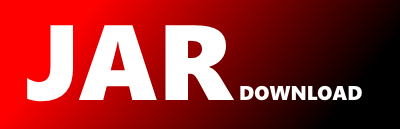
spire.example.mandelbrot.scala Maven / Gradle / Ivy
The newest version!
package spire.example
import spire.implicits._
import spire.math._
import scala.annotation.tailrec
object MandelbrotDemo {
/**
* Compute whether the complex number c stays contained within a radius-2
* circle after 'limit' iterations.
*/
def mandelbrot(c: Complex[Double], limit: Int): Int = {
@tailrec def loop(z: Complex[Double], n: Int): Int =
if (n >= limit) n
else if (z.abs > 2.0) n - 1
else loop(z * z + c, n + 1)
loop(c, 1)
}
/**
* Print an ASCII approximation of the 4x4 box from -2-2i to 2+2i.
*/
def main(args: Array[String]) {
val res = 26 // number of iterations to try before including pt in the set
val rows = if (args.isEmpty) 20 else args(0).toInt // rows to print
val cols = rows * 2 // cols to print. most fonts are roughly 1:2
val h = 4.0 / rows // height per pixel character
val w = 4.0 / cols // width per pixel character
val x0 = -2.0 // starting x value (x offset)
val y0 = -2.0 // starting y value (y offset)
def pt(x: Int, y: Int) = Complex(x * w + x0, y * h + y0)
def display(s: String, n: Int) = print(Xterm.rainbow(n) + s)
// render the area in ASCII, using o's and spaces.
cfor(0)(_ <= rows, _ + 1) { y =>
cfor(0)(_ <= cols, _ + 1) { x =>
// if n
© 2015 - 2025 Weber Informatics LLC | Privacy Policy