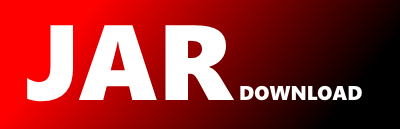
org.spongepowered.api.data.BlockStateKeys Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
The newest version!
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.data;
import org.spongepowered.api.ResourceKey;
import org.spongepowered.api.data.type.AttachmentSurface;
import org.spongepowered.api.data.type.BambooLeavesType;
import org.spongepowered.api.data.type.BellAttachmentType;
import org.spongepowered.api.data.type.ChestAttachmentType;
import org.spongepowered.api.data.type.ComparatorMode;
import org.spongepowered.api.data.type.DoorHinge;
import org.spongepowered.api.data.type.DripstoneSegment;
import org.spongepowered.api.data.type.InstrumentType;
import org.spongepowered.api.data.type.JigsawBlockOrientation;
import org.spongepowered.api.data.type.PistonType;
import org.spongepowered.api.data.type.PortionType;
import org.spongepowered.api.data.type.RailDirection;
import org.spongepowered.api.data.type.SculkSensorState;
import org.spongepowered.api.data.type.SlabPortion;
import org.spongepowered.api.data.type.StairShape;
import org.spongepowered.api.data.type.StructureMode;
import org.spongepowered.api.data.type.Tilt;
import org.spongepowered.api.data.type.TrialSpawnerState;
import org.spongepowered.api.data.type.VaultState;
import org.spongepowered.api.data.type.WallConnectionState;
import org.spongepowered.api.data.type.WireAttachmentType;
import org.spongepowered.api.data.value.Value;
import org.spongepowered.api.util.Axis;
import org.spongepowered.api.util.Direction;
/**
*
*/
@SuppressWarnings("unused")
public final class BlockStateKeys {
public static final Key> AGE_1 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_15 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_2 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_25 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_3 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_4 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_5 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> AGE_7 = BlockStateKeys.key(ResourceKey.minecraft("property/age"), Integer.class);
public static final Key> ATTACHED = BlockStateKeys.key(ResourceKey.minecraft("property/attached"), Boolean.class);
public static final Key> ATTACH_FACE = BlockStateKeys.key(ResourceKey.minecraft("property/face"), AttachmentSurface.class);
public static final Key> AXIS = BlockStateKeys.key(ResourceKey.minecraft("property/axis"), Axis.class);
public static final Key> BAMBOO_LEAVES = BlockStateKeys.key(ResourceKey.minecraft("property/leaves"), BambooLeavesType.class);
public static final Key> BED_PART = BlockStateKeys.key(ResourceKey.minecraft("property/part"), PortionType.class);
public static final Key> BELL_ATTACHMENT = BlockStateKeys.key(ResourceKey.minecraft("property/attachment"), BellAttachmentType.class);
public static final Key> BERRIES = BlockStateKeys.key(ResourceKey.minecraft("property/berries"), Boolean.class);
public static final Key> BITES = BlockStateKeys.key(ResourceKey.minecraft("property/bites"), Integer.class);
public static final Key> BLOOM = BlockStateKeys.key(ResourceKey.minecraft("property/bloom"), Boolean.class);
public static final Key> BOTTOM = BlockStateKeys.key(ResourceKey.minecraft("property/bottom"), Boolean.class);
public static final Key> CANDLES = BlockStateKeys.key(ResourceKey.minecraft("property/candles"), Integer.class);
public static final Key> CAN_SUMMON = BlockStateKeys.key(ResourceKey.minecraft("property/can_summon"), Boolean.class);
public static final Key> CHEST_TYPE = BlockStateKeys.key(ResourceKey.minecraft("property/type"), ChestAttachmentType.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_0_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_0_occupied"), Boolean.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_1_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_1_occupied"), Boolean.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_2_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_2_occupied"), Boolean.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_3_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_3_occupied"), Boolean.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_4_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_4_occupied"), Boolean.class);
public static final Key> CHISELED_BOOKSHELF_SLOT_5_OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/slot_5_occupied"), Boolean.class);
public static final Key> CONDITIONAL = BlockStateKeys.key(ResourceKey.minecraft("property/conditional"), Boolean.class);
public static final Key> CRACKED = BlockStateKeys.key(ResourceKey.minecraft("property/cracked"), Boolean.class);
public static final Key> CRAFTING = BlockStateKeys.key(ResourceKey.minecraft("property/crafting"), Boolean.class);
public static final Key> DELAY = BlockStateKeys.key(ResourceKey.minecraft("property/delay"), Integer.class);
public static final Key> DISARMED = BlockStateKeys.key(ResourceKey.minecraft("property/disarmed"), Boolean.class);
public static final Key> DISTANCE = BlockStateKeys.key(ResourceKey.minecraft("property/distance"), Integer.class);
public static final Key> DOOR_HINGE = BlockStateKeys.key(ResourceKey.minecraft("property/hinge"), DoorHinge.class);
public static final Key> DOUBLE_BLOCK_HALF = BlockStateKeys.key(ResourceKey.minecraft("property/half"), PortionType.class);
public static final Key> DOWN = BlockStateKeys.key(ResourceKey.minecraft("property/down"), Boolean.class);
public static final Key> DRAG = BlockStateKeys.key(ResourceKey.minecraft("property/drag"), Boolean.class);
public static final Key> DRIPSTONE_THICKNESS = BlockStateKeys.key(ResourceKey.minecraft("property/thickness"), DripstoneSegment.class);
public static final Key> DUSTED = BlockStateKeys.key(ResourceKey.minecraft("property/dusted"), Integer.class);
public static final Key> EAST = BlockStateKeys.key(ResourceKey.minecraft("property/east"), Boolean.class);
public static final Key> EAST_REDSTONE = BlockStateKeys.key(ResourceKey.minecraft("property/east"), WireAttachmentType.class);
public static final Key> EAST_WALL = BlockStateKeys.key(ResourceKey.minecraft("property/east"), WallConnectionState.class);
public static final Key> EGGS = BlockStateKeys.key(ResourceKey.minecraft("property/eggs"), Integer.class);
public static final Key> ENABLED = BlockStateKeys.key(ResourceKey.minecraft("property/enabled"), Boolean.class);
public static final Key> EXTENDED = BlockStateKeys.key(ResourceKey.minecraft("property/extended"), Boolean.class);
public static final Key> EYE = BlockStateKeys.key(ResourceKey.minecraft("property/eye"), Boolean.class);
public static final Key> FACING = BlockStateKeys.key(ResourceKey.minecraft("property/facing"), Direction.class);
public static final Key> FACING_HOPPER = BlockStateKeys.key(ResourceKey.minecraft("property/facing"), Direction.class);
public static final Key> FALLING = BlockStateKeys.key(ResourceKey.minecraft("property/falling"), Boolean.class);
public static final Key> FLOWER_AMOUNT = BlockStateKeys.key(ResourceKey.minecraft("property/flower_amount"), Integer.class);
public static final Key> HALF = BlockStateKeys.key(ResourceKey.minecraft("property/half"), PortionType.class);
public static final Key> HANGING = BlockStateKeys.key(ResourceKey.minecraft("property/hanging"), Boolean.class);
public static final Key> HAS_BOOK = BlockStateKeys.key(ResourceKey.minecraft("property/has_book"), Boolean.class);
public static final Key> HAS_BOTTLE_0 = BlockStateKeys.key(ResourceKey.minecraft("property/has_bottle_0"), Boolean.class);
public static final Key> HAS_BOTTLE_1 = BlockStateKeys.key(ResourceKey.minecraft("property/has_bottle_1"), Boolean.class);
public static final Key> HAS_BOTTLE_2 = BlockStateKeys.key(ResourceKey.minecraft("property/has_bottle_2"), Boolean.class);
public static final Key> HAS_RECORD = BlockStateKeys.key(ResourceKey.minecraft("property/has_record"), Boolean.class);
public static final Key> HATCH = BlockStateKeys.key(ResourceKey.minecraft("property/hatch"), Integer.class);
public static final Key> HORIZONTAL_AXIS = BlockStateKeys.key(ResourceKey.minecraft("property/axis"), Axis.class);
public static final Key> HORIZONTAL_FACING = BlockStateKeys.key(ResourceKey.minecraft("property/facing"), Direction.class);
public static final Key> INVERTED = BlockStateKeys.key(ResourceKey.minecraft("property/inverted"), Boolean.class);
public static final Key> IN_WALL = BlockStateKeys.key(ResourceKey.minecraft("property/in_wall"), Boolean.class);
public static final Key> LAYERS = BlockStateKeys.key(ResourceKey.minecraft("property/layers"), Integer.class);
public static final Key> LEVEL = BlockStateKeys.key(ResourceKey.minecraft("property/level"), Integer.class);
public static final Key> LEVEL_CAULDRON = BlockStateKeys.key(ResourceKey.minecraft("property/level"), Integer.class);
public static final Key> LEVEL_COMPOSTER = BlockStateKeys.key(ResourceKey.minecraft("property/level"), Integer.class);
public static final Key> LEVEL_FLOWING = BlockStateKeys.key(ResourceKey.minecraft("property/level"), Integer.class);
public static final Key> LEVEL_HONEY = BlockStateKeys.key(ResourceKey.minecraft("property/honey_level"), Integer.class);
public static final Key> LIT = BlockStateKeys.key(ResourceKey.minecraft("property/lit"), Boolean.class);
public static final Key> LOCKED = BlockStateKeys.key(ResourceKey.minecraft("property/locked"), Boolean.class);
public static final Key> MODE_COMPARATOR = BlockStateKeys.key(ResourceKey.minecraft("property/mode"), ComparatorMode.class);
public static final Key> MOISTURE = BlockStateKeys.key(ResourceKey.minecraft("property/moisture"), Integer.class);
public static final Key> NORTH = BlockStateKeys.key(ResourceKey.minecraft("property/north"), Boolean.class);
public static final Key> NORTH_REDSTONE = BlockStateKeys.key(ResourceKey.minecraft("property/north"), WireAttachmentType.class);
public static final Key> NORTH_WALL = BlockStateKeys.key(ResourceKey.minecraft("property/north"), WallConnectionState.class);
public static final Key> NOTE = BlockStateKeys.key(ResourceKey.minecraft("property/note"), Integer.class);
public static final Key> NOTEBLOCK_INSTRUMENT = BlockStateKeys.key(ResourceKey.minecraft("property/instrument"), InstrumentType.class);
public static final Key> OCCUPIED = BlockStateKeys.key(ResourceKey.minecraft("property/occupied"), Boolean.class);
public static final Key> OMINOUS = BlockStateKeys.key(ResourceKey.minecraft("property/ominous"), Boolean.class);
public static final Key> OPEN = BlockStateKeys.key(ResourceKey.minecraft("property/open"), Boolean.class);
public static final Key> ORIENTATION = BlockStateKeys.key(ResourceKey.minecraft("property/orientation"), JigsawBlockOrientation.class);
public static final Key> PERSISTENT = BlockStateKeys.key(ResourceKey.minecraft("property/persistent"), Boolean.class);
public static final Key> PICKLES = BlockStateKeys.key(ResourceKey.minecraft("property/pickles"), Integer.class);
public static final Key> PISTON_TYPE = BlockStateKeys.key(ResourceKey.minecraft("property/type"), PistonType.class);
public static final Key> POWER = BlockStateKeys.key(ResourceKey.minecraft("property/power"), Integer.class);
public static final Key> POWERED = BlockStateKeys.key(ResourceKey.minecraft("property/powered"), Boolean.class);
public static final Key> RAIL_SHAPE = BlockStateKeys.key(ResourceKey.minecraft("property/shape"), RailDirection.class);
public static final Key> RAIL_SHAPE_STRAIGHT = BlockStateKeys.key(ResourceKey.minecraft("property/shape"), RailDirection.class);
public static final Key> RESPAWN_ANCHOR_CHARGES = BlockStateKeys.key(ResourceKey.minecraft("property/charges"), Integer.class);
public static final Key> ROTATION_16 = BlockStateKeys.key(ResourceKey.minecraft("property/rotation"), Integer.class);
public static final Key> SCULK_SENSOR_PHASE = BlockStateKeys.key(ResourceKey.minecraft("property/sculk_sensor_phase"), SculkSensorState.class);
public static final Key> SHORT = BlockStateKeys.key(ResourceKey.minecraft("property/short"), Boolean.class);
public static final Key> SHRIEKING = BlockStateKeys.key(ResourceKey.minecraft("property/shrieking"), Boolean.class);
public static final Key> SIGNAL_FIRE = BlockStateKeys.key(ResourceKey.minecraft("property/signal_fire"), Boolean.class);
public static final Key> SLAB_TYPE = BlockStateKeys.key(ResourceKey.minecraft("property/type"), SlabPortion.class);
public static final Key> SNOWY = BlockStateKeys.key(ResourceKey.minecraft("property/snowy"), Boolean.class);
public static final Key> SOUTH = BlockStateKeys.key(ResourceKey.minecraft("property/south"), Boolean.class);
public static final Key> SOUTH_REDSTONE = BlockStateKeys.key(ResourceKey.minecraft("property/south"), WireAttachmentType.class);
public static final Key> SOUTH_WALL = BlockStateKeys.key(ResourceKey.minecraft("property/south"), WallConnectionState.class);
public static final Key> STABILITY_DISTANCE = BlockStateKeys.key(ResourceKey.minecraft("property/distance"), Integer.class);
public static final Key> STAGE = BlockStateKeys.key(ResourceKey.minecraft("property/stage"), Integer.class);
public static final Key> STAIRS_SHAPE = BlockStateKeys.key(ResourceKey.minecraft("property/shape"), StairShape.class);
public static final Key> STRUCTUREBLOCK_MODE = BlockStateKeys.key(ResourceKey.minecraft("property/mode"), StructureMode.class);
public static final Key> TILT = BlockStateKeys.key(ResourceKey.minecraft("property/tilt"), Tilt.class);
public static final Key> TRIAL_SPAWNER_STATE = BlockStateKeys.key(ResourceKey.minecraft("property/trial_spawner_state"), TrialSpawnerState.class);
public static final Key> TRIGGERED = BlockStateKeys.key(ResourceKey.minecraft("property/triggered"), Boolean.class);
public static final Key> UNSTABLE = BlockStateKeys.key(ResourceKey.minecraft("property/unstable"), Boolean.class);
public static final Key> UP = BlockStateKeys.key(ResourceKey.minecraft("property/up"), Boolean.class);
public static final Key> VAULT_STATE = BlockStateKeys.key(ResourceKey.minecraft("property/vault_state"), VaultState.class);
public static final Key> VERTICAL_DIRECTION = BlockStateKeys.key(ResourceKey.minecraft("property/vertical_direction"), Direction.class);
public static final Key> WATERLOGGED = BlockStateKeys.key(ResourceKey.minecraft("property/waterlogged"), Boolean.class);
public static final Key> WEST = BlockStateKeys.key(ResourceKey.minecraft("property/west"), Boolean.class);
public static final Key> WEST_REDSTONE = BlockStateKeys.key(ResourceKey.minecraft("property/west"), WireAttachmentType.class);
public static final Key> WEST_WALL = BlockStateKeys.key(ResourceKey.minecraft("property/west"), WallConnectionState.class);
private BlockStateKeys() {
}
private static Key> key(final ResourceKey resourceKey, final Class type) {
return Key.builder().key(resourceKey).elementType(type).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy