValue string_value = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder>
getStringValueFieldBuilder() {
if (stringValueBuilder_ == null) {
if (!(valueCase_ == 6)) {
value_ = org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.getDefaultInstance();
}
stringValueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue.Builder, org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValueOrBuilder>(
(org.sonar.scanner.protocol.output.ScannerReport.Measure.StringValue) value_,
getParentForChildren(),
isClean());
value_ = null;
}
valueCase_ = 6;
onChanged();;
return stringValueBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Measure)
}
// @@protoc_insertion_point(class_scope:Measure)
private static final org.sonar.scanner.protocol.output.ScannerReport.Measure DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Measure();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Measure getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Measure parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Measure(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Measure getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IssueOrBuilder extends
// @@protoc_insertion_point(interface_extends:Issue)
com.google.protobuf.MessageOrBuilder {
/**
* optional string rule_repository = 1;
*/
java.lang.String getRuleRepository();
/**
* optional string rule_repository = 1;
*/
com.google.protobuf.ByteString
getRuleRepositoryBytes();
/**
* optional string rule_key = 2;
*/
java.lang.String getRuleKey();
/**
* optional string rule_key = 2;
*/
com.google.protobuf.ByteString
getRuleKeyBytes();
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
java.lang.String getMsg();
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
com.google.protobuf.ByteString
getMsgBytes();
/**
* optional .Severity severity = 4;
*/
int getSeverityValue();
/**
* optional .Severity severity = 4;
*/
org.sonar.scanner.protocol.Constants.Severity getSeverity();
/**
* optional double gap = 5;
*/
double getGap();
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
boolean hasTextRange();
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange();
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder();
/**
* repeated .Flow flow = 7;
*/
java.util.List
getFlowList();
/**
* repeated .Flow flow = 7;
*/
org.sonar.scanner.protocol.output.ScannerReport.Flow getFlow(int index);
/**
* repeated .Flow flow = 7;
*/
int getFlowCount();
/**
* repeated .Flow flow = 7;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder>
getFlowOrBuilderList();
/**
* repeated .Flow flow = 7;
*/
org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder getFlowOrBuilder(
int index);
}
/**
* Protobuf type {@code Issue}
*/
public static final class Issue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Issue)
IssueOrBuilder {
// Use Issue.newBuilder() to construct.
private Issue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Issue() {
ruleRepository_ = "";
ruleKey_ = "";
msg_ = "";
severity_ = 0;
gap_ = 0D;
flow_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Issue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ruleRepository_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
ruleKey_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
msg_ = s;
break;
}
case 32: {
int rawValue = input.readEnum();
severity_ = rawValue;
break;
}
case 41: {
gap_ = input.readDouble();
break;
}
case 50: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (textRange_ != null) {
subBuilder = textRange_.toBuilder();
}
textRange_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(textRange_);
textRange_ = subBuilder.buildPartial();
}
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
flow_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
flow_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Flow.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
flow_ = java.util.Collections.unmodifiableList(flow_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Issue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Issue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Issue.class, org.sonar.scanner.protocol.output.ScannerReport.Issue.Builder.class);
}
private int bitField0_;
public static final int RULE_REPOSITORY_FIELD_NUMBER = 1;
private volatile java.lang.Object ruleRepository_;
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleRepository_ = s;
return s;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RULE_KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object ruleKey_;
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleKey_ = s;
return s;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MSG_FIELD_NUMBER = 3;
private volatile java.lang.Object msg_;
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
}
}
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEVERITY_FIELD_NUMBER = 4;
private int severity_;
/**
* optional .Severity severity = 4;
*/
public int getSeverityValue() {
return severity_;
}
/**
* optional .Severity severity = 4;
*/
public org.sonar.scanner.protocol.Constants.Severity getSeverity() {
org.sonar.scanner.protocol.Constants.Severity result = org.sonar.scanner.protocol.Constants.Severity.valueOf(severity_);
return result == null ? org.sonar.scanner.protocol.Constants.Severity.UNRECOGNIZED : result;
}
public static final int GAP_FIELD_NUMBER = 5;
private double gap_;
/**
* optional double gap = 5;
*/
public double getGap() {
return gap_;
}
public static final int TEXT_RANGE_FIELD_NUMBER = 6;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange textRange_;
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public boolean hasTextRange() {
return textRange_ != null;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange() {
return textRange_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder() {
return getTextRange();
}
public static final int FLOW_FIELD_NUMBER = 7;
private java.util.List flow_;
/**
* repeated .Flow flow = 7;
*/
public java.util.List getFlowList() {
return flow_;
}
/**
* repeated .Flow flow = 7;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder>
getFlowOrBuilderList() {
return flow_;
}
/**
* repeated .Flow flow = 7;
*/
public int getFlowCount() {
return flow_.size();
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Flow getFlow(int index) {
return flow_.get(index);
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder getFlowOrBuilder(
int index) {
return flow_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRuleRepositoryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, ruleRepository_);
}
if (!getRuleKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, ruleKey_);
}
if (!getMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, msg_);
}
if (severity_ != org.sonar.scanner.protocol.Constants.Severity.UNSET_SEVERITY.getNumber()) {
output.writeEnum(4, severity_);
}
if (gap_ != 0D) {
output.writeDouble(5, gap_);
}
if (textRange_ != null) {
output.writeMessage(6, getTextRange());
}
for (int i = 0; i < flow_.size(); i++) {
output.writeMessage(7, flow_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRuleRepositoryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, ruleRepository_);
}
if (!getRuleKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, ruleKey_);
}
if (!getMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, msg_);
}
if (severity_ != org.sonar.scanner.protocol.Constants.Severity.UNSET_SEVERITY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, severity_);
}
if (gap_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, gap_);
}
if (textRange_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getTextRange());
}
for (int i = 0; i < flow_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, flow_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Issue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Issue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Issue)
org.sonar.scanner.protocol.output.ScannerReport.IssueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Issue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Issue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Issue.class, org.sonar.scanner.protocol.output.ScannerReport.Issue.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Issue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFlowFieldBuilder();
}
}
public Builder clear() {
super.clear();
ruleRepository_ = "";
ruleKey_ = "";
msg_ = "";
severity_ = 0;
gap_ = 0D;
if (textRangeBuilder_ == null) {
textRange_ = null;
} else {
textRange_ = null;
textRangeBuilder_ = null;
}
if (flowBuilder_ == null) {
flow_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
flowBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Issue_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Issue getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Issue.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Issue build() {
org.sonar.scanner.protocol.output.ScannerReport.Issue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Issue buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Issue result = new org.sonar.scanner.protocol.output.ScannerReport.Issue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.ruleRepository_ = ruleRepository_;
result.ruleKey_ = ruleKey_;
result.msg_ = msg_;
result.severity_ = severity_;
result.gap_ = gap_;
if (textRangeBuilder_ == null) {
result.textRange_ = textRange_;
} else {
result.textRange_ = textRangeBuilder_.build();
}
if (flowBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
flow_ = java.util.Collections.unmodifiableList(flow_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.flow_ = flow_;
} else {
result.flow_ = flowBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Issue) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Issue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Issue other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Issue.getDefaultInstance()) return this;
if (!other.getRuleRepository().isEmpty()) {
ruleRepository_ = other.ruleRepository_;
onChanged();
}
if (!other.getRuleKey().isEmpty()) {
ruleKey_ = other.ruleKey_;
onChanged();
}
if (!other.getMsg().isEmpty()) {
msg_ = other.msg_;
onChanged();
}
if (other.severity_ != 0) {
setSeverityValue(other.getSeverityValue());
}
if (other.getGap() != 0D) {
setGap(other.getGap());
}
if (other.hasTextRange()) {
mergeTextRange(other.getTextRange());
}
if (flowBuilder_ == null) {
if (!other.flow_.isEmpty()) {
if (flow_.isEmpty()) {
flow_ = other.flow_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureFlowIsMutable();
flow_.addAll(other.flow_);
}
onChanged();
}
} else {
if (!other.flow_.isEmpty()) {
if (flowBuilder_.isEmpty()) {
flowBuilder_.dispose();
flowBuilder_ = null;
flow_ = other.flow_;
bitField0_ = (bitField0_ & ~0x00000040);
flowBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFlowFieldBuilder() : null;
} else {
flowBuilder_.addAllMessages(other.flow_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Issue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Issue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object ruleRepository_ = "";
/**
* optional string rule_repository = 1;
*/
public java.lang.String getRuleRepository() {
java.lang.Object ref = ruleRepository_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleRepository_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public com.google.protobuf.ByteString
getRuleRepositoryBytes() {
java.lang.Object ref = ruleRepository_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepository(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ruleRepository_ = value;
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder clearRuleRepository() {
ruleRepository_ = getDefaultInstance().getRuleRepository();
onChanged();
return this;
}
/**
* optional string rule_repository = 1;
*/
public Builder setRuleRepositoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ruleRepository_ = value;
onChanged();
return this;
}
private java.lang.Object ruleKey_ = "";
/**
* optional string rule_key = 2;
*/
public java.lang.String getRuleKey() {
java.lang.Object ref = ruleKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ruleKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string rule_key = 2;
*/
public com.google.protobuf.ByteString
getRuleKeyBytes() {
java.lang.Object ref = ruleKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ruleKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ruleKey_ = value;
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder clearRuleKey() {
ruleKey_ = getDefaultInstance().getRuleKey();
onChanged();
return this;
}
/**
* optional string rule_key = 2;
*/
public Builder setRuleKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ruleKey_ = value;
onChanged();
return this;
}
private java.lang.Object msg_ = "";
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public Builder setMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
msg_ = value;
onChanged();
return this;
}
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public Builder clearMsg() {
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
/**
* optional string msg = 3;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
*
*/
public Builder setMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
msg_ = value;
onChanged();
return this;
}
private int severity_ = 0;
/**
* optional .Severity severity = 4;
*/
public int getSeverityValue() {
return severity_;
}
/**
* optional .Severity severity = 4;
*/
public Builder setSeverityValue(int value) {
severity_ = value;
onChanged();
return this;
}
/**
* optional .Severity severity = 4;
*/
public org.sonar.scanner.protocol.Constants.Severity getSeverity() {
org.sonar.scanner.protocol.Constants.Severity result = org.sonar.scanner.protocol.Constants.Severity.valueOf(severity_);
return result == null ? org.sonar.scanner.protocol.Constants.Severity.UNRECOGNIZED : result;
}
/**
* optional .Severity severity = 4;
*/
public Builder setSeverity(org.sonar.scanner.protocol.Constants.Severity value) {
if (value == null) {
throw new NullPointerException();
}
severity_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .Severity severity = 4;
*/
public Builder clearSeverity() {
severity_ = 0;
onChanged();
return this;
}
private double gap_ ;
/**
* optional double gap = 5;
*/
public double getGap() {
return gap_;
}
/**
* optional double gap = 5;
*/
public Builder setGap(double value) {
gap_ = value;
onChanged();
return this;
}
/**
* optional double gap = 5;
*/
public Builder clearGap() {
gap_ = 0D;
onChanged();
return this;
}
private org.sonar.scanner.protocol.output.ScannerReport.TextRange textRange_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> textRangeBuilder_;
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public boolean hasTextRange() {
return textRangeBuilder_ != null || textRange_ != null;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange() {
if (textRangeBuilder_ == null) {
return textRange_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
} else {
return textRangeBuilder_.getMessage();
}
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public Builder setTextRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (textRangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
textRange_ = value;
onChanged();
} else {
textRangeBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public Builder setTextRange(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (textRangeBuilder_ == null) {
textRange_ = builderForValue.build();
onChanged();
} else {
textRangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public Builder mergeTextRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (textRangeBuilder_ == null) {
if (textRange_ != null) {
textRange_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(textRange_).mergeFrom(value).buildPartial();
} else {
textRange_ = value;
}
onChanged();
} else {
textRangeBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public Builder clearTextRange() {
if (textRangeBuilder_ == null) {
textRange_ = null;
onChanged();
} else {
textRange_ = null;
textRangeBuilder_ = null;
}
return this;
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getTextRangeBuilder() {
onChanged();
return getTextRangeFieldBuilder().getBuilder();
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder() {
if (textRangeBuilder_ != null) {
return textRangeBuilder_.getMessageOrBuilder();
} else {
return textRange_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
}
}
/**
* optional .TextRange text_range = 6;
*
*
* Only when issue component is a file. Can also be empty for a file if this is an issue global to the file.
* Will be identical to the first location of the first flow
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getTextRangeFieldBuilder() {
if (textRangeBuilder_ == null) {
textRangeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getTextRange(),
getParentForChildren(),
isClean());
textRange_ = null;
}
return textRangeBuilder_;
}
private java.util.List flow_ =
java.util.Collections.emptyList();
private void ensureFlowIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
flow_ = new java.util.ArrayList(flow_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Flow, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder, org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder> flowBuilder_;
/**
* repeated .Flow flow = 7;
*/
public java.util.List getFlowList() {
if (flowBuilder_ == null) {
return java.util.Collections.unmodifiableList(flow_);
} else {
return flowBuilder_.getMessageList();
}
}
/**
* repeated .Flow flow = 7;
*/
public int getFlowCount() {
if (flowBuilder_ == null) {
return flow_.size();
} else {
return flowBuilder_.getCount();
}
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Flow getFlow(int index) {
if (flowBuilder_ == null) {
return flow_.get(index);
} else {
return flowBuilder_.getMessage(index);
}
}
/**
* repeated .Flow flow = 7;
*/
public Builder setFlow(
int index, org.sonar.scanner.protocol.output.ScannerReport.Flow value) {
if (flowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFlowIsMutable();
flow_.set(index, value);
onChanged();
} else {
flowBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder setFlow(
int index, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder builderForValue) {
if (flowBuilder_ == null) {
ensureFlowIsMutable();
flow_.set(index, builderForValue.build());
onChanged();
} else {
flowBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder addFlow(org.sonar.scanner.protocol.output.ScannerReport.Flow value) {
if (flowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFlowIsMutable();
flow_.add(value);
onChanged();
} else {
flowBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder addFlow(
int index, org.sonar.scanner.protocol.output.ScannerReport.Flow value) {
if (flowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFlowIsMutable();
flow_.add(index, value);
onChanged();
} else {
flowBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder addFlow(
org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder builderForValue) {
if (flowBuilder_ == null) {
ensureFlowIsMutable();
flow_.add(builderForValue.build());
onChanged();
} else {
flowBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder addFlow(
int index, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder builderForValue) {
if (flowBuilder_ == null) {
ensureFlowIsMutable();
flow_.add(index, builderForValue.build());
onChanged();
} else {
flowBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder addAllFlow(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.Flow> values) {
if (flowBuilder_ == null) {
ensureFlowIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, flow_);
onChanged();
} else {
flowBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder clearFlow() {
if (flowBuilder_ == null) {
flow_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
flowBuilder_.clear();
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public Builder removeFlow(int index) {
if (flowBuilder_ == null) {
ensureFlowIsMutable();
flow_.remove(index);
onChanged();
} else {
flowBuilder_.remove(index);
}
return this;
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder getFlowBuilder(
int index) {
return getFlowFieldBuilder().getBuilder(index);
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder getFlowOrBuilder(
int index) {
if (flowBuilder_ == null) {
return flow_.get(index); } else {
return flowBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Flow flow = 7;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder>
getFlowOrBuilderList() {
if (flowBuilder_ != null) {
return flowBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(flow_);
}
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder addFlowBuilder() {
return getFlowFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.Flow.getDefaultInstance());
}
/**
* repeated .Flow flow = 7;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder addFlowBuilder(
int index) {
return getFlowFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.Flow.getDefaultInstance());
}
/**
* repeated .Flow flow = 7;
*/
public java.util.List
getFlowBuilderList() {
return getFlowFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Flow, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder, org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder>
getFlowFieldBuilder() {
if (flowBuilder_ == null) {
flowBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Flow, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder, org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder>(
flow_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
flow_ = null;
}
return flowBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Issue)
}
// @@protoc_insertion_point(class_scope:Issue)
private static final org.sonar.scanner.protocol.output.ScannerReport.Issue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Issue();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Issue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Issue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Issue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Issue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IssueLocationOrBuilder extends
// @@protoc_insertion_point(interface_extends:IssueLocation)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 component_ref = 1;
*/
int getComponentRef();
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
boolean hasTextRange();
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange();
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder();
/**
* optional string msg = 3;
*/
java.lang.String getMsg();
/**
* optional string msg = 3;
*/
com.google.protobuf.ByteString
getMsgBytes();
}
/**
* Protobuf type {@code IssueLocation}
*/
public static final class IssueLocation extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:IssueLocation)
IssueLocationOrBuilder {
// Use IssueLocation.newBuilder() to construct.
private IssueLocation(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private IssueLocation() {
componentRef_ = 0;
msg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private IssueLocation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
componentRef_ = input.readInt32();
break;
}
case 18: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (textRange_ != null) {
subBuilder = textRange_.toBuilder();
}
textRange_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(textRange_);
textRange_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
msg_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_IssueLocation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_IssueLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.class, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder.class);
}
public static final int COMPONENT_REF_FIELD_NUMBER = 1;
private int componentRef_;
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
public static final int TEXT_RANGE_FIELD_NUMBER = 2;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange textRange_;
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public boolean hasTextRange() {
return textRange_ != null;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange() {
return textRange_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder() {
return getTextRange();
}
public static final int MSG_FIELD_NUMBER = 3;
private volatile java.lang.Object msg_;
/**
* optional string msg = 3;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
}
}
/**
* optional string msg = 3;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (componentRef_ != 0) {
output.writeInt32(1, componentRef_);
}
if (textRange_ != null) {
output.writeMessage(2, getTextRange());
}
if (!getMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, msg_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (componentRef_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, componentRef_);
}
if (textRange_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTextRange());
}
if (!getMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, msg_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.IssueLocation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code IssueLocation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:IssueLocation)
org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_IssueLocation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_IssueLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.class, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
componentRef_ = 0;
if (textRangeBuilder_ == null) {
textRange_ = null;
} else {
textRange_ = null;
textRangeBuilder_ = null;
}
msg_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_IssueLocation_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation build() {
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation result = new org.sonar.scanner.protocol.output.ScannerReport.IssueLocation(this);
result.componentRef_ = componentRef_;
if (textRangeBuilder_ == null) {
result.textRange_ = textRange_;
} else {
result.textRange_ = textRangeBuilder_.build();
}
result.msg_ = msg_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.IssueLocation) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.IssueLocation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.IssueLocation other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.getDefaultInstance()) return this;
if (other.getComponentRef() != 0) {
setComponentRef(other.getComponentRef());
}
if (other.hasTextRange()) {
mergeTextRange(other.getTextRange());
}
if (!other.getMsg().isEmpty()) {
msg_ = other.msg_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.IssueLocation) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int componentRef_ ;
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
/**
* optional int32 component_ref = 1;
*/
public Builder setComponentRef(int value) {
componentRef_ = value;
onChanged();
return this;
}
/**
* optional int32 component_ref = 1;
*/
public Builder clearComponentRef() {
componentRef_ = 0;
onChanged();
return this;
}
private org.sonar.scanner.protocol.output.ScannerReport.TextRange textRange_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> textRangeBuilder_;
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public boolean hasTextRange() {
return textRangeBuilder_ != null || textRange_ != null;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getTextRange() {
if (textRangeBuilder_ == null) {
return textRange_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
} else {
return textRangeBuilder_.getMessage();
}
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public Builder setTextRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (textRangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
textRange_ = value;
onChanged();
} else {
textRangeBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public Builder setTextRange(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (textRangeBuilder_ == null) {
textRange_ = builderForValue.build();
onChanged();
} else {
textRangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public Builder mergeTextRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (textRangeBuilder_ == null) {
if (textRange_ != null) {
textRange_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(textRange_).mergeFrom(value).buildPartial();
} else {
textRange_ = value;
}
onChanged();
} else {
textRangeBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public Builder clearTextRange() {
if (textRangeBuilder_ == null) {
textRange_ = null;
onChanged();
} else {
textRange_ = null;
textRangeBuilder_ = null;
}
return this;
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getTextRangeBuilder() {
onChanged();
return getTextRangeFieldBuilder().getBuilder();
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getTextRangeOrBuilder() {
if (textRangeBuilder_ != null) {
return textRangeBuilder_.getMessageOrBuilder();
} else {
return textRange_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : textRange_;
}
}
/**
* optional .TextRange text_range = 2;
*
*
* Only when component is a file. Can be empty for a file if this is an issue global to the file.
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getTextRangeFieldBuilder() {
if (textRangeBuilder_ == null) {
textRangeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getTextRange(),
getParentForChildren(),
isClean());
textRange_ = null;
}
return textRangeBuilder_;
}
private java.lang.Object msg_ = "";
/**
* optional string msg = 3;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msg = 3;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msg = 3;
*/
public Builder setMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
msg_ = value;
onChanged();
return this;
}
/**
* optional string msg = 3;
*/
public Builder clearMsg() {
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
/**
* optional string msg = 3;
*/
public Builder setMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
msg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:IssueLocation)
}
// @@protoc_insertion_point(class_scope:IssueLocation)
private static final org.sonar.scanner.protocol.output.ScannerReport.IssueLocation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.IssueLocation();
}
public static org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public IssueLocation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new IssueLocation(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FlowOrBuilder extends
// @@protoc_insertion_point(interface_extends:Flow)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .IssueLocation location = 1;
*/
java.util.List
getLocationList();
/**
* repeated .IssueLocation location = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getLocation(int index);
/**
* repeated .IssueLocation location = 1;
*/
int getLocationCount();
/**
* repeated .IssueLocation location = 1;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder>
getLocationOrBuilderList();
/**
* repeated .IssueLocation location = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder getLocationOrBuilder(
int index);
}
/**
* Protobuf type {@code Flow}
*/
public static final class Flow extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Flow)
FlowOrBuilder {
// Use Flow.newBuilder() to construct.
private Flow(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Flow() {
location_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Flow(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
location_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
location_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
location_ = java.util.Collections.unmodifiableList(location_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Flow_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Flow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Flow.class, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder.class);
}
public static final int LOCATION_FIELD_NUMBER = 1;
private java.util.List location_;
/**
* repeated .IssueLocation location = 1;
*/
public java.util.List getLocationList() {
return location_;
}
/**
* repeated .IssueLocation location = 1;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder>
getLocationOrBuilderList() {
return location_;
}
/**
* repeated .IssueLocation location = 1;
*/
public int getLocationCount() {
return location_.size();
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getLocation(int index) {
return location_.get(index);
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder getLocationOrBuilder(
int index) {
return location_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < location_.size(); i++) {
output.writeMessage(1, location_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < location_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, location_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Flow prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Flow}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Flow)
org.sonar.scanner.protocol.output.ScannerReport.FlowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Flow_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Flow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Flow.class, org.sonar.scanner.protocol.output.ScannerReport.Flow.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Flow.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getLocationFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (locationBuilder_ == null) {
location_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
locationBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Flow_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Flow getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Flow.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Flow build() {
org.sonar.scanner.protocol.output.ScannerReport.Flow result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Flow buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Flow result = new org.sonar.scanner.protocol.output.ScannerReport.Flow(this);
int from_bitField0_ = bitField0_;
if (locationBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
location_ = java.util.Collections.unmodifiableList(location_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.location_ = location_;
} else {
result.location_ = locationBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Flow) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Flow)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Flow other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Flow.getDefaultInstance()) return this;
if (locationBuilder_ == null) {
if (!other.location_.isEmpty()) {
if (location_.isEmpty()) {
location_ = other.location_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLocationIsMutable();
location_.addAll(other.location_);
}
onChanged();
}
} else {
if (!other.location_.isEmpty()) {
if (locationBuilder_.isEmpty()) {
locationBuilder_.dispose();
locationBuilder_ = null;
location_ = other.location_;
bitField0_ = (bitField0_ & ~0x00000001);
locationBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getLocationFieldBuilder() : null;
} else {
locationBuilder_.addAllMessages(other.location_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Flow parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Flow) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List location_ =
java.util.Collections.emptyList();
private void ensureLocationIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
location_ = new java.util.ArrayList(location_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder, org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder> locationBuilder_;
/**
* repeated .IssueLocation location = 1;
*/
public java.util.List getLocationList() {
if (locationBuilder_ == null) {
return java.util.Collections.unmodifiableList(location_);
} else {
return locationBuilder_.getMessageList();
}
}
/**
* repeated .IssueLocation location = 1;
*/
public int getLocationCount() {
if (locationBuilder_ == null) {
return location_.size();
} else {
return locationBuilder_.getCount();
}
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation getLocation(int index) {
if (locationBuilder_ == null) {
return location_.get(index);
} else {
return locationBuilder_.getMessage(index);
}
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder setLocation(
int index, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationIsMutable();
location_.set(index, value);
onChanged();
} else {
locationBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder setLocation(
int index, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder builderForValue) {
if (locationBuilder_ == null) {
ensureLocationIsMutable();
location_.set(index, builderForValue.build());
onChanged();
} else {
locationBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder addLocation(org.sonar.scanner.protocol.output.ScannerReport.IssueLocation value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationIsMutable();
location_.add(value);
onChanged();
} else {
locationBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder addLocation(
int index, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationIsMutable();
location_.add(index, value);
onChanged();
} else {
locationBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder addLocation(
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder builderForValue) {
if (locationBuilder_ == null) {
ensureLocationIsMutable();
location_.add(builderForValue.build());
onChanged();
} else {
locationBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder addLocation(
int index, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder builderForValue) {
if (locationBuilder_ == null) {
ensureLocationIsMutable();
location_.add(index, builderForValue.build());
onChanged();
} else {
locationBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder addAllLocation(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.IssueLocation> values) {
if (locationBuilder_ == null) {
ensureLocationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, location_);
onChanged();
} else {
locationBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
location_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
locationBuilder_.clear();
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public Builder removeLocation(int index) {
if (locationBuilder_ == null) {
ensureLocationIsMutable();
location_.remove(index);
onChanged();
} else {
locationBuilder_.remove(index);
}
return this;
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder getLocationBuilder(
int index) {
return getLocationFieldBuilder().getBuilder(index);
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder getLocationOrBuilder(
int index) {
if (locationBuilder_ == null) {
return location_.get(index); } else {
return locationBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .IssueLocation location = 1;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder>
getLocationOrBuilderList() {
if (locationBuilder_ != null) {
return locationBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(location_);
}
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder addLocationBuilder() {
return getLocationFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.getDefaultInstance());
}
/**
* repeated .IssueLocation location = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder addLocationBuilder(
int index) {
return getLocationFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.getDefaultInstance());
}
/**
* repeated .IssueLocation location = 1;
*/
public java.util.List
getLocationBuilderList() {
return getLocationFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder, org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
locationBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.IssueLocation, org.sonar.scanner.protocol.output.ScannerReport.IssueLocation.Builder, org.sonar.scanner.protocol.output.ScannerReport.IssueLocationOrBuilder>(
location_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
location_ = null;
}
return locationBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Flow)
}
// @@protoc_insertion_point(class_scope:Flow)
private static final org.sonar.scanner.protocol.output.ScannerReport.Flow DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Flow();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Flow getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Flow parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Flow(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Flow getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ChangesetsOrBuilder extends
// @@protoc_insertion_point(interface_extends:Changesets)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 component_ref = 1;
*/
int getComponentRef();
/**
* optional bool copy_from_previous = 2;
*
*
* If set to true then it means changeset attribute is empty and compute engine should copy data from previous analysis
*
*/
boolean getCopyFromPrevious();
/**
* repeated .Changesets.Changeset changeset = 3;
*/
java.util.List
getChangesetList();
/**
* repeated .Changesets.Changeset changeset = 3;
*/
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getChangeset(int index);
/**
* repeated .Changesets.Changeset changeset = 3;
*/
int getChangesetCount();
/**
* repeated .Changesets.Changeset changeset = 3;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder>
getChangesetOrBuilderList();
/**
* repeated .Changesets.Changeset changeset = 3;
*/
org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder getChangesetOrBuilder(
int index);
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
java.util.List getChangesetIndexByLineList();
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
int getChangesetIndexByLineCount();
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
int getChangesetIndexByLine(int index);
}
/**
* Protobuf type {@code Changesets}
*/
public static final class Changesets extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Changesets)
ChangesetsOrBuilder {
// Use Changesets.newBuilder() to construct.
private Changesets(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Changesets() {
componentRef_ = 0;
copyFromPrevious_ = false;
changeset_ = java.util.Collections.emptyList();
changesetIndexByLine_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Changesets(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
componentRef_ = input.readInt32();
break;
}
case 16: {
copyFromPrevious_ = input.readBool();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
changeset_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
changeset_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.parser(), extensionRegistry));
break;
}
case 32: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
changesetIndexByLine_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
changesetIndexByLine_.add(input.readInt32());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008) && input.getBytesUntilLimit() > 0) {
changesetIndexByLine_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
changesetIndexByLine_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
changeset_ = java.util.Collections.unmodifiableList(changeset_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
changesetIndexByLine_ = java.util.Collections.unmodifiableList(changesetIndexByLine_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.class, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Builder.class);
}
public interface ChangesetOrBuilder extends
// @@protoc_insertion_point(interface_extends:Changesets.Changeset)
com.google.protobuf.MessageOrBuilder {
/**
* optional string revision = 1;
*/
java.lang.String getRevision();
/**
* optional string revision = 1;
*/
com.google.protobuf.ByteString
getRevisionBytes();
/**
* optional string author = 2;
*/
java.lang.String getAuthor();
/**
* optional string author = 2;
*/
com.google.protobuf.ByteString
getAuthorBytes();
/**
* optional int64 date = 3;
*/
long getDate();
}
/**
* Protobuf type {@code Changesets.Changeset}
*/
public static final class Changeset extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Changesets.Changeset)
ChangesetOrBuilder {
// Use Changeset.newBuilder() to construct.
private Changeset(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Changeset() {
revision_ = "";
author_ = "";
date_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Changeset(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
revision_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
author_ = s;
break;
}
case 24: {
date_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_Changeset_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_Changeset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.class, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder.class);
}
public static final int REVISION_FIELD_NUMBER = 1;
private volatile java.lang.Object revision_;
/**
* optional string revision = 1;
*/
public java.lang.String getRevision() {
java.lang.Object ref = revision_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
revision_ = s;
return s;
}
}
/**
* optional string revision = 1;
*/
public com.google.protobuf.ByteString
getRevisionBytes() {
java.lang.Object ref = revision_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
revision_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTHOR_FIELD_NUMBER = 2;
private volatile java.lang.Object author_;
/**
* optional string author = 2;
*/
public java.lang.String getAuthor() {
java.lang.Object ref = author_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
author_ = s;
return s;
}
}
/**
* optional string author = 2;
*/
public com.google.protobuf.ByteString
getAuthorBytes() {
java.lang.Object ref = author_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
author_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATE_FIELD_NUMBER = 3;
private long date_;
/**
* optional int64 date = 3;
*/
public long getDate() {
return date_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRevisionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, revision_);
}
if (!getAuthorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, author_);
}
if (date_ != 0L) {
output.writeInt64(3, date_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRevisionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, revision_);
}
if (!getAuthorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, author_);
}
if (date_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, date_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Changesets.Changeset}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Changesets.Changeset)
org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_Changeset_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_Changeset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.class, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
revision_ = "";
author_ = "";
date_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_Changeset_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset build() {
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset result = new org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset(this);
result.revision_ = revision_;
result.author_ = author_;
result.date_ = date_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.getDefaultInstance()) return this;
if (!other.getRevision().isEmpty()) {
revision_ = other.revision_;
onChanged();
}
if (!other.getAuthor().isEmpty()) {
author_ = other.author_;
onChanged();
}
if (other.getDate() != 0L) {
setDate(other.getDate());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object revision_ = "";
/**
* optional string revision = 1;
*/
public java.lang.String getRevision() {
java.lang.Object ref = revision_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
revision_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string revision = 1;
*/
public com.google.protobuf.ByteString
getRevisionBytes() {
java.lang.Object ref = revision_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
revision_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string revision = 1;
*/
public Builder setRevision(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
revision_ = value;
onChanged();
return this;
}
/**
* optional string revision = 1;
*/
public Builder clearRevision() {
revision_ = getDefaultInstance().getRevision();
onChanged();
return this;
}
/**
* optional string revision = 1;
*/
public Builder setRevisionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
revision_ = value;
onChanged();
return this;
}
private java.lang.Object author_ = "";
/**
* optional string author = 2;
*/
public java.lang.String getAuthor() {
java.lang.Object ref = author_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
author_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string author = 2;
*/
public com.google.protobuf.ByteString
getAuthorBytes() {
java.lang.Object ref = author_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
author_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string author = 2;
*/
public Builder setAuthor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
author_ = value;
onChanged();
return this;
}
/**
* optional string author = 2;
*/
public Builder clearAuthor() {
author_ = getDefaultInstance().getAuthor();
onChanged();
return this;
}
/**
* optional string author = 2;
*/
public Builder setAuthorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
author_ = value;
onChanged();
return this;
}
private long date_ ;
/**
* optional int64 date = 3;
*/
public long getDate() {
return date_;
}
/**
* optional int64 date = 3;
*/
public Builder setDate(long value) {
date_ = value;
onChanged();
return this;
}
/**
* optional int64 date = 3;
*/
public Builder clearDate() {
date_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Changesets.Changeset)
}
// @@protoc_insertion_point(class_scope:Changesets.Changeset)
private static final org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Changeset parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Changeset(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int COMPONENT_REF_FIELD_NUMBER = 1;
private int componentRef_;
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
public static final int COPY_FROM_PREVIOUS_FIELD_NUMBER = 2;
private boolean copyFromPrevious_;
/**
* optional bool copy_from_previous = 2;
*
*
* If set to true then it means changeset attribute is empty and compute engine should copy data from previous analysis
*
*/
public boolean getCopyFromPrevious() {
return copyFromPrevious_;
}
public static final int CHANGESET_FIELD_NUMBER = 3;
private java.util.List changeset_;
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public java.util.List getChangesetList() {
return changeset_;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder>
getChangesetOrBuilderList() {
return changeset_;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public int getChangesetCount() {
return changeset_.size();
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getChangeset(int index) {
return changeset_.get(index);
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder getChangesetOrBuilder(
int index) {
return changeset_.get(index);
}
public static final int CHANGESETINDEXBYLINE_FIELD_NUMBER = 4;
private java.util.List changesetIndexByLine_;
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public java.util.List
getChangesetIndexByLineList() {
return changesetIndexByLine_;
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public int getChangesetIndexByLineCount() {
return changesetIndexByLine_.size();
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public int getChangesetIndexByLine(int index) {
return changesetIndexByLine_.get(index);
}
private int changesetIndexByLineMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (componentRef_ != 0) {
output.writeInt32(1, componentRef_);
}
if (copyFromPrevious_ != false) {
output.writeBool(2, copyFromPrevious_);
}
for (int i = 0; i < changeset_.size(); i++) {
output.writeMessage(3, changeset_.get(i));
}
if (getChangesetIndexByLineList().size() > 0) {
output.writeRawVarint32(34);
output.writeRawVarint32(changesetIndexByLineMemoizedSerializedSize);
}
for (int i = 0; i < changesetIndexByLine_.size(); i++) {
output.writeInt32NoTag(changesetIndexByLine_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (componentRef_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, componentRef_);
}
if (copyFromPrevious_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, copyFromPrevious_);
}
for (int i = 0; i < changeset_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, changeset_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < changesetIndexByLine_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(changesetIndexByLine_.get(i));
}
size += dataSize;
if (!getChangesetIndexByLineList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
changesetIndexByLineMemoizedSerializedSize = dataSize;
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Changesets prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Changesets}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Changesets)
org.sonar.scanner.protocol.output.ScannerReport.ChangesetsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.class, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Changesets.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getChangesetFieldBuilder();
}
}
public Builder clear() {
super.clear();
componentRef_ = 0;
copyFromPrevious_ = false;
if (changesetBuilder_ == null) {
changeset_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
changesetBuilder_.clear();
}
changesetIndexByLine_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Changesets_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Changesets.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets build() {
org.sonar.scanner.protocol.output.ScannerReport.Changesets result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Changesets result = new org.sonar.scanner.protocol.output.ScannerReport.Changesets(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.componentRef_ = componentRef_;
result.copyFromPrevious_ = copyFromPrevious_;
if (changesetBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
changeset_ = java.util.Collections.unmodifiableList(changeset_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.changeset_ = changeset_;
} else {
result.changeset_ = changesetBuilder_.build();
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
changesetIndexByLine_ = java.util.Collections.unmodifiableList(changesetIndexByLine_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.changesetIndexByLine_ = changesetIndexByLine_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Changesets) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Changesets)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Changesets other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Changesets.getDefaultInstance()) return this;
if (other.getComponentRef() != 0) {
setComponentRef(other.getComponentRef());
}
if (other.getCopyFromPrevious() != false) {
setCopyFromPrevious(other.getCopyFromPrevious());
}
if (changesetBuilder_ == null) {
if (!other.changeset_.isEmpty()) {
if (changeset_.isEmpty()) {
changeset_ = other.changeset_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureChangesetIsMutable();
changeset_.addAll(other.changeset_);
}
onChanged();
}
} else {
if (!other.changeset_.isEmpty()) {
if (changesetBuilder_.isEmpty()) {
changesetBuilder_.dispose();
changesetBuilder_ = null;
changeset_ = other.changeset_;
bitField0_ = (bitField0_ & ~0x00000004);
changesetBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getChangesetFieldBuilder() : null;
} else {
changesetBuilder_.addAllMessages(other.changeset_);
}
}
}
if (!other.changesetIndexByLine_.isEmpty()) {
if (changesetIndexByLine_.isEmpty()) {
changesetIndexByLine_ = other.changesetIndexByLine_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureChangesetIndexByLineIsMutable();
changesetIndexByLine_.addAll(other.changesetIndexByLine_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Changesets parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Changesets) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int componentRef_ ;
/**
* optional int32 component_ref = 1;
*/
public int getComponentRef() {
return componentRef_;
}
/**
* optional int32 component_ref = 1;
*/
public Builder setComponentRef(int value) {
componentRef_ = value;
onChanged();
return this;
}
/**
* optional int32 component_ref = 1;
*/
public Builder clearComponentRef() {
componentRef_ = 0;
onChanged();
return this;
}
private boolean copyFromPrevious_ ;
/**
* optional bool copy_from_previous = 2;
*
*
* If set to true then it means changeset attribute is empty and compute engine should copy data from previous analysis
*
*/
public boolean getCopyFromPrevious() {
return copyFromPrevious_;
}
/**
* optional bool copy_from_previous = 2;
*
*
* If set to true then it means changeset attribute is empty and compute engine should copy data from previous analysis
*
*/
public Builder setCopyFromPrevious(boolean value) {
copyFromPrevious_ = value;
onChanged();
return this;
}
/**
* optional bool copy_from_previous = 2;
*
*
* If set to true then it means changeset attribute is empty and compute engine should copy data from previous analysis
*
*/
public Builder clearCopyFromPrevious() {
copyFromPrevious_ = false;
onChanged();
return this;
}
private java.util.List changeset_ =
java.util.Collections.emptyList();
private void ensureChangesetIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
changeset_ = new java.util.ArrayList(changeset_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder, org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder> changesetBuilder_;
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public java.util.List getChangesetList() {
if (changesetBuilder_ == null) {
return java.util.Collections.unmodifiableList(changeset_);
} else {
return changesetBuilder_.getMessageList();
}
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public int getChangesetCount() {
if (changesetBuilder_ == null) {
return changeset_.size();
} else {
return changesetBuilder_.getCount();
}
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset getChangeset(int index) {
if (changesetBuilder_ == null) {
return changeset_.get(index);
} else {
return changesetBuilder_.getMessage(index);
}
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder setChangeset(
int index, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset value) {
if (changesetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetIsMutable();
changeset_.set(index, value);
onChanged();
} else {
changesetBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder setChangeset(
int index, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder builderForValue) {
if (changesetBuilder_ == null) {
ensureChangesetIsMutable();
changeset_.set(index, builderForValue.build());
onChanged();
} else {
changesetBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder addChangeset(org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset value) {
if (changesetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetIsMutable();
changeset_.add(value);
onChanged();
} else {
changesetBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder addChangeset(
int index, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset value) {
if (changesetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetIsMutable();
changeset_.add(index, value);
onChanged();
} else {
changesetBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder addChangeset(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder builderForValue) {
if (changesetBuilder_ == null) {
ensureChangesetIsMutable();
changeset_.add(builderForValue.build());
onChanged();
} else {
changesetBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder addChangeset(
int index, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder builderForValue) {
if (changesetBuilder_ == null) {
ensureChangesetIsMutable();
changeset_.add(index, builderForValue.build());
onChanged();
} else {
changesetBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder addAllChangeset(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset> values) {
if (changesetBuilder_ == null) {
ensureChangesetIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, changeset_);
onChanged();
} else {
changesetBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder clearChangeset() {
if (changesetBuilder_ == null) {
changeset_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
changesetBuilder_.clear();
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public Builder removeChangeset(int index) {
if (changesetBuilder_ == null) {
ensureChangesetIsMutable();
changeset_.remove(index);
onChanged();
} else {
changesetBuilder_.remove(index);
}
return this;
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder getChangesetBuilder(
int index) {
return getChangesetFieldBuilder().getBuilder(index);
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder getChangesetOrBuilder(
int index) {
if (changesetBuilder_ == null) {
return changeset_.get(index); } else {
return changesetBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder>
getChangesetOrBuilderList() {
if (changesetBuilder_ != null) {
return changesetBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(changeset_);
}
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder addChangesetBuilder() {
return getChangesetFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.getDefaultInstance());
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder addChangesetBuilder(
int index) {
return getChangesetFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.getDefaultInstance());
}
/**
* repeated .Changesets.Changeset changeset = 3;
*/
public java.util.List
getChangesetBuilderList() {
return getChangesetFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder, org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder>
getChangesetFieldBuilder() {
if (changesetBuilder_ == null) {
changesetBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset, org.sonar.scanner.protocol.output.ScannerReport.Changesets.Changeset.Builder, org.sonar.scanner.protocol.output.ScannerReport.Changesets.ChangesetOrBuilder>(
changeset_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
changeset_ = null;
}
return changesetBuilder_;
}
private java.util.List changesetIndexByLine_ = java.util.Collections.emptyList();
private void ensureChangesetIndexByLineIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
changesetIndexByLine_ = new java.util.ArrayList(changesetIndexByLine_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public java.util.List
getChangesetIndexByLineList() {
return java.util.Collections.unmodifiableList(changesetIndexByLine_);
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public int getChangesetIndexByLineCount() {
return changesetIndexByLine_.size();
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public int getChangesetIndexByLine(int index) {
return changesetIndexByLine_.get(index);
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public Builder setChangesetIndexByLine(
int index, int value) {
ensureChangesetIndexByLineIsMutable();
changesetIndexByLine_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public Builder addChangesetIndexByLine(int value) {
ensureChangesetIndexByLineIsMutable();
changesetIndexByLine_.add(value);
onChanged();
return this;
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public Builder addAllChangesetIndexByLine(
java.lang.Iterable extends java.lang.Integer> values) {
ensureChangesetIndexByLineIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, changesetIndexByLine_);
onChanged();
return this;
}
/**
* repeated int32 changesetIndexByLine = 4 [packed = true];
*
*
* if changesetIndexByLine[5] = 2 then it means that changeset[2] is the last one on line 6
*
*/
public Builder clearChangesetIndexByLine() {
changesetIndexByLine_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Changesets)
}
// @@protoc_insertion_point(class_scope:Changesets)
private static final org.sonar.scanner.protocol.output.ScannerReport.Changesets DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Changesets();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Changesets getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Changesets parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Changesets(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Changesets getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DuplicateOrBuilder extends
// @@protoc_insertion_point(interface_extends:Duplicate)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 other_file_ref = 1;
*
*
* Will be 0 when duplicate is in the same file
*
*/
int getOtherFileRef();
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
boolean hasRange();
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange();
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder();
}
/**
* Protobuf type {@code Duplicate}
*/
public static final class Duplicate extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Duplicate)
DuplicateOrBuilder {
// Use Duplicate.newBuilder() to construct.
private Duplicate(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Duplicate() {
otherFileRef_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Duplicate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
otherFileRef_ = input.readInt32();
break;
}
case 18: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (range_ != null) {
subBuilder = range_.toBuilder();
}
range_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(range_);
range_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplicate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplicate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Duplicate.class, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder.class);
}
public static final int OTHER_FILE_REF_FIELD_NUMBER = 1;
private int otherFileRef_;
/**
* optional int32 other_file_ref = 1;
*
*
* Will be 0 when duplicate is in the same file
*
*/
public int getOtherFileRef() {
return otherFileRef_;
}
public static final int RANGE_FIELD_NUMBER = 2;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange range_;
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public boolean hasRange() {
return range_ != null;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange() {
return range_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder() {
return getRange();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (otherFileRef_ != 0) {
output.writeInt32(1, otherFileRef_);
}
if (range_ != null) {
output.writeMessage(2, getRange());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (otherFileRef_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, otherFileRef_);
}
if (range_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRange());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Duplicate prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Duplicate}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Duplicate)
org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplicate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplicate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Duplicate.class, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Duplicate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
otherFileRef_ = 0;
if (rangeBuilder_ == null) {
range_ = null;
} else {
range_ = null;
rangeBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplicate_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Duplicate.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate build() {
org.sonar.scanner.protocol.output.ScannerReport.Duplicate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Duplicate result = new org.sonar.scanner.protocol.output.ScannerReport.Duplicate(this);
result.otherFileRef_ = otherFileRef_;
if (rangeBuilder_ == null) {
result.range_ = range_;
} else {
result.range_ = rangeBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Duplicate) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Duplicate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Duplicate other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Duplicate.getDefaultInstance()) return this;
if (other.getOtherFileRef() != 0) {
setOtherFileRef(other.getOtherFileRef());
}
if (other.hasRange()) {
mergeRange(other.getRange());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Duplicate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Duplicate) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int otherFileRef_ ;
/**
* optional int32 other_file_ref = 1;
*
*
* Will be 0 when duplicate is in the same file
*
*/
public int getOtherFileRef() {
return otherFileRef_;
}
/**
* optional int32 other_file_ref = 1;
*
*
* Will be 0 when duplicate is in the same file
*
*/
public Builder setOtherFileRef(int value) {
otherFileRef_ = value;
onChanged();
return this;
}
/**
* optional int32 other_file_ref = 1;
*
*
* Will be 0 when duplicate is in the same file
*
*/
public Builder clearOtherFileRef() {
otherFileRef_ = 0;
onChanged();
return this;
}
private org.sonar.scanner.protocol.output.ScannerReport.TextRange range_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> rangeBuilder_;
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public boolean hasRange() {
return rangeBuilder_ != null || range_ != null;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange() {
if (rangeBuilder_ == null) {
return range_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
} else {
return rangeBuilder_.getMessage();
}
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder setRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
range_ = value;
onChanged();
} else {
rangeBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder setRange(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (rangeBuilder_ == null) {
range_ = builderForValue.build();
onChanged();
} else {
rangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder mergeRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (rangeBuilder_ == null) {
if (range_ != null) {
range_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(range_).mergeFrom(value).buildPartial();
} else {
range_ = value;
}
onChanged();
} else {
rangeBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder clearRange() {
if (rangeBuilder_ == null) {
range_ = null;
onChanged();
} else {
range_ = null;
rangeBuilder_ = null;
}
return this;
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getRangeBuilder() {
onChanged();
return getRangeFieldBuilder().getBuilder();
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder() {
if (rangeBuilder_ != null) {
return rangeBuilder_.getMessageOrBuilder();
} else {
return range_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
}
}
/**
* optional .TextRange range = 2;
*
*
* Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getRangeFieldBuilder() {
if (rangeBuilder_ == null) {
rangeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getRange(),
getParentForChildren(),
isClean());
range_ = null;
}
return rangeBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Duplicate)
}
// @@protoc_insertion_point(class_scope:Duplicate)
private static final org.sonar.scanner.protocol.output.ScannerReport.Duplicate DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Duplicate();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Duplicate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Duplicate(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DuplicationOrBuilder extends
// @@protoc_insertion_point(interface_extends:Duplication)
com.google.protobuf.MessageOrBuilder {
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
boolean hasOriginPosition();
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getOriginPosition();
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getOriginPositionOrBuilder();
/**
* repeated .Duplicate duplicate = 2;
*/
java.util.List
getDuplicateList();
/**
* repeated .Duplicate duplicate = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDuplicate(int index);
/**
* repeated .Duplicate duplicate = 2;
*/
int getDuplicateCount();
/**
* repeated .Duplicate duplicate = 2;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder>
getDuplicateOrBuilderList();
/**
* repeated .Duplicate duplicate = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder getDuplicateOrBuilder(
int index);
}
/**
* Protobuf type {@code Duplication}
*/
public static final class Duplication extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Duplication)
DuplicationOrBuilder {
// Use Duplication.newBuilder() to construct.
private Duplication(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Duplication() {
duplicate_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Duplication(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (originPosition_ != null) {
subBuilder = originPosition_.toBuilder();
}
originPosition_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(originPosition_);
originPosition_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
duplicate_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
duplicate_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.Duplicate.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
duplicate_ = java.util.Collections.unmodifiableList(duplicate_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplication_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplication_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Duplication.class, org.sonar.scanner.protocol.output.ScannerReport.Duplication.Builder.class);
}
private int bitField0_;
public static final int ORIGIN_POSITION_FIELD_NUMBER = 1;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange originPosition_;
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public boolean hasOriginPosition() {
return originPosition_ != null;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getOriginPosition() {
return originPosition_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : originPosition_;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getOriginPositionOrBuilder() {
return getOriginPosition();
}
public static final int DUPLICATE_FIELD_NUMBER = 2;
private java.util.List duplicate_;
/**
* repeated .Duplicate duplicate = 2;
*/
public java.util.List getDuplicateList() {
return duplicate_;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder>
getDuplicateOrBuilderList() {
return duplicate_;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public int getDuplicateCount() {
return duplicate_.size();
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDuplicate(int index) {
return duplicate_.get(index);
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder getDuplicateOrBuilder(
int index) {
return duplicate_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (originPosition_ != null) {
output.writeMessage(1, getOriginPosition());
}
for (int i = 0; i < duplicate_.size(); i++) {
output.writeMessage(2, duplicate_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (originPosition_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getOriginPosition());
}
for (int i = 0; i < duplicate_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, duplicate_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Duplication prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Duplication}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Duplication)
org.sonar.scanner.protocol.output.ScannerReport.DuplicationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplication_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplication_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Duplication.class, org.sonar.scanner.protocol.output.ScannerReport.Duplication.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Duplication.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getDuplicateFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (originPositionBuilder_ == null) {
originPosition_ = null;
} else {
originPosition_ = null;
originPositionBuilder_ = null;
}
if (duplicateBuilder_ == null) {
duplicate_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
duplicateBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Duplication_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplication getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Duplication.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplication build() {
org.sonar.scanner.protocol.output.ScannerReport.Duplication result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplication buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Duplication result = new org.sonar.scanner.protocol.output.ScannerReport.Duplication(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (originPositionBuilder_ == null) {
result.originPosition_ = originPosition_;
} else {
result.originPosition_ = originPositionBuilder_.build();
}
if (duplicateBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
duplicate_ = java.util.Collections.unmodifiableList(duplicate_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.duplicate_ = duplicate_;
} else {
result.duplicate_ = duplicateBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Duplication) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Duplication)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Duplication other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Duplication.getDefaultInstance()) return this;
if (other.hasOriginPosition()) {
mergeOriginPosition(other.getOriginPosition());
}
if (duplicateBuilder_ == null) {
if (!other.duplicate_.isEmpty()) {
if (duplicate_.isEmpty()) {
duplicate_ = other.duplicate_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDuplicateIsMutable();
duplicate_.addAll(other.duplicate_);
}
onChanged();
}
} else {
if (!other.duplicate_.isEmpty()) {
if (duplicateBuilder_.isEmpty()) {
duplicateBuilder_.dispose();
duplicateBuilder_ = null;
duplicate_ = other.duplicate_;
bitField0_ = (bitField0_ & ~0x00000002);
duplicateBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getDuplicateFieldBuilder() : null;
} else {
duplicateBuilder_.addAllMessages(other.duplicate_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Duplication parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Duplication) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange originPosition_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> originPositionBuilder_;
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public boolean hasOriginPosition() {
return originPositionBuilder_ != null || originPosition_ != null;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getOriginPosition() {
if (originPositionBuilder_ == null) {
return originPosition_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : originPosition_;
} else {
return originPositionBuilder_.getMessage();
}
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder setOriginPosition(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (originPositionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
originPosition_ = value;
onChanged();
} else {
originPositionBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder setOriginPosition(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (originPositionBuilder_ == null) {
originPosition_ = builderForValue.build();
onChanged();
} else {
originPositionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder mergeOriginPosition(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (originPositionBuilder_ == null) {
if (originPosition_ != null) {
originPosition_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(originPosition_).mergeFrom(value).buildPartial();
} else {
originPosition_ = value;
}
onChanged();
} else {
originPositionBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public Builder clearOriginPosition() {
if (originPositionBuilder_ == null) {
originPosition_ = null;
onChanged();
} else {
originPosition_ = null;
originPositionBuilder_ = null;
}
return this;
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getOriginPositionBuilder() {
onChanged();
return getOriginPositionFieldBuilder().getBuilder();
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getOriginPositionOrBuilder() {
if (originPositionBuilder_ != null) {
return originPositionBuilder_.getMessageOrBuilder();
} else {
return originPosition_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : originPosition_;
}
}
/**
* optional .TextRange origin_position = 1;
*
*
* Origin position in current file. Only start_line and end_line are provided since we dont support "precise" duplication location.
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getOriginPositionFieldBuilder() {
if (originPositionBuilder_ == null) {
originPositionBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getOriginPosition(),
getParentForChildren(),
isClean());
originPosition_ = null;
}
return originPositionBuilder_;
}
private java.util.List duplicate_ =
java.util.Collections.emptyList();
private void ensureDuplicateIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
duplicate_ = new java.util.ArrayList(duplicate_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Duplicate, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder, org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder> duplicateBuilder_;
/**
* repeated .Duplicate duplicate = 2;
*/
public java.util.List getDuplicateList() {
if (duplicateBuilder_ == null) {
return java.util.Collections.unmodifiableList(duplicate_);
} else {
return duplicateBuilder_.getMessageList();
}
}
/**
* repeated .Duplicate duplicate = 2;
*/
public int getDuplicateCount() {
if (duplicateBuilder_ == null) {
return duplicate_.size();
} else {
return duplicateBuilder_.getCount();
}
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate getDuplicate(int index) {
if (duplicateBuilder_ == null) {
return duplicate_.get(index);
} else {
return duplicateBuilder_.getMessage(index);
}
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder setDuplicate(
int index, org.sonar.scanner.protocol.output.ScannerReport.Duplicate value) {
if (duplicateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDuplicateIsMutable();
duplicate_.set(index, value);
onChanged();
} else {
duplicateBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder setDuplicate(
int index, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder builderForValue) {
if (duplicateBuilder_ == null) {
ensureDuplicateIsMutable();
duplicate_.set(index, builderForValue.build());
onChanged();
} else {
duplicateBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder addDuplicate(org.sonar.scanner.protocol.output.ScannerReport.Duplicate value) {
if (duplicateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDuplicateIsMutable();
duplicate_.add(value);
onChanged();
} else {
duplicateBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder addDuplicate(
int index, org.sonar.scanner.protocol.output.ScannerReport.Duplicate value) {
if (duplicateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDuplicateIsMutable();
duplicate_.add(index, value);
onChanged();
} else {
duplicateBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder addDuplicate(
org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder builderForValue) {
if (duplicateBuilder_ == null) {
ensureDuplicateIsMutable();
duplicate_.add(builderForValue.build());
onChanged();
} else {
duplicateBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder addDuplicate(
int index, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder builderForValue) {
if (duplicateBuilder_ == null) {
ensureDuplicateIsMutable();
duplicate_.add(index, builderForValue.build());
onChanged();
} else {
duplicateBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder addAllDuplicate(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.Duplicate> values) {
if (duplicateBuilder_ == null) {
ensureDuplicateIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, duplicate_);
onChanged();
} else {
duplicateBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder clearDuplicate() {
if (duplicateBuilder_ == null) {
duplicate_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
duplicateBuilder_.clear();
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public Builder removeDuplicate(int index) {
if (duplicateBuilder_ == null) {
ensureDuplicateIsMutable();
duplicate_.remove(index);
onChanged();
} else {
duplicateBuilder_.remove(index);
}
return this;
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder getDuplicateBuilder(
int index) {
return getDuplicateFieldBuilder().getBuilder(index);
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder getDuplicateOrBuilder(
int index) {
if (duplicateBuilder_ == null) {
return duplicate_.get(index); } else {
return duplicateBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Duplicate duplicate = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder>
getDuplicateOrBuilderList() {
if (duplicateBuilder_ != null) {
return duplicateBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(duplicate_);
}
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder addDuplicateBuilder() {
return getDuplicateFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.Duplicate.getDefaultInstance());
}
/**
* repeated .Duplicate duplicate = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder addDuplicateBuilder(
int index) {
return getDuplicateFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.getDefaultInstance());
}
/**
* repeated .Duplicate duplicate = 2;
*/
public java.util.List
getDuplicateBuilderList() {
return getDuplicateFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Duplicate, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder, org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder>
getDuplicateFieldBuilder() {
if (duplicateBuilder_ == null) {
duplicateBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.Duplicate, org.sonar.scanner.protocol.output.ScannerReport.Duplicate.Builder, org.sonar.scanner.protocol.output.ScannerReport.DuplicateOrBuilder>(
duplicate_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
duplicate_ = null;
}
return duplicateBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Duplication)
}
// @@protoc_insertion_point(class_scope:Duplication)
private static final org.sonar.scanner.protocol.output.ScannerReport.Duplication DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Duplication();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Duplication getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Duplication parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Duplication(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Duplication getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CpdTextBlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:CpdTextBlock)
com.google.protobuf.MessageOrBuilder {
/**
* optional string hash = 1;
*/
java.lang.String getHash();
/**
* optional string hash = 1;
*/
com.google.protobuf.ByteString
getHashBytes();
/**
* optional int32 start_line = 2;
*/
int getStartLine();
/**
* optional int32 end_line = 3;
*/
int getEndLine();
/**
* optional int32 start_token_index = 4;
*/
int getStartTokenIndex();
/**
* optional int32 end_token_index = 5;
*/
int getEndTokenIndex();
}
/**
* Protobuf type {@code CpdTextBlock}
*
*
* Used for cross project duplication
*
*/
public static final class CpdTextBlock extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:CpdTextBlock)
CpdTextBlockOrBuilder {
// Use CpdTextBlock.newBuilder() to construct.
private CpdTextBlock(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CpdTextBlock() {
hash_ = "";
startLine_ = 0;
endLine_ = 0;
startTokenIndex_ = 0;
endTokenIndex_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CpdTextBlock(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
hash_ = s;
break;
}
case 16: {
startLine_ = input.readInt32();
break;
}
case 24: {
endLine_ = input.readInt32();
break;
}
case 32: {
startTokenIndex_ = input.readInt32();
break;
}
case 40: {
endTokenIndex_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CpdTextBlock_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CpdTextBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.class, org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.Builder.class);
}
public static final int HASH_FIELD_NUMBER = 1;
private volatile java.lang.Object hash_;
/**
* optional string hash = 1;
*/
public java.lang.String getHash() {
java.lang.Object ref = hash_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hash_ = s;
return s;
}
}
/**
* optional string hash = 1;
*/
public com.google.protobuf.ByteString
getHashBytes() {
java.lang.Object ref = hash_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hash_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_LINE_FIELD_NUMBER = 2;
private int startLine_;
/**
* optional int32 start_line = 2;
*/
public int getStartLine() {
return startLine_;
}
public static final int END_LINE_FIELD_NUMBER = 3;
private int endLine_;
/**
* optional int32 end_line = 3;
*/
public int getEndLine() {
return endLine_;
}
public static final int START_TOKEN_INDEX_FIELD_NUMBER = 4;
private int startTokenIndex_;
/**
* optional int32 start_token_index = 4;
*/
public int getStartTokenIndex() {
return startTokenIndex_;
}
public static final int END_TOKEN_INDEX_FIELD_NUMBER = 5;
private int endTokenIndex_;
/**
* optional int32 end_token_index = 5;
*/
public int getEndTokenIndex() {
return endTokenIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getHashBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, hash_);
}
if (startLine_ != 0) {
output.writeInt32(2, startLine_);
}
if (endLine_ != 0) {
output.writeInt32(3, endLine_);
}
if (startTokenIndex_ != 0) {
output.writeInt32(4, startTokenIndex_);
}
if (endTokenIndex_ != 0) {
output.writeInt32(5, endTokenIndex_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getHashBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, hash_);
}
if (startLine_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, startLine_);
}
if (endLine_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, endLine_);
}
if (startTokenIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, startTokenIndex_);
}
if (endTokenIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, endTokenIndex_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CpdTextBlock}
*
*
* Used for cross project duplication
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:CpdTextBlock)
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlockOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CpdTextBlock_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CpdTextBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.class, org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
hash_ = "";
startLine_ = 0;
endLine_ = 0;
startTokenIndex_ = 0;
endTokenIndex_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CpdTextBlock_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock build() {
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock result = new org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock(this);
result.hash_ = hash_;
result.startLine_ = startLine_;
result.endLine_ = endLine_;
result.startTokenIndex_ = startTokenIndex_;
result.endTokenIndex_ = endTokenIndex_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock.getDefaultInstance()) return this;
if (!other.getHash().isEmpty()) {
hash_ = other.hash_;
onChanged();
}
if (other.getStartLine() != 0) {
setStartLine(other.getStartLine());
}
if (other.getEndLine() != 0) {
setEndLine(other.getEndLine());
}
if (other.getStartTokenIndex() != 0) {
setStartTokenIndex(other.getStartTokenIndex());
}
if (other.getEndTokenIndex() != 0) {
setEndTokenIndex(other.getEndTokenIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object hash_ = "";
/**
* optional string hash = 1;
*/
public java.lang.String getHash() {
java.lang.Object ref = hash_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hash_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string hash = 1;
*/
public com.google.protobuf.ByteString
getHashBytes() {
java.lang.Object ref = hash_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hash_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string hash = 1;
*/
public Builder setHash(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
hash_ = value;
onChanged();
return this;
}
/**
* optional string hash = 1;
*/
public Builder clearHash() {
hash_ = getDefaultInstance().getHash();
onChanged();
return this;
}
/**
* optional string hash = 1;
*/
public Builder setHashBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
hash_ = value;
onChanged();
return this;
}
private int startLine_ ;
/**
* optional int32 start_line = 2;
*/
public int getStartLine() {
return startLine_;
}
/**
* optional int32 start_line = 2;
*/
public Builder setStartLine(int value) {
startLine_ = value;
onChanged();
return this;
}
/**
* optional int32 start_line = 2;
*/
public Builder clearStartLine() {
startLine_ = 0;
onChanged();
return this;
}
private int endLine_ ;
/**
* optional int32 end_line = 3;
*/
public int getEndLine() {
return endLine_;
}
/**
* optional int32 end_line = 3;
*/
public Builder setEndLine(int value) {
endLine_ = value;
onChanged();
return this;
}
/**
* optional int32 end_line = 3;
*/
public Builder clearEndLine() {
endLine_ = 0;
onChanged();
return this;
}
private int startTokenIndex_ ;
/**
* optional int32 start_token_index = 4;
*/
public int getStartTokenIndex() {
return startTokenIndex_;
}
/**
* optional int32 start_token_index = 4;
*/
public Builder setStartTokenIndex(int value) {
startTokenIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 start_token_index = 4;
*/
public Builder clearStartTokenIndex() {
startTokenIndex_ = 0;
onChanged();
return this;
}
private int endTokenIndex_ ;
/**
* optional int32 end_token_index = 5;
*/
public int getEndTokenIndex() {
return endTokenIndex_;
}
/**
* optional int32 end_token_index = 5;
*/
public Builder setEndTokenIndex(int value) {
endTokenIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 end_token_index = 5;
*/
public Builder clearEndTokenIndex() {
endTokenIndex_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:CpdTextBlock)
}
// @@protoc_insertion_point(class_scope:CpdTextBlock)
private static final org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock();
}
public static org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CpdTextBlock parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new CpdTextBlock(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.CpdTextBlock getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TextRangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:TextRange)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 start_line = 1;
*/
int getStartLine();
/**
* optional int32 end_line = 2;
*
*
* End line (inclusive)
*
*/
int getEndLine();
/**
* optional int32 start_offset = 3;
*/
int getStartOffset();
/**
* optional int32 end_offset = 4;
*/
int getEndOffset();
}
/**
* Protobuf type {@code TextRange}
*
*
* Lines start at 1 and line offsets start at 0
*
*/
public static final class TextRange extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TextRange)
TextRangeOrBuilder {
// Use TextRange.newBuilder() to construct.
private TextRange(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TextRange() {
startLine_ = 0;
endLine_ = 0;
startOffset_ = 0;
endOffset_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private TextRange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
startLine_ = input.readInt32();
break;
}
case 16: {
endLine_ = input.readInt32();
break;
}
case 24: {
startOffset_ = input.readInt32();
break;
}
case 32: {
endOffset_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_TextRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_TextRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.class, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder.class);
}
public static final int START_LINE_FIELD_NUMBER = 1;
private int startLine_;
/**
* optional int32 start_line = 1;
*/
public int getStartLine() {
return startLine_;
}
public static final int END_LINE_FIELD_NUMBER = 2;
private int endLine_;
/**
* optional int32 end_line = 2;
*
*
* End line (inclusive)
*
*/
public int getEndLine() {
return endLine_;
}
public static final int START_OFFSET_FIELD_NUMBER = 3;
private int startOffset_;
/**
* optional int32 start_offset = 3;
*/
public int getStartOffset() {
return startOffset_;
}
public static final int END_OFFSET_FIELD_NUMBER = 4;
private int endOffset_;
/**
* optional int32 end_offset = 4;
*/
public int getEndOffset() {
return endOffset_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (startLine_ != 0) {
output.writeInt32(1, startLine_);
}
if (endLine_ != 0) {
output.writeInt32(2, endLine_);
}
if (startOffset_ != 0) {
output.writeInt32(3, startOffset_);
}
if (endOffset_ != 0) {
output.writeInt32(4, endOffset_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (startLine_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, startLine_);
}
if (endLine_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, endLine_);
}
if (startOffset_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, startOffset_);
}
if (endOffset_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, endOffset_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.TextRange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TextRange}
*
*
* Lines start at 1 and line offsets start at 0
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TextRange)
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_TextRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_TextRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.class, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
startLine_ = 0;
endLine_ = 0;
startOffset_ = 0;
endOffset_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_TextRange_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.TextRange build() {
org.sonar.scanner.protocol.output.ScannerReport.TextRange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.TextRange buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.TextRange result = new org.sonar.scanner.protocol.output.ScannerReport.TextRange(this);
result.startLine_ = startLine_;
result.endLine_ = endLine_;
result.startOffset_ = startOffset_;
result.endOffset_ = endOffset_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.TextRange) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.TextRange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.TextRange other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance()) return this;
if (other.getStartLine() != 0) {
setStartLine(other.getStartLine());
}
if (other.getEndLine() != 0) {
setEndLine(other.getEndLine());
}
if (other.getStartOffset() != 0) {
setStartOffset(other.getStartOffset());
}
if (other.getEndOffset() != 0) {
setEndOffset(other.getEndOffset());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.TextRange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.TextRange) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int startLine_ ;
/**
* optional int32 start_line = 1;
*/
public int getStartLine() {
return startLine_;
}
/**
* optional int32 start_line = 1;
*/
public Builder setStartLine(int value) {
startLine_ = value;
onChanged();
return this;
}
/**
* optional int32 start_line = 1;
*/
public Builder clearStartLine() {
startLine_ = 0;
onChanged();
return this;
}
private int endLine_ ;
/**
* optional int32 end_line = 2;
*
*
* End line (inclusive)
*
*/
public int getEndLine() {
return endLine_;
}
/**
* optional int32 end_line = 2;
*
*
* End line (inclusive)
*
*/
public Builder setEndLine(int value) {
endLine_ = value;
onChanged();
return this;
}
/**
* optional int32 end_line = 2;
*
*
* End line (inclusive)
*
*/
public Builder clearEndLine() {
endLine_ = 0;
onChanged();
return this;
}
private int startOffset_ ;
/**
* optional int32 start_offset = 3;
*/
public int getStartOffset() {
return startOffset_;
}
/**
* optional int32 start_offset = 3;
*/
public Builder setStartOffset(int value) {
startOffset_ = value;
onChanged();
return this;
}
/**
* optional int32 start_offset = 3;
*/
public Builder clearStartOffset() {
startOffset_ = 0;
onChanged();
return this;
}
private int endOffset_ ;
/**
* optional int32 end_offset = 4;
*/
public int getEndOffset() {
return endOffset_;
}
/**
* optional int32 end_offset = 4;
*/
public Builder setEndOffset(int value) {
endOffset_ = value;
onChanged();
return this;
}
/**
* optional int32 end_offset = 4;
*/
public Builder clearEndOffset() {
endOffset_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:TextRange)
}
// @@protoc_insertion_point(class_scope:TextRange)
private static final org.sonar.scanner.protocol.output.ScannerReport.TextRange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.TextRange();
}
public static org.sonar.scanner.protocol.output.ScannerReport.TextRange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TextRange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new TextRange(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SymbolOrBuilder extends
// @@protoc_insertion_point(interface_extends:Symbol)
com.google.protobuf.MessageOrBuilder {
/**
* optional .TextRange declaration = 1;
*/
boolean hasDeclaration();
/**
* optional .TextRange declaration = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getDeclaration();
/**
* optional .TextRange declaration = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getDeclarationOrBuilder();
/**
* repeated .TextRange reference = 2;
*/
java.util.List
getReferenceList();
/**
* repeated .TextRange reference = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getReference(int index);
/**
* repeated .TextRange reference = 2;
*/
int getReferenceCount();
/**
* repeated .TextRange reference = 2;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getReferenceOrBuilderList();
/**
* repeated .TextRange reference = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getReferenceOrBuilder(
int index);
}
/**
* Protobuf type {@code Symbol}
*/
public static final class Symbol extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Symbol)
SymbolOrBuilder {
// Use Symbol.newBuilder() to construct.
private Symbol(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Symbol() {
reference_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Symbol(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (declaration_ != null) {
subBuilder = declaration_.toBuilder();
}
declaration_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(declaration_);
declaration_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
reference_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
reference_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
reference_ = java.util.Collections.unmodifiableList(reference_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Symbol_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Symbol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Symbol.class, org.sonar.scanner.protocol.output.ScannerReport.Symbol.Builder.class);
}
private int bitField0_;
public static final int DECLARATION_FIELD_NUMBER = 1;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange declaration_;
/**
* optional .TextRange declaration = 1;
*/
public boolean hasDeclaration() {
return declaration_ != null;
}
/**
* optional .TextRange declaration = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getDeclaration() {
return declaration_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : declaration_;
}
/**
* optional .TextRange declaration = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getDeclarationOrBuilder() {
return getDeclaration();
}
public static final int REFERENCE_FIELD_NUMBER = 2;
private java.util.List reference_;
/**
* repeated .TextRange reference = 2;
*/
public java.util.List getReferenceList() {
return reference_;
}
/**
* repeated .TextRange reference = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getReferenceOrBuilderList() {
return reference_;
}
/**
* repeated .TextRange reference = 2;
*/
public int getReferenceCount() {
return reference_.size();
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getReference(int index) {
return reference_.get(index);
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getReferenceOrBuilder(
int index) {
return reference_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (declaration_ != null) {
output.writeMessage(1, getDeclaration());
}
for (int i = 0; i < reference_.size(); i++) {
output.writeMessage(2, reference_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (declaration_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getDeclaration());
}
for (int i = 0; i < reference_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, reference_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Symbol prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Symbol}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Symbol)
org.sonar.scanner.protocol.output.ScannerReport.SymbolOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Symbol_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Symbol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Symbol.class, org.sonar.scanner.protocol.output.ScannerReport.Symbol.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Symbol.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getReferenceFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (declarationBuilder_ == null) {
declaration_ = null;
} else {
declaration_ = null;
declarationBuilder_ = null;
}
if (referenceBuilder_ == null) {
reference_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
referenceBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Symbol_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Symbol getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Symbol.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Symbol build() {
org.sonar.scanner.protocol.output.ScannerReport.Symbol result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Symbol buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Symbol result = new org.sonar.scanner.protocol.output.ScannerReport.Symbol(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (declarationBuilder_ == null) {
result.declaration_ = declaration_;
} else {
result.declaration_ = declarationBuilder_.build();
}
if (referenceBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
reference_ = java.util.Collections.unmodifiableList(reference_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.reference_ = reference_;
} else {
result.reference_ = referenceBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Symbol) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Symbol)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Symbol other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Symbol.getDefaultInstance()) return this;
if (other.hasDeclaration()) {
mergeDeclaration(other.getDeclaration());
}
if (referenceBuilder_ == null) {
if (!other.reference_.isEmpty()) {
if (reference_.isEmpty()) {
reference_ = other.reference_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureReferenceIsMutable();
reference_.addAll(other.reference_);
}
onChanged();
}
} else {
if (!other.reference_.isEmpty()) {
if (referenceBuilder_.isEmpty()) {
referenceBuilder_.dispose();
referenceBuilder_ = null;
reference_ = other.reference_;
bitField0_ = (bitField0_ & ~0x00000002);
referenceBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getReferenceFieldBuilder() : null;
} else {
referenceBuilder_.addAllMessages(other.reference_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Symbol parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Symbol) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange declaration_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> declarationBuilder_;
/**
* optional .TextRange declaration = 1;
*/
public boolean hasDeclaration() {
return declarationBuilder_ != null || declaration_ != null;
}
/**
* optional .TextRange declaration = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getDeclaration() {
if (declarationBuilder_ == null) {
return declaration_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : declaration_;
} else {
return declarationBuilder_.getMessage();
}
}
/**
* optional .TextRange declaration = 1;
*/
public Builder setDeclaration(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (declarationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
declaration_ = value;
onChanged();
} else {
declarationBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange declaration = 1;
*/
public Builder setDeclaration(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (declarationBuilder_ == null) {
declaration_ = builderForValue.build();
onChanged();
} else {
declarationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange declaration = 1;
*/
public Builder mergeDeclaration(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (declarationBuilder_ == null) {
if (declaration_ != null) {
declaration_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(declaration_).mergeFrom(value).buildPartial();
} else {
declaration_ = value;
}
onChanged();
} else {
declarationBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange declaration = 1;
*/
public Builder clearDeclaration() {
if (declarationBuilder_ == null) {
declaration_ = null;
onChanged();
} else {
declaration_ = null;
declarationBuilder_ = null;
}
return this;
}
/**
* optional .TextRange declaration = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getDeclarationBuilder() {
onChanged();
return getDeclarationFieldBuilder().getBuilder();
}
/**
* optional .TextRange declaration = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getDeclarationOrBuilder() {
if (declarationBuilder_ != null) {
return declarationBuilder_.getMessageOrBuilder();
} else {
return declaration_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : declaration_;
}
}
/**
* optional .TextRange declaration = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getDeclarationFieldBuilder() {
if (declarationBuilder_ == null) {
declarationBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getDeclaration(),
getParentForChildren(),
isClean());
declaration_ = null;
}
return declarationBuilder_;
}
private java.util.List reference_ =
java.util.Collections.emptyList();
private void ensureReferenceIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
reference_ = new java.util.ArrayList(reference_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> referenceBuilder_;
/**
* repeated .TextRange reference = 2;
*/
public java.util.List getReferenceList() {
if (referenceBuilder_ == null) {
return java.util.Collections.unmodifiableList(reference_);
} else {
return referenceBuilder_.getMessageList();
}
}
/**
* repeated .TextRange reference = 2;
*/
public int getReferenceCount() {
if (referenceBuilder_ == null) {
return reference_.size();
} else {
return referenceBuilder_.getCount();
}
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getReference(int index) {
if (referenceBuilder_ == null) {
return reference_.get(index);
} else {
return referenceBuilder_.getMessage(index);
}
}
/**
* repeated .TextRange reference = 2;
*/
public Builder setReference(
int index, org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (referenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferenceIsMutable();
reference_.set(index, value);
onChanged();
} else {
referenceBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder setReference(
int index, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (referenceBuilder_ == null) {
ensureReferenceIsMutable();
reference_.set(index, builderForValue.build());
onChanged();
} else {
referenceBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder addReference(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (referenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferenceIsMutable();
reference_.add(value);
onChanged();
} else {
referenceBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder addReference(
int index, org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (referenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReferenceIsMutable();
reference_.add(index, value);
onChanged();
} else {
referenceBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder addReference(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (referenceBuilder_ == null) {
ensureReferenceIsMutable();
reference_.add(builderForValue.build());
onChanged();
} else {
referenceBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder addReference(
int index, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (referenceBuilder_ == null) {
ensureReferenceIsMutable();
reference_.add(index, builderForValue.build());
onChanged();
} else {
referenceBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder addAllReference(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.TextRange> values) {
if (referenceBuilder_ == null) {
ensureReferenceIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, reference_);
onChanged();
} else {
referenceBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder clearReference() {
if (referenceBuilder_ == null) {
reference_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
referenceBuilder_.clear();
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public Builder removeReference(int index) {
if (referenceBuilder_ == null) {
ensureReferenceIsMutable();
reference_.remove(index);
onChanged();
} else {
referenceBuilder_.remove(index);
}
return this;
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getReferenceBuilder(
int index) {
return getReferenceFieldBuilder().getBuilder(index);
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getReferenceOrBuilder(
int index) {
if (referenceBuilder_ == null) {
return reference_.get(index); } else {
return referenceBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TextRange reference = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getReferenceOrBuilderList() {
if (referenceBuilder_ != null) {
return referenceBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(reference_);
}
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder addReferenceBuilder() {
return getReferenceFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance());
}
/**
* repeated .TextRange reference = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder addReferenceBuilder(
int index) {
return getReferenceFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance());
}
/**
* repeated .TextRange reference = 2;
*/
public java.util.List
getReferenceBuilderList() {
return getReferenceFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getReferenceFieldBuilder() {
if (referenceBuilder_ == null) {
referenceBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
reference_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
reference_ = null;
}
return referenceBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Symbol)
}
// @@protoc_insertion_point(class_scope:Symbol)
private static final org.sonar.scanner.protocol.output.ScannerReport.Symbol DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Symbol();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Symbol getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Symbol parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Symbol(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Symbol getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LineCoverageOrBuilder extends
// @@protoc_insertion_point(interface_extends:LineCoverage)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 line = 1;
*/
int getLine();
/**
* optional int32 conditions = 2;
*
*
* Number of conditions to cover (if set, the value must be greater than 0)
*
*/
int getConditions();
/**
* optional bool ut_hits = 3;
*/
boolean getUtHits();
/**
* optional bool it_hits = 4;
*/
boolean getItHits();
/**
* optional int32 ut_covered_conditions = 5;
*/
int getUtCoveredConditions();
/**
* optional int32 it_covered_conditions = 6;
*/
int getItCoveredConditions();
/**
* optional int32 overall_covered_conditions = 7;
*/
int getOverallCoveredConditions();
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.HasUtHitsCase getHasUtHitsCase();
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.HasItHitsCase getHasItHitsCase();
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.HasUtCoveredConditionsCase getHasUtCoveredConditionsCase();
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.HasItCoveredConditionsCase getHasItCoveredConditionsCase();
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.HasOverallCoveredConditionsCase getHasOverallCoveredConditionsCase();
}
/**
* Protobuf type {@code LineCoverage}
*
*
* Only FILE component has coverage information, and only executable lines should contains this information.
*
*/
public static final class LineCoverage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:LineCoverage)
LineCoverageOrBuilder {
// Use LineCoverage.newBuilder() to construct.
private LineCoverage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private LineCoverage() {
line_ = 0;
conditions_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private LineCoverage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
line_ = input.readInt32();
break;
}
case 16: {
conditions_ = input.readInt32();
break;
}
case 24: {
hasUtHitsCase_ = 3;
hasUtHits_ = input.readBool();
break;
}
case 32: {
hasItHitsCase_ = 4;
hasItHits_ = input.readBool();
break;
}
case 40: {
hasUtCoveredConditionsCase_ = 5;
hasUtCoveredConditions_ = input.readInt32();
break;
}
case 48: {
hasItCoveredConditionsCase_ = 6;
hasItCoveredConditions_ = input.readInt32();
break;
}
case 56: {
hasOverallCoveredConditionsCase_ = 7;
hasOverallCoveredConditions_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_LineCoverage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_LineCoverage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.class, org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.Builder.class);
}
private int hasUtHitsCase_ = 0;
private java.lang.Object hasUtHits_;
public enum HasUtHitsCase
implements com.google.protobuf.Internal.EnumLite {
UT_HITS(3),
HASUTHITS_NOT_SET(0);
private int value = 0;
private HasUtHitsCase(int value) {
this.value = value;
}
public static HasUtHitsCase valueOf(int value) {
switch (value) {
case 3: return UT_HITS;
case 0: return HASUTHITS_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public HasUtHitsCase
getHasUtHitsCase() {
return HasUtHitsCase.valueOf(
hasUtHitsCase_);
}
private int hasItHitsCase_ = 0;
private java.lang.Object hasItHits_;
public enum HasItHitsCase
implements com.google.protobuf.Internal.EnumLite {
IT_HITS(4),
HASITHITS_NOT_SET(0);
private int value = 0;
private HasItHitsCase(int value) {
this.value = value;
}
public static HasItHitsCase valueOf(int value) {
switch (value) {
case 4: return IT_HITS;
case 0: return HASITHITS_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public HasItHitsCase
getHasItHitsCase() {
return HasItHitsCase.valueOf(
hasItHitsCase_);
}
private int hasUtCoveredConditionsCase_ = 0;
private java.lang.Object hasUtCoveredConditions_;
public enum HasUtCoveredConditionsCase
implements com.google.protobuf.Internal.EnumLite {
UT_COVERED_CONDITIONS(5),
HASUTCOVEREDCONDITIONS_NOT_SET(0);
private int value = 0;
private HasUtCoveredConditionsCase(int value) {
this.value = value;
}
public static HasUtCoveredConditionsCase valueOf(int value) {
switch (value) {
case 5: return UT_COVERED_CONDITIONS;
case 0: return HASUTCOVEREDCONDITIONS_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public HasUtCoveredConditionsCase
getHasUtCoveredConditionsCase() {
return HasUtCoveredConditionsCase.valueOf(
hasUtCoveredConditionsCase_);
}
private int hasItCoveredConditionsCase_ = 0;
private java.lang.Object hasItCoveredConditions_;
public enum HasItCoveredConditionsCase
implements com.google.protobuf.Internal.EnumLite {
IT_COVERED_CONDITIONS(6),
HASITCOVEREDCONDITIONS_NOT_SET(0);
private int value = 0;
private HasItCoveredConditionsCase(int value) {
this.value = value;
}
public static HasItCoveredConditionsCase valueOf(int value) {
switch (value) {
case 6: return IT_COVERED_CONDITIONS;
case 0: return HASITCOVEREDCONDITIONS_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public HasItCoveredConditionsCase
getHasItCoveredConditionsCase() {
return HasItCoveredConditionsCase.valueOf(
hasItCoveredConditionsCase_);
}
private int hasOverallCoveredConditionsCase_ = 0;
private java.lang.Object hasOverallCoveredConditions_;
public enum HasOverallCoveredConditionsCase
implements com.google.protobuf.Internal.EnumLite {
OVERALL_COVERED_CONDITIONS(7),
HASOVERALLCOVEREDCONDITIONS_NOT_SET(0);
private int value = 0;
private HasOverallCoveredConditionsCase(int value) {
this.value = value;
}
public static HasOverallCoveredConditionsCase valueOf(int value) {
switch (value) {
case 7: return OVERALL_COVERED_CONDITIONS;
case 0: return HASOVERALLCOVEREDCONDITIONS_NOT_SET;
default: throw new java.lang.IllegalArgumentException(
"Value is undefined for this oneof enum.");
}
}
public int getNumber() {
return this.value;
}
};
public HasOverallCoveredConditionsCase
getHasOverallCoveredConditionsCase() {
return HasOverallCoveredConditionsCase.valueOf(
hasOverallCoveredConditionsCase_);
}
public static final int LINE_FIELD_NUMBER = 1;
private int line_;
/**
* optional int32 line = 1;
*/
public int getLine() {
return line_;
}
public static final int CONDITIONS_FIELD_NUMBER = 2;
private int conditions_;
/**
* optional int32 conditions = 2;
*
*
* Number of conditions to cover (if set, the value must be greater than 0)
*
*/
public int getConditions() {
return conditions_;
}
public static final int UT_HITS_FIELD_NUMBER = 3;
/**
* optional bool ut_hits = 3;
*/
public boolean getUtHits() {
if (hasUtHitsCase_ == 3) {
return (java.lang.Boolean) hasUtHits_;
}
return false;
}
public static final int IT_HITS_FIELD_NUMBER = 4;
/**
* optional bool it_hits = 4;
*/
public boolean getItHits() {
if (hasItHitsCase_ == 4) {
return (java.lang.Boolean) hasItHits_;
}
return false;
}
public static final int UT_COVERED_CONDITIONS_FIELD_NUMBER = 5;
/**
* optional int32 ut_covered_conditions = 5;
*/
public int getUtCoveredConditions() {
if (hasUtCoveredConditionsCase_ == 5) {
return (java.lang.Integer) hasUtCoveredConditions_;
}
return 0;
}
public static final int IT_COVERED_CONDITIONS_FIELD_NUMBER = 6;
/**
* optional int32 it_covered_conditions = 6;
*/
public int getItCoveredConditions() {
if (hasItCoveredConditionsCase_ == 6) {
return (java.lang.Integer) hasItCoveredConditions_;
}
return 0;
}
public static final int OVERALL_COVERED_CONDITIONS_FIELD_NUMBER = 7;
/**
* optional int32 overall_covered_conditions = 7;
*/
public int getOverallCoveredConditions() {
if (hasOverallCoveredConditionsCase_ == 7) {
return (java.lang.Integer) hasOverallCoveredConditions_;
}
return 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (line_ != 0) {
output.writeInt32(1, line_);
}
if (conditions_ != 0) {
output.writeInt32(2, conditions_);
}
if (hasUtHitsCase_ == 3) {
output.writeBool(
3, (boolean)((java.lang.Boolean) hasUtHits_));
}
if (hasItHitsCase_ == 4) {
output.writeBool(
4, (boolean)((java.lang.Boolean) hasItHits_));
}
if (hasUtCoveredConditionsCase_ == 5) {
output.writeInt32(
5, (int)((java.lang.Integer) hasUtCoveredConditions_));
}
if (hasItCoveredConditionsCase_ == 6) {
output.writeInt32(
6, (int)((java.lang.Integer) hasItCoveredConditions_));
}
if (hasOverallCoveredConditionsCase_ == 7) {
output.writeInt32(
7, (int)((java.lang.Integer) hasOverallCoveredConditions_));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (line_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, line_);
}
if (conditions_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, conditions_);
}
if (hasUtHitsCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
3, (boolean)((java.lang.Boolean) hasUtHits_));
}
if (hasItHitsCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
4, (boolean)((java.lang.Boolean) hasItHits_));
}
if (hasUtCoveredConditionsCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
5, (int)((java.lang.Integer) hasUtCoveredConditions_));
}
if (hasItCoveredConditionsCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
6, (int)((java.lang.Integer) hasItCoveredConditions_));
}
if (hasOverallCoveredConditionsCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
7, (int)((java.lang.Integer) hasOverallCoveredConditions_));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.LineCoverage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code LineCoverage}
*
*
* Only FILE component has coverage information, and only executable lines should contains this information.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:LineCoverage)
org.sonar.scanner.protocol.output.ScannerReport.LineCoverageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_LineCoverage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_LineCoverage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.class, org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
line_ = 0;
conditions_ = 0;
hasUtHitsCase_ = 0;
hasUtHits_ = null;
hasItHitsCase_ = 0;
hasItHits_ = null;
hasUtCoveredConditionsCase_ = 0;
hasUtCoveredConditions_ = null;
hasItCoveredConditionsCase_ = 0;
hasItCoveredConditions_ = null;
hasOverallCoveredConditionsCase_ = 0;
hasOverallCoveredConditions_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_LineCoverage_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage build() {
org.sonar.scanner.protocol.output.ScannerReport.LineCoverage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.LineCoverage result = new org.sonar.scanner.protocol.output.ScannerReport.LineCoverage(this);
result.line_ = line_;
result.conditions_ = conditions_;
if (hasUtHitsCase_ == 3) {
result.hasUtHits_ = hasUtHits_;
}
if (hasItHitsCase_ == 4) {
result.hasItHits_ = hasItHits_;
}
if (hasUtCoveredConditionsCase_ == 5) {
result.hasUtCoveredConditions_ = hasUtCoveredConditions_;
}
if (hasItCoveredConditionsCase_ == 6) {
result.hasItCoveredConditions_ = hasItCoveredConditions_;
}
if (hasOverallCoveredConditionsCase_ == 7) {
result.hasOverallCoveredConditions_ = hasOverallCoveredConditions_;
}
result.hasUtHitsCase_ = hasUtHitsCase_;
result.hasItHitsCase_ = hasItHitsCase_;
result.hasUtCoveredConditionsCase_ = hasUtCoveredConditionsCase_;
result.hasItCoveredConditionsCase_ = hasItCoveredConditionsCase_;
result.hasOverallCoveredConditionsCase_ = hasOverallCoveredConditionsCase_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.LineCoverage) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.LineCoverage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.LineCoverage other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.LineCoverage.getDefaultInstance()) return this;
if (other.getLine() != 0) {
setLine(other.getLine());
}
if (other.getConditions() != 0) {
setConditions(other.getConditions());
}
switch (other.getHasUtHitsCase()) {
case UT_HITS: {
setUtHits(other.getUtHits());
break;
}
case HASUTHITS_NOT_SET: {
break;
}
}
switch (other.getHasItHitsCase()) {
case IT_HITS: {
setItHits(other.getItHits());
break;
}
case HASITHITS_NOT_SET: {
break;
}
}
switch (other.getHasUtCoveredConditionsCase()) {
case UT_COVERED_CONDITIONS: {
setUtCoveredConditions(other.getUtCoveredConditions());
break;
}
case HASUTCOVEREDCONDITIONS_NOT_SET: {
break;
}
}
switch (other.getHasItCoveredConditionsCase()) {
case IT_COVERED_CONDITIONS: {
setItCoveredConditions(other.getItCoveredConditions());
break;
}
case HASITCOVEREDCONDITIONS_NOT_SET: {
break;
}
}
switch (other.getHasOverallCoveredConditionsCase()) {
case OVERALL_COVERED_CONDITIONS: {
setOverallCoveredConditions(other.getOverallCoveredConditions());
break;
}
case HASOVERALLCOVEREDCONDITIONS_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.LineCoverage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.LineCoverage) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int hasUtHitsCase_ = 0;
private java.lang.Object hasUtHits_;
public HasUtHitsCase
getHasUtHitsCase() {
return HasUtHitsCase.valueOf(
hasUtHitsCase_);
}
public Builder clearHasUtHits() {
hasUtHitsCase_ = 0;
hasUtHits_ = null;
onChanged();
return this;
}
private int hasItHitsCase_ = 0;
private java.lang.Object hasItHits_;
public HasItHitsCase
getHasItHitsCase() {
return HasItHitsCase.valueOf(
hasItHitsCase_);
}
public Builder clearHasItHits() {
hasItHitsCase_ = 0;
hasItHits_ = null;
onChanged();
return this;
}
private int hasUtCoveredConditionsCase_ = 0;
private java.lang.Object hasUtCoveredConditions_;
public HasUtCoveredConditionsCase
getHasUtCoveredConditionsCase() {
return HasUtCoveredConditionsCase.valueOf(
hasUtCoveredConditionsCase_);
}
public Builder clearHasUtCoveredConditions() {
hasUtCoveredConditionsCase_ = 0;
hasUtCoveredConditions_ = null;
onChanged();
return this;
}
private int hasItCoveredConditionsCase_ = 0;
private java.lang.Object hasItCoveredConditions_;
public HasItCoveredConditionsCase
getHasItCoveredConditionsCase() {
return HasItCoveredConditionsCase.valueOf(
hasItCoveredConditionsCase_);
}
public Builder clearHasItCoveredConditions() {
hasItCoveredConditionsCase_ = 0;
hasItCoveredConditions_ = null;
onChanged();
return this;
}
private int hasOverallCoveredConditionsCase_ = 0;
private java.lang.Object hasOverallCoveredConditions_;
public HasOverallCoveredConditionsCase
getHasOverallCoveredConditionsCase() {
return HasOverallCoveredConditionsCase.valueOf(
hasOverallCoveredConditionsCase_);
}
public Builder clearHasOverallCoveredConditions() {
hasOverallCoveredConditionsCase_ = 0;
hasOverallCoveredConditions_ = null;
onChanged();
return this;
}
private int line_ ;
/**
* optional int32 line = 1;
*/
public int getLine() {
return line_;
}
/**
* optional int32 line = 1;
*/
public Builder setLine(int value) {
line_ = value;
onChanged();
return this;
}
/**
* optional int32 line = 1;
*/
public Builder clearLine() {
line_ = 0;
onChanged();
return this;
}
private int conditions_ ;
/**
* optional int32 conditions = 2;
*
*
* Number of conditions to cover (if set, the value must be greater than 0)
*
*/
public int getConditions() {
return conditions_;
}
/**
* optional int32 conditions = 2;
*
*
* Number of conditions to cover (if set, the value must be greater than 0)
*
*/
public Builder setConditions(int value) {
conditions_ = value;
onChanged();
return this;
}
/**
* optional int32 conditions = 2;
*
*
* Number of conditions to cover (if set, the value must be greater than 0)
*
*/
public Builder clearConditions() {
conditions_ = 0;
onChanged();
return this;
}
/**
* optional bool ut_hits = 3;
*/
public boolean getUtHits() {
if (hasUtHitsCase_ == 3) {
return (java.lang.Boolean) hasUtHits_;
}
return false;
}
/**
* optional bool ut_hits = 3;
*/
public Builder setUtHits(boolean value) {
hasUtHitsCase_ = 3;
hasUtHits_ = value;
onChanged();
return this;
}
/**
* optional bool ut_hits = 3;
*/
public Builder clearUtHits() {
if (hasUtHitsCase_ == 3) {
hasUtHitsCase_ = 0;
hasUtHits_ = null;
onChanged();
}
return this;
}
/**
* optional bool it_hits = 4;
*/
public boolean getItHits() {
if (hasItHitsCase_ == 4) {
return (java.lang.Boolean) hasItHits_;
}
return false;
}
/**
* optional bool it_hits = 4;
*/
public Builder setItHits(boolean value) {
hasItHitsCase_ = 4;
hasItHits_ = value;
onChanged();
return this;
}
/**
* optional bool it_hits = 4;
*/
public Builder clearItHits() {
if (hasItHitsCase_ == 4) {
hasItHitsCase_ = 0;
hasItHits_ = null;
onChanged();
}
return this;
}
/**
* optional int32 ut_covered_conditions = 5;
*/
public int getUtCoveredConditions() {
if (hasUtCoveredConditionsCase_ == 5) {
return (java.lang.Integer) hasUtCoveredConditions_;
}
return 0;
}
/**
* optional int32 ut_covered_conditions = 5;
*/
public Builder setUtCoveredConditions(int value) {
hasUtCoveredConditionsCase_ = 5;
hasUtCoveredConditions_ = value;
onChanged();
return this;
}
/**
* optional int32 ut_covered_conditions = 5;
*/
public Builder clearUtCoveredConditions() {
if (hasUtCoveredConditionsCase_ == 5) {
hasUtCoveredConditionsCase_ = 0;
hasUtCoveredConditions_ = null;
onChanged();
}
return this;
}
/**
* optional int32 it_covered_conditions = 6;
*/
public int getItCoveredConditions() {
if (hasItCoveredConditionsCase_ == 6) {
return (java.lang.Integer) hasItCoveredConditions_;
}
return 0;
}
/**
* optional int32 it_covered_conditions = 6;
*/
public Builder setItCoveredConditions(int value) {
hasItCoveredConditionsCase_ = 6;
hasItCoveredConditions_ = value;
onChanged();
return this;
}
/**
* optional int32 it_covered_conditions = 6;
*/
public Builder clearItCoveredConditions() {
if (hasItCoveredConditionsCase_ == 6) {
hasItCoveredConditionsCase_ = 0;
hasItCoveredConditions_ = null;
onChanged();
}
return this;
}
/**
* optional int32 overall_covered_conditions = 7;
*/
public int getOverallCoveredConditions() {
if (hasOverallCoveredConditionsCase_ == 7) {
return (java.lang.Integer) hasOverallCoveredConditions_;
}
return 0;
}
/**
* optional int32 overall_covered_conditions = 7;
*/
public Builder setOverallCoveredConditions(int value) {
hasOverallCoveredConditionsCase_ = 7;
hasOverallCoveredConditions_ = value;
onChanged();
return this;
}
/**
* optional int32 overall_covered_conditions = 7;
*/
public Builder clearOverallCoveredConditions() {
if (hasOverallCoveredConditionsCase_ == 7) {
hasOverallCoveredConditionsCase_ = 0;
hasOverallCoveredConditions_ = null;
onChanged();
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:LineCoverage)
}
// @@protoc_insertion_point(class_scope:LineCoverage)
private static final org.sonar.scanner.protocol.output.ScannerReport.LineCoverage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.LineCoverage();
}
public static org.sonar.scanner.protocol.output.ScannerReport.LineCoverage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LineCoverage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new LineCoverage(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.LineCoverage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SyntaxHighlightingRuleOrBuilder extends
// @@protoc_insertion_point(interface_extends:SyntaxHighlightingRule)
com.google.protobuf.MessageOrBuilder {
/**
* optional .TextRange range = 1;
*/
boolean hasRange();
/**
* optional .TextRange range = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange();
/**
* optional .TextRange range = 1;
*/
org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder();
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
int getTypeValue();
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType getType();
}
/**
* Protobuf type {@code SyntaxHighlightingRule}
*
*
* Must be sorted by line and start offset
*
*/
public static final class SyntaxHighlightingRule extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:SyntaxHighlightingRule)
SyntaxHighlightingRuleOrBuilder {
// Use SyntaxHighlightingRule.newBuilder() to construct.
private SyntaxHighlightingRule(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private SyntaxHighlightingRule() {
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private SyntaxHighlightingRule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder subBuilder = null;
if (range_ != null) {
subBuilder = range_.toBuilder();
}
range_ = input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.TextRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(range_);
range_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_SyntaxHighlightingRule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_SyntaxHighlightingRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.class, org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.Builder.class);
}
/**
* Protobuf enum {@code SyntaxHighlightingRule.HighlightingType}
*/
public enum HighlightingType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNSET = 0;
*/
UNSET(0, 0),
/**
* ANNOTATION = 1;
*/
ANNOTATION(1, 1),
/**
* CONSTANT = 2;
*/
CONSTANT(2, 2),
/**
* COMMENT = 3;
*/
COMMENT(3, 3),
/**
* CPP_DOC = 4;
*/
CPP_DOC(4, 4),
/**
* STRUCTURED_COMMENT = 5;
*/
STRUCTURED_COMMENT(5, 5),
/**
* KEYWORD = 6;
*/
KEYWORD(6, 6),
/**
* HIGHLIGHTING_STRING = 7;
*/
HIGHLIGHTING_STRING(7, 7),
/**
* KEYWORD_LIGHT = 8;
*/
KEYWORD_LIGHT(8, 8),
/**
* PREPROCESS_DIRECTIVE = 9;
*/
PREPROCESS_DIRECTIVE(9, 9),
UNRECOGNIZED(-1, -1),
;
/**
* UNSET = 0;
*/
public static final int UNSET_VALUE = 0;
/**
* ANNOTATION = 1;
*/
public static final int ANNOTATION_VALUE = 1;
/**
* CONSTANT = 2;
*/
public static final int CONSTANT_VALUE = 2;
/**
* COMMENT = 3;
*/
public static final int COMMENT_VALUE = 3;
/**
* CPP_DOC = 4;
*/
public static final int CPP_DOC_VALUE = 4;
/**
* STRUCTURED_COMMENT = 5;
*/
public static final int STRUCTURED_COMMENT_VALUE = 5;
/**
* KEYWORD = 6;
*/
public static final int KEYWORD_VALUE = 6;
/**
* HIGHLIGHTING_STRING = 7;
*/
public static final int HIGHLIGHTING_STRING_VALUE = 7;
/**
* KEYWORD_LIGHT = 8;
*/
public static final int KEYWORD_LIGHT_VALUE = 8;
/**
* PREPROCESS_DIRECTIVE = 9;
*/
public static final int PREPROCESS_DIRECTIVE_VALUE = 9;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
public static HighlightingType valueOf(int value) {
switch (value) {
case 0: return UNSET;
case 1: return ANNOTATION;
case 2: return CONSTANT;
case 3: return COMMENT;
case 4: return CPP_DOC;
case 5: return STRUCTURED_COMMENT;
case 6: return KEYWORD;
case 7: return HIGHLIGHTING_STRING;
case 8: return KEYWORD_LIGHT;
case 9: return PREPROCESS_DIRECTIVE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
HighlightingType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public HighlightingType findValueByNumber(int number) {
return HighlightingType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.getDescriptor().getEnumTypes().get(0);
}
private static final HighlightingType[] VALUES = values();
public static HighlightingType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private HighlightingType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:SyntaxHighlightingRule.HighlightingType)
}
public static final int RANGE_FIELD_NUMBER = 1;
private org.sonar.scanner.protocol.output.ScannerReport.TextRange range_;
/**
* optional .TextRange range = 1;
*/
public boolean hasRange() {
return range_ != null;
}
/**
* optional .TextRange range = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange() {
return range_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
}
/**
* optional .TextRange range = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder() {
return getRange();
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType getType() {
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType result = org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (range_ != null) {
output.writeMessage(1, getRange());
}
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.UNSET.getNumber()) {
output.writeEnum(2, type_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (range_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRange());
}
if (type_ != org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code SyntaxHighlightingRule}
*
*
* Must be sorted by line and start offset
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:SyntaxHighlightingRule)
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_SyntaxHighlightingRule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_SyntaxHighlightingRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.class, org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (rangeBuilder_ == null) {
range_ = null;
} else {
range_ = null;
rangeBuilder_ = null;
}
type_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_SyntaxHighlightingRule_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule build() {
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule result = new org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule(this);
if (rangeBuilder_ == null) {
result.range_ = range_;
} else {
result.range_ = rangeBuilder_.build();
}
result.type_ = type_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.getDefaultInstance()) return this;
if (other.hasRange()) {
mergeRange(other.getRange());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private org.sonar.scanner.protocol.output.ScannerReport.TextRange range_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder> rangeBuilder_;
/**
* optional .TextRange range = 1;
*/
public boolean hasRange() {
return rangeBuilder_ != null || range_ != null;
}
/**
* optional .TextRange range = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange getRange() {
if (rangeBuilder_ == null) {
return range_ == null ? org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
} else {
return rangeBuilder_.getMessage();
}
}
/**
* optional .TextRange range = 1;
*/
public Builder setRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
range_ = value;
onChanged();
} else {
rangeBuilder_.setMessage(value);
}
return this;
}
/**
* optional .TextRange range = 1;
*/
public Builder setRange(
org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder builderForValue) {
if (rangeBuilder_ == null) {
range_ = builderForValue.build();
onChanged();
} else {
rangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .TextRange range = 1;
*/
public Builder mergeRange(org.sonar.scanner.protocol.output.ScannerReport.TextRange value) {
if (rangeBuilder_ == null) {
if (range_ != null) {
range_ =
org.sonar.scanner.protocol.output.ScannerReport.TextRange.newBuilder(range_).mergeFrom(value).buildPartial();
} else {
range_ = value;
}
onChanged();
} else {
rangeBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .TextRange range = 1;
*/
public Builder clearRange() {
if (rangeBuilder_ == null) {
range_ = null;
onChanged();
} else {
range_ = null;
rangeBuilder_ = null;
}
return this;
}
/**
* optional .TextRange range = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder getRangeBuilder() {
onChanged();
return getRangeFieldBuilder().getBuilder();
}
/**
* optional .TextRange range = 1;
*/
public org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder getRangeOrBuilder() {
if (rangeBuilder_ != null) {
return rangeBuilder_.getMessageOrBuilder();
} else {
return range_ == null ?
org.sonar.scanner.protocol.output.ScannerReport.TextRange.getDefaultInstance() : range_;
}
}
/**
* optional .TextRange range = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>
getRangeFieldBuilder() {
if (rangeBuilder_ == null) {
rangeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.TextRange, org.sonar.scanner.protocol.output.ScannerReport.TextRange.Builder, org.sonar.scanner.protocol.output.ScannerReport.TextRangeOrBuilder>(
getRange(),
getParentForChildren(),
isClean());
range_ = null;
}
return rangeBuilder_;
}
private int type_ = 0;
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType getType() {
org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType result = org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.valueOf(type_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType.UNRECOGNIZED : result;
}
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public Builder setType(org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule.HighlightingType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .SyntaxHighlightingRule.HighlightingType type = 2;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:SyntaxHighlightingRule)
}
// @@protoc_insertion_point(class_scope:SyntaxHighlightingRule)
private static final org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule();
}
public static org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SyntaxHighlightingRule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new SyntaxHighlightingRule(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.SyntaxHighlightingRule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TestOrBuilder extends
// @@protoc_insertion_point(interface_extends:Test)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
*/
java.lang.String getName();
/**
* optional string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional .Test.TestStatus status = 2;
*/
int getStatusValue();
/**
* optional .Test.TestStatus status = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus getStatus();
/**
* optional int64 duration_in_ms = 3;
*/
long getDurationInMs();
/**
* optional string stacktrace = 4;
*/
java.lang.String getStacktrace();
/**
* optional string stacktrace = 4;
*/
com.google.protobuf.ByteString
getStacktraceBytes();
/**
* optional string msg = 5;
*/
java.lang.String getMsg();
/**
* optional string msg = 5;
*/
com.google.protobuf.ByteString
getMsgBytes();
}
/**
* Protobuf type {@code Test}
*/
public static final class Test extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:Test)
TestOrBuilder {
// Use Test.newBuilder() to construct.
private Test(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Test() {
name_ = "";
status_ = 0;
durationInMs_ = 0L;
stacktrace_ = "";
msg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Test(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 24: {
durationInMs_ = input.readInt64();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stacktrace_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
msg_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Test_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Test_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Test.class, org.sonar.scanner.protocol.output.ScannerReport.Test.Builder.class);
}
/**
* Protobuf enum {@code Test.TestStatus}
*/
public enum TestStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNSET = 0;
*/
UNSET(0, 0),
/**
* OK = 1;
*/
OK(1, 1),
/**
* FAILURE = 2;
*/
FAILURE(2, 2),
/**
* ERROR = 3;
*/
ERROR(3, 3),
/**
* SKIPPED = 4;
*/
SKIPPED(4, 4),
UNRECOGNIZED(-1, -1),
;
/**
* UNSET = 0;
*/
public static final int UNSET_VALUE = 0;
/**
* OK = 1;
*/
public static final int OK_VALUE = 1;
/**
* FAILURE = 2;
*/
public static final int FAILURE_VALUE = 2;
/**
* ERROR = 3;
*/
public static final int ERROR_VALUE = 3;
/**
* SKIPPED = 4;
*/
public static final int SKIPPED_VALUE = 4;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
public static TestStatus valueOf(int value) {
switch (value) {
case 0: return UNSET;
case 1: return OK;
case 2: return FAILURE;
case 3: return ERROR;
case 4: return SKIPPED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TestStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TestStatus findValueByNumber(int number) {
return TestStatus.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.Test.getDescriptor().getEnumTypes().get(0);
}
private static final TestStatus[] VALUES = values();
public static TestStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private TestStatus(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:Test.TestStatus)
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_;
/**
* optional .Test.TestStatus status = 2;
*/
public int getStatusValue() {
return status_;
}
/**
* optional .Test.TestStatus status = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus getStatus() {
org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus result = org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.valueOf(status_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.UNRECOGNIZED : result;
}
public static final int DURATION_IN_MS_FIELD_NUMBER = 3;
private long durationInMs_;
/**
* optional int64 duration_in_ms = 3;
*/
public long getDurationInMs() {
return durationInMs_;
}
public static final int STACKTRACE_FIELD_NUMBER = 4;
private volatile java.lang.Object stacktrace_;
/**
* optional string stacktrace = 4;
*/
public java.lang.String getStacktrace() {
java.lang.Object ref = stacktrace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stacktrace_ = s;
return s;
}
}
/**
* optional string stacktrace = 4;
*/
public com.google.protobuf.ByteString
getStacktraceBytes() {
java.lang.Object ref = stacktrace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stacktrace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MSG_FIELD_NUMBER = 5;
private volatile java.lang.Object msg_;
/**
* optional string msg = 5;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
}
}
/**
* optional string msg = 5;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, name_);
}
if (status_ != org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.UNSET.getNumber()) {
output.writeEnum(2, status_);
}
if (durationInMs_ != 0L) {
output.writeInt64(3, durationInMs_);
}
if (!getStacktraceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, stacktrace_);
}
if (!getMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, msg_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, name_);
}
if (status_ != org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (durationInMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, durationInMs_);
}
if (!getStacktraceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, stacktrace_);
}
if (!getMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, msg_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.Test prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Test}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:Test)
org.sonar.scanner.protocol.output.ScannerReport.TestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Test_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Test_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.Test.class, org.sonar.scanner.protocol.output.ScannerReport.Test.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.Test.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
status_ = 0;
durationInMs_ = 0L;
stacktrace_ = "";
msg_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_Test_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.Test getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.Test.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.Test build() {
org.sonar.scanner.protocol.output.ScannerReport.Test result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.Test buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.Test result = new org.sonar.scanner.protocol.output.ScannerReport.Test(this);
result.name_ = name_;
result.status_ = status_;
result.durationInMs_ = durationInMs_;
result.stacktrace_ = stacktrace_;
result.msg_ = msg_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.Test) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.Test)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.Test other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.Test.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getDurationInMs() != 0L) {
setDurationInMs(other.getDurationInMs());
}
if (!other.getStacktrace().isEmpty()) {
stacktrace_ = other.stacktrace_;
onChanged();
}
if (!other.getMsg().isEmpty()) {
msg_ = other.msg_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.Test parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.Test) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
* optional .Test.TestStatus status = 2;
*/
public int getStatusValue() {
return status_;
}
/**
* optional .Test.TestStatus status = 2;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
* optional .Test.TestStatus status = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus getStatus() {
org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus result = org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.valueOf(status_);
return result == null ? org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus.UNRECOGNIZED : result;
}
/**
* optional .Test.TestStatus status = 2;
*/
public Builder setStatus(org.sonar.scanner.protocol.output.ScannerReport.Test.TestStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .Test.TestStatus status = 2;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private long durationInMs_ ;
/**
* optional int64 duration_in_ms = 3;
*/
public long getDurationInMs() {
return durationInMs_;
}
/**
* optional int64 duration_in_ms = 3;
*/
public Builder setDurationInMs(long value) {
durationInMs_ = value;
onChanged();
return this;
}
/**
* optional int64 duration_in_ms = 3;
*/
public Builder clearDurationInMs() {
durationInMs_ = 0L;
onChanged();
return this;
}
private java.lang.Object stacktrace_ = "";
/**
* optional string stacktrace = 4;
*/
public java.lang.String getStacktrace() {
java.lang.Object ref = stacktrace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stacktrace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string stacktrace = 4;
*/
public com.google.protobuf.ByteString
getStacktraceBytes() {
java.lang.Object ref = stacktrace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stacktrace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string stacktrace = 4;
*/
public Builder setStacktrace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stacktrace_ = value;
onChanged();
return this;
}
/**
* optional string stacktrace = 4;
*/
public Builder clearStacktrace() {
stacktrace_ = getDefaultInstance().getStacktrace();
onChanged();
return this;
}
/**
* optional string stacktrace = 4;
*/
public Builder setStacktraceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stacktrace_ = value;
onChanged();
return this;
}
private java.lang.Object msg_ = "";
/**
* optional string msg = 5;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
msg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msg = 5;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msg = 5;
*/
public Builder setMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
msg_ = value;
onChanged();
return this;
}
/**
* optional string msg = 5;
*/
public Builder clearMsg() {
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
/**
* optional string msg = 5;
*/
public Builder setMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
msg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:Test)
}
// @@protoc_insertion_point(class_scope:Test)
private static final org.sonar.scanner.protocol.output.ScannerReport.Test DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.Test();
}
public static org.sonar.scanner.protocol.output.ScannerReport.Test getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Test parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Test(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.Test getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CoverageDetailOrBuilder extends
// @@protoc_insertion_point(interface_extends:CoverageDetail)
com.google.protobuf.MessageOrBuilder {
/**
* optional string test_name = 1;
*/
java.lang.String getTestName();
/**
* optional string test_name = 1;
*/
com.google.protobuf.ByteString
getTestNameBytes();
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
java.util.List
getCoveredFileList();
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getCoveredFile(int index);
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
int getCoveredFileCount();
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder>
getCoveredFileOrBuilderList();
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder getCoveredFileOrBuilder(
int index);
}
/**
* Protobuf type {@code CoverageDetail}
*/
public static final class CoverageDetail extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:CoverageDetail)
CoverageDetailOrBuilder {
// Use CoverageDetail.newBuilder() to construct.
private CoverageDetail(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CoverageDetail() {
testName_ = "";
coveredFile_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CoverageDetail(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
testName_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
coveredFile_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
coveredFile_.add(input.readMessage(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
coveredFile_ = java.util.Collections.unmodifiableList(coveredFile_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.class, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.Builder.class);
}
public interface CoveredFileOrBuilder extends
// @@protoc_insertion_point(interface_extends:CoverageDetail.CoveredFile)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 file_ref = 1;
*/
int getFileRef();
/**
* repeated int32 covered_line = 2 [packed = true];
*/
java.util.List getCoveredLineList();
/**
* repeated int32 covered_line = 2 [packed = true];
*/
int getCoveredLineCount();
/**
* repeated int32 covered_line = 2 [packed = true];
*/
int getCoveredLine(int index);
}
/**
* Protobuf type {@code CoverageDetail.CoveredFile}
*/
public static final class CoveredFile extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:CoverageDetail.CoveredFile)
CoveredFileOrBuilder {
// Use CoveredFile.newBuilder() to construct.
private CoveredFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CoveredFile() {
fileRef_ = 0;
coveredLine_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CoveredFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
fileRef_ = input.readInt32();
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
coveredLine_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
coveredLine_.add(input.readInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
coveredLine_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
coveredLine_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
coveredLine_ = java.util.Collections.unmodifiableList(coveredLine_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_CoveredFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_CoveredFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.class, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder.class);
}
private int bitField0_;
public static final int FILE_REF_FIELD_NUMBER = 1;
private int fileRef_;
/**
* optional int32 file_ref = 1;
*/
public int getFileRef() {
return fileRef_;
}
public static final int COVERED_LINE_FIELD_NUMBER = 2;
private java.util.List coveredLine_;
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public java.util.List
getCoveredLineList() {
return coveredLine_;
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public int getCoveredLineCount() {
return coveredLine_.size();
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public int getCoveredLine(int index) {
return coveredLine_.get(index);
}
private int coveredLineMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (fileRef_ != 0) {
output.writeInt32(1, fileRef_);
}
if (getCoveredLineList().size() > 0) {
output.writeRawVarint32(18);
output.writeRawVarint32(coveredLineMemoizedSerializedSize);
}
for (int i = 0; i < coveredLine_.size(); i++) {
output.writeInt32NoTag(coveredLine_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (fileRef_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, fileRef_);
}
{
int dataSize = 0;
for (int i = 0; i < coveredLine_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(coveredLine_.get(i));
}
size += dataSize;
if (!getCoveredLineList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
coveredLineMemoizedSerializedSize = dataSize;
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CoverageDetail.CoveredFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:CoverageDetail.CoveredFile)
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_CoveredFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_CoveredFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.class, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
fileRef_ = 0;
coveredLine_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_CoveredFile_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile build() {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile result = new org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.fileRef_ = fileRef_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
coveredLine_ = java.util.Collections.unmodifiableList(coveredLine_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.coveredLine_ = coveredLine_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.getDefaultInstance()) return this;
if (other.getFileRef() != 0) {
setFileRef(other.getFileRef());
}
if (!other.coveredLine_.isEmpty()) {
if (coveredLine_.isEmpty()) {
coveredLine_ = other.coveredLine_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCoveredLineIsMutable();
coveredLine_.addAll(other.coveredLine_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int fileRef_ ;
/**
* optional int32 file_ref = 1;
*/
public int getFileRef() {
return fileRef_;
}
/**
* optional int32 file_ref = 1;
*/
public Builder setFileRef(int value) {
fileRef_ = value;
onChanged();
return this;
}
/**
* optional int32 file_ref = 1;
*/
public Builder clearFileRef() {
fileRef_ = 0;
onChanged();
return this;
}
private java.util.List coveredLine_ = java.util.Collections.emptyList();
private void ensureCoveredLineIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
coveredLine_ = new java.util.ArrayList(coveredLine_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public java.util.List
getCoveredLineList() {
return java.util.Collections.unmodifiableList(coveredLine_);
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public int getCoveredLineCount() {
return coveredLine_.size();
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public int getCoveredLine(int index) {
return coveredLine_.get(index);
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public Builder setCoveredLine(
int index, int value) {
ensureCoveredLineIsMutable();
coveredLine_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public Builder addCoveredLine(int value) {
ensureCoveredLineIsMutable();
coveredLine_.add(value);
onChanged();
return this;
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public Builder addAllCoveredLine(
java.lang.Iterable extends java.lang.Integer> values) {
ensureCoveredLineIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, coveredLine_);
onChanged();
return this;
}
/**
* repeated int32 covered_line = 2 [packed = true];
*/
public Builder clearCoveredLine() {
coveredLine_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:CoverageDetail.CoveredFile)
}
// @@protoc_insertion_point(class_scope:CoverageDetail.CoveredFile)
private static final org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile();
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CoveredFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new CoveredFile(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int TEST_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object testName_;
/**
* optional string test_name = 1;
*/
public java.lang.String getTestName() {
java.lang.Object ref = testName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
testName_ = s;
return s;
}
}
/**
* optional string test_name = 1;
*/
public com.google.protobuf.ByteString
getTestNameBytes() {
java.lang.Object ref = testName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
testName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COVERED_FILE_FIELD_NUMBER = 2;
private java.util.List coveredFile_;
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public java.util.List getCoveredFileList() {
return coveredFile_;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder>
getCoveredFileOrBuilderList() {
return coveredFile_;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public int getCoveredFileCount() {
return coveredFile_.size();
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getCoveredFile(int index) {
return coveredFile_.get(index);
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder getCoveredFileOrBuilder(
int index) {
return coveredFile_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTestNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, testName_);
}
for (int i = 0; i < coveredFile_.size(); i++) {
output.writeMessage(2, coveredFile_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTestNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, testName_);
}
for (int i = 0; i < coveredFile_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, coveredFile_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CoverageDetail}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:CoverageDetail)
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetailOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.class, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.Builder.class);
}
// Construct using org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getCoveredFileFieldBuilder();
}
}
public Builder clear() {
super.clear();
testName_ = "";
if (coveredFileBuilder_ == null) {
coveredFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
coveredFileBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonar.scanner.protocol.output.ScannerReport.internal_static_CoverageDetail_descriptor;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail getDefaultInstanceForType() {
return org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.getDefaultInstance();
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail build() {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail buildPartial() {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail result = new org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.testName_ = testName_;
if (coveredFileBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
coveredFile_ = java.util.Collections.unmodifiableList(coveredFile_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.coveredFile_ = coveredFile_;
} else {
result.coveredFile_ = coveredFileBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail) {
return mergeFrom((org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail other) {
if (other == org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.getDefaultInstance()) return this;
if (!other.getTestName().isEmpty()) {
testName_ = other.testName_;
onChanged();
}
if (coveredFileBuilder_ == null) {
if (!other.coveredFile_.isEmpty()) {
if (coveredFile_.isEmpty()) {
coveredFile_ = other.coveredFile_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCoveredFileIsMutable();
coveredFile_.addAll(other.coveredFile_);
}
onChanged();
}
} else {
if (!other.coveredFile_.isEmpty()) {
if (coveredFileBuilder_.isEmpty()) {
coveredFileBuilder_.dispose();
coveredFileBuilder_ = null;
coveredFile_ = other.coveredFile_;
bitField0_ = (bitField0_ & ~0x00000002);
coveredFileBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getCoveredFileFieldBuilder() : null;
} else {
coveredFileBuilder_.addAllMessages(other.coveredFile_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object testName_ = "";
/**
* optional string test_name = 1;
*/
public java.lang.String getTestName() {
java.lang.Object ref = testName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
testName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string test_name = 1;
*/
public com.google.protobuf.ByteString
getTestNameBytes() {
java.lang.Object ref = testName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
testName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string test_name = 1;
*/
public Builder setTestName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
testName_ = value;
onChanged();
return this;
}
/**
* optional string test_name = 1;
*/
public Builder clearTestName() {
testName_ = getDefaultInstance().getTestName();
onChanged();
return this;
}
/**
* optional string test_name = 1;
*/
public Builder setTestNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
testName_ = value;
onChanged();
return this;
}
private java.util.List coveredFile_ =
java.util.Collections.emptyList();
private void ensureCoveredFileIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
coveredFile_ = new java.util.ArrayList(coveredFile_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder> coveredFileBuilder_;
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public java.util.List getCoveredFileList() {
if (coveredFileBuilder_ == null) {
return java.util.Collections.unmodifiableList(coveredFile_);
} else {
return coveredFileBuilder_.getMessageList();
}
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public int getCoveredFileCount() {
if (coveredFileBuilder_ == null) {
return coveredFile_.size();
} else {
return coveredFileBuilder_.getCount();
}
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile getCoveredFile(int index) {
if (coveredFileBuilder_ == null) {
return coveredFile_.get(index);
} else {
return coveredFileBuilder_.getMessage(index);
}
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder setCoveredFile(
int index, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile value) {
if (coveredFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCoveredFileIsMutable();
coveredFile_.set(index, value);
onChanged();
} else {
coveredFileBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder setCoveredFile(
int index, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder builderForValue) {
if (coveredFileBuilder_ == null) {
ensureCoveredFileIsMutable();
coveredFile_.set(index, builderForValue.build());
onChanged();
} else {
coveredFileBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder addCoveredFile(org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile value) {
if (coveredFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCoveredFileIsMutable();
coveredFile_.add(value);
onChanged();
} else {
coveredFileBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder addCoveredFile(
int index, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile value) {
if (coveredFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCoveredFileIsMutable();
coveredFile_.add(index, value);
onChanged();
} else {
coveredFileBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder addCoveredFile(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder builderForValue) {
if (coveredFileBuilder_ == null) {
ensureCoveredFileIsMutable();
coveredFile_.add(builderForValue.build());
onChanged();
} else {
coveredFileBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder addCoveredFile(
int index, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder builderForValue) {
if (coveredFileBuilder_ == null) {
ensureCoveredFileIsMutable();
coveredFile_.add(index, builderForValue.build());
onChanged();
} else {
coveredFileBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder addAllCoveredFile(
java.lang.Iterable extends org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile> values) {
if (coveredFileBuilder_ == null) {
ensureCoveredFileIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, coveredFile_);
onChanged();
} else {
coveredFileBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder clearCoveredFile() {
if (coveredFileBuilder_ == null) {
coveredFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
coveredFileBuilder_.clear();
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public Builder removeCoveredFile(int index) {
if (coveredFileBuilder_ == null) {
ensureCoveredFileIsMutable();
coveredFile_.remove(index);
onChanged();
} else {
coveredFileBuilder_.remove(index);
}
return this;
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder getCoveredFileBuilder(
int index) {
return getCoveredFileFieldBuilder().getBuilder(index);
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder getCoveredFileOrBuilder(
int index) {
if (coveredFileBuilder_ == null) {
return coveredFile_.get(index); } else {
return coveredFileBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public java.util.List extends org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder>
getCoveredFileOrBuilderList() {
if (coveredFileBuilder_ != null) {
return coveredFileBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(coveredFile_);
}
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder addCoveredFileBuilder() {
return getCoveredFileFieldBuilder().addBuilder(
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.getDefaultInstance());
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder addCoveredFileBuilder(
int index) {
return getCoveredFileFieldBuilder().addBuilder(
index, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.getDefaultInstance());
}
/**
* repeated .CoverageDetail.CoveredFile covered_file = 2;
*/
public java.util.List
getCoveredFileBuilderList() {
return getCoveredFileFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder>
getCoveredFileFieldBuilder() {
if (coveredFileBuilder_ == null) {
coveredFileBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFile.Builder, org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail.CoveredFileOrBuilder>(
coveredFile_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
coveredFile_ = null;
}
return coveredFileBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:CoverageDetail)
}
// @@protoc_insertion_point(class_scope:CoverageDetail)
private static final org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail();
}
public static org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CoverageDetail parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new CoverageDetail(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonar.scanner.protocol.output.ScannerReport.CoverageDetail getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Metadata_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Metadata_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Metadata_QprofilesPerLanguageEntry_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Metadata_QprofilesPerLanguageEntry_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Metadata_QProfile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Metadata_QProfile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ActiveRule_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ActiveRule_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ActiveRule_ParamsByKeyEntry_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ActiveRule_ParamsByKeyEntry_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ComponentLink_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ComponentLink_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Component_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Component_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_BoolValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_BoolValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_IntValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_IntValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_LongValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_LongValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_DoubleValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_DoubleValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Measure_StringValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Measure_StringValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Issue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Issue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_IssueLocation_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_IssueLocation_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Flow_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Flow_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Changesets_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Changesets_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Changesets_Changeset_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Changesets_Changeset_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Duplicate_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Duplicate_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Duplication_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Duplication_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_CpdTextBlock_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_CpdTextBlock_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_TextRange_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TextRange_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Symbol_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Symbol_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_LineCoverage_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_LineCoverage_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_SyntaxHighlightingRule_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_SyntaxHighlightingRule_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Test_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Test_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_CoverageDetail_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_CoverageDetail_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_CoverageDetail_CoveredFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_CoverageDetail_CoveredFile_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024scanner_report.proto\032\017constants.proto\"" +
"\366\002\n\010Metadata\022\025\n\ranalysis_date\030\001 \001(\003\022\023\n\013p" +
"roject_key\030\002 \001(\t\022\016\n\006branch\030\003 \001(\t\022\032\n\022root" +
"_component_ref\030\004 \001(\005\022+\n#cross_project_du" +
"plication_activated\030\005 \001(\010\022C\n\026qprofiles_p" +
"er_language\030\006 \003(\0132#.Metadata.QprofilesPe" +
"rLanguageEntry\032O\n\031QprofilesPerLanguageEn" +
"try\022\013\n\003key\030\001 \001(\t\022!\n\005value\030\002 \001(\0132\022.Metada" +
"ta.QProfile:\0028\001\032O\n\010QProfile\022\013\n\003key\030\001 \001(\t" +
"\022\014\n\004name\030\002 \001(\t\022\020\n\010language\030\003 \001(\t\022\026\n\016rule",
"sUpdatedAt\030\004 \001(\003\"\275\001\n\nActiveRule\022\027\n\017rule_" +
"repository\030\001 \001(\t\022\020\n\010rule_key\030\002 \001(\t\022\033\n\010se" +
"verity\030\003 \001(\0162\t.Severity\0223\n\rparams_by_key" +
"\030\004 \003(\0132\034.ActiveRule.ParamsByKeyEntry\0322\n\020" +
"ParamsByKeyEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002" +
" \001(\t:\0028\001\"\240\001\n\rComponentLink\022.\n\004type\030\001 \001(\016" +
"2 .ComponentLink.ComponentLinkType\022\014\n\004hr" +
"ef\030\002 \001(\t\"Q\n\021ComponentLinkType\022\t\n\005UNSET\020\000" +
"\022\010\n\004HOME\020\001\022\007\n\003SCM\020\002\022\013\n\007SCM_DEV\020\003\022\t\n\005ISSU" +
"E\020\004\022\006\n\002CI\020\005\"\304\002\n\tComponent\022\013\n\003ref\030\001 \001(\005\022\014",
"\n\004path\030\002 \001(\t\022\014\n\004name\030\003 \001(\t\022&\n\004type\030\004 \001(\016" +
"2\030.Component.ComponentType\022\017\n\007is_test\030\005 " +
"\001(\010\022\020\n\010language\030\006 \001(\t\022\025\n\tchild_ref\030\007 \003(\005" +
"B\002\020\001\022\034\n\004link\030\010 \003(\0132\016.ComponentLink\022\017\n\007ve" +
"rsion\030\t \001(\t\022\013\n\003key\030\n \001(\t\022\r\n\005lines\030\013 \001(\005\022" +
"\023\n\013description\030\014 \001(\t\"L\n\rComponentType\022\t\n" +
"\005UNSET\020\000\022\013\n\007PROJECT\020\001\022\n\n\006MODULE\020\002\022\r\n\tDIR" +
"ECTORY\020\003\022\010\n\004FILE\020\004\"\310\003\n\007Measure\022\022\n\nmetric" +
"_key\030\001 \001(\t\022+\n\rboolean_value\030\002 \001(\0132\022.Meas" +
"ure.BoolValueH\000\022&\n\tint_value\030\003 \001(\0132\021.Mea",
"sure.IntValueH\000\022(\n\nlong_value\030\004 \001(\0132\022.Me" +
"asure.LongValueH\000\022,\n\014double_value\030\005 \001(\0132" +
"\024.Measure.DoubleValueH\000\022,\n\014string_value\030" +
"\006 \001(\0132\024.Measure.StringValueH\000\032(\n\tBoolVal" +
"ue\022\r\n\005value\030\001 \001(\010\022\014\n\004data\030\002 \001(\t\032\'\n\010IntVa" +
"lue\022\r\n\005value\030\001 \001(\005\022\014\n\004data\030\002 \001(\t\032(\n\tLong" +
"Value\022\r\n\005value\030\001 \001(\003\022\014\n\004data\030\002 \001(\t\032*\n\013Do" +
"ubleValue\022\r\n\005value\030\001 \001(\001\022\014\n\004data\030\002 \001(\t\032\034" +
"\n\013StringValue\022\r\n\005value\030\001 \001(\tB\007\n\005value\"\236\001" +
"\n\005Issue\022\027\n\017rule_repository\030\001 \001(\t\022\020\n\010rule",
"_key\030\002 \001(\t\022\013\n\003msg\030\003 \001(\t\022\033\n\010severity\030\004 \001(" +
"\0162\t.Severity\022\013\n\003gap\030\005 \001(\001\022\036\n\ntext_range\030" +
"\006 \001(\0132\n.TextRange\022\023\n\004flow\030\007 \003(\0132\005.Flow\"S" +
"\n\rIssueLocation\022\025\n\rcomponent_ref\030\001 \001(\005\022\036" +
"\n\ntext_range\030\002 \001(\0132\n.TextRange\022\013\n\003msg\030\003 " +
"\001(\t\"(\n\004Flow\022 \n\010location\030\001 \003(\0132\016.IssueLoc" +
"ation\"\310\001\n\nChangesets\022\025\n\rcomponent_ref\030\001 " +
"\001(\005\022\032\n\022copy_from_previous\030\002 \001(\010\022(\n\tchang" +
"eset\030\003 \003(\0132\025.Changesets.Changeset\022 \n\024cha" +
"ngesetIndexByLine\030\004 \003(\005B\002\020\001\032;\n\tChangeset",
"\022\020\n\010revision\030\001 \001(\t\022\016\n\006author\030\002 \001(\t\022\014\n\004da" +
"te\030\003 \001(\003\">\n\tDuplicate\022\026\n\016other_file_ref\030" +
"\001 \001(\005\022\031\n\005range\030\002 \001(\0132\n.TextRange\"Q\n\013Dupl" +
"ication\022#\n\017origin_position\030\001 \001(\0132\n.TextR" +
"ange\022\035\n\tduplicate\030\002 \003(\0132\n.Duplicate\"v\n\014C" +
"pdTextBlock\022\014\n\004hash\030\001 \001(\t\022\022\n\nstart_line\030" +
"\002 \001(\005\022\020\n\010end_line\030\003 \001(\005\022\031\n\021start_token_i" +
"ndex\030\004 \001(\005\022\027\n\017end_token_index\030\005 \001(\005\"[\n\tT" +
"extRange\022\022\n\nstart_line\030\001 \001(\005\022\020\n\010end_line" +
"\030\002 \001(\005\022\024\n\014start_offset\030\003 \001(\005\022\022\n\nend_offs",
"et\030\004 \001(\005\"H\n\006Symbol\022\037\n\013declaration\030\001 \001(\0132" +
"\n.TextRange\022\035\n\treference\030\002 \003(\0132\n.TextRan" +
"ge\"\270\002\n\014LineCoverage\022\014\n\004line\030\001 \001(\005\022\022\n\ncon" +
"ditions\030\002 \001(\005\022\021\n\007ut_hits\030\003 \001(\010H\000\022\021\n\007it_h" +
"its\030\004 \001(\010H\001\022\037\n\025ut_covered_conditions\030\005 \001" +
"(\005H\002\022\037\n\025it_covered_conditions\030\006 \001(\005H\003\022$\n" +
"\032overall_covered_conditions\030\007 \001(\005H\004B\r\n\013h" +
"as_ut_hitsB\r\n\013has_it_hitsB\033\n\031has_ut_cove" +
"red_conditionsB\033\n\031has_it_covered_conditi" +
"onsB \n\036has_overall_covered_conditions\"\256\002",
"\n\026SyntaxHighlightingRule\022\031\n\005range\030\001 \001(\0132" +
"\n.TextRange\0226\n\004type\030\002 \001(\0162(.SyntaxHighli" +
"ghtingRule.HighlightingType\"\300\001\n\020Highligh" +
"tingType\022\t\n\005UNSET\020\000\022\016\n\nANNOTATION\020\001\022\014\n\010C" +
"ONSTANT\020\002\022\013\n\007COMMENT\020\003\022\013\n\007CPP_DOC\020\004\022\026\n\022S" +
"TRUCTURED_COMMENT\020\005\022\013\n\007KEYWORD\020\006\022\027\n\023HIGH" +
"LIGHTING_STRING\020\007\022\021\n\rKEYWORD_LIGHT\020\010\022\030\n\024" +
"PREPROCESS_DIRECTIVE\020\t\"\265\001\n\004Test\022\014\n\004name\030" +
"\001 \001(\t\022 \n\006status\030\002 \001(\0162\020.Test.TestStatus\022" +
"\026\n\016duration_in_ms\030\003 \001(\003\022\022\n\nstacktrace\030\004 ",
"\001(\t\022\013\n\003msg\030\005 \001(\t\"D\n\nTestStatus\022\t\n\005UNSET\020" +
"\000\022\006\n\002OK\020\001\022\013\n\007FAILURE\020\002\022\t\n\005ERROR\020\003\022\013\n\007SKI" +
"PPED\020\004\"\221\001\n\016CoverageDetail\022\021\n\ttest_name\030\001" +
" \001(\t\0221\n\014covered_file\030\002 \003(\0132\033.CoverageDet" +
"ail.CoveredFile\0329\n\013CoveredFile\022\020\n\010file_r" +
"ef\030\001 \001(\005\022\030\n\014covered_line\030\002 \003(\005B\002\020\001B%\n!or" +
"g.sonar.scanner.protocol.outputH\001b\006proto" +
"3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.sonar.scanner.protocol.Constants.getDescriptor(),
}, assigner);
internal_static_Metadata_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Metadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Metadata_descriptor,
new java.lang.String[] { "AnalysisDate", "ProjectKey", "Branch", "RootComponentRef", "CrossProjectDuplicationActivated", "QprofilesPerLanguage", });
internal_static_Metadata_QprofilesPerLanguageEntry_descriptor =
internal_static_Metadata_descriptor.getNestedTypes().get(0);
internal_static_Metadata_QprofilesPerLanguageEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Metadata_QprofilesPerLanguageEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_Metadata_QProfile_descriptor =
internal_static_Metadata_descriptor.getNestedTypes().get(1);
internal_static_Metadata_QProfile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Metadata_QProfile_descriptor,
new java.lang.String[] { "Key", "Name", "Language", "RulesUpdatedAt", });
internal_static_ActiveRule_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ActiveRule_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ActiveRule_descriptor,
new java.lang.String[] { "RuleRepository", "RuleKey", "Severity", "ParamsByKey", });
internal_static_ActiveRule_ParamsByKeyEntry_descriptor =
internal_static_ActiveRule_descriptor.getNestedTypes().get(0);
internal_static_ActiveRule_ParamsByKeyEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ActiveRule_ParamsByKeyEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_ComponentLink_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ComponentLink_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ComponentLink_descriptor,
new java.lang.String[] { "Type", "Href", });
internal_static_Component_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_Component_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Component_descriptor,
new java.lang.String[] { "Ref", "Path", "Name", "Type", "IsTest", "Language", "ChildRef", "Link", "Version", "Key", "Lines", "Description", });
internal_static_Measure_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_Measure_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_descriptor,
new java.lang.String[] { "MetricKey", "BooleanValue", "IntValue", "LongValue", "DoubleValue", "StringValue", "Value", });
internal_static_Measure_BoolValue_descriptor =
internal_static_Measure_descriptor.getNestedTypes().get(0);
internal_static_Measure_BoolValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_BoolValue_descriptor,
new java.lang.String[] { "Value", "Data", });
internal_static_Measure_IntValue_descriptor =
internal_static_Measure_descriptor.getNestedTypes().get(1);
internal_static_Measure_IntValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_IntValue_descriptor,
new java.lang.String[] { "Value", "Data", });
internal_static_Measure_LongValue_descriptor =
internal_static_Measure_descriptor.getNestedTypes().get(2);
internal_static_Measure_LongValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_LongValue_descriptor,
new java.lang.String[] { "Value", "Data", });
internal_static_Measure_DoubleValue_descriptor =
internal_static_Measure_descriptor.getNestedTypes().get(3);
internal_static_Measure_DoubleValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_DoubleValue_descriptor,
new java.lang.String[] { "Value", "Data", });
internal_static_Measure_StringValue_descriptor =
internal_static_Measure_descriptor.getNestedTypes().get(4);
internal_static_Measure_StringValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Measure_StringValue_descriptor,
new java.lang.String[] { "Value", });
internal_static_Issue_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_Issue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Issue_descriptor,
new java.lang.String[] { "RuleRepository", "RuleKey", "Msg", "Severity", "Gap", "TextRange", "Flow", });
internal_static_IssueLocation_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_IssueLocation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_IssueLocation_descriptor,
new java.lang.String[] { "ComponentRef", "TextRange", "Msg", });
internal_static_Flow_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_Flow_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Flow_descriptor,
new java.lang.String[] { "Location", });
internal_static_Changesets_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_Changesets_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Changesets_descriptor,
new java.lang.String[] { "ComponentRef", "CopyFromPrevious", "Changeset", "ChangesetIndexByLine", });
internal_static_Changesets_Changeset_descriptor =
internal_static_Changesets_descriptor.getNestedTypes().get(0);
internal_static_Changesets_Changeset_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Changesets_Changeset_descriptor,
new java.lang.String[] { "Revision", "Author", "Date", });
internal_static_Duplicate_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_Duplicate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Duplicate_descriptor,
new java.lang.String[] { "OtherFileRef", "Range", });
internal_static_Duplication_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_Duplication_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Duplication_descriptor,
new java.lang.String[] { "OriginPosition", "Duplicate", });
internal_static_CpdTextBlock_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_CpdTextBlock_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_CpdTextBlock_descriptor,
new java.lang.String[] { "Hash", "StartLine", "EndLine", "StartTokenIndex", "EndTokenIndex", });
internal_static_TextRange_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_TextRange_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TextRange_descriptor,
new java.lang.String[] { "StartLine", "EndLine", "StartOffset", "EndOffset", });
internal_static_Symbol_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_Symbol_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Symbol_descriptor,
new java.lang.String[] { "Declaration", "Reference", });
internal_static_LineCoverage_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_LineCoverage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_LineCoverage_descriptor,
new java.lang.String[] { "Line", "Conditions", "UtHits", "ItHits", "UtCoveredConditions", "ItCoveredConditions", "OverallCoveredConditions", "HasUtHits", "HasItHits", "HasUtCoveredConditions", "HasItCoveredConditions", "HasOverallCoveredConditions", });
internal_static_SyntaxHighlightingRule_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_SyntaxHighlightingRule_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_SyntaxHighlightingRule_descriptor,
new java.lang.String[] { "Range", "Type", });
internal_static_Test_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_Test_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Test_descriptor,
new java.lang.String[] { "Name", "Status", "DurationInMs", "Stacktrace", "Msg", });
internal_static_CoverageDetail_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_CoverageDetail_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_CoverageDetail_descriptor,
new java.lang.String[] { "TestName", "CoveredFile", });
internal_static_CoverageDetail_CoveredFile_descriptor =
internal_static_CoverageDetail_descriptor.getNestedTypes().get(0);
internal_static_CoverageDetail_CoveredFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_CoverageDetail_CoveredFile_descriptor,
new java.lang.String[] { "FileRef", "CoveredLine", });
org.sonar.scanner.protocol.Constants.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy