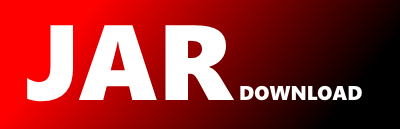
org.soulwing.jdbc.JdbcCall Maven / Gradle / Ivy
/*
* File created on Aug 8, 2015
*
* Copyright (c) 2015 Carl Harris, Jr
* and others as noted
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.soulwing.jdbc;
import java.sql.ResultSet;
import java.util.List;
/**
* A SQL call operation.
*
* The {@link #execute(Parameter...)} method be invoked repeatedly with
* different parameter values. When the call operation is no longer needed,
* it must be closed by either calling the {@link #close()}
* method or by nesting it within a try-with-resources construct.
*
* The call operation supports stored procedures that return values through
* any combination of
*
* - zero or more implicitly returned {@link ResultSet} objects,
*
- an optional update count, and
*
- zero or more parameters configured for OUT or INOUT semantics.
*
*
* While this API supports all of the possible return value mechanisms that
* are inherent with JDBC, the actual support provided for stored procedures
* varies greatly between JDBC vendors, with each vendor choosing which
* mechanisms to support. It is important to understand the capabilities of
* the specific JDBC vendor in order to effectively use this API.
*
* For maximum portability, on return from {@link #execute} the caller should
* first process all results sets and update counts, before retrieving the
* values of registered OUT/INOUT parameters.
*
* @author Carl Harris
*/
public interface JdbcCall extends AutoCloseable {
/**
* Executes this call operation.
* @param parameters values for placeholders in the statement; may be
* any combination of IN, OUT, or INOUT parameters supported by the
* target called procedure
* @return {@code true} if the first result is a {@link ResultSet},
* {@code false} if it is an update count or there are no results
*
*/
boolean execute(Parameter... parameters);
/**
* Closes the JDBC resources associated with this call operation.
*
* After a call operation is closed, its {@link #execute(Parameter...) execute}
* method may not be subsequently invoked.
*/
void close();
/**
* Gets the number of rows inserted/updated.
* @return update count or -1 if no update count is available
* @see {@link java.sql.Statement#getUpdateCount()}
*/
int getUpdateCount();
/**
* Gets a flag indicating the next result type available.
*
* Invoking this method implicitly closes the last {@link ResultSet} returned
* from the {@link #getResultSet()} method.
* @return {@code true} if the next result is a {@link ResultSet},
* {@code false} if it is an update count or there are no more results
* @see {@link java.sql.Statement#getMoreResults()}
*/
boolean getMoreResults();
/**
* Gets the current result set.
* @return result set or {@code null} if the current result is not a result set
* @see {@link java.sql.Statement#getResultSet()}
*/
ResultSet getResultSet();
/**
* Gets the value of an OUT or INOUT parameter.
* @param parameterIndex index of the parameter (starts at 1)
* @param type data type
* @return parameter value
*/
T getOutParameter(int parameterIndex, Class type);
/**
* Gets the value of an OUT or INOUT parameter.
* @param parameterName name of the parameter
* @param type data type
* @return parameter value
*/
T getOutParameter(String parameterName, Class type);
/**
* Processes the current result set using the given handler.
* @param handler the subject handler
* @return object produced by {@code handler}
*/
T handleResult(ResultSetHandler handler);
/**
* Retrieves all values of a given column in the current result set.
* @param columnLabel column label (name)
* @return list of values for the specified column
*/
List retrieveList(String columnLabel, Class type);
/**
* Retrieves all values of a given column in the current result set.
* @param columnIndex column index (starts at 1)
* @return list of values for the specified column
*/
List retrieveList(int columnIndex, Class type);
/**
* Retrieves and maps all rows in the current result set using the given
* row mapper.
* @param rowMapper the subject row mapper
* @return list of objects produced by {@link RowMapper}
*/
List retrieveList(RowMapper rowMapper);
/**
* Retrieves a column value from the current result set, which must contain
* a exactly one row.
*
* @param columnLabel column label (name)
* @return value of the specified column
* @throws SQLNoResultException if the result contains no rows
* @throws SQLNonUniqueResultException if the result contains more than one
* row
*/
T retrieveValue(String columnLabel, Class type);
/**
* Retrieves a column value from the current result set, which must contain
* a exactly one row.
*
* @param columnIndex column index (starts at 1)
* @return value of the specified column
* @throws SQLNoResultException if the result contains no rows
* @throws SQLNonUniqueResultException if the result contains more than one
* row
*/
T retrieveValue(int columnIndex, Class type);
/**
* Retrieves and maps a single row in current result set, which must contain
* exactly one row.
* @param rowMapper the subject row mapper
* @return object produced by {@link RowMapper} for the row
*/
T retrieveValue(RowMapper rowMapper);
}