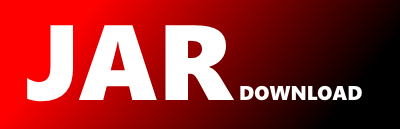
org.spf4j.concurrent.ThreadLocalBufferedConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spf4j-core Show documentation
Show all versions of spf4j-core Show documentation
A continuously growing collection of utilities to measure performance, get better diagnostics,
improve performance, or do things more reliably, faster that other open source libraries...
/*
* Copyright (c) 2001-2017, Zoltan Farkas All Rights Reserved.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*
* Additionally licensed with:
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.spf4j.concurrent;
import com.google.common.annotations.Beta;
import java.io.Closeable;
import java.io.Flushable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import java.util.function.Consumer;
/**
*
* @author zoly
*/
@Beta
public final class ThreadLocalBufferedConsumer implements Flushable, Closeable {
private final ThreadLocal> localBuffer;
private final Map> buffers;
private final Consumer> consumer;
private final int localSize;
private final ScheduledFuture> schedule;
public ThreadLocalBufferedConsumer(final int localSize, final Consumer> consumer, final int delayMillis) {
this.localSize = localSize;
buffers = new ConcurrentHashMap<>();
this.consumer = consumer;
localBuffer = new ThreadLocal>() {
@Override
protected List initialValue() {
List result = new ArrayList<>(localSize);
buffers.put(Thread.currentThread(), result);
return result;
}
};
this.schedule = DefaultScheduler.INSTANCE.scheduleWithFixedDelay(this::flush,
delayMillis, delayMillis, TimeUnit.MILLISECONDS);
}
public void write(final T value) {
List lb = localBuffer.get();
synchronized (lb) {
if (lb.size() >= localSize) {
this.consumer.accept(lb);
lb.clear();
}
lb.add(value);
}
}
@Override
public void flush() {
Iterator>> iterator = buffers.entrySet().iterator();
List toWrite = new ArrayList<>(localSize);
while (iterator.hasNext()) {
Map.Entry> entry = iterator.next();
Thread thread = entry.getKey();
if (!thread.isAlive()) {
iterator.remove();
}
List lb = entry.getValue();
synchronized (lb) {
if (!lb.isEmpty()) {
toWrite.addAll(lb);
}
lb.clear();
}
}
this.consumer.accept(toWrite);
}
@Override
public synchronized void close() {
if (!schedule.isCancelled()) {
schedule.cancel(false);
flush();
}
}
@Override
public String toString() {
return "ThreadLocalBufferedConsumer{ consumer=" + consumer + ", localSize="
+ localSize + ", schedule=" + schedule + '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy