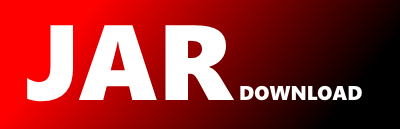
templates.plugins.spincast-http-client.spincast-http-client.html Maven / Gradle / Ivy
Show all versions of spincast-website Show documentation
{% extends "../../layout.html" %}
{% block sectionClasses %}plugins hasBreadCrumb plugins-spincast-http-client{% endblock %}
{% block meta_title %}Plugins - Spincast HTTP Client{% endblock %}
{% block meta_description %}Client to easily create and send HTTP requests.{% endblock %}
{% block scripts %}
{% endblock %}
{% block body %}
Overview
Provides an easy way of creating and sending HTTP requests. The interface to inject to
get a factory to create requests is
HttpClient
Please note that this is the light version of the Spincast HTTP client,
without support for WebSockets
. If you want your client to be able
to establish WebSocket connections, use the
Spincast HTTP Client With WebSocket version!
This client is built around
Apache HttpClient. Note that
no extra dependencies are added by this plugin because it uses the
shaded version of Apache HttpClient, which is already included in the spincast-core
artifact.
All the integration tests of Spincast use this HTTP client so
have a look at them
for a lot of examples!
Usage
To use Spincast's HTTP Client, you can inject HttpClient
where you need it.
This object is a factory : it is thread safe and can be injected in multiple
classes.
public class AppController {
private final HttpClient httpClient;
@Inject
public AppController(HttpClient httpClient) {
this.httpClient = httpClient;
}
protected HttpClient httpClient() {
return this.httpClient;
}
public void myRouteHandler(AppRequestContext context) {
HttpResponse response = httpClient().GET("https://www.google.com").send();
if(response.getStatus() == HttpStatus.SC_OK) {
//...
}
}
}
Some examples :
-
Simple
GET
request. We get the response as a string and print it :
HttpResponse response = httpClient.GET("https://www.google.com").send();
System.out.println(response.getContentAsString());
-
Complexe
POST
request. We send a Json
object representing
a user, with some extra HTTP headers :
User user = getUser();
HttpResponse response = httpClient.POST("https://example.com/api/users")
.setJsonStringBody(user)
.setCookie("cookieKey", "cookieValue")
.setHeader("headerKey", "headerValue")
.send();
if(response.getStatus() == 200) { // or use : HttpStatus.SC_OK
String content = response.getContentAsString();
Cookie cookie = response.getCookie("someCookie");
String header = response.getHeaderFirst("someHeader");
} else {
//...
}
-
Make a request to an API that returns
Json
and
use a JsonObject to represent the response :
String url = "http://example.com/some-api?format=json";
JsonObject apiResponse = httpClient.GET(url).send().getContentAsJsonObject();
Some features
There are a lot of features provided by this client, and the best way to discover all of them
is to read the javadoc of
the various components involved.
Here are some interesting ones:
-
HttpRequestBuilder<T> disableSslCertificateErrors()
The disableSslCertificateErrors()
method lets you easily disable any SSL certificates
validation. For example, this can be used to
test a local API served using a self-signed certificate
.
-
HttpRequestBuilder<T> disableRedirectHandling()
The disableRedirectHandling()
method prevents the client to automatically follow redirects (which it does by default).
-
HttpRequestBuilder<T> setHttpAuthCredentials()
The setHttpAuthCredentials()
method lets you easily set HTTP basic authentication
credentials.
Installation
1. Add this Maven artifact to your project:
<dependency>
<groupId>org.spincast</groupId>
<artifactId>spincast-plugins-http-client</artifactId>
<version>{{spincast.spincastCurrrentVersion}}</version>
</dependency>
2. Add an instance of the SpincastHttpClientPlugin
plugin to your Spincast Bootstrapper:
{% verbatim %}
Spincast.configure()
.plugin(new SpincastHttpClientPlugin())
// ...
{% endverbatim %}
Plugin class
The class implementing the SpincastPlugin
interface is SpincastHttpClientPlugin.
Suggested add-on
-
Name :
httpClient()
-
Component : HttpClient
-
Usage : to be able to make HTTP requests easily from your
Route Handlers
.
Example :
public void myRouteHandler(DefaultRequestContext context) {
// Make to request to an API and get the response as
// a JsonObject
String url = "https://example.com/api/test";
JsonObject response = context.httpClient().GET(url).send().getContentAsJsonObject();
//...
}
Learn how to install an add-on in the dedicated section of the
documentation.
Javadoc
{% endblock %}