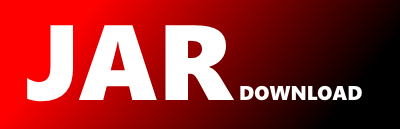
org.sonar.server.ui.JRubyFacade Maven / Gradle / Ivy
/*
* SonarQube
* Copyright (C) 2009-2016 SonarSource SA
* mailto:contact AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.server.ui;
import java.sql.Connection;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import javax.annotation.CheckForNull;
import javax.annotation.Nullable;
import org.sonar.api.Plugin;
import org.sonar.api.config.PropertyDefinitions;
import org.sonar.api.config.Settings;
import org.sonar.api.platform.NewUserHandler;
import org.sonar.api.resources.Language;
import org.sonar.api.resources.ResourceType;
import org.sonar.api.resources.ResourceTypes;
import org.sonar.api.server.authentication.IdentityProvider;
import org.sonar.api.utils.log.Loggers;
import org.sonar.api.web.Footer;
import org.sonar.api.web.Page;
import org.sonar.api.web.RubyRailsWebservice;
import org.sonar.api.web.Widget;
import org.sonar.core.platform.ComponentContainer;
import org.sonar.core.platform.PluginInfo;
import org.sonar.core.platform.PluginRepository;
import org.sonar.core.timemachine.Periods;
import org.sonar.db.Database;
import org.sonar.db.DbClient;
import org.sonar.db.DbSession;
import org.sonar.db.property.PropertiesDao;
import org.sonar.db.property.PropertyDto;
import org.sonar.db.rule.RuleRepositoryDto;
import org.sonar.db.version.DatabaseMigration;
import org.sonar.db.version.DatabaseVersion;
import org.sonar.process.ProcessProperties;
import org.sonar.server.authentication.IdentityProviderRepository;
import org.sonar.server.component.ComponentCleanerService;
import org.sonar.server.measure.MeasureFilterEngine;
import org.sonar.server.measure.MeasureFilterResult;
import org.sonar.server.platform.PersistentSettings;
import org.sonar.server.platform.Platform;
import org.sonar.server.platform.db.migrations.DatabaseMigrator;
import org.sonar.server.platform.ws.UpgradesAction;
import org.sonar.server.user.NewUserNotifier;
import static com.google.common.collect.Lists.newArrayList;
public final class JRubyFacade {
private static final JRubyFacade SINGLETON = new JRubyFacade();
public static JRubyFacade getInstance() {
return SINGLETON;
}
T get(Class componentType) {
return getContainer().getComponentByType(componentType);
}
public MeasureFilterResult executeMeasureFilter(Map map, @Nullable Long userId) {
return get(MeasureFilterEngine.class).execute(map, userId);
}
public Collection getResourceTypesForFilter() {
return get(ResourceTypes.class).getAll(ResourceTypes.AVAILABLE_FOR_FILTERS);
}
public Collection getResourceTypes() {
return get(ResourceTypes.class).getAllOrdered();
}
public Collection getResourceRootTypes() {
return get(ResourceTypes.class).getRoots();
}
public ResourceType getResourceType(String qualifier) {
return get(ResourceTypes.class).get(qualifier);
}
public List getQualifiersWithProperty(final String propertyKey) {
List qualifiers = newArrayList();
for (ResourceType type : getResourceTypes()) {
if (type.getBooleanProperty(propertyKey) == Boolean.TRUE) {
qualifiers.add(type.getQualifier());
}
}
return qualifiers;
}
public Boolean getResourceTypeBooleanProperty(String resourceTypeQualifier, String resourceTypeProperty) {
ResourceType resourceType = getResourceType(resourceTypeQualifier);
if (resourceType != null) {
return resourceType.getBooleanProperty(resourceTypeProperty);
}
return null;
}
public Collection getResourceLeavesQualifiers(String qualifier) {
return get(ResourceTypes.class).getLeavesQualifiers(qualifier);
}
public Collection getResourceChildrenQualifiers(String qualifier) {
return get(ResourceTypes.class).getChildrenQualifiers(qualifier);
}
// PLUGINS ------------------------------------------------------------------
public PropertyDefinitions getPropertyDefinitions() {
return get(PropertyDefinitions.class);
}
/**
* Used for WS api/updatecenter/installed_plugins, to be replaced by api/plugins/installed.
*/
public Collection getPluginInfos() {
return get(PluginRepository.class).getPluginInfos();
}
public List> getWidgets() {
return get(Views.class).getWidgets();
}
public ViewProxy getWidget(String id) {
return get(Views.class).getWidget(id);
}
public ViewProxy getPage(String id) {
return get(Views.class).getPage(id);
}
public Collection getRubyRailsWebservices() {
return getContainer().getComponentsByType(RubyRailsWebservice.class);
}
public Collection getLanguages() {
return getContainer().getComponentsByType(Language.class);
}
public Database getDatabase() {
return get(Database.class);
}
// Only used by Java migration
public DatabaseMigrator databaseMigrator() {
return get(DatabaseMigrator.class);
}
/* PROFILES CONSOLE : RULES AND METRIC THRESHOLDS */
/**
* @deprecated in 4.2
*/
@Deprecated
@CheckForNull
public RuleRepositoryDto getRuleRepository(String repositoryKey) {
DbClient dbClient = get(DbClient.class);
try (DbSession dbSession = dbClient.openSession(false)) {
return dbClient.ruleRepositoryDao().selectByKey(dbSession, repositoryKey).orElse(null);
}
}
public Collection getRuleRepositories() {
DbClient dbClient = get(DbClient.class);
try (DbSession dbSession = dbClient.openSession(false)) {
return dbClient.ruleRepositoryDao().selectAll(dbSession);
}
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy