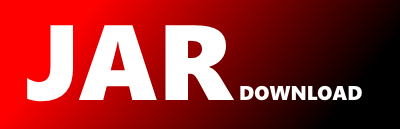
org.spdx.spdx_to_osv.osvmodel.OsvVulnerabilityRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spdx-to-osv Show documentation
Show all versions of spdx-to-osv Show documentation
Produces an OSV vulnerability JSON report from an SPDX file
The newest version!
/**
* SPDX-License-Identifier: Apache-2.0
* Copyright (c) 2021 Source Auditor Inc.
*/
package org.spdx.spdx_to_osv.osvmodel;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
/**
* OSV package and version or commit information
* Matches the format for the Query Vulnerability request as specified at https://osv.dev/docs/#operation/OSV_QueryAffected
*
* @author Gary O'Neall
*/
public class OsvVulnerabilityRequest {
/**
* The package to query against. When a commit hash is given, this is optional.
*/
@SerializedName(value="package")
private OsvPackage osvPackage;
/**
* he version string to query for. A fuzzy match is done against upstream versions.
* If specified, commit should not be set.
*/
private String version;
/**
* The commit hash to query for. If specified, version should not be set.
*/
private String commit;
/**
* @param name package name
* @param version package version
*/
public OsvVulnerabilityRequest(OsvPackage osvPackage, String version) {
Objects.requireNonNull(osvPackage, "Package can not be null");
this.osvPackage = osvPackage;
this.version = version;
this.commit = null;
}
public OsvVulnerabilityRequest(String commit) {
Objects.requireNonNull(commit, "Commit can not be null");
this.commit = commit;
this.osvPackage = null;
this.version = null;
}
/**
* @return the osvPackage
*/
public OsvPackage getPackage() {
return osvPackage;
}
/**
* @param osvPackage the osvPackage to set
*/
public void setPackage(OsvPackage osvPackage) {
this.osvPackage = osvPackage;
}
/**
* @return the version
*/
public String getVersion() {
return version;
}
/**
* @param version the version to set
*/
public void setVersion(String version) {
this.version = version;
}
/**
* @return the commit
*/
public String getCommit() {
return commit;
}
/**
* @param commit the commit to set
*/
public void setCommit(String commit) {
this.commit = commit;
}
@Override
public int hashCode() {
int retval = 101;
if (Objects.nonNull(osvPackage)) {
retval = retval ^ this.osvPackage.hashCode();
}
if (Objects.nonNull(version)) {
retval = retval ^ version.hashCode();
}
if (Objects.nonNull(commit)) {
retval = retval ^ commit.hashCode();
}
return retval;
}
@Override
public boolean equals(Object o) {
if (!(o instanceof OsvVulnerabilityRequest)) {
return false;
}
OsvVulnerabilityRequest compare = (OsvVulnerabilityRequest)o;
return Objects.equals(this.osvPackage, compare.getPackage()) &&
Objects.equals(this.version, compare.getVersion()) &&
Objects.equals(this.commit, compare.getCommit());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy