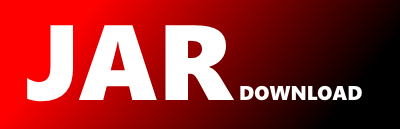
org.sourceprojects.xmlparser.XmlParserFactory Maven / Gradle / Ivy
The newest version!
/*
*
* This file is part of XmlParser.
*
* Foobar is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Foobar is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Foobar. If not, see .
*
*/
package org.sourceprojects.xmlparser;
import org.sourceprojects.xmlparser.exceptions.XmlParserInitializationException;
/**
* Defines a factory API that enables applications to obtain instances of the
* {@link org.sourceprojects.xmlparser.XmlParser} either by given standard
* implementations or by configuring own implementations.
*
* @author noctarius
* @since 0.0.1
*/
public final class XmlParserFactory {
/**
* Classname for the standard {@link org.sourceprojects.xmlparser.XmlParser}
* class to be created if no special implementation is given
*/
public static final String STANDARD_XMLPARSER_CLASS = "org.sourceprojects.xmlparser.internal.XmlParserImpl";
/**
* Classname for the standard XmlParser
* {@link org.sourceprojects.xmlparser.ParserContext} class to be created if
* no special implementation is given
*/
private static String STANDARD_PARSERCONTEXT_CLASS = "org.sourceprojects.xmlparser.internal.ParserContextImpl";
/**
* Obtain a new instance of a {@link org.sourceprojects.xmlparser.XmlParser}
* .
* Implementations that used for
* {@link org.sourceprojects.xmlparser.XmlParser} and
* {@link org.sourceprojects.xmlparser.ParserContext} are the standard
* internal ones. The classloader to load up those implementations is the
* actual ContextClassLoader of the actual thread.
*
* @param
* Type of output class to use in parsing and transforming
* @return New instance of standard XmlParser implementation
* @throws XmlParserInitializationException
* If some exception occurs on initialization of the new
* instance the exception is wrapped into a
* {@link XmlParserInitializationException}
*/
public static final XmlParser newInstance()
throws XmlParserInitializationException {
ClassLoader classLoader = Thread.currentThread()
.getContextClassLoader();
return newInstance(STANDARD_XMLPARSER_CLASS,
STANDARD_PARSERCONTEXT_CLASS, classLoader);
}
/**
* Obtain a new instance of a {@link org.sourceprojects.xmlparser.XmlParser}
* .
* Implementations that used for
* {@link org.sourceprojects.xmlparser.XmlParser} and
* {@link org.sourceprojects.xmlparser.ParserContext} are the standard
* internal ones. The classloader to load up those implementations is the
* given classloader.
*
* @param
* Type of output class to use in parsing and transforming
* @param classLoader
* The classloader to load classes
* @return New instance of standard XmlParser implementation
* @throws XmlParserInitializationException
* If some exception occurs on initialization of the new
* instance the exception is wrapped into a
* {@link XmlParserInitializationException}
*/
public static final XmlParser newInstance(ClassLoader classLoader)
throws XmlParserInitializationException {
return newInstance(STANDARD_XMLPARSER_CLASS,
STANDARD_PARSERCONTEXT_CLASS, classLoader);
}
/**
* Obtain a new instance of a {@link org.sourceprojects.xmlparser.XmlParser}
* .
* Implementations that used for
* {@link org.sourceprojects.xmlparser.XmlParser} and
* {@link org.sourceprojects.xmlparser.ParserContext} must be given as
* parameters to the method. The classloader to load up those
* implementations is the given classloader.
* {@link XmlParserInitializationException} will be thrown if one of both
* implementations is null or not found.
*
* @param
* Type of output class to use in parsing and transforming
* @param parserImplementationClassname
* Classname of the implementation used for XmlParser
* @param contextImplementationClassname
* Classname of the implementation used for ParserContext
* @param classLoader
* The classloader to load classes
* @return New instance of standard XmlParser implementation
* @throws XmlParserInitializationException
* If some exception occurs on initialization of the new
* instance the exception is wrapped into a
* {@link XmlParserInitializationException}
*/
public static final XmlParser newInstance(
String parserImplementationClassname,
String contextImplementationClassname, ClassLoader classLoader)
throws XmlParserInitializationException {
return newInstance(parserImplementationClassname,
contextImplementationClassname, classLoader, true);
}
/**
* Obtain a new instance of a {@link org.sourceprojects.xmlparser.XmlParser}
* .
* Implementations that used for
* {@link org.sourceprojects.xmlparser.XmlParser} and
* {@link org.sourceprojects.xmlparser.ParserContext} must be given as
* parameters to the method. The classloader to load up those
* implementations is the given classloader.
* {@link XmlParserInitializationException} will be thrown if one of both
* implementations is null or not found.
*
* @param
* Type of output class to use in parsing and transforming
* @param parserImplementationClassname
* Classname of the implementation used for XmlParser
* @param contextImplementationClassname
* Classname of the implementation used for ParserContext
* @param classLoader
* The classloader to load classes
* @param createResourceResolver
* Automatically create a basic ResourceResolver for the
* @return New instance of standard XmlParser implementation XmlParser
* instance
* @throws XmlParserInitializationException
* If some exception occurs on initialization of the new
* instance the exception is wrapped into a
* {@link XmlParserInitializationException}
* @since 0.0.6
*/
@SuppressWarnings("unchecked")
public static final XmlParser newInstance(
String parserImplementationClassname,
String contextImplementationClassname, ClassLoader classLoader,
boolean createResourceResolver)
throws XmlParserInitializationException {
try {
Class extends XmlParser> parserClass;
Class extends ParserContext> contextClass;
parserClass = (Class extends XmlParser>) classLoader
.loadClass(parserImplementationClassname);
contextClass = (Class extends ParserContext>) classLoader
.loadClass(contextImplementationClassname);
XmlParser parser = parserClass.newInstance();
ParserContext context = contextClass.newInstance();
parser.setParserContext(context);
if (createResourceResolver)
parser.setResourceResolver(ResourceResolverFactory
.newInstance(classLoader));
return parser;
} catch (Exception e) {
e.printStackTrace();
throw new XmlParserInitializationException(
"Parser initialization failed", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy