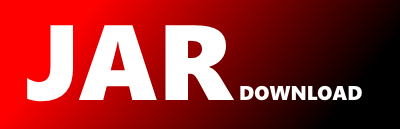
org.spf4j.io.Csv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spf4j-core Show documentation
Show all versions of spf4j-core Show documentation
A continuously growing collection of utilities to measure performance, get better diagnostics,
improve performance, or do things more reliably, faster that other open source libraries...
/*
* Copyright (c) 2001-2017, Zoltan Farkas All Rights Reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*
* Additionally licensed with:
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.spf4j.io;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.io.File;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.io.UncheckedIOException;
import java.nio.charset.Charset;
import java.util.List;
import java.util.Map;
import javax.annotation.CheckReturnValue;
import javax.annotation.ParametersAreNonnullByDefault;
import org.spf4j.base.CharSequences;
import org.spf4j.io.csv.CharSeparatedValues;
import org.spf4j.io.csv.CsvParseException;
import org.spf4j.io.csv.CsvReader;
/**
* Supports CSV format as described at: https://en.wikipedia.org/wiki/Comma-separated_values. either of \n \r or \r\n
* are valid end of line delimiters
*
* why another implementation? because I need one that is as fast as possible, and as flexible as possible.
*
* @author zoly
*/
@ParametersAreNonnullByDefault
@SuppressFBWarnings("NP_LOAD_OF_KNOWN_NULL_VALUE") // FB gets it wrong here
public final class Csv {
public static final CharSeparatedValues CSV = new CharSeparatedValues(',');
private static final char[] TO_ESCAPE = new char[]{',', '\n', '\r', '"'};
private Csv() {
}
public interface CsvHandler extends org.spf4j.io.csv.CsvHandler {
}
public interface CsvRowHandler extends org.spf4j.io.csv.CsvRowHandler {
}
public interface CsvMapHandler extends org.spf4j.io.csv.CsvMapHandler {
}
public static String[] readSystemProperty(final String propertyName, final String... defaults) {
String propertyVal = System.getProperty(propertyName);
if (propertyVal == null) {
return defaults;
} else {
List row;
try {
row = readRow(propertyVal);
} catch (CsvParseException ex) {
throw new RuntimeException("Unable to parser property " + propertyName + " = " + propertyVal, ex);
}
return row.toArray(new String[row.size()]);
}
}
public static void writeCsvRow(final Appendable writer, final Object... elems) throws IOException {
CSV.writeCsvRow(writer, elems);
}
public static void writeCsvRow2(final Appendable writer, final Object obj, final Object... elems)
throws IOException {
CSV.writeCsvRow2(writer, obj, elems);
}
public static void writeCsvRow(final Appendable writer, final long... elems) throws IOException {
CSV.writeCsvRow(writer, elems);
}
public static void writeCsvRowNoEOL(final long[] elems, final Appendable writer) throws IOException {
CSV.writeCsvRowNoEOL(elems, writer);
}
public static void writeCsvRow(final Appendable writer, final Iterable> elems) throws IOException {
CSV.writeCsvRow(writer, elems);
}
public static void writeCsvRowNoEOL(final Iterable> elems, final Appendable writer) throws IOException {
CSV.writeCsvRowNoEOL(elems, writer);
}
public static T read(final File file, final Charset charset,
final CsvMapHandler handler) throws IOException, CsvParseException {
return CSV.read(file, charset, handler);
}
public static T read(final File file, final Charset charset,
final CsvHandler handler) throws IOException, CsvParseException {
return CSV.read(file, charset, handler);
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy