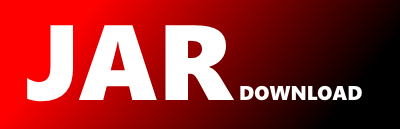
org.spf4j.failsafe.DefaultRetryPredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spf4j-core Show documentation
Show all versions of spf4j-core Show documentation
A continuously growing collection of utilities to measure performance, get better diagnostics,
improve performance, or do things more reliably, faster that other open source libraries...
/*
* Copyright 2017 SPF4J.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.spf4j.failsafe;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.Arrays;
import java.util.concurrent.Callable;
import java.util.function.Function;
import java.util.function.Supplier;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.ThreadSafe;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.spf4j.log.Level;
import org.spf4j.log.SLf4jXLogAdapter;
import org.spf4j.log.XLog;
/**
* @author Zoltan Farkas
*/
@SuppressFBWarnings("AI_ANNOTATION_ISSUES_NEEDS_NULLABLE") // false positive...
@ThreadSafe
final class DefaultRetryPredicate implements RetryPredicate> {
private static final Level RETRY_LOG_LEVEL =
Level.valueOf(System.getProperty("spf4j.failsafe.retryLogLevel", "WARN"));
private static final PartialResultRetryPredicate[] NO_RP = new PartialResultRetryPredicate[0];
private static final PartialExceptionRetryPredicate[] NO_EP = new PartialExceptionRetryPredicate[0];
private static final Logger LOG = LoggerFactory.getLogger(DefaultRetryPredicate.class);
private final Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy