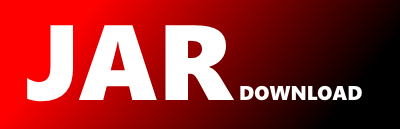
org.spongepowered.api.state.StateMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
The newest version!
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.state;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.spongepowered.api.Sponge;
import org.spongepowered.api.block.BlockState;
import org.spongepowered.api.block.BlockType;
import org.spongepowered.api.data.KeyValueMatcher;
import org.spongepowered.api.fluid.FluidState;
import org.spongepowered.api.fluid.FluidType;
import org.spongepowered.api.util.CopyableBuilder;
import java.util.List;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* A {@link StateMatcher} that will match various {@link State}s
* according to a pre-built list of {@link StateProperty}s and their
* values, such that not all {@link StateProperty}s contained in a
* {@link State} must be matched.
*/
public interface StateMatcher> extends Predicate {
/**
* Creates a new {@link Builder} for matching {@link BlockState}s.
*
* @return The builder
*/
static Builder blockStateMatcherBuilder() {
return Sponge.game().factoryProvider().provide(StateMatcher.Factory.class).blockStateMatcherBuilder();
}
/**
* Creates a new {@link Builder} for matching {@link FluidState}s.
*
* @return The builder
*/
static Builder fluidStateMatcherBuilder() {
return Sponge.game().factoryProvider().provide(StateMatcher.Factory.class).fluidStateMatcherBuilder();
}
/**
* Gets a {@code true} return value if the provided {@link State}
* sufficiently matches the pre-defined {@link StateProperty} values
* and {@link KeyValueMatcher}s.
*
* @param state The state in question
* @return True if the state sufficiently matches
*/
boolean matches(S state);
/**
* Gets a {@link List} of compatible {@link State}s.
*
* @return The list of compatible states
*/
List compatibleStates();
@Override
default boolean test(final S state) {
return this.matches(state);
}
/**
* Factories for generating builders.
*/
interface Factory {
/**
* Gets a {@link Builder} for {@link BlockState} matching.
*
* @return The builder
*/
Builder blockStateMatcherBuilder();
/**
* Gets a {@link Builder} for {@link FluidState} matching.
*
* @return The builder
*/
Builder fluidStateMatcherBuilder();
}
/**
* A builder for {@link StateMatcher}s.
*/
interface Builder, T extends StateContainer> extends org.spongepowered.api.util.Builder, Builder>,
CopyableBuilder, Builder> {
/**
* Sets the root {@link StateContainer} for the {@link StateMatcher}.
*
* @param type The {@link StateContainer} to use
* @return This builder, for chaining
*/
default Builder type(final Supplier extends T> type) {
return this.type(type.get());
}
/**
* Sets the root {@link StateContainer} for the {@link StateMatcher}.
*
* @param type The {@link StateContainer} to use
* @return This builder, for chaining
*/
Builder type(T type);
/**
* Adds a {@link StateProperty} that needs to be present
* on a {@link State} to match.
*
* {@link #type(StateContainer)} or {@link #type(Supplier)}
* must be called before this is called as supported
* {@link StateProperty state properties} are specific to the type
*
* @param stateProperty The state property
* @return This builder, for chaining
*/
Builder supportsStateProperty(StateProperty extends @NonNull Object> stateProperty);
/**
* Adds a {@link StateProperty} that needs to be present
* on a {@link State} to match.
*
* {@link #type(StateContainer)} or {@link #type(Supplier)}
* must be called before this is called as supported
* {@link StateProperty state properties} are specific to the type
*
* @param stateProperty The state property
* @return This builder, for chaining
*/
default Builder supportsStateProperty(final Supplier extends StateProperty extends @NonNull Object>> stateProperty) {
return this.supportsStateProperty(stateProperty.get());
}
/**
* Adds a {@link StateProperty} and value that needs to
* match on a {@link State} to match.
*
* {@link #type(StateContainer)} or {@link #type(Supplier)}
* must be called before this is called as supported
* {@link StateProperty state properties} are specific to the type
*
* @param stateProperty The state property
* @param value The value to match
* @param The value type
* @return This builder, for chaining
*/
> Builder stateProperty(StateProperty stateProperty, V value);
/**
* Adds a {@link StateProperty} and value that needs to
* match on a {@link State} to match.
*
* @param stateProperty The state property
* @param value The value to match
* @param The value type
* @return This builder, for chaining
*/
default > Builder stateProperty(final Supplier extends StateProperty> stateProperty, final V value) {
return this.stateProperty(stateProperty.get(), value);
}
/**
* Adds a {@link KeyValueMatcher} that the {@link State}
* needs to match with.
*
* @param matcher The matcher
* @return This builder, for chaining
*/
Builder matcher(KeyValueMatcher> matcher);
/**
* Builds a {@link StateMatcher}.
*
* @return The built state matcher
*/
@Override
StateMatcher build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy