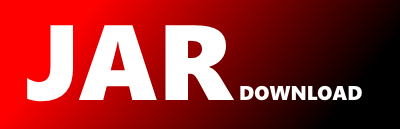
org.sonar.server.qualityprofile.QProfiles Maven / Gradle / Ivy
/*
* SonarQube
* Copyright (C) 2009-2016 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.server.qualityprofile;
import com.google.common.base.Strings;
import java.util.List;
import javax.annotation.CheckForNull;
import org.sonar.api.component.Component;
import org.sonar.api.server.ServerSide;
import org.sonar.server.exceptions.BadRequestException;
import org.sonar.server.user.UserSession;
import org.sonar.server.util.Validation;
/**
* Use {@link org.sonar.server.qualityprofile.QProfileService} instead
*/
@Deprecated
@ServerSide
public class QProfiles {
private static final String LANGUAGE_PARAM = "language";
private final QProfileProjectOperations projectOperations;
private final QProfileProjectLookup projectLookup;
private final QProfileLookup profileLookup;
private final UserSession userSession;
public QProfiles(QProfileProjectOperations projectOperations, QProfileProjectLookup projectLookup,
QProfileLookup profileLookup, UserSession userSession) {
this.projectOperations = projectOperations;
this.projectLookup = projectLookup;
this.profileLookup = profileLookup;
this.userSession = userSession;
}
public List allProfiles() {
return profileLookup.allProfiles();
}
public List profilesByLanguage(String language) {
return profileLookup.profiles(language);
}
@CheckForNull
public QProfile profile(int id) {
return profileLookup.profile(id);
}
@CheckForNull
public QProfile profile(String name, String language) {
checkProfileNameParam(name);
Validation.checkMandatoryParameter(language, LANGUAGE_PARAM);
return profileLookup.profile(name, language);
}
@CheckForNull
public QProfile parent(QProfile profile) {
return profileLookup.parent(profile);
}
public List children(QProfile profile) {
return profileLookup.children(profile);
}
public List ancestors(QProfile profile) {
return profileLookup.ancestors(profile);
}
// PROJECTS
public List projects(int profileId) {
return projectLookup.projects(profileId);
}
public int countProjects(QProfile profile) {
return projectLookup.countProjects(profile);
}
/**
* Used in /project/profile and in /api/profiles
*/
@CheckForNull
public QProfile findProfileByProjectAndLanguage(long projectId, String language) {
return projectLookup.findProfileByProjectAndLanguage(projectId, language);
}
public void addProject(String profileKey, String projectUuid) {
projectOperations.addProject(profileKey, projectUuid, userSession);
}
public void removeProject(String profileKey, String projectUuid) {
projectOperations.removeProject(profileKey, projectUuid, userSession);
}
public void removeProjectByLanguage(String language, long projectId) {
projectOperations.removeProject(language, projectId, userSession);
}
public void removeAllProjects(String profileKey) {
projectOperations.removeAllProjects(profileKey, userSession);
}
private static void checkProfileNameParam(String name) {
if (Strings.isNullOrEmpty(name)) {
throw new BadRequestException("quality_profiles.please_type_profile_name");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy