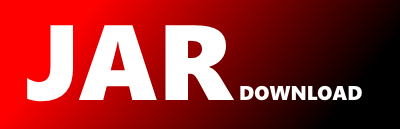
org.spongepowered.api.world.biome.Biome Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
The newest version!
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.world.biome;
import org.spongepowered.api.data.DataHolder;
import org.spongepowered.api.data.Keys;
import org.spongepowered.api.effect.sound.SoundType;
import org.spongepowered.api.entity.EntityCategories;
import org.spongepowered.api.entity.EntityCategory;
import org.spongepowered.api.entity.EntityType;
import org.spongepowered.api.entity.living.golem.SnowGolem;
import org.spongepowered.api.tag.Taggable;
import org.spongepowered.api.util.Color;
import org.spongepowered.api.util.annotation.CatalogedBy;
import org.spongepowered.api.world.biome.ambient.ParticleConfig;
import org.spongepowered.api.world.biome.ambient.SoundConfig;
import org.spongepowered.api.world.biome.climate.GrassColorModifier;
import org.spongepowered.api.world.biome.climate.Precipitation;
import org.spongepowered.api.world.biome.climate.TemperatureModifier;
import org.spongepowered.api.world.biome.spawner.NaturalSpawnCost;
import org.spongepowered.api.world.biome.spawner.NaturalSpawner;
import org.spongepowered.api.world.generation.carver.Carver;
import org.spongepowered.api.world.generation.carver.CarvingStep;
import org.spongepowered.api.world.generation.feature.DecorationStep;
import org.spongepowered.api.world.generation.feature.PlacedFeature;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* Represents a biome.
*/
@CatalogedBy(Biomes.class)
public interface Biome extends DataHolder, Taggable {
// Biome Climate
/**
* Gets the temperature of this biome.
* May influence grass and foliage color
* and {@link SnowGolem} temperature damage
*
* @return The temperature
*/
default double temperature() {
return this.require(Keys.BIOME_TEMPERATURE);
}
/**
* Gets the humidity of this biome.
* May influence grass and foliage color
* and {@link org.spongepowered.api.block.BlockTypes#FIRE} blocks burning out faster.
*
* @return The humidity
*/
default double humidity() {
return this.require(Keys.HUMIDITY);
}
/**
* Gets the default type of precipitation in this biome.
* This is determined by {@link Keys#HAS_PRECIPITATION} and {@link Keys#BIOME_TEMPERATURE}
*
* @return The type of precipitation
*/
default Precipitation precipitation() {
return this.require(Keys.PRECIPITATION);
}
/**
* Gets the type of precipitation in this biome.
*
* @return The type of precipitation
*/
default boolean hasPrecipitation() {
return this.require(Keys.HAS_PRECIPITATION);
}
/**
* Gets the temperature modifier.
*
* @return The temperature modifier
*/
default TemperatureModifier temperatureModifier() {
return this.require(Keys.TEMPERATURE_MODIFIER);
}
// Biome Generation
/**
* Gets the carvers used in world generation.
* For more details see {@link Carver}
*
* @return The carvers
*/
default Map> carvers() {
return this.require(Keys.CARVERS);
}
/**
* Gets the features used in world generation.
* For more details see {@link PlacedFeature}
*
* @return The features
*/
default Map> features() {
return this.require(Keys.FEATURES);
}
// Natural Spawning
/**
* Gets spawn chance during world generation.
* The entity to spawn is picked randomly from {@link #spawners()} of type {@link EntityCategories#CREATURE}
*
* @return The spawn chance
*/
default double generationSpawnChance() {
return this.require(Keys.SPAWN_CHANCE);
}
/**
* Gets the weighted natural spawn settings for each entity category.
*
* @return The weighted natural spawners
*/
default Map> spawners() {
return this.require(Keys.NATURAL_SPAWNERS);
}
/**
* Gets the spawn costs.
* Alternative to the mob-cap
*
* @return The spawn costs
*/
default Map, NaturalSpawnCost> cost() {
return this.require(Keys.NATURAL_SPAWNER_COST);
}
// Effects
/**
* Gets the fog color.
*
* @return The fog color
*/
default Color fogColor() {
return this.require(Keys.FOG_COLOR);
}
/**
* Gets the water color.
*
* @return The water color
*/
default Color waterColor() {
return this.require(Keys.WATER_COLOR);
}
/**
* Gets the water fog color.
*
* @return The water fog color
*/
default Color waterFogColor() {
return this.require(Keys.WATER_FOG_COLOR);
}
/**
* Gets the sky color.
*
* @return The sky color
*/
default Color skyColor() {
return this.require(Keys.SKY_COLOR);
}
/**
* Gets the foliage color override.
*
* @return The foliage color override
*/
default Optional foliageColor() {
return this.get(Keys.FOLIAGE_COLOR);
}
/**
* Gets the grass color override.
*
* @return The grass color override
*/
default Optional grassColor() {
return this.get(Keys.GRASS_COLOR);
}
/**
* Gets the grass color modifier.
*
* @return The grass color modifier
*/
default GrassColorModifier grassColorModifier() {
return this.require(Keys.GRASS_COLOR_MODIFIER);
}
/**
* Gets the ambient particle settings.
*
* @return The ambient particle settings
*/
default Optional ambientParticle() {
return this.get(Keys.AMBIENT_PARTICLE);
}
/**
* Gets the ambient sound.
*
* @return The ambient sound
*/
default Optional ambientSound() {
return this.get(Keys.AMBIENT_SOUND);
}
/**
* Gets the ambient mood settings.
*
* @return The ambient mood settings
*/
default Optional ambientMood() {
return this.get(Keys.AMBIENT_MOOD);
}
/**
* Gets the additional ambient sound settings.
*
* @return The additional ambient sound settings
*/
default Optional additionalAmbientSound() {
return this.get(Keys.AMBIENT_ADDITIONAL_SOUND);
}
/**
* Gets the background music settings.
*
* @return The background music settings
*/
default Optional backgroundMusic() {
return this.get(Keys.BACKGROUND_MUSIC);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy