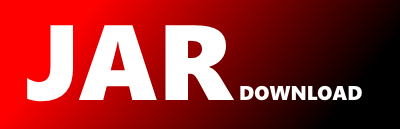
org.sosy_lab.common.Optionals Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Library of common components for SoSy-Lab Projects
/*
* SoSy-Lab Common is a library of useful utilities.
* This file is part of SoSy-Lab Common.
*
* Copyright (C) 2007-2016 Dirk Beyer
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sosy_lab.common;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Ordering;
import java.util.Comparator;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.stream.DoubleStream;
import java.util.stream.IntStream;
import java.util.stream.LongStream;
import java.util.stream.Stream;
/**
* Utilities for {@link Optional}.
*/
public class Optionals {
private Optionals() {}
/**
* Convert an {@link Optional} to a Guava {@link com.google.common.base.Optional}.
*/
public static com.google.common.base.Optional toGuavaOptional(Optional optional) {
return com.google.common.base.Optional.fromNullable(optional.orElse(null));
}
/**
* Convert a Guava {@link com.google.common.base.Optional} to an {@link Optional}.
*/
public static Optional fromGuavaOptional(com.google.common.base.Optional optional) {
return Optional.ofNullable(optional.orNull());
}
/**
* Return a set that is either empty or contains the present instance of the {@link Optional}.
*
* Can be used with {@link FluentIterable#transformAndConcat(com.google.common.base.Function)}
* to project an {@link Iterable} to the present instances.
* However, using {@link #presentInstances(Iterable)} would be more efficient.
*
* @param optional An Optional.
* @return A set with size at most one.
*/
public static ImmutableSet asSet(Optional optional) {
return optional.isPresent() ? ImmutableSet.of(optional.get()) : ImmutableSet.of();
}
/**
* Return a stream that is either empty or contains the present instance of the optional.
*
* Can be used with {@link Stream#flatMap(java.util.function.Function)} to project a stream
* to the present instances.
* However, using {@link #presentInstances(Stream)} would be more efficient.
*
* @param optional An Optional.
* @return A stream with size at most one.
*/
public static Stream asStream(Optional optional) {
return optional.isPresent() ? Stream.of(optional.get()) : Stream.empty();
}
/**
* Get an {@link Iterable} of the present instances of an iterable of {@link Optional}s.
*/
public static FluentIterable presentInstances(Iterable> iterable) {
return FluentIterable.from(iterable).filter(Optional::isPresent).transform(Optional::get);
}
/**
* Get a {@link Stream} of the present instances of a stream of {@link Optional}s.
*/
public static Stream presentInstances(Stream> stream) {
return stream.filter(Optional::isPresent).map(Optional::get);
}
/**
* Get a {@link IntStream} of the present integers of a stream of {@link OptionalInt}s.
*/
public static IntStream presentInts(Stream stream) {
return stream.filter(OptionalInt::isPresent).mapToInt(OptionalInt::getAsInt);
}
/**
* Get a {@link LongStream} of the present longs of a stream of {@link OptionalLong}s.
*/
public static LongStream presentLongs(Stream stream) {
return stream.filter(OptionalLong::isPresent).mapToLong(OptionalLong::getAsLong);
}
/**
* Get a {@link DoubleStream} of the present doubles of a stream of {@link OptionalDouble}s.
*/
public static DoubleStream presentDoubles(Stream stream) {
return stream.filter(OptionalDouble::isPresent).mapToDouble(OptionalDouble::getAsDouble);
}
/**
* Return a {@link Ordering} for {@link Optional} that compares empty optionals as smaller
* than all non-empty instances, and compares present values using their natural order.
*/
@SuppressWarnings("unchecked")
public static > Comparator> comparingEmptyFirst() {
return (Comparator>) OptionalComparators.NATURAL_EMTPY_FIRST;
}
/**
* Return a {@link Ordering} for {@link Optional} that compares empty optionals as smaller
* than all non-empty instances, and compares present values using the given comparator.
*/
public static Comparator> comparingEmptyFirst(Comparator super T> comparator) {
return new OptionalComparators.OptionalComparator<>(true, comparator);
}
/**
* Return a {@link Ordering} for {@link Optional} that compares empty optionals as larger
* than all non-empty instances, and compares present values using their natural order.
*/
@SuppressWarnings("unchecked")
public static > Comparator> comparingEmptyLast() {
return (Comparator>) OptionalComparators.NATURAL_EMTPY_LAST;
}
/**
* Return a {@link Ordering} for {@link Optional} that compares empty optionals as larger
* than all non-empty instances, and compares present values using the given comparator.
*/
public static Comparator> comparingEmptyLast(Comparator super T> comparator) {
return new OptionalComparators.OptionalComparator<>(false, comparator);
}
/**
* Return a {@link Ordering} for {@link OptionalInt} that compares empty optionals as smaller
* than all non-empty instances, and compares present integers using their natural order.
*/
public static Ordering comparingIntEmptyFirst() {
return OptionalComparators.INT_EMTPY_FIRST;
}
/**
* Return a {@link Ordering} for {@link OptionalInt} that compares empty optionals as larger
* than all non-empty instances, and compares present integers using their natural order.
*/
public static Ordering comparingIntEmptyLast() {
return OptionalComparators.INT_EMTPY_LAST;
}
/**
* Return a {@link Ordering} for {@link OptionalLong} that compares empty optionals as smaller
* than all non-empty instances, and compares present longs using their natural order.
*/
public static Ordering comparingLongEmptyFirst() {
return OptionalComparators.LONG_EMTPY_FIRST;
}
/**
* Return a {@link Ordering} for {@link OptionalLong} that compares empty optionals as larger
* than all non-empty instances, and compares present longs using their natural order.
*/
public static Ordering comparingLongEmptyLast() {
return OptionalComparators.LONG_EMTPY_LAST;
}
/**
* Return a {@link Ordering} for {@link OptionalDouble} that compares empty optionals as smaller
* than all non-empty instances, and compares present doubles using their natural order.
*/
public static Ordering comparingDoubleEmptyFirst() {
return OptionalComparators.DOUBLE_EMTPY_FIRST;
}
/**
* Return a {@link Ordering} for {@link OptionalDouble} that compares empty optionals as larger
* than all non-empty instances, and compares present doubles using their natural order.
*/
public static Ordering comparingDoubleEmptyLast() {
return OptionalComparators.DOUBLE_EMTPY_LAST;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy