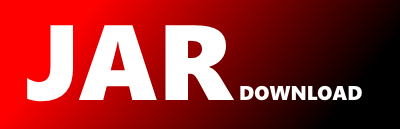
org.sonarqube.ws.Common Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-ws Show documentation
Show all versions of sonar-ws Show documentation
Open source platform for continuous inspection of code quality
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ws-commons.proto
package org.sonarqube.ws;
public final class Common {
private Common() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code sonarqube.ws.commons.Severity}
*/
public enum Severity
implements com.google.protobuf.ProtocolMessageEnum {
/**
* INFO = 0;
*/
INFO(0, 0),
/**
* MINOR = 1;
*/
MINOR(1, 1),
/**
* MAJOR = 2;
*/
MAJOR(2, 2),
/**
* CRITICAL = 3;
*/
CRITICAL(3, 3),
/**
* BLOCKER = 4;
*/
BLOCKER(4, 4),
;
/**
* INFO = 0;
*/
public static final int INFO_VALUE = 0;
/**
* MINOR = 1;
*/
public static final int MINOR_VALUE = 1;
/**
* MAJOR = 2;
*/
public static final int MAJOR_VALUE = 2;
/**
* CRITICAL = 3;
*/
public static final int CRITICAL_VALUE = 3;
/**
* BLOCKER = 4;
*/
public static final int BLOCKER_VALUE = 4;
public final int getNumber() {
return value;
}
public static Severity valueOf(int value) {
switch (value) {
case 0: return INFO;
case 1: return MINOR;
case 2: return MAJOR;
case 3: return CRITICAL;
case 4: return BLOCKER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Severity> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Severity findValueByNumber(int number) {
return Severity.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonarqube.ws.Common.getDescriptor().getEnumTypes().get(0);
}
private static final Severity[] VALUES = values();
public static Severity valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Severity(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sonarqube.ws.commons.Severity)
}
/**
* Protobuf enum {@code sonarqube.ws.commons.RuleStatus}
*/
public enum RuleStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* BETA = 0;
*/
BETA(0, 0),
/**
* DEPRECATED = 1;
*/
DEPRECATED(1, 1),
/**
* READY = 2;
*/
READY(2, 2),
/**
* REMOVED = 3;
*/
REMOVED(3, 3),
;
/**
* BETA = 0;
*/
public static final int BETA_VALUE = 0;
/**
* DEPRECATED = 1;
*/
public static final int DEPRECATED_VALUE = 1;
/**
* READY = 2;
*/
public static final int READY_VALUE = 2;
/**
* REMOVED = 3;
*/
public static final int REMOVED_VALUE = 3;
public final int getNumber() {
return value;
}
public static RuleStatus valueOf(int value) {
switch (value) {
case 0: return BETA;
case 1: return DEPRECATED;
case 2: return READY;
case 3: return REMOVED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RuleStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RuleStatus findValueByNumber(int number) {
return RuleStatus.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonarqube.ws.Common.getDescriptor().getEnumTypes().get(1);
}
private static final RuleStatus[] VALUES = values();
public static RuleStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private RuleStatus(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sonarqube.ws.commons.RuleStatus)
}
/**
* Protobuf enum {@code sonarqube.ws.commons.RuleType}
*/
public enum RuleType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*
*
* Zero is required in order to not get MAINTAINABILITY as default value
* See http://androiddevblog.com/protocol-buffers-pitfall-adding-enum-values/
*
*/
UNKNOWN(0, 0),
/**
* CODE_SMELL = 1;
*
*
* same name as in Java enum IssueType,
* same index values as in database (see column ISSUES.ISSUE_TYPE)
*
*/
CODE_SMELL(1, 1),
/**
* BUG = 2;
*/
BUG(2, 2),
/**
* VULNERABILITY = 3;
*/
VULNERABILITY(3, 3),
;
/**
* UNKNOWN = 0;
*
*
* Zero is required in order to not get MAINTAINABILITY as default value
* See http://androiddevblog.com/protocol-buffers-pitfall-adding-enum-values/
*
*/
public static final int UNKNOWN_VALUE = 0;
/**
* CODE_SMELL = 1;
*
*
* same name as in Java enum IssueType,
* same index values as in database (see column ISSUES.ISSUE_TYPE)
*
*/
public static final int CODE_SMELL_VALUE = 1;
/**
* BUG = 2;
*/
public static final int BUG_VALUE = 2;
/**
* VULNERABILITY = 3;
*/
public static final int VULNERABILITY_VALUE = 3;
public final int getNumber() {
return value;
}
public static RuleType valueOf(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return CODE_SMELL;
case 2: return BUG;
case 3: return VULNERABILITY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RuleType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RuleType findValueByNumber(int number) {
return RuleType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonarqube.ws.Common.getDescriptor().getEnumTypes().get(2);
}
private static final RuleType[] VALUES = values();
public static RuleType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private RuleType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sonarqube.ws.commons.RuleType)
}
public interface PagingOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Paging)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 pageIndex = 1;
*/
boolean hasPageIndex();
/**
* optional int32 pageIndex = 1;
*/
int getPageIndex();
/**
* optional int32 pageSize = 2;
*/
boolean hasPageSize();
/**
* optional int32 pageSize = 2;
*/
int getPageSize();
/**
* optional int32 total = 3;
*/
boolean hasTotal();
/**
* optional int32 total = 3;
*/
int getTotal();
}
/**
* Protobuf type {@code sonarqube.ws.commons.Paging}
*/
public static final class Paging extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Paging)
PagingOrBuilder {
// Use Paging.newBuilder() to construct.
private Paging(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Paging() {
pageIndex_ = 0;
pageSize_ = 0;
total_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Paging(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
pageIndex_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
pageSize_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
total_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Paging_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Paging_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Paging.class, org.sonarqube.ws.Common.Paging.Builder.class);
}
private int bitField0_;
public static final int PAGEINDEX_FIELD_NUMBER = 1;
private int pageIndex_;
/**
* optional int32 pageIndex = 1;
*/
public boolean hasPageIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 pageIndex = 1;
*/
public int getPageIndex() {
return pageIndex_;
}
public static final int PAGESIZE_FIELD_NUMBER = 2;
private int pageSize_;
/**
* optional int32 pageSize = 2;
*/
public boolean hasPageSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 pageSize = 2;
*/
public int getPageSize() {
return pageSize_;
}
public static final int TOTAL_FIELD_NUMBER = 3;
private int total_;
/**
* optional int32 total = 3;
*/
public boolean hasTotal() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 total = 3;
*/
public int getTotal() {
return total_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, pageIndex_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, pageSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, total_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, pageIndex_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, pageSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, total_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Paging parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Paging parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Paging parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Paging parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Paging parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Paging parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Paging parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Paging parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Paging parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Paging parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Paging prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Paging}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Paging)
org.sonarqube.ws.Common.PagingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Paging_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Paging_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Paging.class, org.sonarqube.ws.Common.Paging.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Paging.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
pageIndex_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
pageSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
total_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Paging_descriptor;
}
public org.sonarqube.ws.Common.Paging getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Paging.getDefaultInstance();
}
public org.sonarqube.ws.Common.Paging build() {
org.sonarqube.ws.Common.Paging result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Paging buildPartial() {
org.sonarqube.ws.Common.Paging result = new org.sonarqube.ws.Common.Paging(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pageIndex_ = pageIndex_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.pageSize_ = pageSize_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.total_ = total_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Paging) {
return mergeFrom((org.sonarqube.ws.Common.Paging)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Paging other) {
if (other == org.sonarqube.ws.Common.Paging.getDefaultInstance()) return this;
if (other.hasPageIndex()) {
setPageIndex(other.getPageIndex());
}
if (other.hasPageSize()) {
setPageSize(other.getPageSize());
}
if (other.hasTotal()) {
setTotal(other.getTotal());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Paging parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Paging) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int pageIndex_ ;
/**
* optional int32 pageIndex = 1;
*/
public boolean hasPageIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 pageIndex = 1;
*/
public int getPageIndex() {
return pageIndex_;
}
/**
* optional int32 pageIndex = 1;
*/
public Builder setPageIndex(int value) {
bitField0_ |= 0x00000001;
pageIndex_ = value;
onChanged();
return this;
}
/**
* optional int32 pageIndex = 1;
*/
public Builder clearPageIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
pageIndex_ = 0;
onChanged();
return this;
}
private int pageSize_ ;
/**
* optional int32 pageSize = 2;
*/
public boolean hasPageSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 pageSize = 2;
*/
public int getPageSize() {
return pageSize_;
}
/**
* optional int32 pageSize = 2;
*/
public Builder setPageSize(int value) {
bitField0_ |= 0x00000002;
pageSize_ = value;
onChanged();
return this;
}
/**
* optional int32 pageSize = 2;
*/
public Builder clearPageSize() {
bitField0_ = (bitField0_ & ~0x00000002);
pageSize_ = 0;
onChanged();
return this;
}
private int total_ ;
/**
* optional int32 total = 3;
*/
public boolean hasTotal() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 total = 3;
*/
public int getTotal() {
return total_;
}
/**
* optional int32 total = 3;
*/
public Builder setTotal(int value) {
bitField0_ |= 0x00000004;
total_ = value;
onChanged();
return this;
}
/**
* optional int32 total = 3;
*/
public Builder clearTotal() {
bitField0_ = (bitField0_ & ~0x00000004);
total_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Paging)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Paging)
private static final org.sonarqube.ws.Common.Paging DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Paging();
}
public static org.sonarqube.ws.Common.Paging getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Paging parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Paging(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Paging getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FacetOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Facet)
com.google.protobuf.MessageOrBuilder {
/**
* optional string property = 1;
*
*
* kind of key
*
*/
boolean hasProperty();
/**
* optional string property = 1;
*
*
* kind of key
*
*/
java.lang.String getProperty();
/**
* optional string property = 1;
*
*
* kind of key
*
*/
com.google.protobuf.ByteString
getPropertyBytes();
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
java.util.List
getValuesList();
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
org.sonarqube.ws.Common.FacetValue getValues(int index);
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
int getValuesCount();
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
java.util.List extends org.sonarqube.ws.Common.FacetValueOrBuilder>
getValuesOrBuilderList();
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
org.sonarqube.ws.Common.FacetValueOrBuilder getValuesOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.commons.Facet}
*/
public static final class Facet extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Facet)
FacetOrBuilder {
// Use Facet.newBuilder() to construct.
private Facet(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Facet() {
property_ = "";
values_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Facet(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
property_ = bs;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
values_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
values_.add(input.readMessage(org.sonarqube.ws.Common.FacetValue.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
values_ = java.util.Collections.unmodifiableList(values_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facet_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Facet.class, org.sonarqube.ws.Common.Facet.Builder.class);
}
private int bitField0_;
public static final int PROPERTY_FIELD_NUMBER = 1;
private volatile java.lang.Object property_;
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public boolean hasProperty() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public java.lang.String getProperty() {
java.lang.Object ref = property_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
property_ = s;
}
return s;
}
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public com.google.protobuf.ByteString
getPropertyBytes() {
java.lang.Object ref = property_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
property_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUES_FIELD_NUMBER = 2;
private java.util.List values_;
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public java.util.List getValuesList() {
return values_;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public java.util.List extends org.sonarqube.ws.Common.FacetValueOrBuilder>
getValuesOrBuilderList() {
return values_;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public int getValuesCount() {
return values_.size();
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValue getValues(int index) {
return values_.get(index);
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValueOrBuilder getValuesOrBuilder(
int index) {
return values_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, property_);
}
for (int i = 0; i < values_.size(); i++) {
output.writeMessage(2, values_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, property_);
}
for (int i = 0; i < values_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, values_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Facet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Facet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Facet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Facet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Facet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Facet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Facet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Facet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Facet)
org.sonarqube.ws.Common.FacetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facet_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Facet.class, org.sonarqube.ws.Common.Facet.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Facet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getValuesFieldBuilder();
}
}
public Builder clear() {
super.clear();
property_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
valuesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facet_descriptor;
}
public org.sonarqube.ws.Common.Facet getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Facet.getDefaultInstance();
}
public org.sonarqube.ws.Common.Facet build() {
org.sonarqube.ws.Common.Facet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Facet buildPartial() {
org.sonarqube.ws.Common.Facet result = new org.sonarqube.ws.Common.Facet(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.property_ = property_;
if (valuesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
values_ = java.util.Collections.unmodifiableList(values_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.values_ = values_;
} else {
result.values_ = valuesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Facet) {
return mergeFrom((org.sonarqube.ws.Common.Facet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Facet other) {
if (other == org.sonarqube.ws.Common.Facet.getDefaultInstance()) return this;
if (other.hasProperty()) {
bitField0_ |= 0x00000001;
property_ = other.property_;
onChanged();
}
if (valuesBuilder_ == null) {
if (!other.values_.isEmpty()) {
if (values_.isEmpty()) {
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureValuesIsMutable();
values_.addAll(other.values_);
}
onChanged();
}
} else {
if (!other.values_.isEmpty()) {
if (valuesBuilder_.isEmpty()) {
valuesBuilder_.dispose();
valuesBuilder_ = null;
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000002);
valuesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getValuesFieldBuilder() : null;
} else {
valuesBuilder_.addAllMessages(other.values_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Facet parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Facet) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object property_ = "";
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public boolean hasProperty() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public java.lang.String getProperty() {
java.lang.Object ref = property_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
property_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public com.google.protobuf.ByteString
getPropertyBytes() {
java.lang.Object ref = property_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
property_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public Builder setProperty(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
property_ = value;
onChanged();
return this;
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public Builder clearProperty() {
bitField0_ = (bitField0_ & ~0x00000001);
property_ = getDefaultInstance().getProperty();
onChanged();
return this;
}
/**
* optional string property = 1;
*
*
* kind of key
*
*/
public Builder setPropertyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
property_ = value;
onChanged();
return this;
}
private java.util.List values_ =
java.util.Collections.emptyList();
private void ensureValuesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
values_ = new java.util.ArrayList(values_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.FacetValue, org.sonarqube.ws.Common.FacetValue.Builder, org.sonarqube.ws.Common.FacetValueOrBuilder> valuesBuilder_;
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public java.util.List getValuesList() {
if (valuesBuilder_ == null) {
return java.util.Collections.unmodifiableList(values_);
} else {
return valuesBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public int getValuesCount() {
if (valuesBuilder_ == null) {
return values_.size();
} else {
return valuesBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValue getValues(int index) {
if (valuesBuilder_ == null) {
return values_.get(index);
} else {
return valuesBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder setValues(
int index, org.sonarqube.ws.Common.FacetValue value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.set(index, value);
onChanged();
} else {
valuesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder setValues(
int index, org.sonarqube.ws.Common.FacetValue.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.set(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder addValues(org.sonarqube.ws.Common.FacetValue value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(value);
onChanged();
} else {
valuesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder addValues(
int index, org.sonarqube.ws.Common.FacetValue value) {
if (valuesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(index, value);
onChanged();
} else {
valuesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder addValues(
org.sonarqube.ws.Common.FacetValue.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder addValues(
int index, org.sonarqube.ws.Common.FacetValue.Builder builderForValue) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.add(index, builderForValue.build());
onChanged();
} else {
valuesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder addAllValues(
java.lang.Iterable extends org.sonarqube.ws.Common.FacetValue> values) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, values_);
onChanged();
} else {
valuesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder clearValues() {
if (valuesBuilder_ == null) {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
valuesBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public Builder removeValues(int index) {
if (valuesBuilder_ == null) {
ensureValuesIsMutable();
values_.remove(index);
onChanged();
} else {
valuesBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValue.Builder getValuesBuilder(
int index) {
return getValuesFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValueOrBuilder getValuesOrBuilder(
int index) {
if (valuesBuilder_ == null) {
return values_.get(index); } else {
return valuesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public java.util.List extends org.sonarqube.ws.Common.FacetValueOrBuilder>
getValuesOrBuilderList() {
if (valuesBuilder_ != null) {
return valuesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(values_);
}
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValue.Builder addValuesBuilder() {
return getValuesFieldBuilder().addBuilder(
org.sonarqube.ws.Common.FacetValue.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public org.sonarqube.ws.Common.FacetValue.Builder addValuesBuilder(
int index) {
return getValuesFieldBuilder().addBuilder(
index, org.sonarqube.ws.Common.FacetValue.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.FacetValue values = 2;
*/
public java.util.List
getValuesBuilderList() {
return getValuesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.FacetValue, org.sonarqube.ws.Common.FacetValue.Builder, org.sonarqube.ws.Common.FacetValueOrBuilder>
getValuesFieldBuilder() {
if (valuesBuilder_ == null) {
valuesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.FacetValue, org.sonarqube.ws.Common.FacetValue.Builder, org.sonarqube.ws.Common.FacetValueOrBuilder>(
values_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
values_ = null;
}
return valuesBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Facet)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Facet)
private static final org.sonarqube.ws.Common.Facet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Facet();
}
public static org.sonarqube.ws.Common.Facet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Facet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Facet(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Facet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FacetsOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Facets)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
java.util.List
getFacetsList();
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
org.sonarqube.ws.Common.Facet getFacets(int index);
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
int getFacetsCount();
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
java.util.List extends org.sonarqube.ws.Common.FacetOrBuilder>
getFacetsOrBuilderList();
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
org.sonarqube.ws.Common.FacetOrBuilder getFacetsOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.commons.Facets}
*/
public static final class Facets extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Facets)
FacetsOrBuilder {
// Use Facets.newBuilder() to construct.
private Facets(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Facets() {
facets_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Facets(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
facets_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
facets_.add(input.readMessage(org.sonarqube.ws.Common.Facet.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
facets_ = java.util.Collections.unmodifiableList(facets_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facets_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Facets.class, org.sonarqube.ws.Common.Facets.Builder.class);
}
public static final int FACETS_FIELD_NUMBER = 1;
private java.util.List facets_;
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public java.util.List getFacetsList() {
return facets_;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.FacetOrBuilder>
getFacetsOrBuilderList() {
return facets_;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public int getFacetsCount() {
return facets_.size();
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.Facet getFacets(int index) {
return facets_.get(index);
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.FacetOrBuilder getFacetsOrBuilder(
int index) {
return facets_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < facets_.size(); i++) {
output.writeMessage(1, facets_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < facets_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, facets_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Facets parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Facets parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facets parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Facets parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facets parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Facets parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facets parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Facets parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Facets parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Facets parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Facets prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Facets}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Facets)
org.sonarqube.ws.Common.FacetsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facets_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Facets.class, org.sonarqube.ws.Common.Facets.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Facets.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFacetsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (facetsBuilder_ == null) {
facets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
facetsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Facets_descriptor;
}
public org.sonarqube.ws.Common.Facets getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Facets.getDefaultInstance();
}
public org.sonarqube.ws.Common.Facets build() {
org.sonarqube.ws.Common.Facets result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Facets buildPartial() {
org.sonarqube.ws.Common.Facets result = new org.sonarqube.ws.Common.Facets(this);
int from_bitField0_ = bitField0_;
if (facetsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
facets_ = java.util.Collections.unmodifiableList(facets_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.facets_ = facets_;
} else {
result.facets_ = facetsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Facets) {
return mergeFrom((org.sonarqube.ws.Common.Facets)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Facets other) {
if (other == org.sonarqube.ws.Common.Facets.getDefaultInstance()) return this;
if (facetsBuilder_ == null) {
if (!other.facets_.isEmpty()) {
if (facets_.isEmpty()) {
facets_ = other.facets_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFacetsIsMutable();
facets_.addAll(other.facets_);
}
onChanged();
}
} else {
if (!other.facets_.isEmpty()) {
if (facetsBuilder_.isEmpty()) {
facetsBuilder_.dispose();
facetsBuilder_ = null;
facets_ = other.facets_;
bitField0_ = (bitField0_ & ~0x00000001);
facetsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFacetsFieldBuilder() : null;
} else {
facetsBuilder_.addAllMessages(other.facets_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Facets parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Facets) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List facets_ =
java.util.Collections.emptyList();
private void ensureFacetsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
facets_ = new java.util.ArrayList(facets_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Facet, org.sonarqube.ws.Common.Facet.Builder, org.sonarqube.ws.Common.FacetOrBuilder> facetsBuilder_;
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public java.util.List getFacetsList() {
if (facetsBuilder_ == null) {
return java.util.Collections.unmodifiableList(facets_);
} else {
return facetsBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public int getFacetsCount() {
if (facetsBuilder_ == null) {
return facets_.size();
} else {
return facetsBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.Facet getFacets(int index) {
if (facetsBuilder_ == null) {
return facets_.get(index);
} else {
return facetsBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder setFacets(
int index, org.sonarqube.ws.Common.Facet value) {
if (facetsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetsIsMutable();
facets_.set(index, value);
onChanged();
} else {
facetsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder setFacets(
int index, org.sonarqube.ws.Common.Facet.Builder builderForValue) {
if (facetsBuilder_ == null) {
ensureFacetsIsMutable();
facets_.set(index, builderForValue.build());
onChanged();
} else {
facetsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder addFacets(org.sonarqube.ws.Common.Facet value) {
if (facetsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetsIsMutable();
facets_.add(value);
onChanged();
} else {
facetsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder addFacets(
int index, org.sonarqube.ws.Common.Facet value) {
if (facetsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetsIsMutable();
facets_.add(index, value);
onChanged();
} else {
facetsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder addFacets(
org.sonarqube.ws.Common.Facet.Builder builderForValue) {
if (facetsBuilder_ == null) {
ensureFacetsIsMutable();
facets_.add(builderForValue.build());
onChanged();
} else {
facetsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder addFacets(
int index, org.sonarqube.ws.Common.Facet.Builder builderForValue) {
if (facetsBuilder_ == null) {
ensureFacetsIsMutable();
facets_.add(index, builderForValue.build());
onChanged();
} else {
facetsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder addAllFacets(
java.lang.Iterable extends org.sonarqube.ws.Common.Facet> values) {
if (facetsBuilder_ == null) {
ensureFacetsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, facets_);
onChanged();
} else {
facetsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder clearFacets() {
if (facetsBuilder_ == null) {
facets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
facetsBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public Builder removeFacets(int index) {
if (facetsBuilder_ == null) {
ensureFacetsIsMutable();
facets_.remove(index);
onChanged();
} else {
facetsBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.Facet.Builder getFacetsBuilder(
int index) {
return getFacetsFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.FacetOrBuilder getFacetsOrBuilder(
int index) {
if (facetsBuilder_ == null) {
return facets_.get(index); } else {
return facetsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.FacetOrBuilder>
getFacetsOrBuilderList() {
if (facetsBuilder_ != null) {
return facetsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(facets_);
}
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.Facet.Builder addFacetsBuilder() {
return getFacetsFieldBuilder().addBuilder(
org.sonarqube.ws.Common.Facet.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public org.sonarqube.ws.Common.Facet.Builder addFacetsBuilder(
int index) {
return getFacetsFieldBuilder().addBuilder(
index, org.sonarqube.ws.Common.Facet.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.Facet facets = 1;
*/
public java.util.List
getFacetsBuilderList() {
return getFacetsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Facet, org.sonarqube.ws.Common.Facet.Builder, org.sonarqube.ws.Common.FacetOrBuilder>
getFacetsFieldBuilder() {
if (facetsBuilder_ == null) {
facetsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Facet, org.sonarqube.ws.Common.Facet.Builder, org.sonarqube.ws.Common.FacetOrBuilder>(
facets_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
facets_ = null;
}
return facetsBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Facets)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Facets)
private static final org.sonarqube.ws.Common.Facets DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Facets();
}
public static org.sonarqube.ws.Common.Facets getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Facets parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Facets(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Facets getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FacetValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.FacetValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional string val = 1;
*/
boolean hasVal();
/**
* optional string val = 1;
*/
java.lang.String getVal();
/**
* optional string val = 1;
*/
com.google.protobuf.ByteString
getValBytes();
/**
* optional int64 count = 2;
*/
boolean hasCount();
/**
* optional int64 count = 2;
*/
long getCount();
}
/**
* Protobuf type {@code sonarqube.ws.commons.FacetValue}
*/
public static final class FacetValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.FacetValue)
FacetValueOrBuilder {
// Use FacetValue.newBuilder() to construct.
private FacetValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private FacetValue() {
val_ = "";
count_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FacetValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
val_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
count_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_FacetValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_FacetValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.FacetValue.class, org.sonarqube.ws.Common.FacetValue.Builder.class);
}
private int bitField0_;
public static final int VAL_FIELD_NUMBER = 1;
private volatile java.lang.Object val_;
/**
* optional string val = 1;
*/
public boolean hasVal() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string val = 1;
*/
public java.lang.String getVal() {
java.lang.Object ref = val_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
val_ = s;
}
return s;
}
}
/**
* optional string val = 1;
*/
public com.google.protobuf.ByteString
getValBytes() {
java.lang.Object ref = val_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
val_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COUNT_FIELD_NUMBER = 2;
private long count_;
/**
* optional int64 count = 2;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 count = 2;
*/
public long getCount() {
return count_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, val_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, count_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, val_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, count_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.FacetValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.FacetValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.FacetValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.FacetValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.FacetValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.FacetValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.FacetValue)
org.sonarqube.ws.Common.FacetValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_FacetValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_FacetValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.FacetValue.class, org.sonarqube.ws.Common.FacetValue.Builder.class);
}
// Construct using org.sonarqube.ws.Common.FacetValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
val_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_FacetValue_descriptor;
}
public org.sonarqube.ws.Common.FacetValue getDefaultInstanceForType() {
return org.sonarqube.ws.Common.FacetValue.getDefaultInstance();
}
public org.sonarqube.ws.Common.FacetValue build() {
org.sonarqube.ws.Common.FacetValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.FacetValue buildPartial() {
org.sonarqube.ws.Common.FacetValue result = new org.sonarqube.ws.Common.FacetValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.val_ = val_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.count_ = count_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.FacetValue) {
return mergeFrom((org.sonarqube.ws.Common.FacetValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.FacetValue other) {
if (other == org.sonarqube.ws.Common.FacetValue.getDefaultInstance()) return this;
if (other.hasVal()) {
bitField0_ |= 0x00000001;
val_ = other.val_;
onChanged();
}
if (other.hasCount()) {
setCount(other.getCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.FacetValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.FacetValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object val_ = "";
/**
* optional string val = 1;
*/
public boolean hasVal() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string val = 1;
*/
public java.lang.String getVal() {
java.lang.Object ref = val_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
val_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string val = 1;
*/
public com.google.protobuf.ByteString
getValBytes() {
java.lang.Object ref = val_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
val_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string val = 1;
*/
public Builder setVal(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
val_ = value;
onChanged();
return this;
}
/**
* optional string val = 1;
*/
public Builder clearVal() {
bitField0_ = (bitField0_ & ~0x00000001);
val_ = getDefaultInstance().getVal();
onChanged();
return this;
}
/**
* optional string val = 1;
*/
public Builder setValBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
val_ = value;
onChanged();
return this;
}
private long count_ ;
/**
* optional int64 count = 2;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 count = 2;
*/
public long getCount() {
return count_;
}
/**
* optional int64 count = 2;
*/
public Builder setCount(long value) {
bitField0_ |= 0x00000002;
count_ = value;
onChanged();
return this;
}
/**
* optional int64 count = 2;
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000002);
count_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.FacetValue)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.FacetValue)
private static final org.sonarqube.ws.Common.FacetValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.FacetValue();
}
public static org.sonarqube.ws.Common.FacetValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FacetValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new FacetValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.FacetValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RuleOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Rule)
com.google.protobuf.MessageOrBuilder {
/**
* optional string key = 1;
*/
boolean hasKey();
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional string name = 2;
*/
boolean hasName();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string lang = 3;
*/
boolean hasLang();
/**
* optional string lang = 3;
*/
java.lang.String getLang();
/**
* optional string lang = 3;
*/
com.google.protobuf.ByteString
getLangBytes();
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
boolean hasStatus();
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
org.sonarqube.ws.Common.RuleStatus getStatus();
/**
* optional string langName = 5;
*/
boolean hasLangName();
/**
* optional string langName = 5;
*/
java.lang.String getLangName();
/**
* optional string langName = 5;
*/
com.google.protobuf.ByteString
getLangNameBytes();
}
/**
* Protobuf type {@code sonarqube.ws.commons.Rule}
*/
public static final class Rule extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Rule)
RuleOrBuilder {
// Use Rule.newBuilder() to construct.
private Rule(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Rule() {
key_ = "";
name_ = "";
lang_ = "";
status_ = 0;
langName_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Rule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
key_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
lang_ = bs;
break;
}
case 32: {
int rawValue = input.readEnum();
org.sonarqube.ws.Common.RuleStatus value = org.sonarqube.ws.Common.RuleStatus.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
status_ = rawValue;
}
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
langName_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Rule.class, org.sonarqube.ws.Common.Rule.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANG_FIELD_NUMBER = 3;
private volatile java.lang.Object lang_;
/**
* optional string lang = 3;
*/
public boolean hasLang() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string lang = 3;
*/
public java.lang.String getLang() {
java.lang.Object ref = lang_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
lang_ = s;
}
return s;
}
}
/**
* optional string lang = 3;
*/
public com.google.protobuf.ByteString
getLangBytes() {
java.lang.Object ref = lang_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lang_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 4;
private int status_;
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public org.sonarqube.ws.Common.RuleStatus getStatus() {
org.sonarqube.ws.Common.RuleStatus result = org.sonarqube.ws.Common.RuleStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.Common.RuleStatus.BETA : result;
}
public static final int LANGNAME_FIELD_NUMBER = 5;
private volatile java.lang.Object langName_;
/**
* optional string langName = 5;
*/
public boolean hasLangName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string langName = 5;
*/
public java.lang.String getLangName() {
java.lang.Object ref = langName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
langName_ = s;
}
return s;
}
}
/**
* optional string langName = 5;
*/
public com.google.protobuf.ByteString
getLangNameBytes() {
java.lang.Object ref = langName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
langName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, lang_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, status_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, langName_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, lang_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, status_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, langName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Rule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Rule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Rule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Rule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Rule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Rule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Rule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Rule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Rule)
org.sonarqube.ws.Common.RuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Rule.class, org.sonarqube.ws.Common.Rule.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Rule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
lang_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
status_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
langName_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rule_descriptor;
}
public org.sonarqube.ws.Common.Rule getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Rule.getDefaultInstance();
}
public org.sonarqube.ws.Common.Rule build() {
org.sonarqube.ws.Common.Rule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Rule buildPartial() {
org.sonarqube.ws.Common.Rule result = new org.sonarqube.ws.Common.Rule(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.lang_ = lang_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.status_ = status_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.langName_ = langName_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Rule) {
return mergeFrom((org.sonarqube.ws.Common.Rule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Rule other) {
if (other == org.sonarqube.ws.Common.Rule.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasLang()) {
bitField0_ |= 0x00000004;
lang_ = other.lang_;
onChanged();
}
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasLangName()) {
bitField0_ |= 0x00000010;
langName_ = other.langName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Rule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Rule) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object lang_ = "";
/**
* optional string lang = 3;
*/
public boolean hasLang() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string lang = 3;
*/
public java.lang.String getLang() {
java.lang.Object ref = lang_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
lang_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string lang = 3;
*/
public com.google.protobuf.ByteString
getLangBytes() {
java.lang.Object ref = lang_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lang_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string lang = 3;
*/
public Builder setLang(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
lang_ = value;
onChanged();
return this;
}
/**
* optional string lang = 3;
*/
public Builder clearLang() {
bitField0_ = (bitField0_ & ~0x00000004);
lang_ = getDefaultInstance().getLang();
onChanged();
return this;
}
/**
* optional string lang = 3;
*/
public Builder setLangBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
lang_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public org.sonarqube.ws.Common.RuleStatus getStatus() {
org.sonarqube.ws.Common.RuleStatus result = org.sonarqube.ws.Common.RuleStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.Common.RuleStatus.BETA : result;
}
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public Builder setStatus(org.sonarqube.ws.Common.RuleStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .sonarqube.ws.commons.RuleStatus status = 4;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000008);
status_ = 0;
onChanged();
return this;
}
private java.lang.Object langName_ = "";
/**
* optional string langName = 5;
*/
public boolean hasLangName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string langName = 5;
*/
public java.lang.String getLangName() {
java.lang.Object ref = langName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
langName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string langName = 5;
*/
public com.google.protobuf.ByteString
getLangNameBytes() {
java.lang.Object ref = langName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
langName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string langName = 5;
*/
public Builder setLangName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
langName_ = value;
onChanged();
return this;
}
/**
* optional string langName = 5;
*/
public Builder clearLangName() {
bitField0_ = (bitField0_ & ~0x00000010);
langName_ = getDefaultInstance().getLangName();
onChanged();
return this;
}
/**
* optional string langName = 5;
*/
public Builder setLangNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
langName_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Rule)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Rule)
private static final org.sonarqube.ws.Common.Rule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Rule();
}
public static org.sonarqube.ws.Common.Rule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Rule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Rule(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Rule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RulesOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Rules)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
java.util.List
getRulesList();
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
org.sonarqube.ws.Common.Rule getRules(int index);
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
int getRulesCount();
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
java.util.List extends org.sonarqube.ws.Common.RuleOrBuilder>
getRulesOrBuilderList();
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
org.sonarqube.ws.Common.RuleOrBuilder getRulesOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.commons.Rules}
*/
public static final class Rules extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Rules)
RulesOrBuilder {
// Use Rules.newBuilder() to construct.
private Rules(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Rules() {
rules_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Rules(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rules_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
rules_.add(input.readMessage(org.sonarqube.ws.Common.Rule.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rules_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rules_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Rules.class, org.sonarqube.ws.Common.Rules.Builder.class);
}
public static final int RULES_FIELD_NUMBER = 1;
private java.util.List rules_;
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public java.util.List getRulesList() {
return rules_;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.RuleOrBuilder>
getRulesOrBuilderList() {
return rules_;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public int getRulesCount() {
return rules_.size();
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.Rule getRules(int index) {
return rules_.get(index);
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.RuleOrBuilder getRulesOrBuilder(
int index) {
return rules_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < rules_.size(); i++) {
output.writeMessage(1, rules_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < rules_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, rules_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Rules parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Rules parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rules parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Rules parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rules parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Rules parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rules parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Rules parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Rules parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Rules parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Rules prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Rules}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Rules)
org.sonarqube.ws.Common.RulesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rules_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rules_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Rules.class, org.sonarqube.ws.Common.Rules.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Rules.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRulesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
rulesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Rules_descriptor;
}
public org.sonarqube.ws.Common.Rules getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Rules.getDefaultInstance();
}
public org.sonarqube.ws.Common.Rules build() {
org.sonarqube.ws.Common.Rules result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Rules buildPartial() {
org.sonarqube.ws.Common.Rules result = new org.sonarqube.ws.Common.Rules(this);
int from_bitField0_ = bitField0_;
if (rulesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
rules_ = java.util.Collections.unmodifiableList(rules_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.rules_ = rules_;
} else {
result.rules_ = rulesBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Rules) {
return mergeFrom((org.sonarqube.ws.Common.Rules)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Rules other) {
if (other == org.sonarqube.ws.Common.Rules.getDefaultInstance()) return this;
if (rulesBuilder_ == null) {
if (!other.rules_.isEmpty()) {
if (rules_.isEmpty()) {
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRulesIsMutable();
rules_.addAll(other.rules_);
}
onChanged();
}
} else {
if (!other.rules_.isEmpty()) {
if (rulesBuilder_.isEmpty()) {
rulesBuilder_.dispose();
rulesBuilder_ = null;
rules_ = other.rules_;
bitField0_ = (bitField0_ & ~0x00000001);
rulesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRulesFieldBuilder() : null;
} else {
rulesBuilder_.addAllMessages(other.rules_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Rules parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Rules) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List rules_ =
java.util.Collections.emptyList();
private void ensureRulesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
rules_ = new java.util.ArrayList(rules_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Rule, org.sonarqube.ws.Common.Rule.Builder, org.sonarqube.ws.Common.RuleOrBuilder> rulesBuilder_;
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public java.util.List getRulesList() {
if (rulesBuilder_ == null) {
return java.util.Collections.unmodifiableList(rules_);
} else {
return rulesBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public int getRulesCount() {
if (rulesBuilder_ == null) {
return rules_.size();
} else {
return rulesBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.Rule getRules(int index) {
if (rulesBuilder_ == null) {
return rules_.get(index);
} else {
return rulesBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder setRules(
int index, org.sonarqube.ws.Common.Rule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.set(index, value);
onChanged();
} else {
rulesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder setRules(
int index, org.sonarqube.ws.Common.Rule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.set(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder addRules(org.sonarqube.ws.Common.Rule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(value);
onChanged();
} else {
rulesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder addRules(
int index, org.sonarqube.ws.Common.Rule value) {
if (rulesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRulesIsMutable();
rules_.add(index, value);
onChanged();
} else {
rulesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder addRules(
org.sonarqube.ws.Common.Rule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder addRules(
int index, org.sonarqube.ws.Common.Rule.Builder builderForValue) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.add(index, builderForValue.build());
onChanged();
} else {
rulesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder addAllRules(
java.lang.Iterable extends org.sonarqube.ws.Common.Rule> values) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rules_);
onChanged();
} else {
rulesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder clearRules() {
if (rulesBuilder_ == null) {
rules_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
rulesBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public Builder removeRules(int index) {
if (rulesBuilder_ == null) {
ensureRulesIsMutable();
rules_.remove(index);
onChanged();
} else {
rulesBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.Rule.Builder getRulesBuilder(
int index) {
return getRulesFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.RuleOrBuilder getRulesOrBuilder(
int index) {
if (rulesBuilder_ == null) {
return rules_.get(index); } else {
return rulesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.RuleOrBuilder>
getRulesOrBuilderList() {
if (rulesBuilder_ != null) {
return rulesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rules_);
}
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.Rule.Builder addRulesBuilder() {
return getRulesFieldBuilder().addBuilder(
org.sonarqube.ws.Common.Rule.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public org.sonarqube.ws.Common.Rule.Builder addRulesBuilder(
int index) {
return getRulesFieldBuilder().addBuilder(
index, org.sonarqube.ws.Common.Rule.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.Rule rules = 1;
*/
public java.util.List
getRulesBuilderList() {
return getRulesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Rule, org.sonarqube.ws.Common.Rule.Builder, org.sonarqube.ws.Common.RuleOrBuilder>
getRulesFieldBuilder() {
if (rulesBuilder_ == null) {
rulesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.Rule, org.sonarqube.ws.Common.Rule.Builder, org.sonarqube.ws.Common.RuleOrBuilder>(
rules_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
rules_ = null;
}
return rulesBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Rules)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Rules)
private static final org.sonarqube.ws.Common.Rules DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Rules();
}
public static org.sonarqube.ws.Common.Rules getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Rules parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Rules(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Rules getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.User)
com.google.protobuf.MessageOrBuilder {
/**
* optional string login = 1;
*/
boolean hasLogin();
/**
* optional string login = 1;
*/
java.lang.String getLogin();
/**
* optional string login = 1;
*/
com.google.protobuf.ByteString
getLoginBytes();
/**
* optional string name = 2;
*/
boolean hasName();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string email = 3;
*/
boolean hasEmail();
/**
* optional string email = 3;
*/
java.lang.String getEmail();
/**
* optional string email = 3;
*/
com.google.protobuf.ByteString
getEmailBytes();
/**
* optional bool active = 4;
*/
boolean hasActive();
/**
* optional bool active = 4;
*/
boolean getActive();
}
/**
* Protobuf type {@code sonarqube.ws.commons.User}
*/
public static final class User extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.User)
UserOrBuilder {
// Use User.newBuilder() to construct.
private User(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private User() {
login_ = "";
name_ = "";
email_ = "";
active_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private User(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
login_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
email_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
active_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_User_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_User_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.User.class, org.sonarqube.ws.Common.User.Builder.class);
}
private int bitField0_;
public static final int LOGIN_FIELD_NUMBER = 1;
private volatile java.lang.Object login_;
/**
* optional string login = 1;
*/
public boolean hasLogin() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string login = 1;
*/
public java.lang.String getLogin() {
java.lang.Object ref = login_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
login_ = s;
}
return s;
}
}
/**
* optional string login = 1;
*/
public com.google.protobuf.ByteString
getLoginBytes() {
java.lang.Object ref = login_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
login_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EMAIL_FIELD_NUMBER = 3;
private volatile java.lang.Object email_;
/**
* optional string email = 3;
*/
public boolean hasEmail() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string email = 3;
*/
public java.lang.String getEmail() {
java.lang.Object ref = email_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
email_ = s;
}
return s;
}
}
/**
* optional string email = 3;
*/
public com.google.protobuf.ByteString
getEmailBytes() {
java.lang.Object ref = email_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
email_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIVE_FIELD_NUMBER = 4;
private boolean active_;
/**
* optional bool active = 4;
*/
public boolean hasActive() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool active = 4;
*/
public boolean getActive() {
return active_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, login_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, email_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, active_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, login_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, email_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, active_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.User parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.User parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.User parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.User parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.User parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.User parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.User parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.User parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.User parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.User parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.User prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.User}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.User)
org.sonarqube.ws.Common.UserOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_User_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_User_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.User.class, org.sonarqube.ws.Common.User.Builder.class);
}
// Construct using org.sonarqube.ws.Common.User.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
login_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
email_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
active_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_User_descriptor;
}
public org.sonarqube.ws.Common.User getDefaultInstanceForType() {
return org.sonarqube.ws.Common.User.getDefaultInstance();
}
public org.sonarqube.ws.Common.User build() {
org.sonarqube.ws.Common.User result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.User buildPartial() {
org.sonarqube.ws.Common.User result = new org.sonarqube.ws.Common.User(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.login_ = login_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.email_ = email_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.active_ = active_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.User) {
return mergeFrom((org.sonarqube.ws.Common.User)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.User other) {
if (other == org.sonarqube.ws.Common.User.getDefaultInstance()) return this;
if (other.hasLogin()) {
bitField0_ |= 0x00000001;
login_ = other.login_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasEmail()) {
bitField0_ |= 0x00000004;
email_ = other.email_;
onChanged();
}
if (other.hasActive()) {
setActive(other.getActive());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.User parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.User) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object login_ = "";
/**
* optional string login = 1;
*/
public boolean hasLogin() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string login = 1;
*/
public java.lang.String getLogin() {
java.lang.Object ref = login_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
login_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string login = 1;
*/
public com.google.protobuf.ByteString
getLoginBytes() {
java.lang.Object ref = login_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
login_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string login = 1;
*/
public Builder setLogin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
login_ = value;
onChanged();
return this;
}
/**
* optional string login = 1;
*/
public Builder clearLogin() {
bitField0_ = (bitField0_ & ~0x00000001);
login_ = getDefaultInstance().getLogin();
onChanged();
return this;
}
/**
* optional string login = 1;
*/
public Builder setLoginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
login_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object email_ = "";
/**
* optional string email = 3;
*/
public boolean hasEmail() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string email = 3;
*/
public java.lang.String getEmail() {
java.lang.Object ref = email_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
email_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string email = 3;
*/
public com.google.protobuf.ByteString
getEmailBytes() {
java.lang.Object ref = email_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
email_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string email = 3;
*/
public Builder setEmail(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
email_ = value;
onChanged();
return this;
}
/**
* optional string email = 3;
*/
public Builder clearEmail() {
bitField0_ = (bitField0_ & ~0x00000004);
email_ = getDefaultInstance().getEmail();
onChanged();
return this;
}
/**
* optional string email = 3;
*/
public Builder setEmailBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
email_ = value;
onChanged();
return this;
}
private boolean active_ ;
/**
* optional bool active = 4;
*/
public boolean hasActive() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool active = 4;
*/
public boolean getActive() {
return active_;
}
/**
* optional bool active = 4;
*/
public Builder setActive(boolean value) {
bitField0_ |= 0x00000008;
active_ = value;
onChanged();
return this;
}
/**
* optional bool active = 4;
*/
public Builder clearActive() {
bitField0_ = (bitField0_ & ~0x00000008);
active_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.User)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.User)
private static final org.sonarqube.ws.Common.User DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.User();
}
public static org.sonarqube.ws.Common.User getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public User parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new User(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.User getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UsersOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Users)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
java.util.List
getUsersList();
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
org.sonarqube.ws.Common.User getUsers(int index);
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
int getUsersCount();
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
java.util.List extends org.sonarqube.ws.Common.UserOrBuilder>
getUsersOrBuilderList();
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
org.sonarqube.ws.Common.UserOrBuilder getUsersOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.commons.Users}
*/
public static final class Users extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Users)
UsersOrBuilder {
// Use Users.newBuilder() to construct.
private Users(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Users() {
users_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Users(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
users_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
users_.add(input.readMessage(org.sonarqube.ws.Common.User.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
users_ = java.util.Collections.unmodifiableList(users_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Users_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Users_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Users.class, org.sonarqube.ws.Common.Users.Builder.class);
}
public static final int USERS_FIELD_NUMBER = 1;
private java.util.List users_;
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public java.util.List getUsersList() {
return users_;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.UserOrBuilder>
getUsersOrBuilderList() {
return users_;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public int getUsersCount() {
return users_.size();
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.User getUsers(int index) {
return users_.get(index);
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.UserOrBuilder getUsersOrBuilder(
int index) {
return users_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < users_.size(); i++) {
output.writeMessage(1, users_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < users_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, users_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Users parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Users parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Users parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Users parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Users parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Users parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Users parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Users parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Users parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Users parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Users prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Users}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Users)
org.sonarqube.ws.Common.UsersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Users_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Users_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Users.class, org.sonarqube.ws.Common.Users.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Users.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getUsersFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (usersBuilder_ == null) {
users_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
usersBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Users_descriptor;
}
public org.sonarqube.ws.Common.Users getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Users.getDefaultInstance();
}
public org.sonarqube.ws.Common.Users build() {
org.sonarqube.ws.Common.Users result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Users buildPartial() {
org.sonarqube.ws.Common.Users result = new org.sonarqube.ws.Common.Users(this);
int from_bitField0_ = bitField0_;
if (usersBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
users_ = java.util.Collections.unmodifiableList(users_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.users_ = users_;
} else {
result.users_ = usersBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Users) {
return mergeFrom((org.sonarqube.ws.Common.Users)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Users other) {
if (other == org.sonarqube.ws.Common.Users.getDefaultInstance()) return this;
if (usersBuilder_ == null) {
if (!other.users_.isEmpty()) {
if (users_.isEmpty()) {
users_ = other.users_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUsersIsMutable();
users_.addAll(other.users_);
}
onChanged();
}
} else {
if (!other.users_.isEmpty()) {
if (usersBuilder_.isEmpty()) {
usersBuilder_.dispose();
usersBuilder_ = null;
users_ = other.users_;
bitField0_ = (bitField0_ & ~0x00000001);
usersBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getUsersFieldBuilder() : null;
} else {
usersBuilder_.addAllMessages(other.users_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Users parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Users) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List users_ =
java.util.Collections.emptyList();
private void ensureUsersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
users_ = new java.util.ArrayList(users_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.User, org.sonarqube.ws.Common.User.Builder, org.sonarqube.ws.Common.UserOrBuilder> usersBuilder_;
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public java.util.List getUsersList() {
if (usersBuilder_ == null) {
return java.util.Collections.unmodifiableList(users_);
} else {
return usersBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public int getUsersCount() {
if (usersBuilder_ == null) {
return users_.size();
} else {
return usersBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.User getUsers(int index) {
if (usersBuilder_ == null) {
return users_.get(index);
} else {
return usersBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder setUsers(
int index, org.sonarqube.ws.Common.User value) {
if (usersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.set(index, value);
onChanged();
} else {
usersBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder setUsers(
int index, org.sonarqube.ws.Common.User.Builder builderForValue) {
if (usersBuilder_ == null) {
ensureUsersIsMutable();
users_.set(index, builderForValue.build());
onChanged();
} else {
usersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder addUsers(org.sonarqube.ws.Common.User value) {
if (usersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.add(value);
onChanged();
} else {
usersBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder addUsers(
int index, org.sonarqube.ws.Common.User value) {
if (usersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.add(index, value);
onChanged();
} else {
usersBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder addUsers(
org.sonarqube.ws.Common.User.Builder builderForValue) {
if (usersBuilder_ == null) {
ensureUsersIsMutable();
users_.add(builderForValue.build());
onChanged();
} else {
usersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder addUsers(
int index, org.sonarqube.ws.Common.User.Builder builderForValue) {
if (usersBuilder_ == null) {
ensureUsersIsMutable();
users_.add(index, builderForValue.build());
onChanged();
} else {
usersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder addAllUsers(
java.lang.Iterable extends org.sonarqube.ws.Common.User> values) {
if (usersBuilder_ == null) {
ensureUsersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, users_);
onChanged();
} else {
usersBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder clearUsers() {
if (usersBuilder_ == null) {
users_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
usersBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public Builder removeUsers(int index) {
if (usersBuilder_ == null) {
ensureUsersIsMutable();
users_.remove(index);
onChanged();
} else {
usersBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.User.Builder getUsersBuilder(
int index) {
return getUsersFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.UserOrBuilder getUsersOrBuilder(
int index) {
if (usersBuilder_ == null) {
return users_.get(index); } else {
return usersBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public java.util.List extends org.sonarqube.ws.Common.UserOrBuilder>
getUsersOrBuilderList() {
if (usersBuilder_ != null) {
return usersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(users_);
}
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.User.Builder addUsersBuilder() {
return getUsersFieldBuilder().addBuilder(
org.sonarqube.ws.Common.User.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public org.sonarqube.ws.Common.User.Builder addUsersBuilder(
int index) {
return getUsersFieldBuilder().addBuilder(
index, org.sonarqube.ws.Common.User.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.commons.User users = 1;
*/
public java.util.List
getUsersBuilderList() {
return getUsersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.User, org.sonarqube.ws.Common.User.Builder, org.sonarqube.ws.Common.UserOrBuilder>
getUsersFieldBuilder() {
if (usersBuilder_ == null) {
usersBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.Common.User, org.sonarqube.ws.Common.User.Builder, org.sonarqube.ws.Common.UserOrBuilder>(
users_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
users_ = null;
}
return usersBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Users)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Users)
private static final org.sonarqube.ws.Common.Users DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Users();
}
public static org.sonarqube.ws.Common.Users getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Users parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Users(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Users getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TextRangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.TextRange)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
boolean hasStartLine();
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
int getStartLine();
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
boolean hasEndLine();
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
int getEndLine();
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
boolean hasStartOffset();
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
int getStartOffset();
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
boolean hasEndOffset();
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
int getEndOffset();
}
/**
* Protobuf type {@code sonarqube.ws.commons.TextRange}
*
*
* Lines start at 1 and line offsets start at 0
*
*/
public static final class TextRange extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.TextRange)
TextRangeOrBuilder {
// Use TextRange.newBuilder() to construct.
private TextRange(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TextRange() {
startLine_ = 0;
endLine_ = 0;
startOffset_ = 0;
endOffset_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TextRange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
startLine_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
endLine_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
startOffset_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
endOffset_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_TextRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_TextRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.TextRange.class, org.sonarqube.ws.Common.TextRange.Builder.class);
}
private int bitField0_;
public static final int STARTLINE_FIELD_NUMBER = 1;
private int startLine_;
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public boolean hasStartLine() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public int getStartLine() {
return startLine_;
}
public static final int ENDLINE_FIELD_NUMBER = 2;
private int endLine_;
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public boolean hasEndLine() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public int getEndLine() {
return endLine_;
}
public static final int STARTOFFSET_FIELD_NUMBER = 3;
private int startOffset_;
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public boolean hasStartOffset() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public int getStartOffset() {
return startOffset_;
}
public static final int ENDOFFSET_FIELD_NUMBER = 4;
private int endOffset_;
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public boolean hasEndOffset() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public int getEndOffset() {
return endOffset_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, startLine_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, endLine_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, startOffset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, endOffset_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, startLine_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, endLine_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, startOffset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, endOffset_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.TextRange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.TextRange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.TextRange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.TextRange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.TextRange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.TextRange}
*
*
* Lines start at 1 and line offsets start at 0
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.TextRange)
org.sonarqube.ws.Common.TextRangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_TextRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_TextRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.TextRange.class, org.sonarqube.ws.Common.TextRange.Builder.class);
}
// Construct using org.sonarqube.ws.Common.TextRange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
startLine_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
endLine_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
startOffset_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
endOffset_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_TextRange_descriptor;
}
public org.sonarqube.ws.Common.TextRange getDefaultInstanceForType() {
return org.sonarqube.ws.Common.TextRange.getDefaultInstance();
}
public org.sonarqube.ws.Common.TextRange build() {
org.sonarqube.ws.Common.TextRange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.TextRange buildPartial() {
org.sonarqube.ws.Common.TextRange result = new org.sonarqube.ws.Common.TextRange(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.startLine_ = startLine_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.endLine_ = endLine_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.startOffset_ = startOffset_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.endOffset_ = endOffset_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.TextRange) {
return mergeFrom((org.sonarqube.ws.Common.TextRange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.TextRange other) {
if (other == org.sonarqube.ws.Common.TextRange.getDefaultInstance()) return this;
if (other.hasStartLine()) {
setStartLine(other.getStartLine());
}
if (other.hasEndLine()) {
setEndLine(other.getEndLine());
}
if (other.hasStartOffset()) {
setStartOffset(other.getStartOffset());
}
if (other.hasEndOffset()) {
setEndOffset(other.getEndOffset());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.TextRange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.TextRange) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int startLine_ ;
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public boolean hasStartLine() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public int getStartLine() {
return startLine_;
}
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public Builder setStartLine(int value) {
bitField0_ |= 0x00000001;
startLine_ = value;
onChanged();
return this;
}
/**
* optional int32 startLine = 1;
*
*
* Start line. Should never be absent
*
*/
public Builder clearStartLine() {
bitField0_ = (bitField0_ & ~0x00000001);
startLine_ = 0;
onChanged();
return this;
}
private int endLine_ ;
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public boolean hasEndLine() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public int getEndLine() {
return endLine_;
}
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public Builder setEndLine(int value) {
bitField0_ |= 0x00000002;
endLine_ = value;
onChanged();
return this;
}
/**
* optional int32 endLine = 2;
*
*
* End line (inclusive). Absent means it is same as start line
*
*/
public Builder clearEndLine() {
bitField0_ = (bitField0_ & ~0x00000002);
endLine_ = 0;
onChanged();
return this;
}
private int startOffset_ ;
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public boolean hasStartOffset() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public int getStartOffset() {
return startOffset_;
}
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public Builder setStartOffset(int value) {
bitField0_ |= 0x00000004;
startOffset_ = value;
onChanged();
return this;
}
/**
* optional int32 startOffset = 3;
*
*
* If absent it means range starts at the first offset of start line
*
*/
public Builder clearStartOffset() {
bitField0_ = (bitField0_ & ~0x00000004);
startOffset_ = 0;
onChanged();
return this;
}
private int endOffset_ ;
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public boolean hasEndOffset() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public int getEndOffset() {
return endOffset_;
}
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public Builder setEndOffset(int value) {
bitField0_ |= 0x00000008;
endOffset_ = value;
onChanged();
return this;
}
/**
* optional int32 endOffset = 4;
*
*
* If absent it means range ends at the last offset of end line
*
*/
public Builder clearEndOffset() {
bitField0_ = (bitField0_ & ~0x00000008);
endOffset_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.TextRange)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.TextRange)
private static final org.sonarqube.ws.Common.TextRange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.TextRange();
}
public static org.sonarqube.ws.Common.TextRange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TextRange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new TextRange(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.TextRange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MetricOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.commons.Metric)
com.google.protobuf.MessageOrBuilder {
/**
* optional string key = 1;
*/
boolean hasKey();
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional string name = 2;
*/
boolean hasName();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string description = 3;
*/
boolean hasDescription();
/**
* optional string description = 3;
*/
java.lang.String getDescription();
/**
* optional string description = 3;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* optional string domain = 4;
*/
boolean hasDomain();
/**
* optional string domain = 4;
*/
java.lang.String getDomain();
/**
* optional string domain = 4;
*/
com.google.protobuf.ByteString
getDomainBytes();
/**
* optional string type = 5;
*/
boolean hasType();
/**
* optional string type = 5;
*/
java.lang.String getType();
/**
* optional string type = 5;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* optional bool higherValuesAreBetter = 6;
*/
boolean hasHigherValuesAreBetter();
/**
* optional bool higherValuesAreBetter = 6;
*/
boolean getHigherValuesAreBetter();
/**
* optional bool qualitative = 7;
*/
boolean hasQualitative();
/**
* optional bool qualitative = 7;
*/
boolean getQualitative();
/**
* optional bool hidden = 8;
*/
boolean hasHidden();
/**
* optional bool hidden = 8;
*/
boolean getHidden();
/**
* optional bool custom = 9;
*/
boolean hasCustom();
/**
* optional bool custom = 9;
*/
boolean getCustom();
/**
* optional int32 decimalScale = 10;
*/
boolean hasDecimalScale();
/**
* optional int32 decimalScale = 10;
*/
int getDecimalScale();
/**
* optional string bestValue = 11;
*/
boolean hasBestValue();
/**
* optional string bestValue = 11;
*/
java.lang.String getBestValue();
/**
* optional string bestValue = 11;
*/
com.google.protobuf.ByteString
getBestValueBytes();
/**
* optional string worstValue = 12;
*/
boolean hasWorstValue();
/**
* optional string worstValue = 12;
*/
java.lang.String getWorstValue();
/**
* optional string worstValue = 12;
*/
com.google.protobuf.ByteString
getWorstValueBytes();
}
/**
* Protobuf type {@code sonarqube.ws.commons.Metric}
*/
public static final class Metric extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.commons.Metric)
MetricOrBuilder {
// Use Metric.newBuilder() to construct.
private Metric(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Metric() {
key_ = "";
name_ = "";
description_ = "";
domain_ = "";
type_ = "";
higherValuesAreBetter_ = false;
qualitative_ = false;
hidden_ = false;
custom_ = false;
decimalScale_ = 0;
bestValue_ = "";
worstValue_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Metric(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
key_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
description_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
domain_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
type_ = bs;
break;
}
case 48: {
bitField0_ |= 0x00000020;
higherValuesAreBetter_ = input.readBool();
break;
}
case 56: {
bitField0_ |= 0x00000040;
qualitative_ = input.readBool();
break;
}
case 64: {
bitField0_ |= 0x00000080;
hidden_ = input.readBool();
break;
}
case 72: {
bitField0_ |= 0x00000100;
custom_ = input.readBool();
break;
}
case 80: {
bitField0_ |= 0x00000200;
decimalScale_ = input.readInt32();
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
bestValue_ = bs;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
worstValue_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Metric_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Metric_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Metric.class, org.sonarqube.ws.Common.Metric.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object description_;
/**
* optional string description = 3;
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
}
}
/**
* optional string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DOMAIN_FIELD_NUMBER = 4;
private volatile java.lang.Object domain_;
/**
* optional string domain = 4;
*/
public boolean hasDomain() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string domain = 4;
*/
public java.lang.String getDomain() {
java.lang.Object ref = domain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
domain_ = s;
}
return s;
}
}
/**
* optional string domain = 4;
*/
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = domain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
domain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 5;
private volatile java.lang.Object type_;
/**
* optional string type = 5;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string type = 5;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 5;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HIGHERVALUESAREBETTER_FIELD_NUMBER = 6;
private boolean higherValuesAreBetter_;
/**
* optional bool higherValuesAreBetter = 6;
*/
public boolean hasHigherValuesAreBetter() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool higherValuesAreBetter = 6;
*/
public boolean getHigherValuesAreBetter() {
return higherValuesAreBetter_;
}
public static final int QUALITATIVE_FIELD_NUMBER = 7;
private boolean qualitative_;
/**
* optional bool qualitative = 7;
*/
public boolean hasQualitative() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool qualitative = 7;
*/
public boolean getQualitative() {
return qualitative_;
}
public static final int HIDDEN_FIELD_NUMBER = 8;
private boolean hidden_;
/**
* optional bool hidden = 8;
*/
public boolean hasHidden() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool hidden = 8;
*/
public boolean getHidden() {
return hidden_;
}
public static final int CUSTOM_FIELD_NUMBER = 9;
private boolean custom_;
/**
* optional bool custom = 9;
*/
public boolean hasCustom() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool custom = 9;
*/
public boolean getCustom() {
return custom_;
}
public static final int DECIMALSCALE_FIELD_NUMBER = 10;
private int decimalScale_;
/**
* optional int32 decimalScale = 10;
*/
public boolean hasDecimalScale() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 decimalScale = 10;
*/
public int getDecimalScale() {
return decimalScale_;
}
public static final int BESTVALUE_FIELD_NUMBER = 11;
private volatile java.lang.Object bestValue_;
/**
* optional string bestValue = 11;
*/
public boolean hasBestValue() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string bestValue = 11;
*/
public java.lang.String getBestValue() {
java.lang.Object ref = bestValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
bestValue_ = s;
}
return s;
}
}
/**
* optional string bestValue = 11;
*/
public com.google.protobuf.ByteString
getBestValueBytes() {
java.lang.Object ref = bestValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bestValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WORSTVALUE_FIELD_NUMBER = 12;
private volatile java.lang.Object worstValue_;
/**
* optional string worstValue = 12;
*/
public boolean hasWorstValue() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string worstValue = 12;
*/
public java.lang.String getWorstValue() {
java.lang.Object ref = worstValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
worstValue_ = s;
}
return s;
}
}
/**
* optional string worstValue = 12;
*/
public com.google.protobuf.ByteString
getWorstValueBytes() {
java.lang.Object ref = worstValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
worstValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, description_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, domain_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, type_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(6, higherValuesAreBetter_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(7, qualitative_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBool(8, hidden_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, custom_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(10, decimalScale_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessage.writeString(output, 11, bestValue_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
com.google.protobuf.GeneratedMessage.writeString(output, 12, worstValue_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, description_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, domain_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, type_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, higherValuesAreBetter_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, qualitative_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, hidden_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, custom_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, decimalScale_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(11, bestValue_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(12, worstValue_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.Common.Metric parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Metric parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Metric parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.Common.Metric parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.Common.Metric parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Metric parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Metric parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.Common.Metric parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.Common.Metric parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.Common.Metric parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.Common.Metric prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.commons.Metric}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.commons.Metric)
org.sonarqube.ws.Common.MetricOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Metric_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Metric_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.Common.Metric.class, org.sonarqube.ws.Common.Metric.Builder.class);
}
// Construct using org.sonarqube.ws.Common.Metric.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
description_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
domain_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
higherValuesAreBetter_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
qualitative_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
hidden_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
custom_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
decimalScale_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
bestValue_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
worstValue_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.Common.internal_static_sonarqube_ws_commons_Metric_descriptor;
}
public org.sonarqube.ws.Common.Metric getDefaultInstanceForType() {
return org.sonarqube.ws.Common.Metric.getDefaultInstance();
}
public org.sonarqube.ws.Common.Metric build() {
org.sonarqube.ws.Common.Metric result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.Common.Metric buildPartial() {
org.sonarqube.ws.Common.Metric result = new org.sonarqube.ws.Common.Metric(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.description_ = description_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.domain_ = domain_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.higherValuesAreBetter_ = higherValuesAreBetter_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.qualitative_ = qualitative_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.hidden_ = hidden_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.custom_ = custom_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.decimalScale_ = decimalScale_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.bestValue_ = bestValue_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.worstValue_ = worstValue_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.Common.Metric) {
return mergeFrom((org.sonarqube.ws.Common.Metric)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.Common.Metric other) {
if (other == org.sonarqube.ws.Common.Metric.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasDescription()) {
bitField0_ |= 0x00000004;
description_ = other.description_;
onChanged();
}
if (other.hasDomain()) {
bitField0_ |= 0x00000008;
domain_ = other.domain_;
onChanged();
}
if (other.hasType()) {
bitField0_ |= 0x00000010;
type_ = other.type_;
onChanged();
}
if (other.hasHigherValuesAreBetter()) {
setHigherValuesAreBetter(other.getHigherValuesAreBetter());
}
if (other.hasQualitative()) {
setQualitative(other.getQualitative());
}
if (other.hasHidden()) {
setHidden(other.getHidden());
}
if (other.hasCustom()) {
setCustom(other.getCustom());
}
if (other.hasDecimalScale()) {
setDecimalScale(other.getDecimalScale());
}
if (other.hasBestValue()) {
bitField0_ |= 0x00000400;
bestValue_ = other.bestValue_;
onChanged();
}
if (other.hasWorstValue()) {
bitField0_ |= 0x00000800;
worstValue_ = other.worstValue_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.Common.Metric parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.Common.Metric) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* optional string description = 3;
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string description = 3;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
description_ = value;
onChanged();
return this;
}
/**
* optional string description = 3;
*/
public Builder clearDescription() {
bitField0_ = (bitField0_ & ~0x00000004);
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* optional string description = 3;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
description_ = value;
onChanged();
return this;
}
private java.lang.Object domain_ = "";
/**
* optional string domain = 4;
*/
public boolean hasDomain() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string domain = 4;
*/
public java.lang.String getDomain() {
java.lang.Object ref = domain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
domain_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string domain = 4;
*/
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = domain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
domain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string domain = 4;
*/
public Builder setDomain(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
domain_ = value;
onChanged();
return this;
}
/**
* optional string domain = 4;
*/
public Builder clearDomain() {
bitField0_ = (bitField0_ & ~0x00000008);
domain_ = getDefaultInstance().getDomain();
onChanged();
return this;
}
/**
* optional string domain = 4;
*/
public Builder setDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
domain_ = value;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* optional string type = 5;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string type = 5;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 5;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 5;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 5;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000010);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 5;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
type_ = value;
onChanged();
return this;
}
private boolean higherValuesAreBetter_ ;
/**
* optional bool higherValuesAreBetter = 6;
*/
public boolean hasHigherValuesAreBetter() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool higherValuesAreBetter = 6;
*/
public boolean getHigherValuesAreBetter() {
return higherValuesAreBetter_;
}
/**
* optional bool higherValuesAreBetter = 6;
*/
public Builder setHigherValuesAreBetter(boolean value) {
bitField0_ |= 0x00000020;
higherValuesAreBetter_ = value;
onChanged();
return this;
}
/**
* optional bool higherValuesAreBetter = 6;
*/
public Builder clearHigherValuesAreBetter() {
bitField0_ = (bitField0_ & ~0x00000020);
higherValuesAreBetter_ = false;
onChanged();
return this;
}
private boolean qualitative_ ;
/**
* optional bool qualitative = 7;
*/
public boolean hasQualitative() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool qualitative = 7;
*/
public boolean getQualitative() {
return qualitative_;
}
/**
* optional bool qualitative = 7;
*/
public Builder setQualitative(boolean value) {
bitField0_ |= 0x00000040;
qualitative_ = value;
onChanged();
return this;
}
/**
* optional bool qualitative = 7;
*/
public Builder clearQualitative() {
bitField0_ = (bitField0_ & ~0x00000040);
qualitative_ = false;
onChanged();
return this;
}
private boolean hidden_ ;
/**
* optional bool hidden = 8;
*/
public boolean hasHidden() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool hidden = 8;
*/
public boolean getHidden() {
return hidden_;
}
/**
* optional bool hidden = 8;
*/
public Builder setHidden(boolean value) {
bitField0_ |= 0x00000080;
hidden_ = value;
onChanged();
return this;
}
/**
* optional bool hidden = 8;
*/
public Builder clearHidden() {
bitField0_ = (bitField0_ & ~0x00000080);
hidden_ = false;
onChanged();
return this;
}
private boolean custom_ ;
/**
* optional bool custom = 9;
*/
public boolean hasCustom() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool custom = 9;
*/
public boolean getCustom() {
return custom_;
}
/**
* optional bool custom = 9;
*/
public Builder setCustom(boolean value) {
bitField0_ |= 0x00000100;
custom_ = value;
onChanged();
return this;
}
/**
* optional bool custom = 9;
*/
public Builder clearCustom() {
bitField0_ = (bitField0_ & ~0x00000100);
custom_ = false;
onChanged();
return this;
}
private int decimalScale_ ;
/**
* optional int32 decimalScale = 10;
*/
public boolean hasDecimalScale() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 decimalScale = 10;
*/
public int getDecimalScale() {
return decimalScale_;
}
/**
* optional int32 decimalScale = 10;
*/
public Builder setDecimalScale(int value) {
bitField0_ |= 0x00000200;
decimalScale_ = value;
onChanged();
return this;
}
/**
* optional int32 decimalScale = 10;
*/
public Builder clearDecimalScale() {
bitField0_ = (bitField0_ & ~0x00000200);
decimalScale_ = 0;
onChanged();
return this;
}
private java.lang.Object bestValue_ = "";
/**
* optional string bestValue = 11;
*/
public boolean hasBestValue() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string bestValue = 11;
*/
public java.lang.String getBestValue() {
java.lang.Object ref = bestValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
bestValue_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string bestValue = 11;
*/
public com.google.protobuf.ByteString
getBestValueBytes() {
java.lang.Object ref = bestValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bestValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string bestValue = 11;
*/
public Builder setBestValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
bestValue_ = value;
onChanged();
return this;
}
/**
* optional string bestValue = 11;
*/
public Builder clearBestValue() {
bitField0_ = (bitField0_ & ~0x00000400);
bestValue_ = getDefaultInstance().getBestValue();
onChanged();
return this;
}
/**
* optional string bestValue = 11;
*/
public Builder setBestValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
bestValue_ = value;
onChanged();
return this;
}
private java.lang.Object worstValue_ = "";
/**
* optional string worstValue = 12;
*/
public boolean hasWorstValue() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string worstValue = 12;
*/
public java.lang.String getWorstValue() {
java.lang.Object ref = worstValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
worstValue_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string worstValue = 12;
*/
public com.google.protobuf.ByteString
getWorstValueBytes() {
java.lang.Object ref = worstValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
worstValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string worstValue = 12;
*/
public Builder setWorstValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
worstValue_ = value;
onChanged();
return this;
}
/**
* optional string worstValue = 12;
*/
public Builder clearWorstValue() {
bitField0_ = (bitField0_ & ~0x00000800);
worstValue_ = getDefaultInstance().getWorstValue();
onChanged();
return this;
}
/**
* optional string worstValue = 12;
*/
public Builder setWorstValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
worstValue_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.commons.Metric)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.commons.Metric)
private static final org.sonarqube.ws.Common.Metric DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.Common.Metric();
}
public static org.sonarqube.ws.Common.Metric getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Metric parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Metric(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.Common.Metric getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Paging_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Paging_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Facet_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Facet_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Facets_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Facets_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_FacetValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_FacetValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Rule_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Rule_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Rules_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Rules_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_User_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_User_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Users_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Users_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_TextRange_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_TextRange_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_commons_Metric_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_commons_Metric_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\020ws-commons.proto\022\024sonarqube.ws.commons" +
"\"<\n\006Paging\022\021\n\tpageIndex\030\001 \001(\005\022\020\n\010pageSiz" +
"e\030\002 \001(\005\022\r\n\005total\030\003 \001(\005\"K\n\005Facet\022\020\n\010prope" +
"rty\030\001 \001(\t\0220\n\006values\030\002 \003(\0132 .sonarqube.ws" +
".commons.FacetValue\"5\n\006Facets\022+\n\006facets\030" +
"\001 \003(\0132\033.sonarqube.ws.commons.Facet\"(\n\nFa" +
"cetValue\022\013\n\003val\030\001 \001(\t\022\r\n\005count\030\002 \001(\003\"s\n\004" +
"Rule\022\013\n\003key\030\001 \001(\t\022\014\n\004name\030\002 \001(\t\022\014\n\004lang\030" +
"\003 \001(\t\0220\n\006status\030\004 \001(\0162 .sonarqube.ws.com" +
"mons.RuleStatus\022\020\n\010langName\030\005 \001(\t\"2\n\005Rul",
"es\022)\n\005rules\030\001 \003(\0132\032.sonarqube.ws.commons" +
".Rule\"B\n\004User\022\r\n\005login\030\001 \001(\t\022\014\n\004name\030\002 \001" +
"(\t\022\r\n\005email\030\003 \001(\t\022\016\n\006active\030\004 \001(\010\"2\n\005Use" +
"rs\022)\n\005users\030\001 \003(\0132\032.sonarqube.ws.commons" +
".User\"W\n\tTextRange\022\021\n\tstartLine\030\001 \001(\005\022\017\n" +
"\007endLine\030\002 \001(\005\022\023\n\013startOffset\030\003 \001(\005\022\021\n\te" +
"ndOffset\030\004 \001(\005\"\347\001\n\006Metric\022\013\n\003key\030\001 \001(\t\022\014" +
"\n\004name\030\002 \001(\t\022\023\n\013description\030\003 \001(\t\022\016\n\006dom" +
"ain\030\004 \001(\t\022\014\n\004type\030\005 \001(\t\022\035\n\025higherValuesA" +
"reBetter\030\006 \001(\010\022\023\n\013qualitative\030\007 \001(\010\022\016\n\006h",
"idden\030\010 \001(\010\022\016\n\006custom\030\t \001(\010\022\024\n\014decimalSc" +
"ale\030\n \001(\005\022\021\n\tbestValue\030\013 \001(\t\022\022\n\nworstVal" +
"ue\030\014 \001(\t*E\n\010Severity\022\010\n\004INFO\020\000\022\t\n\005MINOR\020" +
"\001\022\t\n\005MAJOR\020\002\022\014\n\010CRITICAL\020\003\022\013\n\007BLOCKER\020\004*" +
">\n\nRuleStatus\022\010\n\004BETA\020\000\022\016\n\nDEPRECATED\020\001\022" +
"\t\n\005READY\020\002\022\013\n\007REMOVED\020\003*C\n\010RuleType\022\013\n\007U" +
"NKNOWN\020\000\022\016\n\nCODE_SMELL\020\001\022\007\n\003BUG\020\002\022\021\n\rVUL" +
"NERABILITY\020\003B\034\n\020org.sonarqube.wsB\006Common" +
"H\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_sonarqube_ws_commons_Paging_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sonarqube_ws_commons_Paging_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Paging_descriptor,
new java.lang.String[] { "PageIndex", "PageSize", "Total", });
internal_static_sonarqube_ws_commons_Facet_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sonarqube_ws_commons_Facet_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Facet_descriptor,
new java.lang.String[] { "Property", "Values", });
internal_static_sonarqube_ws_commons_Facets_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sonarqube_ws_commons_Facets_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Facets_descriptor,
new java.lang.String[] { "Facets", });
internal_static_sonarqube_ws_commons_FacetValue_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_sonarqube_ws_commons_FacetValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_FacetValue_descriptor,
new java.lang.String[] { "Val", "Count", });
internal_static_sonarqube_ws_commons_Rule_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_sonarqube_ws_commons_Rule_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Rule_descriptor,
new java.lang.String[] { "Key", "Name", "Lang", "Status", "LangName", });
internal_static_sonarqube_ws_commons_Rules_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_sonarqube_ws_commons_Rules_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Rules_descriptor,
new java.lang.String[] { "Rules", });
internal_static_sonarqube_ws_commons_User_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_sonarqube_ws_commons_User_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_User_descriptor,
new java.lang.String[] { "Login", "Name", "Email", "Active", });
internal_static_sonarqube_ws_commons_Users_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_sonarqube_ws_commons_Users_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Users_descriptor,
new java.lang.String[] { "Users", });
internal_static_sonarqube_ws_commons_TextRange_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_sonarqube_ws_commons_TextRange_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_TextRange_descriptor,
new java.lang.String[] { "StartLine", "EndLine", "StartOffset", "EndOffset", });
internal_static_sonarqube_ws_commons_Metric_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_sonarqube_ws_commons_Metric_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_commons_Metric_descriptor,
new java.lang.String[] { "Key", "Name", "Description", "Domain", "Type", "HigherValuesAreBetter", "Qualitative", "Hidden", "Custom", "DecimalScale", "BestValue", "WorstValue", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy