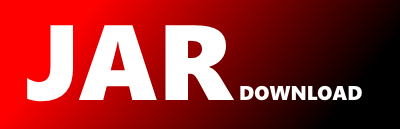
templates.demos.helloWorld.better.html Maven / Gradle / Ivy
Show all versions of spincast-website Show documentation
{% extends "../helloWorld.html" %}
{% block demoSectionClasses %}demo_hello_world{% endblock %}
{% block meta_title %}Demo - Hello World - Better{% endblock %}
{% block meta_description %}"Hello World!" demo and tutorial using Spincast - Better version{% endblock %}
{% set demoId = "better" %}
{% block demoBody %}
2. Better version
In general, you want to create a custom Guice module for your application, so you can bind custom components.
This is what we are going to do in this tutorial.
We will also create a controller so our Route Handlers
are not defined inline but have their
own dedicated method.
First, we add the latest version of the org.spincast:spincast-default
Maven artifact to our pom.xml
(or build.gradle
) :
<dependency>
<groupId>org.spincast</groupId>
<artifactId>spincast-default</artifactId>
<version>{{spincast.spincastCurrrentVersion}}</version>
</dependency>
Let's then create our controller :
public class AppController {
public void index(DefaultRequestContext context) {
JsonObject user = context.json().create();
user.set("name", "Stromgol");
user.set("age", 42);
context.response().sendJson(user);
}
}
This controller contains one Route Handler
: "index". A
Route Handler
is a method that receives, as a parameter, an object implementing
RequestContext.
In this simple handler, we create a "user" object as a JsonObject
and we send it as "application/json"
.
Now, let's create a custom Guice module, so we can bind our components :
public class AppModule extends SpincastGuiceModuleBase {
@Override
protected void configure() {
// Binds our controller
bind(AppController.class).in(Scopes.SINGLETON);
// ... binds all our other application components here
}
}
Finally, let's bootstrap and start the application! We do that in the standard main(...)
method, using the Bootstrapper :
public class App {
public static void main(String[] args) {
Spincast.configure()
.module(new AppModule())
.init(args);
}
@Inject
protected void init(Server server, DefaultRouter router, AppController ctrl) {
router.GET("/").handle(ctrl::index);
server.start();
}
}
Explanation :
-
3 : A standard
main(...)
method is the entry point of our application.
-
4 :
Spincast.configure()
starts the bootstrapper, so we can configure and initialize our application.
-
5 : We add our custom module
to the Guice context!
-
6 : The
init()
method will create the Guice
context using the default plugins and our custom module, will bind the current
App
class in the context and will load it.
-
9-10 : This init method
will be called automatically when the Guice context is ready. We inject in it the
default Router, the
default Server and our
controller.
-
11 : We define a
Route
for the index page
using a method reference targeting our controller's "index" method as
the Route Handler
. Spincast is going to call this method each time a GET
request
for the index page is received.
-
12 : We start the server.
Try it!
Download this application, and run it by yourself :
-
Download spincast-demos-better.zip.
-
Unzip the file.
-
Enter the root directory using a terminal.
-
Compile the application using Maven :
mvn clean package
-
Launch the application :
java -jar target/spincast-demos-better-{{spincast.spincastCurrrentVersion}}.jar
The application is then accessible at http://localhost:44419
Too simple for you?
Try the Supercalifragilisticexpialidocious "Hello World!" demo, for an improved version!
Too complex?
Try the Quick "Hello World!" demo, for an easier version!
{% endblock %}