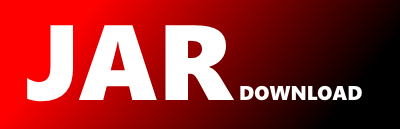
org.sonarqube.ws.WsCe Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-ws Show documentation
Show all versions of sonar-ws Show documentation
Open source platform for continuous inspection of code quality
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ws-ce.proto
package org.sonarqube.ws;
public final class WsCe {
private WsCe() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code sonarqube.ws.ce.TaskStatus}
*/
public enum TaskStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PENDING = 0;
*/
PENDING(0, 0),
/**
* IN_PROGRESS = 1;
*/
IN_PROGRESS(1, 1),
/**
* SUCCESS = 2;
*/
SUCCESS(2, 2),
/**
* FAILED = 3;
*/
FAILED(3, 3),
/**
* CANCELED = 4;
*/
CANCELED(4, 4),
;
/**
* PENDING = 0;
*/
public static final int PENDING_VALUE = 0;
/**
* IN_PROGRESS = 1;
*/
public static final int IN_PROGRESS_VALUE = 1;
/**
* SUCCESS = 2;
*/
public static final int SUCCESS_VALUE = 2;
/**
* FAILED = 3;
*/
public static final int FAILED_VALUE = 3;
/**
* CANCELED = 4;
*/
public static final int CANCELED_VALUE = 4;
public final int getNumber() {
return value;
}
public static TaskStatus valueOf(int value) {
switch (value) {
case 0: return PENDING;
case 1: return IN_PROGRESS;
case 2: return SUCCESS;
case 3: return FAILED;
case 4: return CANCELED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TaskStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TaskStatus findValueByNumber(int number) {
return TaskStatus.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.getDescriptor().getEnumTypes().get(0);
}
private static final TaskStatus[] VALUES = values();
public static TaskStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private TaskStatus(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sonarqube.ws.ce.TaskStatus)
}
public interface SubmitResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.SubmitResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional string taskId = 1;
*/
boolean hasTaskId();
/**
* optional string taskId = 1;
*/
java.lang.String getTaskId();
/**
* optional string taskId = 1;
*/
com.google.protobuf.ByteString
getTaskIdBytes();
/**
* optional string projectId = 2;
*/
boolean hasProjectId();
/**
* optional string projectId = 2;
*/
java.lang.String getProjectId();
/**
* optional string projectId = 2;
*/
com.google.protobuf.ByteString
getProjectIdBytes();
}
/**
* Protobuf type {@code sonarqube.ws.ce.SubmitResponse}
*
*
* POST api/ce/submit
*
*/
public static final class SubmitResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.SubmitResponse)
SubmitResponseOrBuilder {
// Use SubmitResponse.newBuilder() to construct.
private SubmitResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private SubmitResponse() {
taskId_ = "";
projectId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubmitResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
taskId_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
projectId_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_SubmitResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_SubmitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.SubmitResponse.class, org.sonarqube.ws.WsCe.SubmitResponse.Builder.class);
}
private int bitField0_;
public static final int TASKID_FIELD_NUMBER = 1;
private volatile java.lang.Object taskId_;
/**
* optional string taskId = 1;
*/
public boolean hasTaskId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string taskId = 1;
*/
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
taskId_ = s;
}
return s;
}
}
/**
* optional string taskId = 1;
*/
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROJECTID_FIELD_NUMBER = 2;
private volatile java.lang.Object projectId_;
/**
* optional string projectId = 2;
*/
public boolean hasProjectId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string projectId = 2;
*/
public java.lang.String getProjectId() {
java.lang.Object ref = projectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
projectId_ = s;
}
return s;
}
}
/**
* optional string projectId = 2;
*/
public com.google.protobuf.ByteString
getProjectIdBytes() {
java.lang.Object ref = projectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, taskId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, projectId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, taskId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, projectId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.SubmitResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.SubmitResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.SubmitResponse}
*
*
* POST api/ce/submit
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.SubmitResponse)
org.sonarqube.ws.WsCe.SubmitResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_SubmitResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_SubmitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.SubmitResponse.class, org.sonarqube.ws.WsCe.SubmitResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.SubmitResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
taskId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
projectId_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_SubmitResponse_descriptor;
}
public org.sonarqube.ws.WsCe.SubmitResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.SubmitResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.SubmitResponse build() {
org.sonarqube.ws.WsCe.SubmitResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.SubmitResponse buildPartial() {
org.sonarqube.ws.WsCe.SubmitResponse result = new org.sonarqube.ws.WsCe.SubmitResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.taskId_ = taskId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.projectId_ = projectId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.SubmitResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.SubmitResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.SubmitResponse other) {
if (other == org.sonarqube.ws.WsCe.SubmitResponse.getDefaultInstance()) return this;
if (other.hasTaskId()) {
bitField0_ |= 0x00000001;
taskId_ = other.taskId_;
onChanged();
}
if (other.hasProjectId()) {
bitField0_ |= 0x00000002;
projectId_ = other.projectId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.SubmitResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.SubmitResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object taskId_ = "";
/**
* optional string taskId = 1;
*/
public boolean hasTaskId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string taskId = 1;
*/
public java.lang.String getTaskId() {
java.lang.Object ref = taskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
taskId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string taskId = 1;
*/
public com.google.protobuf.ByteString
getTaskIdBytes() {
java.lang.Object ref = taskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
taskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string taskId = 1;
*/
public Builder setTaskId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
taskId_ = value;
onChanged();
return this;
}
/**
* optional string taskId = 1;
*/
public Builder clearTaskId() {
bitField0_ = (bitField0_ & ~0x00000001);
taskId_ = getDefaultInstance().getTaskId();
onChanged();
return this;
}
/**
* optional string taskId = 1;
*/
public Builder setTaskIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
taskId_ = value;
onChanged();
return this;
}
private java.lang.Object projectId_ = "";
/**
* optional string projectId = 2;
*/
public boolean hasProjectId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string projectId = 2;
*/
public java.lang.String getProjectId() {
java.lang.Object ref = projectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
projectId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string projectId = 2;
*/
public com.google.protobuf.ByteString
getProjectIdBytes() {
java.lang.Object ref = projectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string projectId = 2;
*/
public Builder setProjectId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
projectId_ = value;
onChanged();
return this;
}
/**
* optional string projectId = 2;
*/
public Builder clearProjectId() {
bitField0_ = (bitField0_ & ~0x00000002);
projectId_ = getDefaultInstance().getProjectId();
onChanged();
return this;
}
/**
* optional string projectId = 2;
*/
public Builder setProjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
projectId_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.SubmitResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.SubmitResponse)
private static final org.sonarqube.ws.WsCe.SubmitResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.SubmitResponse();
}
public static org.sonarqube.ws.WsCe.SubmitResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SubmitResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new SubmitResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.SubmitResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.TaskResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
boolean hasTask();
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
org.sonarqube.ws.WsCe.Task getTask();
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
org.sonarqube.ws.WsCe.TaskOrBuilder getTaskOrBuilder();
}
/**
* Protobuf type {@code sonarqube.ws.ce.TaskResponse}
*
*
* GET api/ce/task
*
*/
public static final class TaskResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.TaskResponse)
TaskResponseOrBuilder {
// Use TaskResponse.newBuilder() to construct.
private TaskResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TaskResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.sonarqube.ws.WsCe.Task.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = task_.toBuilder();
}
task_ = input.readMessage(org.sonarqube.ws.WsCe.Task.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(task_);
task_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.TaskResponse.class, org.sonarqube.ws.WsCe.TaskResponse.Builder.class);
}
private int bitField0_;
public static final int TASK_FIELD_NUMBER = 1;
private org.sonarqube.ws.WsCe.Task task_;
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public boolean hasTask() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public org.sonarqube.ws.WsCe.Task getTask() {
return task_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : task_;
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getTaskOrBuilder() {
return task_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : task_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getTask());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTask());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.TaskResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.TaskResponse}
*
*
* GET api/ce/task
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.TaskResponse)
org.sonarqube.ws.WsCe.TaskResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.TaskResponse.class, org.sonarqube.ws.WsCe.TaskResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.TaskResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTaskFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (taskBuilder_ == null) {
task_ = null;
} else {
taskBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskResponse_descriptor;
}
public org.sonarqube.ws.WsCe.TaskResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.TaskResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.TaskResponse build() {
org.sonarqube.ws.WsCe.TaskResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.TaskResponse buildPartial() {
org.sonarqube.ws.WsCe.TaskResponse result = new org.sonarqube.ws.WsCe.TaskResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (taskBuilder_ == null) {
result.task_ = task_;
} else {
result.task_ = taskBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.TaskResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.TaskResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.TaskResponse other) {
if (other == org.sonarqube.ws.WsCe.TaskResponse.getDefaultInstance()) return this;
if (other.hasTask()) {
mergeTask(other.getTask());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.TaskResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.TaskResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.sonarqube.ws.WsCe.Task task_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder> taskBuilder_;
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public boolean hasTask() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public org.sonarqube.ws.WsCe.Task getTask() {
if (taskBuilder_ == null) {
return task_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : task_;
} else {
return taskBuilder_.getMessage();
}
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public Builder setTask(org.sonarqube.ws.WsCe.Task value) {
if (taskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
task_ = value;
onChanged();
} else {
taskBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public Builder setTask(
org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (taskBuilder_ == null) {
task_ = builderForValue.build();
onChanged();
} else {
taskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public Builder mergeTask(org.sonarqube.ws.WsCe.Task value) {
if (taskBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
task_ != null &&
task_ != org.sonarqube.ws.WsCe.Task.getDefaultInstance()) {
task_ =
org.sonarqube.ws.WsCe.Task.newBuilder(task_).mergeFrom(value).buildPartial();
} else {
task_ = value;
}
onChanged();
} else {
taskBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public Builder clearTask() {
if (taskBuilder_ == null) {
task_ = null;
onChanged();
} else {
taskBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public org.sonarqube.ws.WsCe.Task.Builder getTaskBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTaskFieldBuilder().getBuilder();
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getTaskOrBuilder() {
if (taskBuilder_ != null) {
return taskBuilder_.getMessageOrBuilder();
} else {
return task_ == null ?
org.sonarqube.ws.WsCe.Task.getDefaultInstance() : task_;
}
}
/**
* optional .sonarqube.ws.ce.Task task = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>
getTaskFieldBuilder() {
if (taskBuilder_ == null) {
taskBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>(
getTask(),
getParentForChildren(),
isClean());
task_ = null;
}
return taskBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.TaskResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.TaskResponse)
private static final org.sonarqube.ws.WsCe.TaskResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.TaskResponse();
}
public static org.sonarqube.ws.WsCe.TaskResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TaskResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new TaskResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.TaskResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ActivityResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.ActivityResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
boolean hasUnusedPaging();
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
org.sonarqube.ws.Common.Paging getUnusedPaging();
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
org.sonarqube.ws.Common.PagingOrBuilder getUnusedPagingOrBuilder();
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
java.util.List
getTasksList();
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
org.sonarqube.ws.WsCe.Task getTasks(int index);
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
int getTasksCount();
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getTasksOrBuilderList();
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
org.sonarqube.ws.WsCe.TaskOrBuilder getTasksOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.ce.ActivityResponse}
*
*
* GET api/ce/activity
*
*/
public static final class ActivityResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.ActivityResponse)
ActivityResponseOrBuilder {
// Use ActivityResponse.newBuilder() to construct.
private ActivityResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ActivityResponse() {
tasks_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ActivityResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.sonarqube.ws.Common.Paging.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = unusedPaging_.toBuilder();
}
unusedPaging_ = input.readMessage(org.sonarqube.ws.Common.Paging.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unusedPaging_);
unusedPaging_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
tasks_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
tasks_.add(input.readMessage(org.sonarqube.ws.WsCe.Task.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
tasks_ = java.util.Collections.unmodifiableList(tasks_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ActivityResponse.class, org.sonarqube.ws.WsCe.ActivityResponse.Builder.class);
}
private int bitField0_;
public static final int UNUSEDPAGING_FIELD_NUMBER = 1;
private org.sonarqube.ws.Common.Paging unusedPaging_;
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public boolean hasUnusedPaging() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public org.sonarqube.ws.Common.Paging getUnusedPaging() {
return unusedPaging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : unusedPaging_;
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public org.sonarqube.ws.Common.PagingOrBuilder getUnusedPagingOrBuilder() {
return unusedPaging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : unusedPaging_;
}
public static final int TASKS_FIELD_NUMBER = 2;
private java.util.List tasks_;
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public java.util.List getTasksList() {
return tasks_;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getTasksOrBuilderList() {
return tasks_;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public int getTasksCount() {
return tasks_.size();
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.Task getTasks(int index) {
return tasks_.get(index);
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getTasksOrBuilder(
int index) {
return tasks_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getUnusedPaging());
}
for (int i = 0; i < tasks_.size(); i++) {
output.writeMessage(2, tasks_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getUnusedPaging());
}
for (int i = 0; i < tasks_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, tasks_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.ActivityResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.ActivityResponse}
*
*
* GET api/ce/activity
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.ActivityResponse)
org.sonarqube.ws.WsCe.ActivityResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ActivityResponse.class, org.sonarqube.ws.WsCe.ActivityResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.ActivityResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getUnusedPagingFieldBuilder();
getTasksFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (unusedPagingBuilder_ == null) {
unusedPaging_ = null;
} else {
unusedPagingBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (tasksBuilder_ == null) {
tasks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
tasksBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityResponse_descriptor;
}
public org.sonarqube.ws.WsCe.ActivityResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.ActivityResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.ActivityResponse build() {
org.sonarqube.ws.WsCe.ActivityResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.ActivityResponse buildPartial() {
org.sonarqube.ws.WsCe.ActivityResponse result = new org.sonarqube.ws.WsCe.ActivityResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (unusedPagingBuilder_ == null) {
result.unusedPaging_ = unusedPaging_;
} else {
result.unusedPaging_ = unusedPagingBuilder_.build();
}
if (tasksBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
tasks_ = java.util.Collections.unmodifiableList(tasks_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.tasks_ = tasks_;
} else {
result.tasks_ = tasksBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.ActivityResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.ActivityResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.ActivityResponse other) {
if (other == org.sonarqube.ws.WsCe.ActivityResponse.getDefaultInstance()) return this;
if (other.hasUnusedPaging()) {
mergeUnusedPaging(other.getUnusedPaging());
}
if (tasksBuilder_ == null) {
if (!other.tasks_.isEmpty()) {
if (tasks_.isEmpty()) {
tasks_ = other.tasks_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTasksIsMutable();
tasks_.addAll(other.tasks_);
}
onChanged();
}
} else {
if (!other.tasks_.isEmpty()) {
if (tasksBuilder_.isEmpty()) {
tasksBuilder_.dispose();
tasksBuilder_ = null;
tasks_ = other.tasks_;
bitField0_ = (bitField0_ & ~0x00000002);
tasksBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getTasksFieldBuilder() : null;
} else {
tasksBuilder_.addAllMessages(other.tasks_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.ActivityResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.ActivityResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.sonarqube.ws.Common.Paging unusedPaging_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder> unusedPagingBuilder_;
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public boolean hasUnusedPaging() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public org.sonarqube.ws.Common.Paging getUnusedPaging() {
if (unusedPagingBuilder_ == null) {
return unusedPaging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : unusedPaging_;
} else {
return unusedPagingBuilder_.getMessage();
}
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public Builder setUnusedPaging(org.sonarqube.ws.Common.Paging value) {
if (unusedPagingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unusedPaging_ = value;
onChanged();
} else {
unusedPagingBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public Builder setUnusedPaging(
org.sonarqube.ws.Common.Paging.Builder builderForValue) {
if (unusedPagingBuilder_ == null) {
unusedPaging_ = builderForValue.build();
onChanged();
} else {
unusedPagingBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public Builder mergeUnusedPaging(org.sonarqube.ws.Common.Paging value) {
if (unusedPagingBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
unusedPaging_ != null &&
unusedPaging_ != org.sonarqube.ws.Common.Paging.getDefaultInstance()) {
unusedPaging_ =
org.sonarqube.ws.Common.Paging.newBuilder(unusedPaging_).mergeFrom(value).buildPartial();
} else {
unusedPaging_ = value;
}
onChanged();
} else {
unusedPagingBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public Builder clearUnusedPaging() {
if (unusedPagingBuilder_ == null) {
unusedPaging_ = null;
onChanged();
} else {
unusedPagingBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public org.sonarqube.ws.Common.Paging.Builder getUnusedPagingBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getUnusedPagingFieldBuilder().getBuilder();
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
public org.sonarqube.ws.Common.PagingOrBuilder getUnusedPagingOrBuilder() {
if (unusedPagingBuilder_ != null) {
return unusedPagingBuilder_.getMessageOrBuilder();
} else {
return unusedPaging_ == null ?
org.sonarqube.ws.Common.Paging.getDefaultInstance() : unusedPaging_;
}
}
/**
* optional .sonarqube.ws.commons.Paging unusedPaging = 1;
*
*
* paging has been deprecated in 5.5
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder>
getUnusedPagingFieldBuilder() {
if (unusedPagingBuilder_ == null) {
unusedPagingBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder>(
getUnusedPaging(),
getParentForChildren(),
isClean());
unusedPaging_ = null;
}
return unusedPagingBuilder_;
}
private java.util.List tasks_ =
java.util.Collections.emptyList();
private void ensureTasksIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
tasks_ = new java.util.ArrayList(tasks_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder> tasksBuilder_;
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public java.util.List getTasksList() {
if (tasksBuilder_ == null) {
return java.util.Collections.unmodifiableList(tasks_);
} else {
return tasksBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public int getTasksCount() {
if (tasksBuilder_ == null) {
return tasks_.size();
} else {
return tasksBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.Task getTasks(int index) {
if (tasksBuilder_ == null) {
return tasks_.get(index);
} else {
return tasksBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder setTasks(
int index, org.sonarqube.ws.WsCe.Task value) {
if (tasksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTasksIsMutable();
tasks_.set(index, value);
onChanged();
} else {
tasksBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder setTasks(
int index, org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (tasksBuilder_ == null) {
ensureTasksIsMutable();
tasks_.set(index, builderForValue.build());
onChanged();
} else {
tasksBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder addTasks(org.sonarqube.ws.WsCe.Task value) {
if (tasksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTasksIsMutable();
tasks_.add(value);
onChanged();
} else {
tasksBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder addTasks(
int index, org.sonarqube.ws.WsCe.Task value) {
if (tasksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTasksIsMutable();
tasks_.add(index, value);
onChanged();
} else {
tasksBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder addTasks(
org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (tasksBuilder_ == null) {
ensureTasksIsMutable();
tasks_.add(builderForValue.build());
onChanged();
} else {
tasksBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder addTasks(
int index, org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (tasksBuilder_ == null) {
ensureTasksIsMutable();
tasks_.add(index, builderForValue.build());
onChanged();
} else {
tasksBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder addAllTasks(
java.lang.Iterable extends org.sonarqube.ws.WsCe.Task> values) {
if (tasksBuilder_ == null) {
ensureTasksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tasks_);
onChanged();
} else {
tasksBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder clearTasks() {
if (tasksBuilder_ == null) {
tasks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
tasksBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public Builder removeTasks(int index) {
if (tasksBuilder_ == null) {
ensureTasksIsMutable();
tasks_.remove(index);
onChanged();
} else {
tasksBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.Task.Builder getTasksBuilder(
int index) {
return getTasksFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getTasksOrBuilder(
int index) {
if (tasksBuilder_ == null) {
return tasks_.get(index); } else {
return tasksBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getTasksOrBuilderList() {
if (tasksBuilder_ != null) {
return tasksBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tasks_);
}
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.Task.Builder addTasksBuilder() {
return getTasksFieldBuilder().addBuilder(
org.sonarqube.ws.WsCe.Task.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public org.sonarqube.ws.WsCe.Task.Builder addTasksBuilder(
int index) {
return getTasksFieldBuilder().addBuilder(
index, org.sonarqube.ws.WsCe.Task.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.ce.Task tasks = 2;
*/
public java.util.List
getTasksBuilderList() {
return getTasksFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>
getTasksFieldBuilder() {
if (tasksBuilder_ == null) {
tasksBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>(
tasks_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
tasks_ = null;
}
return tasksBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.ActivityResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.ActivityResponse)
private static final org.sonarqube.ws.WsCe.ActivityResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.ActivityResponse();
}
public static org.sonarqube.ws.WsCe.ActivityResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ActivityResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ActivityResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.ActivityResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ActivityStatusWsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.ActivityStatusWsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 pending = 1;
*/
boolean hasPending();
/**
* optional int32 pending = 1;
*/
int getPending();
/**
* optional int32 failing = 2;
*/
boolean hasFailing();
/**
* optional int32 failing = 2;
*/
int getFailing();
}
/**
* Protobuf type {@code sonarqube.ws.ce.ActivityStatusWsResponse}
*
*
* GET api/ce/activity_status
*
*/
public static final class ActivityStatusWsResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.ActivityStatusWsResponse)
ActivityStatusWsResponseOrBuilder {
// Use ActivityStatusWsResponse.newBuilder() to construct.
private ActivityStatusWsResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ActivityStatusWsResponse() {
pending_ = 0;
failing_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ActivityStatusWsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
pending_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
failing_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ActivityStatusWsResponse.class, org.sonarqube.ws.WsCe.ActivityStatusWsResponse.Builder.class);
}
private int bitField0_;
public static final int PENDING_FIELD_NUMBER = 1;
private int pending_;
/**
* optional int32 pending = 1;
*/
public boolean hasPending() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 pending = 1;
*/
public int getPending() {
return pending_;
}
public static final int FAILING_FIELD_NUMBER = 2;
private int failing_;
/**
* optional int32 failing = 2;
*/
public boolean hasFailing() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 failing = 2;
*/
public int getFailing() {
return failing_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, pending_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, failing_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, pending_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, failing_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.ActivityStatusWsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.ActivityStatusWsResponse}
*
*
* GET api/ce/activity_status
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.ActivityStatusWsResponse)
org.sonarqube.ws.WsCe.ActivityStatusWsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ActivityStatusWsResponse.class, org.sonarqube.ws.WsCe.ActivityStatusWsResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.ActivityStatusWsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
pending_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
failing_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor;
}
public org.sonarqube.ws.WsCe.ActivityStatusWsResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.ActivityStatusWsResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.ActivityStatusWsResponse build() {
org.sonarqube.ws.WsCe.ActivityStatusWsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.ActivityStatusWsResponse buildPartial() {
org.sonarqube.ws.WsCe.ActivityStatusWsResponse result = new org.sonarqube.ws.WsCe.ActivityStatusWsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pending_ = pending_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.failing_ = failing_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.ActivityStatusWsResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.ActivityStatusWsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.ActivityStatusWsResponse other) {
if (other == org.sonarqube.ws.WsCe.ActivityStatusWsResponse.getDefaultInstance()) return this;
if (other.hasPending()) {
setPending(other.getPending());
}
if (other.hasFailing()) {
setFailing(other.getFailing());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.ActivityStatusWsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.ActivityStatusWsResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int pending_ ;
/**
* optional int32 pending = 1;
*/
public boolean hasPending() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 pending = 1;
*/
public int getPending() {
return pending_;
}
/**
* optional int32 pending = 1;
*/
public Builder setPending(int value) {
bitField0_ |= 0x00000001;
pending_ = value;
onChanged();
return this;
}
/**
* optional int32 pending = 1;
*/
public Builder clearPending() {
bitField0_ = (bitField0_ & ~0x00000001);
pending_ = 0;
onChanged();
return this;
}
private int failing_ ;
/**
* optional int32 failing = 2;
*/
public boolean hasFailing() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 failing = 2;
*/
public int getFailing() {
return failing_;
}
/**
* optional int32 failing = 2;
*/
public Builder setFailing(int value) {
bitField0_ |= 0x00000002;
failing_ = value;
onChanged();
return this;
}
/**
* optional int32 failing = 2;
*/
public Builder clearFailing() {
bitField0_ = (bitField0_ & ~0x00000002);
failing_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.ActivityStatusWsResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.ActivityStatusWsResponse)
private static final org.sonarqube.ws.WsCe.ActivityStatusWsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.ActivityStatusWsResponse();
}
public static org.sonarqube.ws.WsCe.ActivityStatusWsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ActivityStatusWsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ActivityStatusWsResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.ActivityStatusWsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProjectResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.ProjectResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
java.util.List
getQueueList();
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
org.sonarqube.ws.WsCe.Task getQueue(int index);
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
int getQueueCount();
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getQueueOrBuilderList();
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
org.sonarqube.ws.WsCe.TaskOrBuilder getQueueOrBuilder(
int index);
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
boolean hasCurrent();
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
org.sonarqube.ws.WsCe.Task getCurrent();
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
org.sonarqube.ws.WsCe.TaskOrBuilder getCurrentOrBuilder();
}
/**
* Protobuf type {@code sonarqube.ws.ce.ProjectResponse}
*
*
* GET api/ce/project
*
*/
public static final class ProjectResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.ProjectResponse)
ProjectResponseOrBuilder {
// Use ProjectResponse.newBuilder() to construct.
private ProjectResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProjectResponse() {
queue_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProjectResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
queue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
queue_.add(input.readMessage(org.sonarqube.ws.WsCe.Task.parser(), extensionRegistry));
break;
}
case 18: {
org.sonarqube.ws.WsCe.Task.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = current_.toBuilder();
}
current_ = input.readMessage(org.sonarqube.ws.WsCe.Task.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(current_);
current_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
queue_ = java.util.Collections.unmodifiableList(queue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ProjectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ProjectResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ProjectResponse.class, org.sonarqube.ws.WsCe.ProjectResponse.Builder.class);
}
private int bitField0_;
public static final int QUEUE_FIELD_NUMBER = 1;
private java.util.List queue_;
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public java.util.List getQueueList() {
return queue_;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getQueueOrBuilderList() {
return queue_;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public int getQueueCount() {
return queue_.size();
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.Task getQueue(int index) {
return queue_.get(index);
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getQueueOrBuilder(
int index) {
return queue_.get(index);
}
public static final int CURRENT_FIELD_NUMBER = 2;
private org.sonarqube.ws.WsCe.Task current_;
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public boolean hasCurrent() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public org.sonarqube.ws.WsCe.Task getCurrent() {
return current_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : current_;
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getCurrentOrBuilder() {
return current_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : current_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < queue_.size(); i++) {
output.writeMessage(1, queue_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(2, getCurrent());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < queue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, queue_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCurrent());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.ProjectResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.ProjectResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.ProjectResponse}
*
*
* GET api/ce/project
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.ProjectResponse)
org.sonarqube.ws.WsCe.ProjectResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ProjectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ProjectResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.ProjectResponse.class, org.sonarqube.ws.WsCe.ProjectResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.ProjectResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getQueueFieldBuilder();
getCurrentFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (queueBuilder_ == null) {
queue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
queueBuilder_.clear();
}
if (currentBuilder_ == null) {
current_ = null;
} else {
currentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_ProjectResponse_descriptor;
}
public org.sonarqube.ws.WsCe.ProjectResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.ProjectResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.ProjectResponse build() {
org.sonarqube.ws.WsCe.ProjectResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.ProjectResponse buildPartial() {
org.sonarqube.ws.WsCe.ProjectResponse result = new org.sonarqube.ws.WsCe.ProjectResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (queueBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
queue_ = java.util.Collections.unmodifiableList(queue_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.queue_ = queue_;
} else {
result.queue_ = queueBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
if (currentBuilder_ == null) {
result.current_ = current_;
} else {
result.current_ = currentBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.ProjectResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.ProjectResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.ProjectResponse other) {
if (other == org.sonarqube.ws.WsCe.ProjectResponse.getDefaultInstance()) return this;
if (queueBuilder_ == null) {
if (!other.queue_.isEmpty()) {
if (queue_.isEmpty()) {
queue_ = other.queue_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureQueueIsMutable();
queue_.addAll(other.queue_);
}
onChanged();
}
} else {
if (!other.queue_.isEmpty()) {
if (queueBuilder_.isEmpty()) {
queueBuilder_.dispose();
queueBuilder_ = null;
queue_ = other.queue_;
bitField0_ = (bitField0_ & ~0x00000001);
queueBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getQueueFieldBuilder() : null;
} else {
queueBuilder_.addAllMessages(other.queue_);
}
}
}
if (other.hasCurrent()) {
mergeCurrent(other.getCurrent());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.ProjectResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.ProjectResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List queue_ =
java.util.Collections.emptyList();
private void ensureQueueIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
queue_ = new java.util.ArrayList(queue_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder> queueBuilder_;
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public java.util.List getQueueList() {
if (queueBuilder_ == null) {
return java.util.Collections.unmodifiableList(queue_);
} else {
return queueBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public int getQueueCount() {
if (queueBuilder_ == null) {
return queue_.size();
} else {
return queueBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.Task getQueue(int index) {
if (queueBuilder_ == null) {
return queue_.get(index);
} else {
return queueBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder setQueue(
int index, org.sonarqube.ws.WsCe.Task value) {
if (queueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueueIsMutable();
queue_.set(index, value);
onChanged();
} else {
queueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder setQueue(
int index, org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (queueBuilder_ == null) {
ensureQueueIsMutable();
queue_.set(index, builderForValue.build());
onChanged();
} else {
queueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder addQueue(org.sonarqube.ws.WsCe.Task value) {
if (queueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueueIsMutable();
queue_.add(value);
onChanged();
} else {
queueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder addQueue(
int index, org.sonarqube.ws.WsCe.Task value) {
if (queueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueueIsMutable();
queue_.add(index, value);
onChanged();
} else {
queueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder addQueue(
org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (queueBuilder_ == null) {
ensureQueueIsMutable();
queue_.add(builderForValue.build());
onChanged();
} else {
queueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder addQueue(
int index, org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (queueBuilder_ == null) {
ensureQueueIsMutable();
queue_.add(index, builderForValue.build());
onChanged();
} else {
queueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder addAllQueue(
java.lang.Iterable extends org.sonarqube.ws.WsCe.Task> values) {
if (queueBuilder_ == null) {
ensureQueueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, queue_);
onChanged();
} else {
queueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder clearQueue() {
if (queueBuilder_ == null) {
queue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
queueBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public Builder removeQueue(int index) {
if (queueBuilder_ == null) {
ensureQueueIsMutable();
queue_.remove(index);
onChanged();
} else {
queueBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.Task.Builder getQueueBuilder(
int index) {
return getQueueFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getQueueOrBuilder(
int index) {
if (queueBuilder_ == null) {
return queue_.get(index); } else {
return queueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public java.util.List extends org.sonarqube.ws.WsCe.TaskOrBuilder>
getQueueOrBuilderList() {
if (queueBuilder_ != null) {
return queueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(queue_);
}
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.Task.Builder addQueueBuilder() {
return getQueueFieldBuilder().addBuilder(
org.sonarqube.ws.WsCe.Task.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public org.sonarqube.ws.WsCe.Task.Builder addQueueBuilder(
int index) {
return getQueueFieldBuilder().addBuilder(
index, org.sonarqube.ws.WsCe.Task.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.ce.Task queue = 1;
*/
public java.util.List
getQueueBuilderList() {
return getQueueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>
getQueueFieldBuilder() {
if (queueBuilder_ == null) {
queueBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>(
queue_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
queue_ = null;
}
return queueBuilder_;
}
private org.sonarqube.ws.WsCe.Task current_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder> currentBuilder_;
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public boolean hasCurrent() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public org.sonarqube.ws.WsCe.Task getCurrent() {
if (currentBuilder_ == null) {
return current_ == null ? org.sonarqube.ws.WsCe.Task.getDefaultInstance() : current_;
} else {
return currentBuilder_.getMessage();
}
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public Builder setCurrent(org.sonarqube.ws.WsCe.Task value) {
if (currentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
current_ = value;
onChanged();
} else {
currentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public Builder setCurrent(
org.sonarqube.ws.WsCe.Task.Builder builderForValue) {
if (currentBuilder_ == null) {
current_ = builderForValue.build();
onChanged();
} else {
currentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public Builder mergeCurrent(org.sonarqube.ws.WsCe.Task value) {
if (currentBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
current_ != null &&
current_ != org.sonarqube.ws.WsCe.Task.getDefaultInstance()) {
current_ =
org.sonarqube.ws.WsCe.Task.newBuilder(current_).mergeFrom(value).buildPartial();
} else {
current_ = value;
}
onChanged();
} else {
currentBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public Builder clearCurrent() {
if (currentBuilder_ == null) {
current_ = null;
onChanged();
} else {
currentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public org.sonarqube.ws.WsCe.Task.Builder getCurrentBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCurrentFieldBuilder().getBuilder();
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
public org.sonarqube.ws.WsCe.TaskOrBuilder getCurrentOrBuilder() {
if (currentBuilder_ != null) {
return currentBuilder_.getMessageOrBuilder();
} else {
return current_ == null ?
org.sonarqube.ws.WsCe.Task.getDefaultInstance() : current_;
}
}
/**
* optional .sonarqube.ws.ce.Task current = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>
getCurrentFieldBuilder() {
if (currentBuilder_ == null) {
currentBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.WsCe.Task, org.sonarqube.ws.WsCe.Task.Builder, org.sonarqube.ws.WsCe.TaskOrBuilder>(
getCurrent(),
getParentForChildren(),
isClean());
current_ = null;
}
return currentBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.ProjectResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.ProjectResponse)
private static final org.sonarqube.ws.WsCe.ProjectResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.ProjectResponse();
}
public static org.sonarqube.ws.WsCe.ProjectResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ProjectResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ProjectResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.ProjectResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskTypesWsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.TaskTypesWsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string taskTypes = 1;
*/
com.google.protobuf.ProtocolStringList
getTaskTypesList();
/**
* repeated string taskTypes = 1;
*/
int getTaskTypesCount();
/**
* repeated string taskTypes = 1;
*/
java.lang.String getTaskTypes(int index);
/**
* repeated string taskTypes = 1;
*/
com.google.protobuf.ByteString
getTaskTypesBytes(int index);
}
/**
* Protobuf type {@code sonarqube.ws.ce.TaskTypesWsResponse}
*
*
* GET api/ce/task_types
*
*/
public static final class TaskTypesWsResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.TaskTypesWsResponse)
TaskTypesWsResponseOrBuilder {
// Use TaskTypesWsResponse.newBuilder() to construct.
private TaskTypesWsResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TaskTypesWsResponse() {
taskTypes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskTypesWsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
taskTypes_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
taskTypes_.add(bs);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
taskTypes_ = taskTypes_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskTypesWsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.TaskTypesWsResponse.class, org.sonarqube.ws.WsCe.TaskTypesWsResponse.Builder.class);
}
public static final int TASKTYPES_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList taskTypes_;
/**
* repeated string taskTypes = 1;
*/
public com.google.protobuf.ProtocolStringList
getTaskTypesList() {
return taskTypes_;
}
/**
* repeated string taskTypes = 1;
*/
public int getTaskTypesCount() {
return taskTypes_.size();
}
/**
* repeated string taskTypes = 1;
*/
public java.lang.String getTaskTypes(int index) {
return taskTypes_.get(index);
}
/**
* repeated string taskTypes = 1;
*/
public com.google.protobuf.ByteString
getTaskTypesBytes(int index) {
return taskTypes_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < taskTypes_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, taskTypes_.getRaw(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < taskTypes_.size(); i++) {
dataSize += computeStringSizeNoTag(taskTypes_.getRaw(i));
}
size += dataSize;
size += 1 * getTaskTypesList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.TaskTypesWsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.TaskTypesWsResponse}
*
*
* GET api/ce/task_types
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.TaskTypesWsResponse)
org.sonarqube.ws.WsCe.TaskTypesWsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskTypesWsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.TaskTypesWsResponse.class, org.sonarqube.ws.WsCe.TaskTypesWsResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.TaskTypesWsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
taskTypes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor;
}
public org.sonarqube.ws.WsCe.TaskTypesWsResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.TaskTypesWsResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.TaskTypesWsResponse build() {
org.sonarqube.ws.WsCe.TaskTypesWsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.TaskTypesWsResponse buildPartial() {
org.sonarqube.ws.WsCe.TaskTypesWsResponse result = new org.sonarqube.ws.WsCe.TaskTypesWsResponse(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
taskTypes_ = taskTypes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.taskTypes_ = taskTypes_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.TaskTypesWsResponse) {
return mergeFrom((org.sonarqube.ws.WsCe.TaskTypesWsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.TaskTypesWsResponse other) {
if (other == org.sonarqube.ws.WsCe.TaskTypesWsResponse.getDefaultInstance()) return this;
if (!other.taskTypes_.isEmpty()) {
if (taskTypes_.isEmpty()) {
taskTypes_ = other.taskTypes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTaskTypesIsMutable();
taskTypes_.addAll(other.taskTypes_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.TaskTypesWsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.TaskTypesWsResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList taskTypes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTaskTypesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
taskTypes_ = new com.google.protobuf.LazyStringArrayList(taskTypes_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string taskTypes = 1;
*/
public com.google.protobuf.ProtocolStringList
getTaskTypesList() {
return taskTypes_.getUnmodifiableView();
}
/**
* repeated string taskTypes = 1;
*/
public int getTaskTypesCount() {
return taskTypes_.size();
}
/**
* repeated string taskTypes = 1;
*/
public java.lang.String getTaskTypes(int index) {
return taskTypes_.get(index);
}
/**
* repeated string taskTypes = 1;
*/
public com.google.protobuf.ByteString
getTaskTypesBytes(int index) {
return taskTypes_.getByteString(index);
}
/**
* repeated string taskTypes = 1;
*/
public Builder setTaskTypes(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTaskTypesIsMutable();
taskTypes_.set(index, value);
onChanged();
return this;
}
/**
* repeated string taskTypes = 1;
*/
public Builder addTaskTypes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTaskTypesIsMutable();
taskTypes_.add(value);
onChanged();
return this;
}
/**
* repeated string taskTypes = 1;
*/
public Builder addAllTaskTypes(
java.lang.Iterable values) {
ensureTaskTypesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, taskTypes_);
onChanged();
return this;
}
/**
* repeated string taskTypes = 1;
*/
public Builder clearTaskTypes() {
taskTypes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string taskTypes = 1;
*/
public Builder addTaskTypesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTaskTypesIsMutable();
taskTypes_.add(value);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.TaskTypesWsResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.TaskTypesWsResponse)
private static final org.sonarqube.ws.WsCe.TaskTypesWsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.TaskTypesWsResponse();
}
public static org.sonarqube.ws.WsCe.TaskTypesWsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TaskTypesWsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new TaskTypesWsResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.TaskTypesWsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.ce.Task)
com.google.protobuf.MessageOrBuilder {
/**
* optional string id = 1;
*/
boolean hasId();
/**
* optional string id = 1;
*/
java.lang.String getId();
/**
* optional string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional string type = 2;
*/
boolean hasType();
/**
* optional string type = 2;
*/
java.lang.String getType();
/**
* optional string type = 2;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* optional string componentId = 3;
*/
boolean hasComponentId();
/**
* optional string componentId = 3;
*/
java.lang.String getComponentId();
/**
* optional string componentId = 3;
*/
com.google.protobuf.ByteString
getComponentIdBytes();
/**
* optional string componentKey = 4;
*/
boolean hasComponentKey();
/**
* optional string componentKey = 4;
*/
java.lang.String getComponentKey();
/**
* optional string componentKey = 4;
*/
com.google.protobuf.ByteString
getComponentKeyBytes();
/**
* optional string componentName = 5;
*/
boolean hasComponentName();
/**
* optional string componentName = 5;
*/
java.lang.String getComponentName();
/**
* optional string componentName = 5;
*/
com.google.protobuf.ByteString
getComponentNameBytes();
/**
* optional string componentQualifier = 6;
*/
boolean hasComponentQualifier();
/**
* optional string componentQualifier = 6;
*/
java.lang.String getComponentQualifier();
/**
* optional string componentQualifier = 6;
*/
com.google.protobuf.ByteString
getComponentQualifierBytes();
/**
* optional string analysisId = 7;
*/
boolean hasAnalysisId();
/**
* optional string analysisId = 7;
*/
java.lang.String getAnalysisId();
/**
* optional string analysisId = 7;
*/
com.google.protobuf.ByteString
getAnalysisIdBytes();
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
boolean hasStatus();
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
org.sonarqube.ws.WsCe.TaskStatus getStatus();
/**
* optional string submittedAt = 9;
*/
boolean hasSubmittedAt();
/**
* optional string submittedAt = 9;
*/
java.lang.String getSubmittedAt();
/**
* optional string submittedAt = 9;
*/
com.google.protobuf.ByteString
getSubmittedAtBytes();
/**
* optional string submitterLogin = 10;
*/
boolean hasSubmitterLogin();
/**
* optional string submitterLogin = 10;
*/
java.lang.String getSubmitterLogin();
/**
* optional string submitterLogin = 10;
*/
com.google.protobuf.ByteString
getSubmitterLoginBytes();
/**
* optional string startedAt = 11;
*/
boolean hasStartedAt();
/**
* optional string startedAt = 11;
*/
java.lang.String getStartedAt();
/**
* optional string startedAt = 11;
*/
com.google.protobuf.ByteString
getStartedAtBytes();
/**
* optional string executedAt = 12;
*/
boolean hasExecutedAt();
/**
* optional string executedAt = 12;
*/
java.lang.String getExecutedAt();
/**
* optional string executedAt = 12;
*/
com.google.protobuf.ByteString
getExecutedAtBytes();
/**
* optional bool isLastExecuted = 13;
*/
boolean hasIsLastExecuted();
/**
* optional bool isLastExecuted = 13;
*/
boolean getIsLastExecuted();
/**
* optional int64 executionTimeMs = 14;
*/
boolean hasExecutionTimeMs();
/**
* optional int64 executionTimeMs = 14;
*/
long getExecutionTimeMs();
/**
* optional bool logs = 15;
*/
boolean hasLogs();
/**
* optional bool logs = 15;
*/
boolean getLogs();
}
/**
* Protobuf type {@code sonarqube.ws.ce.Task}
*/
public static final class Task extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.ce.Task)
TaskOrBuilder {
// Use Task.newBuilder() to construct.
private Task(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Task() {
id_ = "";
type_ = "";
componentId_ = "";
componentKey_ = "";
componentName_ = "";
componentQualifier_ = "";
analysisId_ = "";
status_ = 0;
submittedAt_ = "";
submitterLogin_ = "";
startedAt_ = "";
executedAt_ = "";
isLastExecuted_ = false;
executionTimeMs_ = 0L;
logs_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Task(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
type_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
componentId_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
componentKey_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
componentName_ = bs;
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
componentQualifier_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
analysisId_ = bs;
break;
}
case 64: {
int rawValue = input.readEnum();
org.sonarqube.ws.WsCe.TaskStatus value = org.sonarqube.ws.WsCe.TaskStatus.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(8, rawValue);
} else {
bitField0_ |= 0x00000080;
status_ = rawValue;
}
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
submittedAt_ = bs;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
submitterLogin_ = bs;
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
startedAt_ = bs;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
executedAt_ = bs;
break;
}
case 104: {
bitField0_ |= 0x00001000;
isLastExecuted_ = input.readBool();
break;
}
case 112: {
bitField0_ |= 0x00002000;
executionTimeMs_ = input.readInt64();
break;
}
case 120: {
bitField0_ |= 0x00004000;
logs_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_Task_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.Task.class, org.sonarqube.ws.WsCe.Task.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object type_;
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPONENTID_FIELD_NUMBER = 3;
private volatile java.lang.Object componentId_;
/**
* optional string componentId = 3;
*/
public boolean hasComponentId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string componentId = 3;
*/
public java.lang.String getComponentId() {
java.lang.Object ref = componentId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentId_ = s;
}
return s;
}
}
/**
* optional string componentId = 3;
*/
public com.google.protobuf.ByteString
getComponentIdBytes() {
java.lang.Object ref = componentId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPONENTKEY_FIELD_NUMBER = 4;
private volatile java.lang.Object componentKey_;
/**
* optional string componentKey = 4;
*/
public boolean hasComponentKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string componentKey = 4;
*/
public java.lang.String getComponentKey() {
java.lang.Object ref = componentKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentKey_ = s;
}
return s;
}
}
/**
* optional string componentKey = 4;
*/
public com.google.protobuf.ByteString
getComponentKeyBytes() {
java.lang.Object ref = componentKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPONENTNAME_FIELD_NUMBER = 5;
private volatile java.lang.Object componentName_;
/**
* optional string componentName = 5;
*/
public boolean hasComponentName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string componentName = 5;
*/
public java.lang.String getComponentName() {
java.lang.Object ref = componentName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentName_ = s;
}
return s;
}
}
/**
* optional string componentName = 5;
*/
public com.google.protobuf.ByteString
getComponentNameBytes() {
java.lang.Object ref = componentName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPONENTQUALIFIER_FIELD_NUMBER = 6;
private volatile java.lang.Object componentQualifier_;
/**
* optional string componentQualifier = 6;
*/
public boolean hasComponentQualifier() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string componentQualifier = 6;
*/
public java.lang.String getComponentQualifier() {
java.lang.Object ref = componentQualifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentQualifier_ = s;
}
return s;
}
}
/**
* optional string componentQualifier = 6;
*/
public com.google.protobuf.ByteString
getComponentQualifierBytes() {
java.lang.Object ref = componentQualifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentQualifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ANALYSISID_FIELD_NUMBER = 7;
private volatile java.lang.Object analysisId_;
/**
* optional string analysisId = 7;
*/
public boolean hasAnalysisId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string analysisId = 7;
*/
public java.lang.String getAnalysisId() {
java.lang.Object ref = analysisId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
analysisId_ = s;
}
return s;
}
}
/**
* optional string analysisId = 7;
*/
public com.google.protobuf.ByteString
getAnalysisIdBytes() {
java.lang.Object ref = analysisId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
analysisId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 8;
private int status_;
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public org.sonarqube.ws.WsCe.TaskStatus getStatus() {
org.sonarqube.ws.WsCe.TaskStatus result = org.sonarqube.ws.WsCe.TaskStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.WsCe.TaskStatus.PENDING : result;
}
public static final int SUBMITTEDAT_FIELD_NUMBER = 9;
private volatile java.lang.Object submittedAt_;
/**
* optional string submittedAt = 9;
*/
public boolean hasSubmittedAt() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string submittedAt = 9;
*/
public java.lang.String getSubmittedAt() {
java.lang.Object ref = submittedAt_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
submittedAt_ = s;
}
return s;
}
}
/**
* optional string submittedAt = 9;
*/
public com.google.protobuf.ByteString
getSubmittedAtBytes() {
java.lang.Object ref = submittedAt_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
submittedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBMITTERLOGIN_FIELD_NUMBER = 10;
private volatile java.lang.Object submitterLogin_;
/**
* optional string submitterLogin = 10;
*/
public boolean hasSubmitterLogin() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string submitterLogin = 10;
*/
public java.lang.String getSubmitterLogin() {
java.lang.Object ref = submitterLogin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
submitterLogin_ = s;
}
return s;
}
}
/**
* optional string submitterLogin = 10;
*/
public com.google.protobuf.ByteString
getSubmitterLoginBytes() {
java.lang.Object ref = submitterLogin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
submitterLogin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STARTEDAT_FIELD_NUMBER = 11;
private volatile java.lang.Object startedAt_;
/**
* optional string startedAt = 11;
*/
public boolean hasStartedAt() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string startedAt = 11;
*/
public java.lang.String getStartedAt() {
java.lang.Object ref = startedAt_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
startedAt_ = s;
}
return s;
}
}
/**
* optional string startedAt = 11;
*/
public com.google.protobuf.ByteString
getStartedAtBytes() {
java.lang.Object ref = startedAt_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXECUTEDAT_FIELD_NUMBER = 12;
private volatile java.lang.Object executedAt_;
/**
* optional string executedAt = 12;
*/
public boolean hasExecutedAt() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string executedAt = 12;
*/
public java.lang.String getExecutedAt() {
java.lang.Object ref = executedAt_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
executedAt_ = s;
}
return s;
}
}
/**
* optional string executedAt = 12;
*/
public com.google.protobuf.ByteString
getExecutedAtBytes() {
java.lang.Object ref = executedAt_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISLASTEXECUTED_FIELD_NUMBER = 13;
private boolean isLastExecuted_;
/**
* optional bool isLastExecuted = 13;
*/
public boolean hasIsLastExecuted() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool isLastExecuted = 13;
*/
public boolean getIsLastExecuted() {
return isLastExecuted_;
}
public static final int EXECUTIONTIMEMS_FIELD_NUMBER = 14;
private long executionTimeMs_;
/**
* optional int64 executionTimeMs = 14;
*/
public boolean hasExecutionTimeMs() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional int64 executionTimeMs = 14;
*/
public long getExecutionTimeMs() {
return executionTimeMs_;
}
public static final int LOGS_FIELD_NUMBER = 15;
private boolean logs_;
/**
* optional bool logs = 15;
*/
public boolean hasLogs() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool logs = 15;
*/
public boolean getLogs() {
return logs_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, type_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, componentId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, componentKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, componentName_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, componentQualifier_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, analysisId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeEnum(8, status_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, submittedAt_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, submitterLogin_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessage.writeString(output, 11, startedAt_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
com.google.protobuf.GeneratedMessage.writeString(output, 12, executedAt_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(13, isLastExecuted_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeInt64(14, executionTimeMs_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBool(15, logs_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, type_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, componentId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, componentKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, componentName_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, componentQualifier_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(7, analysisId_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, status_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(9, submittedAt_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, submitterLogin_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(11, startedAt_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(12, executedAt_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, isLastExecuted_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, executionTimeMs_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, logs_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsCe.Task parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.Task parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsCe.Task parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsCe.Task parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsCe.Task prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.ce.Task}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.ce.Task)
org.sonarqube.ws.WsCe.TaskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_Task_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsCe.Task.class, org.sonarqube.ws.WsCe.Task.Builder.class);
}
// Construct using org.sonarqube.ws.WsCe.Task.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
componentId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
componentKey_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
componentName_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
componentQualifier_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
analysisId_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
status_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
submittedAt_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
submitterLogin_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
startedAt_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
executedAt_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
isLastExecuted_ = false;
bitField0_ = (bitField0_ & ~0x00001000);
executionTimeMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00002000);
logs_ = false;
bitField0_ = (bitField0_ & ~0x00004000);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsCe.internal_static_sonarqube_ws_ce_Task_descriptor;
}
public org.sonarqube.ws.WsCe.Task getDefaultInstanceForType() {
return org.sonarqube.ws.WsCe.Task.getDefaultInstance();
}
public org.sonarqube.ws.WsCe.Task build() {
org.sonarqube.ws.WsCe.Task result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsCe.Task buildPartial() {
org.sonarqube.ws.WsCe.Task result = new org.sonarqube.ws.WsCe.Task(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.componentId_ = componentId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.componentKey_ = componentKey_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.componentName_ = componentName_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.componentQualifier_ = componentQualifier_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.analysisId_ = analysisId_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.status_ = status_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.submittedAt_ = submittedAt_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.submitterLogin_ = submitterLogin_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.startedAt_ = startedAt_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.executedAt_ = executedAt_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.isLastExecuted_ = isLastExecuted_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00002000;
}
result.executionTimeMs_ = executionTimeMs_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00004000;
}
result.logs_ = logs_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsCe.Task) {
return mergeFrom((org.sonarqube.ws.WsCe.Task)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsCe.Task other) {
if (other == org.sonarqube.ws.WsCe.Task.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasType()) {
bitField0_ |= 0x00000002;
type_ = other.type_;
onChanged();
}
if (other.hasComponentId()) {
bitField0_ |= 0x00000004;
componentId_ = other.componentId_;
onChanged();
}
if (other.hasComponentKey()) {
bitField0_ |= 0x00000008;
componentKey_ = other.componentKey_;
onChanged();
}
if (other.hasComponentName()) {
bitField0_ |= 0x00000010;
componentName_ = other.componentName_;
onChanged();
}
if (other.hasComponentQualifier()) {
bitField0_ |= 0x00000020;
componentQualifier_ = other.componentQualifier_;
onChanged();
}
if (other.hasAnalysisId()) {
bitField0_ |= 0x00000040;
analysisId_ = other.analysisId_;
onChanged();
}
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasSubmittedAt()) {
bitField0_ |= 0x00000100;
submittedAt_ = other.submittedAt_;
onChanged();
}
if (other.hasSubmitterLogin()) {
bitField0_ |= 0x00000200;
submitterLogin_ = other.submitterLogin_;
onChanged();
}
if (other.hasStartedAt()) {
bitField0_ |= 0x00000400;
startedAt_ = other.startedAt_;
onChanged();
}
if (other.hasExecutedAt()) {
bitField0_ |= 0x00000800;
executedAt_ = other.executedAt_;
onChanged();
}
if (other.hasIsLastExecuted()) {
setIsLastExecuted(other.getIsLastExecuted());
}
if (other.hasExecutionTimeMs()) {
setExecutionTimeMs(other.getExecutionTimeMs());
}
if (other.hasLogs()) {
setLogs(other.getLogs());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsCe.Task parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsCe.Task) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 2;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
private java.lang.Object componentId_ = "";
/**
* optional string componentId = 3;
*/
public boolean hasComponentId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string componentId = 3;
*/
public java.lang.String getComponentId() {
java.lang.Object ref = componentId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string componentId = 3;
*/
public com.google.protobuf.ByteString
getComponentIdBytes() {
java.lang.Object ref = componentId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string componentId = 3;
*/
public Builder setComponentId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
componentId_ = value;
onChanged();
return this;
}
/**
* optional string componentId = 3;
*/
public Builder clearComponentId() {
bitField0_ = (bitField0_ & ~0x00000004);
componentId_ = getDefaultInstance().getComponentId();
onChanged();
return this;
}
/**
* optional string componentId = 3;
*/
public Builder setComponentIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
componentId_ = value;
onChanged();
return this;
}
private java.lang.Object componentKey_ = "";
/**
* optional string componentKey = 4;
*/
public boolean hasComponentKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string componentKey = 4;
*/
public java.lang.String getComponentKey() {
java.lang.Object ref = componentKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentKey_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string componentKey = 4;
*/
public com.google.protobuf.ByteString
getComponentKeyBytes() {
java.lang.Object ref = componentKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string componentKey = 4;
*/
public Builder setComponentKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
componentKey_ = value;
onChanged();
return this;
}
/**
* optional string componentKey = 4;
*/
public Builder clearComponentKey() {
bitField0_ = (bitField0_ & ~0x00000008);
componentKey_ = getDefaultInstance().getComponentKey();
onChanged();
return this;
}
/**
* optional string componentKey = 4;
*/
public Builder setComponentKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
componentKey_ = value;
onChanged();
return this;
}
private java.lang.Object componentName_ = "";
/**
* optional string componentName = 5;
*/
public boolean hasComponentName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string componentName = 5;
*/
public java.lang.String getComponentName() {
java.lang.Object ref = componentName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string componentName = 5;
*/
public com.google.protobuf.ByteString
getComponentNameBytes() {
java.lang.Object ref = componentName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string componentName = 5;
*/
public Builder setComponentName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
componentName_ = value;
onChanged();
return this;
}
/**
* optional string componentName = 5;
*/
public Builder clearComponentName() {
bitField0_ = (bitField0_ & ~0x00000010);
componentName_ = getDefaultInstance().getComponentName();
onChanged();
return this;
}
/**
* optional string componentName = 5;
*/
public Builder setComponentNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
componentName_ = value;
onChanged();
return this;
}
private java.lang.Object componentQualifier_ = "";
/**
* optional string componentQualifier = 6;
*/
public boolean hasComponentQualifier() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string componentQualifier = 6;
*/
public java.lang.String getComponentQualifier() {
java.lang.Object ref = componentQualifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
componentQualifier_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string componentQualifier = 6;
*/
public com.google.protobuf.ByteString
getComponentQualifierBytes() {
java.lang.Object ref = componentQualifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
componentQualifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string componentQualifier = 6;
*/
public Builder setComponentQualifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
componentQualifier_ = value;
onChanged();
return this;
}
/**
* optional string componentQualifier = 6;
*/
public Builder clearComponentQualifier() {
bitField0_ = (bitField0_ & ~0x00000020);
componentQualifier_ = getDefaultInstance().getComponentQualifier();
onChanged();
return this;
}
/**
* optional string componentQualifier = 6;
*/
public Builder setComponentQualifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
componentQualifier_ = value;
onChanged();
return this;
}
private java.lang.Object analysisId_ = "";
/**
* optional string analysisId = 7;
*/
public boolean hasAnalysisId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string analysisId = 7;
*/
public java.lang.String getAnalysisId() {
java.lang.Object ref = analysisId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
analysisId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string analysisId = 7;
*/
public com.google.protobuf.ByteString
getAnalysisIdBytes() {
java.lang.Object ref = analysisId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
analysisId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string analysisId = 7;
*/
public Builder setAnalysisId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
analysisId_ = value;
onChanged();
return this;
}
/**
* optional string analysisId = 7;
*/
public Builder clearAnalysisId() {
bitField0_ = (bitField0_ & ~0x00000040);
analysisId_ = getDefaultInstance().getAnalysisId();
onChanged();
return this;
}
/**
* optional string analysisId = 7;
*/
public Builder setAnalysisIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
analysisId_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public org.sonarqube.ws.WsCe.TaskStatus getStatus() {
org.sonarqube.ws.WsCe.TaskStatus result = org.sonarqube.ws.WsCe.TaskStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.WsCe.TaskStatus.PENDING : result;
}
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public Builder setStatus(org.sonarqube.ws.WsCe.TaskStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .sonarqube.ws.ce.TaskStatus status = 8;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000080);
status_ = 0;
onChanged();
return this;
}
private java.lang.Object submittedAt_ = "";
/**
* optional string submittedAt = 9;
*/
public boolean hasSubmittedAt() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string submittedAt = 9;
*/
public java.lang.String getSubmittedAt() {
java.lang.Object ref = submittedAt_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
submittedAt_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string submittedAt = 9;
*/
public com.google.protobuf.ByteString
getSubmittedAtBytes() {
java.lang.Object ref = submittedAt_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
submittedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string submittedAt = 9;
*/
public Builder setSubmittedAt(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
submittedAt_ = value;
onChanged();
return this;
}
/**
* optional string submittedAt = 9;
*/
public Builder clearSubmittedAt() {
bitField0_ = (bitField0_ & ~0x00000100);
submittedAt_ = getDefaultInstance().getSubmittedAt();
onChanged();
return this;
}
/**
* optional string submittedAt = 9;
*/
public Builder setSubmittedAtBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
submittedAt_ = value;
onChanged();
return this;
}
private java.lang.Object submitterLogin_ = "";
/**
* optional string submitterLogin = 10;
*/
public boolean hasSubmitterLogin() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string submitterLogin = 10;
*/
public java.lang.String getSubmitterLogin() {
java.lang.Object ref = submitterLogin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
submitterLogin_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string submitterLogin = 10;
*/
public com.google.protobuf.ByteString
getSubmitterLoginBytes() {
java.lang.Object ref = submitterLogin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
submitterLogin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string submitterLogin = 10;
*/
public Builder setSubmitterLogin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
submitterLogin_ = value;
onChanged();
return this;
}
/**
* optional string submitterLogin = 10;
*/
public Builder clearSubmitterLogin() {
bitField0_ = (bitField0_ & ~0x00000200);
submitterLogin_ = getDefaultInstance().getSubmitterLogin();
onChanged();
return this;
}
/**
* optional string submitterLogin = 10;
*/
public Builder setSubmitterLoginBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
submitterLogin_ = value;
onChanged();
return this;
}
private java.lang.Object startedAt_ = "";
/**
* optional string startedAt = 11;
*/
public boolean hasStartedAt() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string startedAt = 11;
*/
public java.lang.String getStartedAt() {
java.lang.Object ref = startedAt_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
startedAt_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string startedAt = 11;
*/
public com.google.protobuf.ByteString
getStartedAtBytes() {
java.lang.Object ref = startedAt_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string startedAt = 11;
*/
public Builder setStartedAt(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
startedAt_ = value;
onChanged();
return this;
}
/**
* optional string startedAt = 11;
*/
public Builder clearStartedAt() {
bitField0_ = (bitField0_ & ~0x00000400);
startedAt_ = getDefaultInstance().getStartedAt();
onChanged();
return this;
}
/**
* optional string startedAt = 11;
*/
public Builder setStartedAtBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
startedAt_ = value;
onChanged();
return this;
}
private java.lang.Object executedAt_ = "";
/**
* optional string executedAt = 12;
*/
public boolean hasExecutedAt() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string executedAt = 12;
*/
public java.lang.String getExecutedAt() {
java.lang.Object ref = executedAt_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
executedAt_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string executedAt = 12;
*/
public com.google.protobuf.ByteString
getExecutedAtBytes() {
java.lang.Object ref = executedAt_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executedAt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string executedAt = 12;
*/
public Builder setExecutedAt(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
executedAt_ = value;
onChanged();
return this;
}
/**
* optional string executedAt = 12;
*/
public Builder clearExecutedAt() {
bitField0_ = (bitField0_ & ~0x00000800);
executedAt_ = getDefaultInstance().getExecutedAt();
onChanged();
return this;
}
/**
* optional string executedAt = 12;
*/
public Builder setExecutedAtBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
executedAt_ = value;
onChanged();
return this;
}
private boolean isLastExecuted_ ;
/**
* optional bool isLastExecuted = 13;
*/
public boolean hasIsLastExecuted() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool isLastExecuted = 13;
*/
public boolean getIsLastExecuted() {
return isLastExecuted_;
}
/**
* optional bool isLastExecuted = 13;
*/
public Builder setIsLastExecuted(boolean value) {
bitField0_ |= 0x00001000;
isLastExecuted_ = value;
onChanged();
return this;
}
/**
* optional bool isLastExecuted = 13;
*/
public Builder clearIsLastExecuted() {
bitField0_ = (bitField0_ & ~0x00001000);
isLastExecuted_ = false;
onChanged();
return this;
}
private long executionTimeMs_ ;
/**
* optional int64 executionTimeMs = 14;
*/
public boolean hasExecutionTimeMs() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional int64 executionTimeMs = 14;
*/
public long getExecutionTimeMs() {
return executionTimeMs_;
}
/**
* optional int64 executionTimeMs = 14;
*/
public Builder setExecutionTimeMs(long value) {
bitField0_ |= 0x00002000;
executionTimeMs_ = value;
onChanged();
return this;
}
/**
* optional int64 executionTimeMs = 14;
*/
public Builder clearExecutionTimeMs() {
bitField0_ = (bitField0_ & ~0x00002000);
executionTimeMs_ = 0L;
onChanged();
return this;
}
private boolean logs_ ;
/**
* optional bool logs = 15;
*/
public boolean hasLogs() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool logs = 15;
*/
public boolean getLogs() {
return logs_;
}
/**
* optional bool logs = 15;
*/
public Builder setLogs(boolean value) {
bitField0_ |= 0x00004000;
logs_ = value;
onChanged();
return this;
}
/**
* optional bool logs = 15;
*/
public Builder clearLogs() {
bitField0_ = (bitField0_ & ~0x00004000);
logs_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.ce.Task)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.ce.Task)
private static final org.sonarqube.ws.WsCe.Task DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsCe.Task();
}
public static org.sonarqube.ws.WsCe.Task getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Task parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Task(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsCe.Task getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_SubmitResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_SubmitResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_TaskResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_TaskResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_ActivityResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_ActivityResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_ProjectResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_ProjectResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_TaskTypesWsResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_ce_Task_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_ce_Task_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\013ws-ce.proto\022\017sonarqube.ws.ce\032\020ws-commo" +
"ns.proto\"3\n\016SubmitResponse\022\016\n\006taskId\030\001 \001" +
"(\t\022\021\n\tprojectId\030\002 \001(\t\"3\n\014TaskResponse\022#\n" +
"\004task\030\001 \001(\0132\025.sonarqube.ws.ce.Task\"l\n\020Ac" +
"tivityResponse\0222\n\014unusedPaging\030\001 \001(\0132\034.s" +
"onarqube.ws.commons.Paging\022$\n\005tasks\030\002 \003(" +
"\0132\025.sonarqube.ws.ce.Task\"<\n\030ActivityStat" +
"usWsResponse\022\017\n\007pending\030\001 \001(\005\022\017\n\007failing" +
"\030\002 \001(\005\"_\n\017ProjectResponse\022$\n\005queue\030\001 \003(\013" +
"2\025.sonarqube.ws.ce.Task\022&\n\007current\030\002 \001(\013",
"2\025.sonarqube.ws.ce.Task\"(\n\023TaskTypesWsRe" +
"sponse\022\021\n\ttaskTypes\030\001 \003(\t\"\322\002\n\004Task\022\n\n\002id" +
"\030\001 \001(\t\022\014\n\004type\030\002 \001(\t\022\023\n\013componentId\030\003 \001(" +
"\t\022\024\n\014componentKey\030\004 \001(\t\022\025\n\rcomponentName" +
"\030\005 \001(\t\022\032\n\022componentQualifier\030\006 \001(\t\022\022\n\nan" +
"alysisId\030\007 \001(\t\022+\n\006status\030\010 \001(\0162\033.sonarqu" +
"be.ws.ce.TaskStatus\022\023\n\013submittedAt\030\t \001(\t" +
"\022\026\n\016submitterLogin\030\n \001(\t\022\021\n\tstartedAt\030\013 " +
"\001(\t\022\022\n\nexecutedAt\030\014 \001(\t\022\026\n\016isLastExecute" +
"d\030\r \001(\010\022\027\n\017executionTimeMs\030\016 \001(\003\022\014\n\004logs",
"\030\017 \001(\010*Q\n\nTaskStatus\022\013\n\007PENDING\020\000\022\017\n\013IN_" +
"PROGRESS\020\001\022\013\n\007SUCCESS\020\002\022\n\n\006FAILED\020\003\022\014\n\010C" +
"ANCELED\020\004B\032\n\020org.sonarqube.wsB\004WsCeH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.sonarqube.ws.Common.getDescriptor(),
}, assigner);
internal_static_sonarqube_ws_ce_SubmitResponse_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sonarqube_ws_ce_SubmitResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_SubmitResponse_descriptor,
new java.lang.String[] { "TaskId", "ProjectId", });
internal_static_sonarqube_ws_ce_TaskResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sonarqube_ws_ce_TaskResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_TaskResponse_descriptor,
new java.lang.String[] { "Task", });
internal_static_sonarqube_ws_ce_ActivityResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sonarqube_ws_ce_ActivityResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_ActivityResponse_descriptor,
new java.lang.String[] { "UnusedPaging", "Tasks", });
internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_ActivityStatusWsResponse_descriptor,
new java.lang.String[] { "Pending", "Failing", });
internal_static_sonarqube_ws_ce_ProjectResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_sonarqube_ws_ce_ProjectResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_ProjectResponse_descriptor,
new java.lang.String[] { "Queue", "Current", });
internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_sonarqube_ws_ce_TaskTypesWsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_TaskTypesWsResponse_descriptor,
new java.lang.String[] { "TaskTypes", });
internal_static_sonarqube_ws_ce_Task_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_sonarqube_ws_ce_Task_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_ce_Task_descriptor,
new java.lang.String[] { "Id", "Type", "ComponentId", "ComponentKey", "ComponentName", "ComponentQualifier", "AnalysisId", "Status", "SubmittedAt", "SubmitterLogin", "StartedAt", "ExecutedAt", "IsLastExecuted", "ExecutionTimeMs", "Logs", });
org.sonarqube.ws.Common.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy