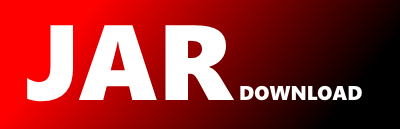
org.sonar.sslr.internal.toolkit.ToolkitPresenter Maven / Gradle / Ivy
The newest version!
/*
* SonarSource Language Recognizer
* Copyright (C) 2010-2020 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.sonar.sslr.internal.toolkit;
import com.sonar.sslr.api.AstNode;
import com.sonar.sslr.xpath.api.AstNodeXPathQuery;
import org.sonar.sslr.toolkit.ConfigurationModel;
import org.sonar.sslr.toolkit.ConfigurationProperty;
import java.awt.Point;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.io.Writer;
import java.lang.Thread.UncaughtExceptionHandler;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Objects;
public class ToolkitPresenter {
private final ConfigurationModel configurationModel;
private final SourceCodeModel model;
private ToolkitView view = null;
public ToolkitPresenter(ConfigurationModel configurationModel, SourceCodeModel model) {
this.configurationModel = configurationModel;
this.model = model;
}
public void setView(ToolkitView view) {
Objects.requireNonNull(view);
this.view = view;
}
// @VisibleForTesting
void checkInitialized() {
if (view == null) {
throw new IllegalStateException("the view must be set before the presenter can be ran");
}
}
// @VisibleForTesting
void initUncaughtExceptionsHandler() {
Thread.currentThread().setUncaughtExceptionHandler(new UncaughtExceptionHandler() {
@Override
public void uncaughtException(Thread t, Throwable e) {
Writer result = new StringWriter();
PrintWriter printWriter = new PrintWriter(result);
e.printStackTrace(printWriter);
view.appendToConsole(result.toString());
view.setFocusOnConsoleView();
}
});
}
// @VisibleForTesting
void initConfigurationTab() {
for (ConfigurationProperty configurationProperty : configurationModel.getProperties()) {
view.addConfigurationProperty(configurationProperty.getName(), configurationProperty.getDescription());
view.setConfigurationPropertyValue(configurationProperty.getName(), configurationProperty.getValue());
}
}
public void run(String title) {
checkInitialized();
initUncaughtExceptionsHandler();
view.setTitle(title);
view.displayHighlightedSourceCode("");
view.displayAst(null);
view.displayXml("");
view.disableXPathEvaluateButton();
initConfigurationTab();
view.run();
}
public void onSourceCodeOpenButtonClick() {
File fileToParse = view.pickFileToParse();
if (fileToParse != null) {
view.clearConsole();
try {
view.displayHighlightedSourceCode(new String(Files.readAllBytes(Paths.get(fileToParse.getPath())), configurationModel.getCharset()));
} catch (IOException e) {
throw new RuntimeException(e);
}
model.setSourceCode(fileToParse, configurationModel.getCharset());
view.displayHighlightedSourceCode(model.getHighlightedSourceCode());
view.displayAst(model.getAstNode());
view.displayXml(model.getXml());
view.scrollSourceCodeTo(new Point(0, 0));
view.setFocusOnAbstractSyntaxTreeView();
view.enableXPathEvaluateButton();
}
}
public void onSourceCodeParseButtonClick() {
view.clearConsole();
String sourceCode = view.getSourceCode();
model.setSourceCode(sourceCode);
Point sourceCodeScrollbarPosition = view.getSourceCodeScrollbarPosition();
view.displayHighlightedSourceCode(model.getHighlightedSourceCode());
view.displayAst(model.getAstNode());
view.displayXml(model.getXml());
view.scrollSourceCodeTo(sourceCodeScrollbarPosition);
view.setFocusOnAbstractSyntaxTreeView();
view.enableXPathEvaluateButton();
}
public void onXPathEvaluateButtonClick() {
String xpath = view.getXPath();
AstNodeXPathQuery
© 2015 - 2025 Weber Informatics LLC | Privacy Policy