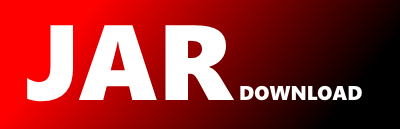
org.sonar.server.component.ws.ShowData Maven / Gradle / Ivy
/*
* SonarQube
* Copyright (C) 2009-2016 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.server.component.ws;
import com.google.common.base.Function;
import com.google.common.base.Splitter;
import com.google.common.collect.Lists;
import com.google.common.collect.Ordering;
import java.util.Collections;
import java.util.List;
import javax.annotation.Nonnull;
import org.sonar.db.component.ComponentDto;
import org.sonar.db.component.ComponentDtoFunctions;
import org.sonar.db.component.SnapshotDto;
import org.sonar.db.component.SnapshotDtoFunctions;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Preconditions.checkState;
class ShowData {
private final List components;
private ShowData(List components) {
this.components = components;
}
static Builder builder(SnapshotDto snapshot) {
return new Builder(snapshot);
}
List getComponents() {
return components;
}
static class Builder {
private Ordering snapshotOrdering;
private List orderedSnapshotIds;
private List orderedComponentIds;
private Builder(SnapshotDto snapshot) {
List orderedSnapshotIdsAsString = snapshot.getPath() == null ? Collections.emptyList() : Splitter.on(".").omitEmptyStrings().splitToList(snapshot.getPath());
orderedSnapshotIds = Lists.transform(orderedSnapshotIdsAsString, StringToLongFunction.INSTANCE);
snapshotOrdering = Ordering
.explicit(orderedSnapshotIds)
.onResultOf(SnapshotDtoFunctions.toId())
.reverse();
}
Builder withAncestorsSnapshots(List ancestorsSnapshots) {
checkNotNull(snapshotOrdering, "Snapshot must be set before the ancestors");
checkState(orderedSnapshotIds.size() == ancestorsSnapshots.size(), "Missing ancestor");
orderedComponentIds = Lists.transform(
snapshotOrdering.immutableSortedCopy(ancestorsSnapshots),
SnapshotDtoFunctions.toComponentId());
return this;
}
ShowData andAncestorComponents(List ancestorComponents) {
checkNotNull(orderedComponentIds, "Snapshot ancestors must be set before the component ancestors");
checkState(orderedComponentIds.size() == ancestorComponents.size(), "Missing ancestor");
return new ShowData(Ordering
.explicit(orderedComponentIds)
.onResultOf(ComponentDtoFunctions.toId())
.immutableSortedCopy(ancestorComponents));
}
List getOrderedSnapshotIds() {
return orderedSnapshotIds;
}
List getOrderedComponentIds() {
return orderedComponentIds;
}
}
private enum StringToLongFunction implements Function {
INSTANCE;
@Override
public Long apply(@Nonnull String input) {
return Long.parseLong(input);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy