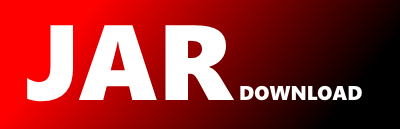
org.sonarqube.ws.WsTests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-ws Show documentation
Show all versions of sonar-ws Show documentation
Open source platform for continuous inspection of code quality
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ws-tests.proto
package org.sonarqube.ws;
public final class WsTests {
private WsTests() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code sonarqube.ws.tests.TestStatus}
*/
public enum TestStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OK = 1;
*/
OK(0, 1),
/**
* FAILURE = 2;
*/
FAILURE(1, 2),
/**
* ERROR = 3;
*/
ERROR(2, 3),
/**
* SKIPPED = 4;
*/
SKIPPED(3, 4),
;
/**
* OK = 1;
*/
public static final int OK_VALUE = 1;
/**
* FAILURE = 2;
*/
public static final int FAILURE_VALUE = 2;
/**
* ERROR = 3;
*/
public static final int ERROR_VALUE = 3;
/**
* SKIPPED = 4;
*/
public static final int SKIPPED_VALUE = 4;
public final int getNumber() {
return value;
}
public static TestStatus valueOf(int value) {
switch (value) {
case 1: return OK;
case 2: return FAILURE;
case 3: return ERROR;
case 4: return SKIPPED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TestStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TestStatus findValueByNumber(int number) {
return TestStatus.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.getDescriptor().getEnumTypes().get(0);
}
private static final TestStatus[] VALUES = values();
public static TestStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private TestStatus(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:sonarqube.ws.tests.TestStatus)
}
public interface ListResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.tests.ListResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
boolean hasPaging();
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
org.sonarqube.ws.Common.Paging getPaging();
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
org.sonarqube.ws.Common.PagingOrBuilder getPagingOrBuilder();
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
java.util.List
getTestsList();
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
org.sonarqube.ws.WsTests.Test getTests(int index);
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
int getTestsCount();
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
java.util.List extends org.sonarqube.ws.WsTests.TestOrBuilder>
getTestsOrBuilderList();
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
org.sonarqube.ws.WsTests.TestOrBuilder getTestsOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.tests.ListResponse}
*
*
* WS api/tests/list
*
*/
public static final class ListResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.tests.ListResponse)
ListResponseOrBuilder {
// Use ListResponse.newBuilder() to construct.
private ListResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ListResponse() {
tests_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.sonarqube.ws.Common.Paging.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = paging_.toBuilder();
}
paging_ = input.readMessage(org.sonarqube.ws.Common.Paging.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(paging_);
paging_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
tests_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
tests_.add(input.readMessage(org.sonarqube.ws.WsTests.Test.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
tests_ = java.util.Collections.unmodifiableList(tests_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_ListResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_ListResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.ListResponse.class, org.sonarqube.ws.WsTests.ListResponse.Builder.class);
}
private int bitField0_;
public static final int PAGING_FIELD_NUMBER = 1;
private org.sonarqube.ws.Common.Paging paging_;
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public boolean hasPaging() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public org.sonarqube.ws.Common.Paging getPaging() {
return paging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : paging_;
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public org.sonarqube.ws.Common.PagingOrBuilder getPagingOrBuilder() {
return paging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : paging_;
}
public static final int TESTS_FIELD_NUMBER = 2;
private java.util.List tests_;
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public java.util.List getTestsList() {
return tests_;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public java.util.List extends org.sonarqube.ws.WsTests.TestOrBuilder>
getTestsOrBuilderList() {
return tests_;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public int getTestsCount() {
return tests_.size();
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.Test getTests(int index) {
return tests_.get(index);
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.TestOrBuilder getTestsOrBuilder(
int index) {
return tests_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getPaging());
}
for (int i = 0; i < tests_.size(); i++) {
output.writeMessage(2, tests_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPaging());
}
for (int i = 0; i < tests_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, tests_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.ListResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsTests.ListResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.ListResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsTests.ListResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.tests.ListResponse}
*
*
* WS api/tests/list
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.tests.ListResponse)
org.sonarqube.ws.WsTests.ListResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_ListResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_ListResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.ListResponse.class, org.sonarqube.ws.WsTests.ListResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsTests.ListResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPagingFieldBuilder();
getTestsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (pagingBuilder_ == null) {
paging_ = null;
} else {
pagingBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (testsBuilder_ == null) {
tests_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
testsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_ListResponse_descriptor;
}
public org.sonarqube.ws.WsTests.ListResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsTests.ListResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsTests.ListResponse build() {
org.sonarqube.ws.WsTests.ListResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsTests.ListResponse buildPartial() {
org.sonarqube.ws.WsTests.ListResponse result = new org.sonarqube.ws.WsTests.ListResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (pagingBuilder_ == null) {
result.paging_ = paging_;
} else {
result.paging_ = pagingBuilder_.build();
}
if (testsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
tests_ = java.util.Collections.unmodifiableList(tests_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.tests_ = tests_;
} else {
result.tests_ = testsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsTests.ListResponse) {
return mergeFrom((org.sonarqube.ws.WsTests.ListResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsTests.ListResponse other) {
if (other == org.sonarqube.ws.WsTests.ListResponse.getDefaultInstance()) return this;
if (other.hasPaging()) {
mergePaging(other.getPaging());
}
if (testsBuilder_ == null) {
if (!other.tests_.isEmpty()) {
if (tests_.isEmpty()) {
tests_ = other.tests_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTestsIsMutable();
tests_.addAll(other.tests_);
}
onChanged();
}
} else {
if (!other.tests_.isEmpty()) {
if (testsBuilder_.isEmpty()) {
testsBuilder_.dispose();
testsBuilder_ = null;
tests_ = other.tests_;
bitField0_ = (bitField0_ & ~0x00000002);
testsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getTestsFieldBuilder() : null;
} else {
testsBuilder_.addAllMessages(other.tests_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsTests.ListResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsTests.ListResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.sonarqube.ws.Common.Paging paging_ = null;
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder> pagingBuilder_;
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public boolean hasPaging() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public org.sonarqube.ws.Common.Paging getPaging() {
if (pagingBuilder_ == null) {
return paging_ == null ? org.sonarqube.ws.Common.Paging.getDefaultInstance() : paging_;
} else {
return pagingBuilder_.getMessage();
}
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public Builder setPaging(org.sonarqube.ws.Common.Paging value) {
if (pagingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
paging_ = value;
onChanged();
} else {
pagingBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public Builder setPaging(
org.sonarqube.ws.Common.Paging.Builder builderForValue) {
if (pagingBuilder_ == null) {
paging_ = builderForValue.build();
onChanged();
} else {
pagingBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public Builder mergePaging(org.sonarqube.ws.Common.Paging value) {
if (pagingBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
paging_ != null &&
paging_ != org.sonarqube.ws.Common.Paging.getDefaultInstance()) {
paging_ =
org.sonarqube.ws.Common.Paging.newBuilder(paging_).mergeFrom(value).buildPartial();
} else {
paging_ = value;
}
onChanged();
} else {
pagingBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public Builder clearPaging() {
if (pagingBuilder_ == null) {
paging_ = null;
onChanged();
} else {
pagingBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public org.sonarqube.ws.Common.Paging.Builder getPagingBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getPagingFieldBuilder().getBuilder();
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
public org.sonarqube.ws.Common.PagingOrBuilder getPagingOrBuilder() {
if (pagingBuilder_ != null) {
return pagingBuilder_.getMessageOrBuilder();
} else {
return paging_ == null ?
org.sonarqube.ws.Common.Paging.getDefaultInstance() : paging_;
}
}
/**
* optional .sonarqube.ws.commons.Paging paging = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder>
getPagingFieldBuilder() {
if (pagingBuilder_ == null) {
pagingBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.sonarqube.ws.Common.Paging, org.sonarqube.ws.Common.Paging.Builder, org.sonarqube.ws.Common.PagingOrBuilder>(
getPaging(),
getParentForChildren(),
isClean());
paging_ = null;
}
return pagingBuilder_;
}
private java.util.List tests_ =
java.util.Collections.emptyList();
private void ensureTestsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
tests_ = new java.util.ArrayList(tests_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.Test, org.sonarqube.ws.WsTests.Test.Builder, org.sonarqube.ws.WsTests.TestOrBuilder> testsBuilder_;
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public java.util.List getTestsList() {
if (testsBuilder_ == null) {
return java.util.Collections.unmodifiableList(tests_);
} else {
return testsBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public int getTestsCount() {
if (testsBuilder_ == null) {
return tests_.size();
} else {
return testsBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.Test getTests(int index) {
if (testsBuilder_ == null) {
return tests_.get(index);
} else {
return testsBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder setTests(
int index, org.sonarqube.ws.WsTests.Test value) {
if (testsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTestsIsMutable();
tests_.set(index, value);
onChanged();
} else {
testsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder setTests(
int index, org.sonarqube.ws.WsTests.Test.Builder builderForValue) {
if (testsBuilder_ == null) {
ensureTestsIsMutable();
tests_.set(index, builderForValue.build());
onChanged();
} else {
testsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder addTests(org.sonarqube.ws.WsTests.Test value) {
if (testsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTestsIsMutable();
tests_.add(value);
onChanged();
} else {
testsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder addTests(
int index, org.sonarqube.ws.WsTests.Test value) {
if (testsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTestsIsMutable();
tests_.add(index, value);
onChanged();
} else {
testsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder addTests(
org.sonarqube.ws.WsTests.Test.Builder builderForValue) {
if (testsBuilder_ == null) {
ensureTestsIsMutable();
tests_.add(builderForValue.build());
onChanged();
} else {
testsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder addTests(
int index, org.sonarqube.ws.WsTests.Test.Builder builderForValue) {
if (testsBuilder_ == null) {
ensureTestsIsMutable();
tests_.add(index, builderForValue.build());
onChanged();
} else {
testsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder addAllTests(
java.lang.Iterable extends org.sonarqube.ws.WsTests.Test> values) {
if (testsBuilder_ == null) {
ensureTestsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tests_);
onChanged();
} else {
testsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder clearTests() {
if (testsBuilder_ == null) {
tests_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
testsBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public Builder removeTests(int index) {
if (testsBuilder_ == null) {
ensureTestsIsMutable();
tests_.remove(index);
onChanged();
} else {
testsBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.Test.Builder getTestsBuilder(
int index) {
return getTestsFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.TestOrBuilder getTestsOrBuilder(
int index) {
if (testsBuilder_ == null) {
return tests_.get(index); } else {
return testsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public java.util.List extends org.sonarqube.ws.WsTests.TestOrBuilder>
getTestsOrBuilderList() {
if (testsBuilder_ != null) {
return testsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tests_);
}
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.Test.Builder addTestsBuilder() {
return getTestsFieldBuilder().addBuilder(
org.sonarqube.ws.WsTests.Test.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public org.sonarqube.ws.WsTests.Test.Builder addTestsBuilder(
int index) {
return getTestsFieldBuilder().addBuilder(
index, org.sonarqube.ws.WsTests.Test.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.tests.Test tests = 2;
*/
public java.util.List
getTestsBuilderList() {
return getTestsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.Test, org.sonarqube.ws.WsTests.Test.Builder, org.sonarqube.ws.WsTests.TestOrBuilder>
getTestsFieldBuilder() {
if (testsBuilder_ == null) {
testsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.Test, org.sonarqube.ws.WsTests.Test.Builder, org.sonarqube.ws.WsTests.TestOrBuilder>(
tests_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
tests_ = null;
}
return testsBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.tests.ListResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.tests.ListResponse)
private static final org.sonarqube.ws.WsTests.ListResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsTests.ListResponse();
}
public static org.sonarqube.ws.WsTests.ListResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ListResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ListResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsTests.ListResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CoveredFilesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.tests.CoveredFilesResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
java.util.List
getFilesList();
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getFiles(int index);
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
int getFilesCount();
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
java.util.List extends org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder>
getFilesOrBuilderList();
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder getFilesOrBuilder(
int index);
}
/**
* Protobuf type {@code sonarqube.ws.tests.CoveredFilesResponse}
*
*
* WS api/tests/covered_files
*
*/
public static final class CoveredFilesResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.tests.CoveredFilesResponse)
CoveredFilesResponseOrBuilder {
// Use CoveredFilesResponse.newBuilder() to construct.
private CoveredFilesResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CoveredFilesResponse() {
files_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CoveredFilesResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
files_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
files_.add(input.readMessage(org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
files_ = java.util.Collections.unmodifiableList(files_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.CoveredFilesResponse.class, org.sonarqube.ws.WsTests.CoveredFilesResponse.Builder.class);
}
public interface CoveredFileOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.tests.CoveredFilesResponse.CoveredFile)
com.google.protobuf.MessageOrBuilder {
/**
* optional string id = 1;
*/
boolean hasId();
/**
* optional string id = 1;
*/
java.lang.String getId();
/**
* optional string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional string key = 2;
*/
boolean hasKey();
/**
* optional string key = 2;
*/
java.lang.String getKey();
/**
* optional string key = 2;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional string longName = 3;
*/
boolean hasLongName();
/**
* optional string longName = 3;
*/
java.lang.String getLongName();
/**
* optional string longName = 3;
*/
com.google.protobuf.ByteString
getLongNameBytes();
/**
* optional int32 coveredLines = 4;
*/
boolean hasCoveredLines();
/**
* optional int32 coveredLines = 4;
*/
int getCoveredLines();
}
/**
* Protobuf type {@code sonarqube.ws.tests.CoveredFilesResponse.CoveredFile}
*/
public static final class CoveredFile extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.tests.CoveredFilesResponse.CoveredFile)
CoveredFileOrBuilder {
// Use CoveredFile.newBuilder() to construct.
private CoveredFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CoveredFile() {
id_ = "";
key_ = "";
longName_ = "";
coveredLines_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CoveredFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
key_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
longName_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
coveredLines_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.class, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object key_;
/**
* optional string key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string key = 2;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 2;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LONGNAME_FIELD_NUMBER = 3;
private volatile java.lang.Object longName_;
/**
* optional string longName = 3;
*/
public boolean hasLongName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string longName = 3;
*/
public java.lang.String getLongName() {
java.lang.Object ref = longName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longName_ = s;
}
return s;
}
}
/**
* optional string longName = 3;
*/
public com.google.protobuf.ByteString
getLongNameBytes() {
java.lang.Object ref = longName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COVEREDLINES_FIELD_NUMBER = 4;
private int coveredLines_;
/**
* optional int32 coveredLines = 4;
*/
public boolean hasCoveredLines() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 coveredLines = 4;
*/
public int getCoveredLines() {
return coveredLines_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, longName_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, coveredLines_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, longName_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, coveredLines_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.tests.CoveredFilesResponse.CoveredFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.tests.CoveredFilesResponse.CoveredFile)
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.class, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder.class);
}
// Construct using org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
key_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
longName_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
coveredLines_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getDefaultInstanceForType() {
return org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.getDefaultInstance();
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile build() {
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile buildPartial() {
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile result = new org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.longName_ = longName_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.coveredLines_ = coveredLines_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile) {
return mergeFrom((org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile other) {
if (other == org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasKey()) {
bitField0_ |= 0x00000002;
key_ = other.key_;
onChanged();
}
if (other.hasLongName()) {
bitField0_ |= 0x00000004;
longName_ = other.longName_;
onChanged();
}
if (other.hasCoveredLines()) {
setCoveredLines(other.getCoveredLines());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private java.lang.Object key_ = "";
/**
* optional string key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string key = 2;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 2;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 2;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 2;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000002);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 2;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
key_ = value;
onChanged();
return this;
}
private java.lang.Object longName_ = "";
/**
* optional string longName = 3;
*/
public boolean hasLongName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string longName = 3;
*/
public java.lang.String getLongName() {
java.lang.Object ref = longName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
longName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string longName = 3;
*/
public com.google.protobuf.ByteString
getLongNameBytes() {
java.lang.Object ref = longName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string longName = 3;
*/
public Builder setLongName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
longName_ = value;
onChanged();
return this;
}
/**
* optional string longName = 3;
*/
public Builder clearLongName() {
bitField0_ = (bitField0_ & ~0x00000004);
longName_ = getDefaultInstance().getLongName();
onChanged();
return this;
}
/**
* optional string longName = 3;
*/
public Builder setLongNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
longName_ = value;
onChanged();
return this;
}
private int coveredLines_ ;
/**
* optional int32 coveredLines = 4;
*/
public boolean hasCoveredLines() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 coveredLines = 4;
*/
public int getCoveredLines() {
return coveredLines_;
}
/**
* optional int32 coveredLines = 4;
*/
public Builder setCoveredLines(int value) {
bitField0_ |= 0x00000008;
coveredLines_ = value;
onChanged();
return this;
}
/**
* optional int32 coveredLines = 4;
*/
public Builder clearCoveredLines() {
bitField0_ = (bitField0_ & ~0x00000008);
coveredLines_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.tests.CoveredFilesResponse.CoveredFile)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.tests.CoveredFilesResponse.CoveredFile)
private static final org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile();
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CoveredFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new CoveredFile(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FILES_FIELD_NUMBER = 1;
private java.util.List files_;
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public java.util.List getFilesList() {
return files_;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public java.util.List extends org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder>
getFilesOrBuilderList() {
return files_;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public int getFilesCount() {
return files_.size();
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getFiles(int index) {
return files_.get(index);
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder getFilesOrBuilder(
int index) {
return files_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < files_.size(); i++) {
output.writeMessage(1, files_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < files_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, files_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsTests.CoveredFilesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.tests.CoveredFilesResponse}
*
*
* WS api/tests/covered_files
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.tests.CoveredFilesResponse)
org.sonarqube.ws.WsTests.CoveredFilesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.CoveredFilesResponse.class, org.sonarqube.ws.WsTests.CoveredFilesResponse.Builder.class);
}
// Construct using org.sonarqube.ws.WsTests.CoveredFilesResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFilesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
filesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse getDefaultInstanceForType() {
return org.sonarqube.ws.WsTests.CoveredFilesResponse.getDefaultInstance();
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse build() {
org.sonarqube.ws.WsTests.CoveredFilesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse buildPartial() {
org.sonarqube.ws.WsTests.CoveredFilesResponse result = new org.sonarqube.ws.WsTests.CoveredFilesResponse(this);
int from_bitField0_ = bitField0_;
if (filesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
files_ = java.util.Collections.unmodifiableList(files_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.files_ = files_;
} else {
result.files_ = filesBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsTests.CoveredFilesResponse) {
return mergeFrom((org.sonarqube.ws.WsTests.CoveredFilesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsTests.CoveredFilesResponse other) {
if (other == org.sonarqube.ws.WsTests.CoveredFilesResponse.getDefaultInstance()) return this;
if (filesBuilder_ == null) {
if (!other.files_.isEmpty()) {
if (files_.isEmpty()) {
files_ = other.files_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFilesIsMutable();
files_.addAll(other.files_);
}
onChanged();
}
} else {
if (!other.files_.isEmpty()) {
if (filesBuilder_.isEmpty()) {
filesBuilder_.dispose();
filesBuilder_ = null;
files_ = other.files_;
bitField0_ = (bitField0_ & ~0x00000001);
filesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFilesFieldBuilder() : null;
} else {
filesBuilder_.addAllMessages(other.files_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsTests.CoveredFilesResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsTests.CoveredFilesResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List files_ =
java.util.Collections.emptyList();
private void ensureFilesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
files_ = new java.util.ArrayList(files_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder> filesBuilder_;
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public java.util.List getFilesList() {
if (filesBuilder_ == null) {
return java.util.Collections.unmodifiableList(files_);
} else {
return filesBuilder_.getMessageList();
}
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public int getFilesCount() {
if (filesBuilder_ == null) {
return files_.size();
} else {
return filesBuilder_.getCount();
}
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile getFiles(int index) {
if (filesBuilder_ == null) {
return files_.get(index);
} else {
return filesBuilder_.getMessage(index);
}
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder setFiles(
int index, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.set(index, value);
onChanged();
} else {
filesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder setFiles(
int index, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.set(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder addFiles(org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(value);
onChanged();
} else {
filesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder addFiles(
int index, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(index, value);
onChanged();
} else {
filesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder addFiles(
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder addFiles(
int index, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder addAllFiles(
java.lang.Iterable extends org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile> values) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, files_);
onChanged();
} else {
filesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder clearFiles() {
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
filesBuilder_.clear();
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public Builder removeFiles(int index) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.remove(index);
onChanged();
} else {
filesBuilder_.remove(index);
}
return this;
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder getFilesBuilder(
int index) {
return getFilesFieldBuilder().getBuilder(index);
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder getFilesOrBuilder(
int index) {
if (filesBuilder_ == null) {
return files_.get(index); } else {
return filesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public java.util.List extends org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder>
getFilesOrBuilderList() {
if (filesBuilder_ != null) {
return filesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(files_);
}
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder addFilesBuilder() {
return getFilesFieldBuilder().addBuilder(
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder addFilesBuilder(
int index) {
return getFilesFieldBuilder().addBuilder(
index, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.getDefaultInstance());
}
/**
* repeated .sonarqube.ws.tests.CoveredFilesResponse.CoveredFile files = 1;
*/
public java.util.List
getFilesBuilderList() {
return getFilesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder>
getFilesFieldBuilder() {
if (filesBuilder_ == null) {
filesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFile.Builder, org.sonarqube.ws.WsTests.CoveredFilesResponse.CoveredFileOrBuilder>(
files_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
files_ = null;
}
return filesBuilder_;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.tests.CoveredFilesResponse)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.tests.CoveredFilesResponse)
private static final org.sonarqube.ws.WsTests.CoveredFilesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsTests.CoveredFilesResponse();
}
public static org.sonarqube.ws.WsTests.CoveredFilesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CoveredFilesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new CoveredFilesResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsTests.CoveredFilesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TestOrBuilder extends
// @@protoc_insertion_point(interface_extends:sonarqube.ws.tests.Test)
com.google.protobuf.MessageOrBuilder {
/**
* optional string id = 1;
*/
boolean hasId();
/**
* optional string id = 1;
*/
java.lang.String getId();
/**
* optional string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional string name = 2;
*/
boolean hasName();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string fileId = 3;
*/
boolean hasFileId();
/**
* optional string fileId = 3;
*/
java.lang.String getFileId();
/**
* optional string fileId = 3;
*/
com.google.protobuf.ByteString
getFileIdBytes();
/**
* optional string fileKey = 4;
*/
boolean hasFileKey();
/**
* optional string fileKey = 4;
*/
java.lang.String getFileKey();
/**
* optional string fileKey = 4;
*/
com.google.protobuf.ByteString
getFileKeyBytes();
/**
* optional string fileName = 5;
*/
boolean hasFileName();
/**
* optional string fileName = 5;
*/
java.lang.String getFileName();
/**
* optional string fileName = 5;
*/
com.google.protobuf.ByteString
getFileNameBytes();
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
boolean hasStatus();
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
org.sonarqube.ws.WsTests.TestStatus getStatus();
/**
* optional int64 durationInMs = 7;
*/
boolean hasDurationInMs();
/**
* optional int64 durationInMs = 7;
*/
long getDurationInMs();
/**
* optional int32 coveredLines = 8;
*/
boolean hasCoveredLines();
/**
* optional int32 coveredLines = 8;
*/
int getCoveredLines();
/**
* optional string message = 9;
*/
boolean hasMessage();
/**
* optional string message = 9;
*/
java.lang.String getMessage();
/**
* optional string message = 9;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* optional string stacktrace = 10;
*/
boolean hasStacktrace();
/**
* optional string stacktrace = 10;
*/
java.lang.String getStacktrace();
/**
* optional string stacktrace = 10;
*/
com.google.protobuf.ByteString
getStacktraceBytes();
}
/**
* Protobuf type {@code sonarqube.ws.tests.Test}
*/
public static final class Test extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:sonarqube.ws.tests.Test)
TestOrBuilder {
// Use Test.newBuilder() to construct.
private Test(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Test() {
id_ = "";
name_ = "";
fileId_ = "";
fileKey_ = "";
fileName_ = "";
status_ = 1;
durationInMs_ = 0L;
coveredLines_ = 0;
message_ = "";
stacktrace_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Test(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
fileId_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
fileKey_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
fileName_ = bs;
break;
}
case 48: {
int rawValue = input.readEnum();
org.sonarqube.ws.WsTests.TestStatus value = org.sonarqube.ws.WsTests.TestStatus.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(6, rawValue);
} else {
bitField0_ |= 0x00000020;
status_ = rawValue;
}
break;
}
case 56: {
bitField0_ |= 0x00000040;
durationInMs_ = input.readInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
coveredLines_ = input.readInt32();
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
message_ = bs;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000200;
stacktrace_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_Test_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_Test_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.Test.class, org.sonarqube.ws.WsTests.Test.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILEID_FIELD_NUMBER = 3;
private volatile java.lang.Object fileId_;
/**
* optional string fileId = 3;
*/
public boolean hasFileId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fileId = 3;
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileId_ = s;
}
return s;
}
}
/**
* optional string fileId = 3;
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILEKEY_FIELD_NUMBER = 4;
private volatile java.lang.Object fileKey_;
/**
* optional string fileKey = 4;
*/
public boolean hasFileKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string fileKey = 4;
*/
public java.lang.String getFileKey() {
java.lang.Object ref = fileKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileKey_ = s;
}
return s;
}
}
/**
* optional string fileKey = 4;
*/
public com.google.protobuf.ByteString
getFileKeyBytes() {
java.lang.Object ref = fileKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILENAME_FIELD_NUMBER = 5;
private volatile java.lang.Object fileName_;
/**
* optional string fileName = 5;
*/
public boolean hasFileName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string fileName = 5;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileName_ = s;
}
return s;
}
}
/**
* optional string fileName = 5;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 6;
private int status_;
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public org.sonarqube.ws.WsTests.TestStatus getStatus() {
org.sonarqube.ws.WsTests.TestStatus result = org.sonarqube.ws.WsTests.TestStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.WsTests.TestStatus.OK : result;
}
public static final int DURATIONINMS_FIELD_NUMBER = 7;
private long durationInMs_;
/**
* optional int64 durationInMs = 7;
*/
public boolean hasDurationInMs() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 durationInMs = 7;
*/
public long getDurationInMs() {
return durationInMs_;
}
public static final int COVEREDLINES_FIELD_NUMBER = 8;
private int coveredLines_;
/**
* optional int32 coveredLines = 8;
*/
public boolean hasCoveredLines() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 coveredLines = 8;
*/
public int getCoveredLines() {
return coveredLines_;
}
public static final int MESSAGE_FIELD_NUMBER = 9;
private volatile java.lang.Object message_;
/**
* optional string message = 9;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string message = 9;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
message_ = s;
}
return s;
}
}
/**
* optional string message = 9;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STACKTRACE_FIELD_NUMBER = 10;
private volatile java.lang.Object stacktrace_;
/**
* optional string stacktrace = 10;
*/
public boolean hasStacktrace() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string stacktrace = 10;
*/
public java.lang.String getStacktrace() {
java.lang.Object ref = stacktrace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stacktrace_ = s;
}
return s;
}
}
/**
* optional string stacktrace = 10;
*/
public com.google.protobuf.ByteString
getStacktraceBytes() {
java.lang.Object ref = stacktrace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stacktrace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, fileId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, fileKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, fileName_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeEnum(6, status_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt64(7, durationInMs_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(8, coveredLines_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, message_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, stacktrace_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, fileId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, fileKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, fileName_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, status_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, durationInMs_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, coveredLines_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(9, message_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, stacktrace_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static org.sonarqube.ws.WsTests.Test parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.Test parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.sonarqube.ws.WsTests.Test parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.sonarqube.ws.WsTests.Test parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.sonarqube.ws.WsTests.Test prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code sonarqube.ws.tests.Test}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:sonarqube.ws.tests.Test)
org.sonarqube.ws.WsTests.TestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_Test_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_Test_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.sonarqube.ws.WsTests.Test.class, org.sonarqube.ws.WsTests.Test.Builder.class);
}
// Construct using org.sonarqube.ws.WsTests.Test.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
fileId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
fileKey_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
fileName_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
status_ = 1;
bitField0_ = (bitField0_ & ~0x00000020);
durationInMs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
coveredLines_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
message_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
stacktrace_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.sonarqube.ws.WsTests.internal_static_sonarqube_ws_tests_Test_descriptor;
}
public org.sonarqube.ws.WsTests.Test getDefaultInstanceForType() {
return org.sonarqube.ws.WsTests.Test.getDefaultInstance();
}
public org.sonarqube.ws.WsTests.Test build() {
org.sonarqube.ws.WsTests.Test result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.sonarqube.ws.WsTests.Test buildPartial() {
org.sonarqube.ws.WsTests.Test result = new org.sonarqube.ws.WsTests.Test(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.fileId_ = fileId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.fileKey_ = fileKey_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.fileName_ = fileName_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.status_ = status_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.durationInMs_ = durationInMs_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.coveredLines_ = coveredLines_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.message_ = message_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.stacktrace_ = stacktrace_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.sonarqube.ws.WsTests.Test) {
return mergeFrom((org.sonarqube.ws.WsTests.Test)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.sonarqube.ws.WsTests.Test other) {
if (other == org.sonarqube.ws.WsTests.Test.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasFileId()) {
bitField0_ |= 0x00000004;
fileId_ = other.fileId_;
onChanged();
}
if (other.hasFileKey()) {
bitField0_ |= 0x00000008;
fileKey_ = other.fileKey_;
onChanged();
}
if (other.hasFileName()) {
bitField0_ |= 0x00000010;
fileName_ = other.fileName_;
onChanged();
}
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasDurationInMs()) {
setDurationInMs(other.getDurationInMs());
}
if (other.hasCoveredLines()) {
setCoveredLines(other.getCoveredLines());
}
if (other.hasMessage()) {
bitField0_ |= 0x00000100;
message_ = other.message_;
onChanged();
}
if (other.hasStacktrace()) {
bitField0_ |= 0x00000200;
stacktrace_ = other.stacktrace_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.sonarqube.ws.WsTests.Test parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.sonarqube.ws.WsTests.Test) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object fileId_ = "";
/**
* optional string fileId = 3;
*/
public boolean hasFileId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fileId = 3;
*/
public java.lang.String getFileId() {
java.lang.Object ref = fileId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fileId = 3;
*/
public com.google.protobuf.ByteString
getFileIdBytes() {
java.lang.Object ref = fileId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fileId = 3;
*/
public Builder setFileId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fileId_ = value;
onChanged();
return this;
}
/**
* optional string fileId = 3;
*/
public Builder clearFileId() {
bitField0_ = (bitField0_ & ~0x00000004);
fileId_ = getDefaultInstance().getFileId();
onChanged();
return this;
}
/**
* optional string fileId = 3;
*/
public Builder setFileIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fileId_ = value;
onChanged();
return this;
}
private java.lang.Object fileKey_ = "";
/**
* optional string fileKey = 4;
*/
public boolean hasFileKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string fileKey = 4;
*/
public java.lang.String getFileKey() {
java.lang.Object ref = fileKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileKey_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fileKey = 4;
*/
public com.google.protobuf.ByteString
getFileKeyBytes() {
java.lang.Object ref = fileKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fileKey = 4;
*/
public Builder setFileKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
fileKey_ = value;
onChanged();
return this;
}
/**
* optional string fileKey = 4;
*/
public Builder clearFileKey() {
bitField0_ = (bitField0_ & ~0x00000008);
fileKey_ = getDefaultInstance().getFileKey();
onChanged();
return this;
}
/**
* optional string fileKey = 4;
*/
public Builder setFileKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
fileKey_ = value;
onChanged();
return this;
}
private java.lang.Object fileName_ = "";
/**
* optional string fileName = 5;
*/
public boolean hasFileName() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string fileName = 5;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fileName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fileName = 5;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fileName = 5;
*/
public Builder setFileName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
fileName_ = value;
onChanged();
return this;
}
/**
* optional string fileName = 5;
*/
public Builder clearFileName() {
bitField0_ = (bitField0_ & ~0x00000010);
fileName_ = getDefaultInstance().getFileName();
onChanged();
return this;
}
/**
* optional string fileName = 5;
*/
public Builder setFileNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
fileName_ = value;
onChanged();
return this;
}
private int status_ = 1;
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public org.sonarqube.ws.WsTests.TestStatus getStatus() {
org.sonarqube.ws.WsTests.TestStatus result = org.sonarqube.ws.WsTests.TestStatus.valueOf(status_);
return result == null ? org.sonarqube.ws.WsTests.TestStatus.OK : result;
}
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public Builder setStatus(org.sonarqube.ws.WsTests.TestStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .sonarqube.ws.tests.TestStatus status = 6;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000020);
status_ = 1;
onChanged();
return this;
}
private long durationInMs_ ;
/**
* optional int64 durationInMs = 7;
*/
public boolean hasDurationInMs() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 durationInMs = 7;
*/
public long getDurationInMs() {
return durationInMs_;
}
/**
* optional int64 durationInMs = 7;
*/
public Builder setDurationInMs(long value) {
bitField0_ |= 0x00000040;
durationInMs_ = value;
onChanged();
return this;
}
/**
* optional int64 durationInMs = 7;
*/
public Builder clearDurationInMs() {
bitField0_ = (bitField0_ & ~0x00000040);
durationInMs_ = 0L;
onChanged();
return this;
}
private int coveredLines_ ;
/**
* optional int32 coveredLines = 8;
*/
public boolean hasCoveredLines() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 coveredLines = 8;
*/
public int getCoveredLines() {
return coveredLines_;
}
/**
* optional int32 coveredLines = 8;
*/
public Builder setCoveredLines(int value) {
bitField0_ |= 0x00000080;
coveredLines_ = value;
onChanged();
return this;
}
/**
* optional int32 coveredLines = 8;
*/
public Builder clearCoveredLines() {
bitField0_ = (bitField0_ & ~0x00000080);
coveredLines_ = 0;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* optional string message = 9;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string message = 9;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
message_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string message = 9;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string message = 9;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
message_ = value;
onChanged();
return this;
}
/**
* optional string message = 9;
*/
public Builder clearMessage() {
bitField0_ = (bitField0_ & ~0x00000100);
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* optional string message = 9;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
message_ = value;
onChanged();
return this;
}
private java.lang.Object stacktrace_ = "";
/**
* optional string stacktrace = 10;
*/
public boolean hasStacktrace() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string stacktrace = 10;
*/
public java.lang.String getStacktrace() {
java.lang.Object ref = stacktrace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stacktrace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string stacktrace = 10;
*/
public com.google.protobuf.ByteString
getStacktraceBytes() {
java.lang.Object ref = stacktrace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stacktrace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string stacktrace = 10;
*/
public Builder setStacktrace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
stacktrace_ = value;
onChanged();
return this;
}
/**
* optional string stacktrace = 10;
*/
public Builder clearStacktrace() {
bitField0_ = (bitField0_ & ~0x00000200);
stacktrace_ = getDefaultInstance().getStacktrace();
onChanged();
return this;
}
/**
* optional string stacktrace = 10;
*/
public Builder setStacktraceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
stacktrace_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:sonarqube.ws.tests.Test)
}
// @@protoc_insertion_point(class_scope:sonarqube.ws.tests.Test)
private static final org.sonarqube.ws.WsTests.Test DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.sonarqube.ws.WsTests.Test();
}
public static org.sonarqube.ws.WsTests.Test getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Test parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Test(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.sonarqube.ws.WsTests.Test getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_tests_ListResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_tests_ListResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_tests_CoveredFilesResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_sonarqube_ws_tests_Test_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_sonarqube_ws_tests_Test_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016ws-tests.proto\022\022sonarqube.ws.tests\032\020ws" +
"-commons.proto\"e\n\014ListResponse\022,\n\006paging" +
"\030\001 \001(\0132\034.sonarqube.ws.commons.Paging\022\'\n\005" +
"tests\030\002 \003(\0132\030.sonarqube.ws.tests.Test\"\253\001" +
"\n\024CoveredFilesResponse\022C\n\005files\030\001 \003(\01324." +
"sonarqube.ws.tests.CoveredFilesResponse." +
"CoveredFile\032N\n\013CoveredFile\022\n\n\002id\030\001 \001(\t\022\013" +
"\n\003key\030\002 \001(\t\022\020\n\010longName\030\003 \001(\t\022\024\n\014covered" +
"Lines\030\004 \001(\005\"\324\001\n\004Test\022\n\n\002id\030\001 \001(\t\022\014\n\004name" +
"\030\002 \001(\t\022\016\n\006fileId\030\003 \001(\t\022\017\n\007fileKey\030\004 \001(\t\022",
"\020\n\010fileName\030\005 \001(\t\022.\n\006status\030\006 \001(\0162\036.sona" +
"rqube.ws.tests.TestStatus\022\024\n\014durationInM" +
"s\030\007 \001(\003\022\024\n\014coveredLines\030\010 \001(\005\022\017\n\007message" +
"\030\t \001(\t\022\022\n\nstacktrace\030\n \001(\t*9\n\nTestStatus" +
"\022\006\n\002OK\020\001\022\013\n\007FAILURE\020\002\022\t\n\005ERROR\020\003\022\013\n\007SKIP" +
"PED\020\004B\035\n\020org.sonarqube.wsB\007WsTestsH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.sonarqube.ws.Common.getDescriptor(),
}, assigner);
internal_static_sonarqube_ws_tests_ListResponse_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_sonarqube_ws_tests_ListResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_tests_ListResponse_descriptor,
new java.lang.String[] { "Paging", "Tests", });
internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_sonarqube_ws_tests_CoveredFilesResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor,
new java.lang.String[] { "Files", });
internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor =
internal_static_sonarqube_ws_tests_CoveredFilesResponse_descriptor.getNestedTypes().get(0);
internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_tests_CoveredFilesResponse_CoveredFile_descriptor,
new java.lang.String[] { "Id", "Key", "LongName", "CoveredLines", });
internal_static_sonarqube_ws_tests_Test_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_sonarqube_ws_tests_Test_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_sonarqube_ws_tests_Test_descriptor,
new java.lang.String[] { "Id", "Name", "FileId", "FileKey", "FileName", "Status", "DurationInMs", "CoveredLines", "Message", "Stacktrace", });
org.sonarqube.ws.Common.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy