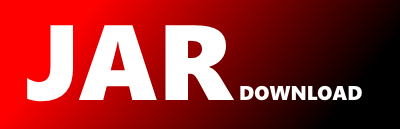
soot.toolkits.scalar.FlowSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of soot Show documentation
Show all versions of soot Show documentation
A Java Optimization Framework
package soot.toolkits.scalar;
/*-
* #%L
* Soot - a J*va Optimization Framework
* %%
* Copyright (C) 1997 - 1999 Raja Vallee-Rai
* modified 2002 Florian Loitsch
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Iterator;
import java.util.List;
/**
* Represents information for flow analysis. A FlowSet is an element of a lattice; this lattice might be described by a
* FlowUniverse. If add, remove, size, isEmpty, toList and contains are implemented, the lattice must be the powerset of some
* set.
*
* @see: FlowUniverse
*/
public interface FlowSet extends Iterable {
/**
* Clones the current FlowSet.
*/
public FlowSet clone();
/**
* returns an empty set, most often more efficient than: ((FlowSet)clone()).clear()
*/
public FlowSet emptySet();
/**
* Copies the current FlowSet into dest.
*/
public void copy(FlowSet dest);
/**
* Sets this FlowSet to the empty set (more generally, the bottom element of the lattice.)
*/
public void clear();
/**
* Returns the union (join) of this FlowSet and other
, putting result into this
.
*/
public void union(FlowSet other);
/**
* Returns the union (join) of this FlowSet and other
, putting result into dest
.
* dest
, other
and this
could be the same object.
*/
public void union(FlowSet other, FlowSet dest);
/**
* Returns the intersection (meet) of this FlowSet and other
, putting result into this
.
*/
public void intersection(FlowSet other);
/**
* Returns the intersection (meet) of this FlowSet and other
, putting result into dest
.
* dest
, other
and this
could be the same object.
*/
public void intersection(FlowSet other, FlowSet dest);
/**
* Returns the set difference (this intersect ~other) of this FlowSet and other
, putting result into
* this
.
*/
public void difference(FlowSet other);
/**
* Returns the set difference (this intersect ~other) of this FlowSet and other
, putting result into
* dest
. dest
, other
and this
could be the same object.
*/
public void difference(FlowSet other, FlowSet dest);
/**
* Returns true if this FlowSet is the empty set.
*/
public boolean isEmpty();
/* The following methods force the FlowSet to be a powerset. */
/**
* Returns the size of the current FlowSet.
*/
public int size();
/**
* Adds obj
to this
.
*/
public void add(T obj);
/**
* puts this
union obj
into dest
.
*/
public void add(T obj, FlowSet dest);
/**
* Removes obj
from this
.
*/
public void remove(T obj);
/**
* Puts this
minus obj
into dest
.
*/
public void remove(T obj, FlowSet dest);
/**
* Returns true if this FlowSet contains obj
.
*/
public boolean contains(T obj);
/**
* Returns true if the other
FlowSet is a subset of this
FlowSet.
*/
public boolean isSubSet(FlowSet other);
/**
* returns an iterator over the elements of the flowSet. Note that the iterator might be backed, and hence be faster in the
* creation, than doing toList().iterator()
.
*/
public Iterator iterator();
/**
* Returns an unbacked list of contained objects for this FlowSet.
*/
public List toList();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy