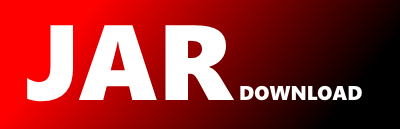
org.spongepowered.api.advancement.criteria.trigger.Triggers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
The newest version!
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.advancement.criteria.trigger;
import org.spongepowered.api.ResourceKey;
import org.spongepowered.api.Sponge;
import org.spongepowered.api.registry.DefaultedRegistryReference;
import org.spongepowered.api.registry.Registry;
import org.spongepowered.api.registry.RegistryKey;
import org.spongepowered.api.registry.RegistryScope;
import org.spongepowered.api.registry.RegistryScopes;
import org.spongepowered.api.registry.RegistryTypes;
/**
*
*/
@SuppressWarnings("unused")
@RegistryScopes(scopes = RegistryScope.GAME)
public final class Triggers {
public static final DefaultedRegistryReference> ALLAY_DROP_ITEM_ON_BLOCK = Triggers.key(ResourceKey.minecraft("allay_drop_item_on_block"));
public static final DefaultedRegistryReference> ANY_BLOCK_USE = Triggers.key(ResourceKey.minecraft("any_block_use"));
public static final DefaultedRegistryReference> AVOID_VIBRATION = Triggers.key(ResourceKey.minecraft("avoid_vibration"));
public static final DefaultedRegistryReference> BEE_NEST_DESTROYED = Triggers.key(ResourceKey.minecraft("bee_nest_destroyed"));
public static final DefaultedRegistryReference> BRED_ANIMALS = Triggers.key(ResourceKey.minecraft("bred_animals"));
public static final DefaultedRegistryReference> BREWED_POTION = Triggers.key(ResourceKey.minecraft("brewed_potion"));
public static final DefaultedRegistryReference> CHANGED_DIMENSION = Triggers.key(ResourceKey.minecraft("changed_dimension"));
public static final DefaultedRegistryReference> CHANNELED_LIGHTNING = Triggers.key(ResourceKey.minecraft("channeled_lightning"));
public static final DefaultedRegistryReference> CONSTRUCT_BEACON = Triggers.key(ResourceKey.minecraft("construct_beacon"));
public static final DefaultedRegistryReference> CONSUME_ITEM = Triggers.key(ResourceKey.minecraft("consume_item"));
public static final DefaultedRegistryReference> CRAFTER_RECIPE_CRAFTED = Triggers.key(ResourceKey.minecraft("crafter_recipe_crafted"));
public static final DefaultedRegistryReference> CURED_ZOMBIE_VILLAGER = Triggers.key(ResourceKey.minecraft("cured_zombie_villager"));
public static final DefaultedRegistryReference> DEFAULT_BLOCK_USE = Triggers.key(ResourceKey.minecraft("default_block_use"));
public static final DefaultedRegistryReference> EFFECTS_CHANGED = Triggers.key(ResourceKey.minecraft("effects_changed"));
public static final DefaultedRegistryReference> ENCHANTED_ITEM = Triggers.key(ResourceKey.minecraft("enchanted_item"));
public static final DefaultedRegistryReference> ENTER_BLOCK = Triggers.key(ResourceKey.minecraft("enter_block"));
public static final DefaultedRegistryReference> ENTITY_HURT_PLAYER = Triggers.key(ResourceKey.minecraft("entity_hurt_player"));
public static final DefaultedRegistryReference> ENTITY_KILLED_PLAYER = Triggers.key(ResourceKey.minecraft("entity_killed_player"));
public static final DefaultedRegistryReference> FALL_AFTER_EXPLOSION = Triggers.key(ResourceKey.minecraft("fall_after_explosion"));
public static final DefaultedRegistryReference> FALL_FROM_HEIGHT = Triggers.key(ResourceKey.minecraft("fall_from_height"));
public static final DefaultedRegistryReference> FILLED_BUCKET = Triggers.key(ResourceKey.minecraft("filled_bucket"));
public static final DefaultedRegistryReference> FISHING_ROD_HOOKED = Triggers.key(ResourceKey.minecraft("fishing_rod_hooked"));
public static final DefaultedRegistryReference> HERO_OF_THE_VILLAGE = Triggers.key(ResourceKey.minecraft("hero_of_the_village"));
public static final DefaultedRegistryReference> IMPOSSIBLE = Triggers.key(ResourceKey.minecraft("impossible"));
public static final DefaultedRegistryReference> INVENTORY_CHANGED = Triggers.key(ResourceKey.minecraft("inventory_changed"));
public static final DefaultedRegistryReference> ITEM_DURABILITY_CHANGED = Triggers.key(ResourceKey.minecraft("item_durability_changed"));
public static final DefaultedRegistryReference> ITEM_USED_ON_BLOCK = Triggers.key(ResourceKey.minecraft("item_used_on_block"));
public static final DefaultedRegistryReference> KILLED_BY_CROSSBOW = Triggers.key(ResourceKey.minecraft("killed_by_crossbow"));
public static final DefaultedRegistryReference> KILL_MOB_NEAR_SCULK_CATALYST = Triggers.key(ResourceKey.minecraft("kill_mob_near_sculk_catalyst"));
public static final DefaultedRegistryReference> LEVITATION = Triggers.key(ResourceKey.minecraft("levitation"));
public static final DefaultedRegistryReference> LIGHTNING_STRIKE = Triggers.key(ResourceKey.minecraft("lightning_strike"));
public static final DefaultedRegistryReference> LOCATION = Triggers.key(ResourceKey.minecraft("location"));
public static final DefaultedRegistryReference> NETHER_TRAVEL = Triggers.key(ResourceKey.minecraft("nether_travel"));
public static final DefaultedRegistryReference> PLACED_BLOCK = Triggers.key(ResourceKey.minecraft("placed_block"));
public static final DefaultedRegistryReference> PLAYER_GENERATES_CONTAINER_LOOT = Triggers.key(ResourceKey.minecraft("player_generates_container_loot"));
public static final DefaultedRegistryReference> PLAYER_HURT_ENTITY = Triggers.key(ResourceKey.minecraft("player_hurt_entity"));
public static final DefaultedRegistryReference> PLAYER_INTERACTED_WITH_ENTITY = Triggers.key(ResourceKey.minecraft("player_interacted_with_entity"));
public static final DefaultedRegistryReference> PLAYER_KILLED_ENTITY = Triggers.key(ResourceKey.minecraft("player_killed_entity"));
public static final DefaultedRegistryReference> RECIPE_CRAFTED = Triggers.key(ResourceKey.minecraft("recipe_crafted"));
public static final DefaultedRegistryReference> RECIPE_UNLOCKED = Triggers.key(ResourceKey.minecraft("recipe_unlocked"));
public static final DefaultedRegistryReference> RIDE_ENTITY_IN_LAVA = Triggers.key(ResourceKey.minecraft("ride_entity_in_lava"));
public static final DefaultedRegistryReference> SHOT_CROSSBOW = Triggers.key(ResourceKey.minecraft("shot_crossbow"));
public static final DefaultedRegistryReference> SLEPT_IN_BED = Triggers.key(ResourceKey.minecraft("slept_in_bed"));
public static final DefaultedRegistryReference> SLIDE_DOWN_BLOCK = Triggers.key(ResourceKey.minecraft("slide_down_block"));
public static final DefaultedRegistryReference> STARTED_RIDING = Triggers.key(ResourceKey.minecraft("started_riding"));
public static final DefaultedRegistryReference> SUMMONED_ENTITY = Triggers.key(ResourceKey.minecraft("summoned_entity"));
public static final DefaultedRegistryReference> TAME_ANIMAL = Triggers.key(ResourceKey.minecraft("tame_animal"));
public static final DefaultedRegistryReference> TARGET_HIT = Triggers.key(ResourceKey.minecraft("target_hit"));
public static final DefaultedRegistryReference> THROWN_ITEM_PICKED_UP_BY_ENTITY = Triggers.key(ResourceKey.minecraft("thrown_item_picked_up_by_entity"));
public static final DefaultedRegistryReference> THROWN_ITEM_PICKED_UP_BY_PLAYER = Triggers.key(ResourceKey.minecraft("thrown_item_picked_up_by_player"));
public static final DefaultedRegistryReference> TICK = Triggers.key(ResourceKey.minecraft("tick"));
public static final DefaultedRegistryReference> USED_ENDER_EYE = Triggers.key(ResourceKey.minecraft("used_ender_eye"));
public static final DefaultedRegistryReference> USED_TOTEM = Triggers.key(ResourceKey.minecraft("used_totem"));
public static final DefaultedRegistryReference> USING_ITEM = Triggers.key(ResourceKey.minecraft("using_item"));
public static final DefaultedRegistryReference> VILLAGER_TRADE = Triggers.key(ResourceKey.minecraft("villager_trade"));
public static final DefaultedRegistryReference> VOLUNTARY_EXILE = Triggers.key(ResourceKey.minecraft("voluntary_exile"));
private Triggers() {
}
public static Registry> registry() {
return Sponge.game().registry(RegistryTypes.TRIGGER);
}
private static DefaultedRegistryReference> key(final ResourceKey location) {
return RegistryKey.of(RegistryTypes.TRIGGER, location).asDefaultedReference(Sponge::game);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy