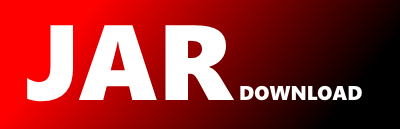
akka.remote.ContainerFormats Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ContainerFormats.proto
package akka.remote;
public final class ContainerFormats {
private ContainerFormats() {}
public static void registerAllExtensions(
org.spark_project.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code PatternType}
*/
public enum PatternType
implements org.spark_project.protobuf.ProtocolMessageEnum {
/**
* PARENT = 0;
*/
PARENT(0, 0),
/**
* CHILD_NAME = 1;
*/
CHILD_NAME(1, 1),
/**
* CHILD_PATTERN = 2;
*/
CHILD_PATTERN(2, 2),
;
/**
* PARENT = 0;
*/
public static final int PARENT_VALUE = 0;
/**
* CHILD_NAME = 1;
*/
public static final int CHILD_NAME_VALUE = 1;
/**
* CHILD_PATTERN = 2;
*/
public static final int CHILD_PATTERN_VALUE = 2;
public final int getNumber() { return value; }
public static PatternType valueOf(int value) {
switch (value) {
case 0: return PARENT;
case 1: return CHILD_NAME;
case 2: return CHILD_PATTERN;
default: return null;
}
}
public static org.spark_project.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static org.spark_project.protobuf.Internal.EnumLiteMap
internalValueMap =
new org.spark_project.protobuf.Internal.EnumLiteMap() {
public PatternType findValueByNumber(int number) {
return PatternType.valueOf(number);
}
};
public final org.spark_project.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final org.spark_project.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final org.spark_project.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return akka.remote.ContainerFormats.getDescriptor().getEnumTypes().get(0);
}
private static final PatternType[] VALUES = values();
public static PatternType valueOf(
org.spark_project.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private PatternType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:PatternType)
}
public interface SelectionEnvelopeOrBuilder
extends org.spark_project.protobuf.MessageOrBuilder {
// required bytes enclosedMessage = 1;
/**
* required bytes enclosedMessage = 1;
*/
boolean hasEnclosedMessage();
/**
* required bytes enclosedMessage = 1;
*/
org.spark_project.protobuf.ByteString getEnclosedMessage();
// required int32 serializerId = 2;
/**
* required int32 serializerId = 2;
*/
boolean hasSerializerId();
/**
* required int32 serializerId = 2;
*/
int getSerializerId();
// repeated .Selection pattern = 3;
/**
* repeated .Selection pattern = 3;
*/
java.util.List
getPatternList();
/**
* repeated .Selection pattern = 3;
*/
akka.remote.ContainerFormats.Selection getPattern(int index);
/**
* repeated .Selection pattern = 3;
*/
int getPatternCount();
/**
* repeated .Selection pattern = 3;
*/
java.util.List extends akka.remote.ContainerFormats.SelectionOrBuilder>
getPatternOrBuilderList();
/**
* repeated .Selection pattern = 3;
*/
akka.remote.ContainerFormats.SelectionOrBuilder getPatternOrBuilder(
int index);
// optional bytes messageManifest = 4;
/**
* optional bytes messageManifest = 4;
*/
boolean hasMessageManifest();
/**
* optional bytes messageManifest = 4;
*/
org.spark_project.protobuf.ByteString getMessageManifest();
// optional bool wildcardFanOut = 5;
/**
* optional bool wildcardFanOut = 5;
*/
boolean hasWildcardFanOut();
/**
* optional bool wildcardFanOut = 5;
*/
boolean getWildcardFanOut();
}
/**
* Protobuf type {@code SelectionEnvelope}
*/
public static final class SelectionEnvelope extends
org.spark_project.protobuf.GeneratedMessage
implements SelectionEnvelopeOrBuilder {
// Use SelectionEnvelope.newBuilder() to construct.
private SelectionEnvelope(org.spark_project.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SelectionEnvelope(boolean noInit) { this.unknownFields = org.spark_project.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SelectionEnvelope defaultInstance;
public static SelectionEnvelope getDefaultInstance() {
return defaultInstance;
}
public SelectionEnvelope getDefaultInstanceForType() {
return defaultInstance;
}
private final org.spark_project.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final org.spark_project.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SelectionEnvelope(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
org.spark_project.protobuf.UnknownFieldSet.Builder unknownFields =
org.spark_project.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
enclosedMessage_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
serializerId_ = input.readInt32();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
pattern_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
pattern_.add(input.readMessage(akka.remote.ContainerFormats.Selection.PARSER, extensionRegistry));
break;
}
case 34: {
bitField0_ |= 0x00000004;
messageManifest_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000008;
wildcardFanOut_ = input.readBool();
break;
}
}
}
} catch (org.spark_project.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new org.spark_project.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
pattern_ = java.util.Collections.unmodifiableList(pattern_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final org.spark_project.protobuf.Descriptors.Descriptor
getDescriptor() {
return akka.remote.ContainerFormats.internal_static_SelectionEnvelope_descriptor;
}
protected org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return akka.remote.ContainerFormats.internal_static_SelectionEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
akka.remote.ContainerFormats.SelectionEnvelope.class, akka.remote.ContainerFormats.SelectionEnvelope.Builder.class);
}
public static org.spark_project.protobuf.Parser PARSER =
new org.spark_project.protobuf.AbstractParser() {
public SelectionEnvelope parsePartialFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return new SelectionEnvelope(input, extensionRegistry);
}
};
@java.lang.Override
public org.spark_project.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes enclosedMessage = 1;
public static final int ENCLOSEDMESSAGE_FIELD_NUMBER = 1;
private org.spark_project.protobuf.ByteString enclosedMessage_;
/**
* required bytes enclosedMessage = 1;
*/
public boolean hasEnclosedMessage() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes enclosedMessage = 1;
*/
public org.spark_project.protobuf.ByteString getEnclosedMessage() {
return enclosedMessage_;
}
// required int32 serializerId = 2;
public static final int SERIALIZERID_FIELD_NUMBER = 2;
private int serializerId_;
/**
* required int32 serializerId = 2;
*/
public boolean hasSerializerId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 serializerId = 2;
*/
public int getSerializerId() {
return serializerId_;
}
// repeated .Selection pattern = 3;
public static final int PATTERN_FIELD_NUMBER = 3;
private java.util.List pattern_;
/**
* repeated .Selection pattern = 3;
*/
public java.util.List getPatternList() {
return pattern_;
}
/**
* repeated .Selection pattern = 3;
*/
public java.util.List extends akka.remote.ContainerFormats.SelectionOrBuilder>
getPatternOrBuilderList() {
return pattern_;
}
/**
* repeated .Selection pattern = 3;
*/
public int getPatternCount() {
return pattern_.size();
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.Selection getPattern(int index) {
return pattern_.get(index);
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.SelectionOrBuilder getPatternOrBuilder(
int index) {
return pattern_.get(index);
}
// optional bytes messageManifest = 4;
public static final int MESSAGEMANIFEST_FIELD_NUMBER = 4;
private org.spark_project.protobuf.ByteString messageManifest_;
/**
* optional bytes messageManifest = 4;
*/
public boolean hasMessageManifest() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes messageManifest = 4;
*/
public org.spark_project.protobuf.ByteString getMessageManifest() {
return messageManifest_;
}
// optional bool wildcardFanOut = 5;
public static final int WILDCARDFANOUT_FIELD_NUMBER = 5;
private boolean wildcardFanOut_;
/**
* optional bool wildcardFanOut = 5;
*/
public boolean hasWildcardFanOut() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool wildcardFanOut = 5;
*/
public boolean getWildcardFanOut() {
return wildcardFanOut_;
}
private void initFields() {
enclosedMessage_ = org.spark_project.protobuf.ByteString.EMPTY;
serializerId_ = 0;
pattern_ = java.util.Collections.emptyList();
messageManifest_ = org.spark_project.protobuf.ByteString.EMPTY;
wildcardFanOut_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasEnclosedMessage()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSerializerId()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getPatternCount(); i++) {
if (!getPattern(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(org.spark_project.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, enclosedMessage_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, serializerId_);
}
for (int i = 0; i < pattern_.size(); i++) {
output.writeMessage(3, pattern_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(4, messageManifest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, wildcardFanOut_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeBytesSize(1, enclosedMessage_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeInt32Size(2, serializerId_);
}
for (int i = 0; i < pattern_.size(); i++) {
size += org.spark_project.protobuf.CodedOutputStream
.computeMessageSize(3, pattern_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeBytesSize(4, messageManifest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeBoolSize(5, wildcardFanOut_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
org.spark_project.protobuf.ByteString data)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
org.spark_project.protobuf.ByteString data,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(byte[] data)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
byte[] data,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
java.io.InputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseDelimitedFrom(
java.io.InputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
org.spark_project.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static akka.remote.ContainerFormats.SelectionEnvelope parseFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(akka.remote.ContainerFormats.SelectionEnvelope prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
org.spark_project.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code SelectionEnvelope}
*/
public static final class Builder extends
org.spark_project.protobuf.GeneratedMessage.Builder
implements akka.remote.ContainerFormats.SelectionEnvelopeOrBuilder {
public static final org.spark_project.protobuf.Descriptors.Descriptor
getDescriptor() {
return akka.remote.ContainerFormats.internal_static_SelectionEnvelope_descriptor;
}
protected org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return akka.remote.ContainerFormats.internal_static_SelectionEnvelope_fieldAccessorTable
.ensureFieldAccessorsInitialized(
akka.remote.ContainerFormats.SelectionEnvelope.class, akka.remote.ContainerFormats.SelectionEnvelope.Builder.class);
}
// Construct using akka.remote.ContainerFormats.SelectionEnvelope.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
org.spark_project.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (org.spark_project.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPatternFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
enclosedMessage_ = org.spark_project.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
serializerId_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
if (patternBuilder_ == null) {
pattern_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
patternBuilder_.clear();
}
messageManifest_ = org.spark_project.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
wildcardFanOut_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.spark_project.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return akka.remote.ContainerFormats.internal_static_SelectionEnvelope_descriptor;
}
public akka.remote.ContainerFormats.SelectionEnvelope getDefaultInstanceForType() {
return akka.remote.ContainerFormats.SelectionEnvelope.getDefaultInstance();
}
public akka.remote.ContainerFormats.SelectionEnvelope build() {
akka.remote.ContainerFormats.SelectionEnvelope result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public akka.remote.ContainerFormats.SelectionEnvelope buildPartial() {
akka.remote.ContainerFormats.SelectionEnvelope result = new akka.remote.ContainerFormats.SelectionEnvelope(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.enclosedMessage_ = enclosedMessage_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serializerId_ = serializerId_;
if (patternBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
pattern_ = java.util.Collections.unmodifiableList(pattern_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.pattern_ = pattern_;
} else {
result.pattern_ = patternBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.messageManifest_ = messageManifest_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.wildcardFanOut_ = wildcardFanOut_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(org.spark_project.protobuf.Message other) {
if (other instanceof akka.remote.ContainerFormats.SelectionEnvelope) {
return mergeFrom((akka.remote.ContainerFormats.SelectionEnvelope)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(akka.remote.ContainerFormats.SelectionEnvelope other) {
if (other == akka.remote.ContainerFormats.SelectionEnvelope.getDefaultInstance()) return this;
if (other.hasEnclosedMessage()) {
setEnclosedMessage(other.getEnclosedMessage());
}
if (other.hasSerializerId()) {
setSerializerId(other.getSerializerId());
}
if (patternBuilder_ == null) {
if (!other.pattern_.isEmpty()) {
if (pattern_.isEmpty()) {
pattern_ = other.pattern_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePatternIsMutable();
pattern_.addAll(other.pattern_);
}
onChanged();
}
} else {
if (!other.pattern_.isEmpty()) {
if (patternBuilder_.isEmpty()) {
patternBuilder_.dispose();
patternBuilder_ = null;
pattern_ = other.pattern_;
bitField0_ = (bitField0_ & ~0x00000004);
patternBuilder_ =
org.spark_project.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPatternFieldBuilder() : null;
} else {
patternBuilder_.addAllMessages(other.pattern_);
}
}
}
if (other.hasMessageManifest()) {
setMessageManifest(other.getMessageManifest());
}
if (other.hasWildcardFanOut()) {
setWildcardFanOut(other.getWildcardFanOut());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasEnclosedMessage()) {
return false;
}
if (!hasSerializerId()) {
return false;
}
for (int i = 0; i < getPatternCount(); i++) {
if (!getPattern(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
akka.remote.ContainerFormats.SelectionEnvelope parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (org.spark_project.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (akka.remote.ContainerFormats.SelectionEnvelope) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes enclosedMessage = 1;
private org.spark_project.protobuf.ByteString enclosedMessage_ = org.spark_project.protobuf.ByteString.EMPTY;
/**
* required bytes enclosedMessage = 1;
*/
public boolean hasEnclosedMessage() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes enclosedMessage = 1;
*/
public org.spark_project.protobuf.ByteString getEnclosedMessage() {
return enclosedMessage_;
}
/**
* required bytes enclosedMessage = 1;
*/
public Builder setEnclosedMessage(org.spark_project.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
enclosedMessage_ = value;
onChanged();
return this;
}
/**
* required bytes enclosedMessage = 1;
*/
public Builder clearEnclosedMessage() {
bitField0_ = (bitField0_ & ~0x00000001);
enclosedMessage_ = getDefaultInstance().getEnclosedMessage();
onChanged();
return this;
}
// required int32 serializerId = 2;
private int serializerId_ ;
/**
* required int32 serializerId = 2;
*/
public boolean hasSerializerId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 serializerId = 2;
*/
public int getSerializerId() {
return serializerId_;
}
/**
* required int32 serializerId = 2;
*/
public Builder setSerializerId(int value) {
bitField0_ |= 0x00000002;
serializerId_ = value;
onChanged();
return this;
}
/**
* required int32 serializerId = 2;
*/
public Builder clearSerializerId() {
bitField0_ = (bitField0_ & ~0x00000002);
serializerId_ = 0;
onChanged();
return this;
}
// repeated .Selection pattern = 3;
private java.util.List pattern_ =
java.util.Collections.emptyList();
private void ensurePatternIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
pattern_ = new java.util.ArrayList(pattern_);
bitField0_ |= 0x00000004;
}
}
private org.spark_project.protobuf.RepeatedFieldBuilder<
akka.remote.ContainerFormats.Selection, akka.remote.ContainerFormats.Selection.Builder, akka.remote.ContainerFormats.SelectionOrBuilder> patternBuilder_;
/**
* repeated .Selection pattern = 3;
*/
public java.util.List getPatternList() {
if (patternBuilder_ == null) {
return java.util.Collections.unmodifiableList(pattern_);
} else {
return patternBuilder_.getMessageList();
}
}
/**
* repeated .Selection pattern = 3;
*/
public int getPatternCount() {
if (patternBuilder_ == null) {
return pattern_.size();
} else {
return patternBuilder_.getCount();
}
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.Selection getPattern(int index) {
if (patternBuilder_ == null) {
return pattern_.get(index);
} else {
return patternBuilder_.getMessage(index);
}
}
/**
* repeated .Selection pattern = 3;
*/
public Builder setPattern(
int index, akka.remote.ContainerFormats.Selection value) {
if (patternBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatternIsMutable();
pattern_.set(index, value);
onChanged();
} else {
patternBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder setPattern(
int index, akka.remote.ContainerFormats.Selection.Builder builderForValue) {
if (patternBuilder_ == null) {
ensurePatternIsMutable();
pattern_.set(index, builderForValue.build());
onChanged();
} else {
patternBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder addPattern(akka.remote.ContainerFormats.Selection value) {
if (patternBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatternIsMutable();
pattern_.add(value);
onChanged();
} else {
patternBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder addPattern(
int index, akka.remote.ContainerFormats.Selection value) {
if (patternBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatternIsMutable();
pattern_.add(index, value);
onChanged();
} else {
patternBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder addPattern(
akka.remote.ContainerFormats.Selection.Builder builderForValue) {
if (patternBuilder_ == null) {
ensurePatternIsMutable();
pattern_.add(builderForValue.build());
onChanged();
} else {
patternBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder addPattern(
int index, akka.remote.ContainerFormats.Selection.Builder builderForValue) {
if (patternBuilder_ == null) {
ensurePatternIsMutable();
pattern_.add(index, builderForValue.build());
onChanged();
} else {
patternBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder addAllPattern(
java.lang.Iterable extends akka.remote.ContainerFormats.Selection> values) {
if (patternBuilder_ == null) {
ensurePatternIsMutable();
super.addAll(values, pattern_);
onChanged();
} else {
patternBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder clearPattern() {
if (patternBuilder_ == null) {
pattern_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
patternBuilder_.clear();
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public Builder removePattern(int index) {
if (patternBuilder_ == null) {
ensurePatternIsMutable();
pattern_.remove(index);
onChanged();
} else {
patternBuilder_.remove(index);
}
return this;
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.Selection.Builder getPatternBuilder(
int index) {
return getPatternFieldBuilder().getBuilder(index);
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.SelectionOrBuilder getPatternOrBuilder(
int index) {
if (patternBuilder_ == null) {
return pattern_.get(index); } else {
return patternBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Selection pattern = 3;
*/
public java.util.List extends akka.remote.ContainerFormats.SelectionOrBuilder>
getPatternOrBuilderList() {
if (patternBuilder_ != null) {
return patternBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pattern_);
}
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.Selection.Builder addPatternBuilder() {
return getPatternFieldBuilder().addBuilder(
akka.remote.ContainerFormats.Selection.getDefaultInstance());
}
/**
* repeated .Selection pattern = 3;
*/
public akka.remote.ContainerFormats.Selection.Builder addPatternBuilder(
int index) {
return getPatternFieldBuilder().addBuilder(
index, akka.remote.ContainerFormats.Selection.getDefaultInstance());
}
/**
* repeated .Selection pattern = 3;
*/
public java.util.List
getPatternBuilderList() {
return getPatternFieldBuilder().getBuilderList();
}
private org.spark_project.protobuf.RepeatedFieldBuilder<
akka.remote.ContainerFormats.Selection, akka.remote.ContainerFormats.Selection.Builder, akka.remote.ContainerFormats.SelectionOrBuilder>
getPatternFieldBuilder() {
if (patternBuilder_ == null) {
patternBuilder_ = new org.spark_project.protobuf.RepeatedFieldBuilder<
akka.remote.ContainerFormats.Selection, akka.remote.ContainerFormats.Selection.Builder, akka.remote.ContainerFormats.SelectionOrBuilder>(
pattern_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
pattern_ = null;
}
return patternBuilder_;
}
// optional bytes messageManifest = 4;
private org.spark_project.protobuf.ByteString messageManifest_ = org.spark_project.protobuf.ByteString.EMPTY;
/**
* optional bytes messageManifest = 4;
*/
public boolean hasMessageManifest() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes messageManifest = 4;
*/
public org.spark_project.protobuf.ByteString getMessageManifest() {
return messageManifest_;
}
/**
* optional bytes messageManifest = 4;
*/
public Builder setMessageManifest(org.spark_project.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
messageManifest_ = value;
onChanged();
return this;
}
/**
* optional bytes messageManifest = 4;
*/
public Builder clearMessageManifest() {
bitField0_ = (bitField0_ & ~0x00000008);
messageManifest_ = getDefaultInstance().getMessageManifest();
onChanged();
return this;
}
// optional bool wildcardFanOut = 5;
private boolean wildcardFanOut_ ;
/**
* optional bool wildcardFanOut = 5;
*/
public boolean hasWildcardFanOut() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool wildcardFanOut = 5;
*/
public boolean getWildcardFanOut() {
return wildcardFanOut_;
}
/**
* optional bool wildcardFanOut = 5;
*/
public Builder setWildcardFanOut(boolean value) {
bitField0_ |= 0x00000010;
wildcardFanOut_ = value;
onChanged();
return this;
}
/**
* optional bool wildcardFanOut = 5;
*/
public Builder clearWildcardFanOut() {
bitField0_ = (bitField0_ & ~0x00000010);
wildcardFanOut_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:SelectionEnvelope)
}
static {
defaultInstance = new SelectionEnvelope(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:SelectionEnvelope)
}
public interface SelectionOrBuilder
extends org.spark_project.protobuf.MessageOrBuilder {
// required .PatternType type = 1;
/**
* required .PatternType type = 1;
*/
boolean hasType();
/**
* required .PatternType type = 1;
*/
akka.remote.ContainerFormats.PatternType getType();
// optional string matcher = 2;
/**
* optional string matcher = 2;
*/
boolean hasMatcher();
/**
* optional string matcher = 2;
*/
java.lang.String getMatcher();
/**
* optional string matcher = 2;
*/
org.spark_project.protobuf.ByteString
getMatcherBytes();
}
/**
* Protobuf type {@code Selection}
*/
public static final class Selection extends
org.spark_project.protobuf.GeneratedMessage
implements SelectionOrBuilder {
// Use Selection.newBuilder() to construct.
private Selection(org.spark_project.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Selection(boolean noInit) { this.unknownFields = org.spark_project.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Selection defaultInstance;
public static Selection getDefaultInstance() {
return defaultInstance;
}
public Selection getDefaultInstanceForType() {
return defaultInstance;
}
private final org.spark_project.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final org.spark_project.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Selection(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
org.spark_project.protobuf.UnknownFieldSet.Builder unknownFields =
org.spark_project.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
akka.remote.ContainerFormats.PatternType value = akka.remote.ContainerFormats.PatternType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = value;
}
break;
}
case 18: {
bitField0_ |= 0x00000002;
matcher_ = input.readBytes();
break;
}
}
}
} catch (org.spark_project.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new org.spark_project.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final org.spark_project.protobuf.Descriptors.Descriptor
getDescriptor() {
return akka.remote.ContainerFormats.internal_static_Selection_descriptor;
}
protected org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return akka.remote.ContainerFormats.internal_static_Selection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
akka.remote.ContainerFormats.Selection.class, akka.remote.ContainerFormats.Selection.Builder.class);
}
public static org.spark_project.protobuf.Parser PARSER =
new org.spark_project.protobuf.AbstractParser() {
public Selection parsePartialFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return new Selection(input, extensionRegistry);
}
};
@java.lang.Override
public org.spark_project.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .PatternType type = 1;
public static final int TYPE_FIELD_NUMBER = 1;
private akka.remote.ContainerFormats.PatternType type_;
/**
* required .PatternType type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .PatternType type = 1;
*/
public akka.remote.ContainerFormats.PatternType getType() {
return type_;
}
// optional string matcher = 2;
public static final int MATCHER_FIELD_NUMBER = 2;
private java.lang.Object matcher_;
/**
* optional string matcher = 2;
*/
public boolean hasMatcher() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string matcher = 2;
*/
public java.lang.String getMatcher() {
java.lang.Object ref = matcher_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
org.spark_project.protobuf.ByteString bs =
(org.spark_project.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
matcher_ = s;
}
return s;
}
}
/**
* optional string matcher = 2;
*/
public org.spark_project.protobuf.ByteString
getMatcherBytes() {
java.lang.Object ref = matcher_;
if (ref instanceof java.lang.String) {
org.spark_project.protobuf.ByteString b =
org.spark_project.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
matcher_ = b;
return b;
} else {
return (org.spark_project.protobuf.ByteString) ref;
}
}
private void initFields() {
type_ = akka.remote.ContainerFormats.PatternType.PARENT;
matcher_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(org.spark_project.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getMatcherBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeEnumSize(1, type_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += org.spark_project.protobuf.CodedOutputStream
.computeBytesSize(2, getMatcherBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static akka.remote.ContainerFormats.Selection parseFrom(
org.spark_project.protobuf.ByteString data)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static akka.remote.ContainerFormats.Selection parseFrom(
org.spark_project.protobuf.ByteString data,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static akka.remote.ContainerFormats.Selection parseFrom(byte[] data)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static akka.remote.ContainerFormats.Selection parseFrom(
byte[] data,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws org.spark_project.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static akka.remote.ContainerFormats.Selection parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static akka.remote.ContainerFormats.Selection parseFrom(
java.io.InputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static akka.remote.ContainerFormats.Selection parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static akka.remote.ContainerFormats.Selection parseDelimitedFrom(
java.io.InputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static akka.remote.ContainerFormats.Selection parseFrom(
org.spark_project.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static akka.remote.ContainerFormats.Selection parseFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(akka.remote.ContainerFormats.Selection prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
org.spark_project.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Selection}
*/
public static final class Builder extends
org.spark_project.protobuf.GeneratedMessage.Builder
implements akka.remote.ContainerFormats.SelectionOrBuilder {
public static final org.spark_project.protobuf.Descriptors.Descriptor
getDescriptor() {
return akka.remote.ContainerFormats.internal_static_Selection_descriptor;
}
protected org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return akka.remote.ContainerFormats.internal_static_Selection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
akka.remote.ContainerFormats.Selection.class, akka.remote.ContainerFormats.Selection.Builder.class);
}
// Construct using akka.remote.ContainerFormats.Selection.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
org.spark_project.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (org.spark_project.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
type_ = akka.remote.ContainerFormats.PatternType.PARENT;
bitField0_ = (bitField0_ & ~0x00000001);
matcher_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.spark_project.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return akka.remote.ContainerFormats.internal_static_Selection_descriptor;
}
public akka.remote.ContainerFormats.Selection getDefaultInstanceForType() {
return akka.remote.ContainerFormats.Selection.getDefaultInstance();
}
public akka.remote.ContainerFormats.Selection build() {
akka.remote.ContainerFormats.Selection result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public akka.remote.ContainerFormats.Selection buildPartial() {
akka.remote.ContainerFormats.Selection result = new akka.remote.ContainerFormats.Selection(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.matcher_ = matcher_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(org.spark_project.protobuf.Message other) {
if (other instanceof akka.remote.ContainerFormats.Selection) {
return mergeFrom((akka.remote.ContainerFormats.Selection)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(akka.remote.ContainerFormats.Selection other) {
if (other == akka.remote.ContainerFormats.Selection.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasMatcher()) {
bitField0_ |= 0x00000002;
matcher_ = other.matcher_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
return true;
}
public Builder mergeFrom(
org.spark_project.protobuf.CodedInputStream input,
org.spark_project.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
akka.remote.ContainerFormats.Selection parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (org.spark_project.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (akka.remote.ContainerFormats.Selection) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .PatternType type = 1;
private akka.remote.ContainerFormats.PatternType type_ = akka.remote.ContainerFormats.PatternType.PARENT;
/**
* required .PatternType type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .PatternType type = 1;
*/
public akka.remote.ContainerFormats.PatternType getType() {
return type_;
}
/**
* required .PatternType type = 1;
*/
public Builder setType(akka.remote.ContainerFormats.PatternType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value;
onChanged();
return this;
}
/**
* required .PatternType type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = akka.remote.ContainerFormats.PatternType.PARENT;
onChanged();
return this;
}
// optional string matcher = 2;
private java.lang.Object matcher_ = "";
/**
* optional string matcher = 2;
*/
public boolean hasMatcher() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string matcher = 2;
*/
public java.lang.String getMatcher() {
java.lang.Object ref = matcher_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((org.spark_project.protobuf.ByteString) ref)
.toStringUtf8();
matcher_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string matcher = 2;
*/
public org.spark_project.protobuf.ByteString
getMatcherBytes() {
java.lang.Object ref = matcher_;
if (ref instanceof String) {
org.spark_project.protobuf.ByteString b =
org.spark_project.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
matcher_ = b;
return b;
} else {
return (org.spark_project.protobuf.ByteString) ref;
}
}
/**
* optional string matcher = 2;
*/
public Builder setMatcher(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
matcher_ = value;
onChanged();
return this;
}
/**
* optional string matcher = 2;
*/
public Builder clearMatcher() {
bitField0_ = (bitField0_ & ~0x00000002);
matcher_ = getDefaultInstance().getMatcher();
onChanged();
return this;
}
/**
* optional string matcher = 2;
*/
public Builder setMatcherBytes(
org.spark_project.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
matcher_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Selection)
}
static {
defaultInstance = new Selection(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Selection)
}
private static org.spark_project.protobuf.Descriptors.Descriptor
internal_static_SelectionEnvelope_descriptor;
private static
org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_SelectionEnvelope_fieldAccessorTable;
private static org.spark_project.protobuf.Descriptors.Descriptor
internal_static_Selection_descriptor;
private static
org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Selection_fieldAccessorTable;
public static org.spark_project.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static org.spark_project.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\026ContainerFormats.proto\"\220\001\n\021SelectionEn" +
"velope\022\027\n\017enclosedMessage\030\001 \002(\014\022\024\n\014seria" +
"lizerId\030\002 \002(\005\022\033\n\007pattern\030\003 \003(\0132\n.Selecti" +
"on\022\027\n\017messageManifest\030\004 \001(\014\022\026\n\016wildcardF" +
"anOut\030\005 \001(\010\"8\n\tSelection\022\032\n\004type\030\001 \002(\0162\014" +
".PatternType\022\017\n\007matcher\030\002 \001(\t*<\n\013Pattern" +
"Type\022\n\n\006PARENT\020\000\022\016\n\nCHILD_NAME\020\001\022\021\n\rCHIL" +
"D_PATTERN\020\002B\017\n\013akka.remoteH\001"
};
org.spark_project.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new org.spark_project.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public org.spark_project.protobuf.ExtensionRegistry assignDescriptors(
org.spark_project.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_SelectionEnvelope_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_SelectionEnvelope_fieldAccessorTable = new
org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_SelectionEnvelope_descriptor,
new java.lang.String[] { "EnclosedMessage", "SerializerId", "Pattern", "MessageManifest", "WildcardFanOut", });
internal_static_Selection_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_Selection_fieldAccessorTable = new
org.spark_project.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Selection_descriptor,
new java.lang.String[] { "Type", "Matcher", });
return null;
}
};
org.spark_project.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new org.spark_project.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy