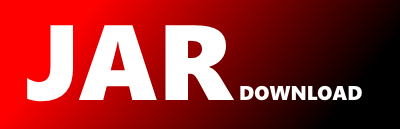
org.spongepowered.api.state.BooleanStateProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.state;
import org.spongepowered.api.ResourceKey;
import org.spongepowered.api.Sponge;
import org.spongepowered.api.registry.DefaultedRegistryReference;
import org.spongepowered.api.registry.Registry;
import org.spongepowered.api.registry.RegistryKey;
import org.spongepowered.api.registry.RegistryScope;
import org.spongepowered.api.registry.RegistryScopes;
import org.spongepowered.api.registry.RegistryTypes;
/**
*
*/
@SuppressWarnings("unused")
@RegistryScopes(scopes = RegistryScope.GAME)
public final class BooleanStateProperties {
// @formatter:off
public static final DefaultedRegistryReference ACACIA_BUTTON_POWERED = BooleanStateProperties.key(ResourceKey.sponge("acacia_button_powered"));
public static final DefaultedRegistryReference ACACIA_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("acacia_door_open"));
public static final DefaultedRegistryReference ACACIA_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("acacia_door_powered"));
public static final DefaultedRegistryReference ACACIA_FENCE_EAST = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_east"));
public static final DefaultedRegistryReference ACACIA_FENCE_GATE_IN_WALL = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_gate_in_wall"));
public static final DefaultedRegistryReference ACACIA_FENCE_GATE_OPEN = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_gate_open"));
public static final DefaultedRegistryReference ACACIA_FENCE_GATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_gate_powered"));
public static final DefaultedRegistryReference ACACIA_FENCE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_north"));
public static final DefaultedRegistryReference ACACIA_FENCE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_south"));
public static final DefaultedRegistryReference ACACIA_FENCE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_waterlogged"));
public static final DefaultedRegistryReference ACACIA_FENCE_WEST = BooleanStateProperties.key(ResourceKey.sponge("acacia_fence_west"));
public static final DefaultedRegistryReference ACACIA_LEAVES_PERSISTENT = BooleanStateProperties.key(ResourceKey.sponge("acacia_leaves_persistent"));
public static final DefaultedRegistryReference ACACIA_PRESSURE_PLATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("acacia_pressure_plate_powered"));
public static final DefaultedRegistryReference ACACIA_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_sign_waterlogged"));
public static final DefaultedRegistryReference ACACIA_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_slab_waterlogged"));
public static final DefaultedRegistryReference ACACIA_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_stairs_waterlogged"));
public static final DefaultedRegistryReference ACACIA_TRAPDOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("acacia_trapdoor_open"));
public static final DefaultedRegistryReference ACACIA_TRAPDOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("acacia_trapdoor_powered"));
public static final DefaultedRegistryReference ACACIA_TRAPDOOR_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_trapdoor_waterlogged"));
public static final DefaultedRegistryReference ACACIA_WALL_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("acacia_wall_sign_waterlogged"));
public static final DefaultedRegistryReference ACTIVATOR_RAIL_POWERED = BooleanStateProperties.key(ResourceKey.sponge("activator_rail_powered"));
public static final DefaultedRegistryReference ANDESITE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("andesite_slab_waterlogged"));
public static final DefaultedRegistryReference ANDESITE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("andesite_stairs_waterlogged"));
public static final DefaultedRegistryReference ANDESITE_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("andesite_wall_up"));
public static final DefaultedRegistryReference ANDESITE_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("andesite_wall_waterlogged"));
public static final DefaultedRegistryReference BARREL_OPEN = BooleanStateProperties.key(ResourceKey.sponge("barrel_open"));
public static final DefaultedRegistryReference BELL_POWERED = BooleanStateProperties.key(ResourceKey.sponge("bell_powered"));
public static final DefaultedRegistryReference BIRCH_BUTTON_POWERED = BooleanStateProperties.key(ResourceKey.sponge("birch_button_powered"));
public static final DefaultedRegistryReference BIRCH_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("birch_door_open"));
public static final DefaultedRegistryReference BIRCH_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("birch_door_powered"));
public static final DefaultedRegistryReference BIRCH_FENCE_EAST = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_east"));
public static final DefaultedRegistryReference BIRCH_FENCE_GATE_IN_WALL = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_gate_in_wall"));
public static final DefaultedRegistryReference BIRCH_FENCE_GATE_OPEN = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_gate_open"));
public static final DefaultedRegistryReference BIRCH_FENCE_GATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_gate_powered"));
public static final DefaultedRegistryReference BIRCH_FENCE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_north"));
public static final DefaultedRegistryReference BIRCH_FENCE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_south"));
public static final DefaultedRegistryReference BIRCH_FENCE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_waterlogged"));
public static final DefaultedRegistryReference BIRCH_FENCE_WEST = BooleanStateProperties.key(ResourceKey.sponge("birch_fence_west"));
public static final DefaultedRegistryReference BIRCH_LEAVES_PERSISTENT = BooleanStateProperties.key(ResourceKey.sponge("birch_leaves_persistent"));
public static final DefaultedRegistryReference BIRCH_PRESSURE_PLATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("birch_pressure_plate_powered"));
public static final DefaultedRegistryReference BIRCH_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_sign_waterlogged"));
public static final DefaultedRegistryReference BIRCH_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_slab_waterlogged"));
public static final DefaultedRegistryReference BIRCH_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_stairs_waterlogged"));
public static final DefaultedRegistryReference BIRCH_TRAPDOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("birch_trapdoor_open"));
public static final DefaultedRegistryReference BIRCH_TRAPDOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("birch_trapdoor_powered"));
public static final DefaultedRegistryReference BIRCH_TRAPDOOR_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_trapdoor_waterlogged"));
public static final DefaultedRegistryReference BIRCH_WALL_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("birch_wall_sign_waterlogged"));
public static final DefaultedRegistryReference BLACKSTONE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("blackstone_slab_waterlogged"));
public static final DefaultedRegistryReference BLACKSTONE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("blackstone_stairs_waterlogged"));
public static final DefaultedRegistryReference BLACKSTONE_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("blackstone_wall_up"));
public static final DefaultedRegistryReference BLACKSTONE_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("blackstone_wall_waterlogged"));
public static final DefaultedRegistryReference BLACK_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("black_bed_occupied"));
public static final DefaultedRegistryReference BLACK_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("black_stained_glass_pane_east"));
public static final DefaultedRegistryReference BLACK_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("black_stained_glass_pane_north"));
public static final DefaultedRegistryReference BLACK_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("black_stained_glass_pane_south"));
public static final DefaultedRegistryReference BLACK_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("black_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference BLACK_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("black_stained_glass_pane_west"));
public static final DefaultedRegistryReference BLAST_FURNACE_LIT = BooleanStateProperties.key(ResourceKey.sponge("blast_furnace_lit"));
public static final DefaultedRegistryReference BLUE_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("blue_bed_occupied"));
public static final DefaultedRegistryReference BLUE_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("blue_stained_glass_pane_east"));
public static final DefaultedRegistryReference BLUE_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("blue_stained_glass_pane_north"));
public static final DefaultedRegistryReference BLUE_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("blue_stained_glass_pane_south"));
public static final DefaultedRegistryReference BLUE_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("blue_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference BLUE_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("blue_stained_glass_pane_west"));
public static final DefaultedRegistryReference BRAIN_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brain_coral_fan_waterlogged"));
public static final DefaultedRegistryReference BRAIN_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brain_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference BRAIN_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brain_coral_waterlogged"));
public static final DefaultedRegistryReference BREWING_STAND_HAS_BOTTLE_0 = BooleanStateProperties.key(ResourceKey.sponge("brewing_stand_has_bottle_0"));
public static final DefaultedRegistryReference BREWING_STAND_HAS_BOTTLE_1 = BooleanStateProperties.key(ResourceKey.sponge("brewing_stand_has_bottle_1"));
public static final DefaultedRegistryReference BREWING_STAND_HAS_BOTTLE_2 = BooleanStateProperties.key(ResourceKey.sponge("brewing_stand_has_bottle_2"));
public static final DefaultedRegistryReference BRICK_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brick_slab_waterlogged"));
public static final DefaultedRegistryReference BRICK_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brick_stairs_waterlogged"));
public static final DefaultedRegistryReference BRICK_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("brick_wall_up"));
public static final DefaultedRegistryReference BRICK_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brick_wall_waterlogged"));
public static final DefaultedRegistryReference BROWN_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("brown_bed_occupied"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_DOWN = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_down"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_EAST = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_east"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_NORTH = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_north"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_south"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_UP = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_up"));
public static final DefaultedRegistryReference BROWN_MUSHROOM_BLOCK_WEST = BooleanStateProperties.key(ResourceKey.sponge("brown_mushroom_block_west"));
public static final DefaultedRegistryReference BROWN_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("brown_stained_glass_pane_east"));
public static final DefaultedRegistryReference BROWN_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("brown_stained_glass_pane_north"));
public static final DefaultedRegistryReference BROWN_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("brown_stained_glass_pane_south"));
public static final DefaultedRegistryReference BROWN_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("brown_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference BROWN_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("brown_stained_glass_pane_west"));
public static final DefaultedRegistryReference BUBBLE_COLUMN_DRAG = BooleanStateProperties.key(ResourceKey.sponge("bubble_column_drag"));
public static final DefaultedRegistryReference BUBBLE_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("bubble_coral_fan_waterlogged"));
public static final DefaultedRegistryReference BUBBLE_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("bubble_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference BUBBLE_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("bubble_coral_waterlogged"));
public static final DefaultedRegistryReference CAMPFIRE_LIT = BooleanStateProperties.key(ResourceKey.sponge("campfire_lit"));
public static final DefaultedRegistryReference CAMPFIRE_SIGNAL_FIRE = BooleanStateProperties.key(ResourceKey.sponge("campfire_signal_fire"));
public static final DefaultedRegistryReference CAMPFIRE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("campfire_waterlogged"));
public static final DefaultedRegistryReference CHAIN_COMMAND_BLOCK_CONDITIONAL = BooleanStateProperties.key(ResourceKey.sponge("chain_command_block_conditional"));
public static final DefaultedRegistryReference CHAIN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("chain_waterlogged"));
public static final DefaultedRegistryReference CHEST_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("chest_waterlogged"));
public static final DefaultedRegistryReference CHORUS_PLANT_DOWN = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_down"));
public static final DefaultedRegistryReference CHORUS_PLANT_EAST = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_east"));
public static final DefaultedRegistryReference CHORUS_PLANT_NORTH = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_north"));
public static final DefaultedRegistryReference CHORUS_PLANT_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_south"));
public static final DefaultedRegistryReference CHORUS_PLANT_UP = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_up"));
public static final DefaultedRegistryReference CHORUS_PLANT_WEST = BooleanStateProperties.key(ResourceKey.sponge("chorus_plant_west"));
public static final DefaultedRegistryReference COBBLESTONE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cobblestone_slab_waterlogged"));
public static final DefaultedRegistryReference COBBLESTONE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cobblestone_stairs_waterlogged"));
public static final DefaultedRegistryReference COBBLESTONE_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("cobblestone_wall_up"));
public static final DefaultedRegistryReference COBBLESTONE_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cobblestone_wall_waterlogged"));
public static final DefaultedRegistryReference COMMAND_BLOCK_CONDITIONAL = BooleanStateProperties.key(ResourceKey.sponge("command_block_conditional"));
public static final DefaultedRegistryReference COMPARATOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("comparator_powered"));
public static final DefaultedRegistryReference CONDUIT_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("conduit_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_BUTTON_POWERED = BooleanStateProperties.key(ResourceKey.sponge("crimson_button_powered"));
public static final DefaultedRegistryReference CRIMSON_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("crimson_door_open"));
public static final DefaultedRegistryReference CRIMSON_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("crimson_door_powered"));
public static final DefaultedRegistryReference CRIMSON_FENCE_EAST = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_east"));
public static final DefaultedRegistryReference CRIMSON_FENCE_GATE_IN_WALL = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_gate_in_wall"));
public static final DefaultedRegistryReference CRIMSON_FENCE_GATE_OPEN = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_gate_open"));
public static final DefaultedRegistryReference CRIMSON_FENCE_GATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_gate_powered"));
public static final DefaultedRegistryReference CRIMSON_FENCE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_north"));
public static final DefaultedRegistryReference CRIMSON_FENCE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_south"));
public static final DefaultedRegistryReference CRIMSON_FENCE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_FENCE_WEST = BooleanStateProperties.key(ResourceKey.sponge("crimson_fence_west"));
public static final DefaultedRegistryReference CRIMSON_PRESSURE_PLATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("crimson_pressure_plate_powered"));
public static final DefaultedRegistryReference CRIMSON_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_sign_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_slab_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_stairs_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_TRAPDOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("crimson_trapdoor_open"));
public static final DefaultedRegistryReference CRIMSON_TRAPDOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("crimson_trapdoor_powered"));
public static final DefaultedRegistryReference CRIMSON_TRAPDOOR_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_trapdoor_waterlogged"));
public static final DefaultedRegistryReference CRIMSON_WALL_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("crimson_wall_sign_waterlogged"));
public static final DefaultedRegistryReference CUT_RED_SANDSTONE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cut_red_sandstone_slab_waterlogged"));
public static final DefaultedRegistryReference CUT_SANDSTONE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cut_sandstone_slab_waterlogged"));
public static final DefaultedRegistryReference CYAN_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("cyan_bed_occupied"));
public static final DefaultedRegistryReference CYAN_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("cyan_stained_glass_pane_east"));
public static final DefaultedRegistryReference CYAN_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("cyan_stained_glass_pane_north"));
public static final DefaultedRegistryReference CYAN_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("cyan_stained_glass_pane_south"));
public static final DefaultedRegistryReference CYAN_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("cyan_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference CYAN_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("cyan_stained_glass_pane_west"));
public static final DefaultedRegistryReference DARK_OAK_BUTTON_POWERED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_button_powered"));
public static final DefaultedRegistryReference DARK_OAK_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_door_open"));
public static final DefaultedRegistryReference DARK_OAK_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_door_powered"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_EAST = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_east"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_GATE_IN_WALL = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_gate_in_wall"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_GATE_OPEN = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_gate_open"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_GATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_gate_powered"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_north"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_south"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_waterlogged"));
public static final DefaultedRegistryReference DARK_OAK_FENCE_WEST = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_fence_west"));
public static final DefaultedRegistryReference DARK_OAK_LEAVES_PERSISTENT = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_leaves_persistent"));
public static final DefaultedRegistryReference DARK_OAK_PRESSURE_PLATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_pressure_plate_powered"));
public static final DefaultedRegistryReference DARK_OAK_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_sign_waterlogged"));
public static final DefaultedRegistryReference DARK_OAK_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_slab_waterlogged"));
public static final DefaultedRegistryReference DARK_OAK_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_stairs_waterlogged"));
public static final DefaultedRegistryReference DARK_OAK_TRAPDOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_trapdoor_open"));
public static final DefaultedRegistryReference DARK_OAK_TRAPDOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_trapdoor_powered"));
public static final DefaultedRegistryReference DARK_OAK_TRAPDOOR_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_trapdoor_waterlogged"));
public static final DefaultedRegistryReference DARK_OAK_WALL_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_oak_wall_sign_waterlogged"));
public static final DefaultedRegistryReference DARK_PRISMARINE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_prismarine_slab_waterlogged"));
public static final DefaultedRegistryReference DARK_PRISMARINE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dark_prismarine_stairs_waterlogged"));
public static final DefaultedRegistryReference DAYLIGHT_DETECTOR_INVERTED = BooleanStateProperties.key(ResourceKey.sponge("daylight_detector_inverted"));
public static final DefaultedRegistryReference DEAD_BRAIN_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_brain_coral_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_BRAIN_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_brain_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_BRAIN_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_brain_coral_waterlogged"));
public static final DefaultedRegistryReference DEAD_BUBBLE_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_bubble_coral_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_BUBBLE_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_bubble_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_BUBBLE_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_bubble_coral_waterlogged"));
public static final DefaultedRegistryReference DEAD_FIRE_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_fire_coral_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_FIRE_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_fire_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_FIRE_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_fire_coral_waterlogged"));
public static final DefaultedRegistryReference DEAD_HORN_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_horn_coral_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_HORN_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_horn_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_HORN_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_horn_coral_waterlogged"));
public static final DefaultedRegistryReference DEAD_TUBE_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_tube_coral_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_TUBE_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_tube_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference DEAD_TUBE_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("dead_tube_coral_waterlogged"));
public static final DefaultedRegistryReference DETECTOR_RAIL_POWERED = BooleanStateProperties.key(ResourceKey.sponge("detector_rail_powered"));
public static final DefaultedRegistryReference DIORITE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("diorite_slab_waterlogged"));
public static final DefaultedRegistryReference DIORITE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("diorite_stairs_waterlogged"));
public static final DefaultedRegistryReference DIORITE_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("diorite_wall_up"));
public static final DefaultedRegistryReference DIORITE_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("diorite_wall_waterlogged"));
public static final DefaultedRegistryReference DISPENSER_TRIGGERED = BooleanStateProperties.key(ResourceKey.sponge("dispenser_triggered"));
public static final DefaultedRegistryReference DROPPER_TRIGGERED = BooleanStateProperties.key(ResourceKey.sponge("dropper_triggered"));
public static final DefaultedRegistryReference ENDER_CHEST_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("ender_chest_waterlogged"));
public static final DefaultedRegistryReference END_PORTAL_FRAME_EYE = BooleanStateProperties.key(ResourceKey.sponge("end_portal_frame_eye"));
public static final DefaultedRegistryReference END_STONE_BRICK_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("end_stone_brick_slab_waterlogged"));
public static final DefaultedRegistryReference END_STONE_BRICK_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("end_stone_brick_stairs_waterlogged"));
public static final DefaultedRegistryReference END_STONE_BRICK_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("end_stone_brick_wall_up"));
public static final DefaultedRegistryReference END_STONE_BRICK_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("end_stone_brick_wall_waterlogged"));
public static final DefaultedRegistryReference FIRE_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("fire_coral_fan_waterlogged"));
public static final DefaultedRegistryReference FIRE_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("fire_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference FIRE_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("fire_coral_waterlogged"));
public static final DefaultedRegistryReference FIRE_EAST = BooleanStateProperties.key(ResourceKey.sponge("fire_east"));
public static final DefaultedRegistryReference FIRE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("fire_north"));
public static final DefaultedRegistryReference FIRE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("fire_south"));
public static final DefaultedRegistryReference FIRE_UP = BooleanStateProperties.key(ResourceKey.sponge("fire_up"));
public static final DefaultedRegistryReference FIRE_WEST = BooleanStateProperties.key(ResourceKey.sponge("fire_west"));
public static final DefaultedRegistryReference FURNACE_LIT = BooleanStateProperties.key(ResourceKey.sponge("furnace_lit"));
public static final DefaultedRegistryReference GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("glass_pane_east"));
public static final DefaultedRegistryReference GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("glass_pane_north"));
public static final DefaultedRegistryReference GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("glass_pane_south"));
public static final DefaultedRegistryReference GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("glass_pane_waterlogged"));
public static final DefaultedRegistryReference GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("glass_pane_west"));
public static final DefaultedRegistryReference GRANITE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("granite_slab_waterlogged"));
public static final DefaultedRegistryReference GRANITE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("granite_stairs_waterlogged"));
public static final DefaultedRegistryReference GRANITE_WALL_UP = BooleanStateProperties.key(ResourceKey.sponge("granite_wall_up"));
public static final DefaultedRegistryReference GRANITE_WALL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("granite_wall_waterlogged"));
public static final DefaultedRegistryReference GRASS_BLOCK_SNOWY = BooleanStateProperties.key(ResourceKey.sponge("grass_block_snowy"));
public static final DefaultedRegistryReference GRAY_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("gray_bed_occupied"));
public static final DefaultedRegistryReference GRAY_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("gray_stained_glass_pane_east"));
public static final DefaultedRegistryReference GRAY_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("gray_stained_glass_pane_north"));
public static final DefaultedRegistryReference GRAY_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("gray_stained_glass_pane_south"));
public static final DefaultedRegistryReference GRAY_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("gray_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference GRAY_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("gray_stained_glass_pane_west"));
public static final DefaultedRegistryReference GREEN_BED_OCCUPIED = BooleanStateProperties.key(ResourceKey.sponge("green_bed_occupied"));
public static final DefaultedRegistryReference GREEN_STAINED_GLASS_PANE_EAST = BooleanStateProperties.key(ResourceKey.sponge("green_stained_glass_pane_east"));
public static final DefaultedRegistryReference GREEN_STAINED_GLASS_PANE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("green_stained_glass_pane_north"));
public static final DefaultedRegistryReference GREEN_STAINED_GLASS_PANE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("green_stained_glass_pane_south"));
public static final DefaultedRegistryReference GREEN_STAINED_GLASS_PANE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("green_stained_glass_pane_waterlogged"));
public static final DefaultedRegistryReference GREEN_STAINED_GLASS_PANE_WEST = BooleanStateProperties.key(ResourceKey.sponge("green_stained_glass_pane_west"));
public static final DefaultedRegistryReference HOPPER_ENABLED = BooleanStateProperties.key(ResourceKey.sponge("hopper_enabled"));
public static final DefaultedRegistryReference HORN_CORAL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("horn_coral_fan_waterlogged"));
public static final DefaultedRegistryReference HORN_CORAL_WALL_FAN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("horn_coral_wall_fan_waterlogged"));
public static final DefaultedRegistryReference HORN_CORAL_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("horn_coral_waterlogged"));
public static final DefaultedRegistryReference IRON_BARS_EAST = BooleanStateProperties.key(ResourceKey.sponge("iron_bars_east"));
public static final DefaultedRegistryReference IRON_BARS_NORTH = BooleanStateProperties.key(ResourceKey.sponge("iron_bars_north"));
public static final DefaultedRegistryReference IRON_BARS_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("iron_bars_south"));
public static final DefaultedRegistryReference IRON_BARS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("iron_bars_waterlogged"));
public static final DefaultedRegistryReference IRON_BARS_WEST = BooleanStateProperties.key(ResourceKey.sponge("iron_bars_west"));
public static final DefaultedRegistryReference IRON_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("iron_door_open"));
public static final DefaultedRegistryReference IRON_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("iron_door_powered"));
public static final DefaultedRegistryReference IRON_TRAPDOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("iron_trapdoor_open"));
public static final DefaultedRegistryReference IRON_TRAPDOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("iron_trapdoor_powered"));
public static final DefaultedRegistryReference IRON_TRAPDOOR_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("iron_trapdoor_waterlogged"));
public static final DefaultedRegistryReference JUKEBOX_HAS_RECORD = BooleanStateProperties.key(ResourceKey.sponge("jukebox_has_record"));
public static final DefaultedRegistryReference JUNGLE_BUTTON_POWERED = BooleanStateProperties.key(ResourceKey.sponge("jungle_button_powered"));
public static final DefaultedRegistryReference JUNGLE_DOOR_OPEN = BooleanStateProperties.key(ResourceKey.sponge("jungle_door_open"));
public static final DefaultedRegistryReference JUNGLE_DOOR_POWERED = BooleanStateProperties.key(ResourceKey.sponge("jungle_door_powered"));
public static final DefaultedRegistryReference JUNGLE_FENCE_EAST = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_east"));
public static final DefaultedRegistryReference JUNGLE_FENCE_GATE_IN_WALL = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_gate_in_wall"));
public static final DefaultedRegistryReference JUNGLE_FENCE_GATE_OPEN = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_gate_open"));
public static final DefaultedRegistryReference JUNGLE_FENCE_GATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_gate_powered"));
public static final DefaultedRegistryReference JUNGLE_FENCE_NORTH = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_north"));
public static final DefaultedRegistryReference JUNGLE_FENCE_SOUTH = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_south"));
public static final DefaultedRegistryReference JUNGLE_FENCE_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_waterlogged"));
public static final DefaultedRegistryReference JUNGLE_FENCE_WEST = BooleanStateProperties.key(ResourceKey.sponge("jungle_fence_west"));
public static final DefaultedRegistryReference JUNGLE_LEAVES_PERSISTENT = BooleanStateProperties.key(ResourceKey.sponge("jungle_leaves_persistent"));
public static final DefaultedRegistryReference JUNGLE_PRESSURE_PLATE_POWERED = BooleanStateProperties.key(ResourceKey.sponge("jungle_pressure_plate_powered"));
public static final DefaultedRegistryReference JUNGLE_SIGN_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("jungle_sign_waterlogged"));
public static final DefaultedRegistryReference JUNGLE_SLAB_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("jungle_slab_waterlogged"));
public static final DefaultedRegistryReference JUNGLE_STAIRS_WATERLOGGED = BooleanStateProperties.key(ResourceKey.sponge("jungle_stairs_waterlogged"));
public static final DefaultedRegistryReference