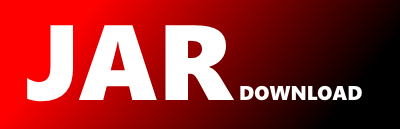
org.spongepowered.api.state.IntegerStateProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spongeapi Show documentation
Show all versions of spongeapi Show documentation
A plugin API for Minecraft: Java Edition
/*
* This file is part of SpongeAPI, licensed under the MIT License (MIT).
*
* Copyright (c) SpongePowered
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.spongepowered.api.state;
import org.spongepowered.api.ResourceKey;
import org.spongepowered.api.Sponge;
import org.spongepowered.api.registry.DefaultedRegistryReference;
import org.spongepowered.api.registry.Registry;
import org.spongepowered.api.registry.RegistryKey;
import org.spongepowered.api.registry.RegistryScope;
import org.spongepowered.api.registry.RegistryScopes;
import org.spongepowered.api.registry.RegistryTypes;
/**
*
*/
@SuppressWarnings("unused")
@RegistryScopes(scopes = RegistryScope.GAME)
public final class IntegerStateProperties {
// @formatter:off
public static final DefaultedRegistryReference ACACIA_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("acacia_leaves_distance"));
public static final DefaultedRegistryReference ACACIA_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("acacia_sapling_stage"));
public static final DefaultedRegistryReference ACACIA_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("acacia_sign_rotation"));
public static final DefaultedRegistryReference BAMBOO_AGE = IntegerStateProperties.key(ResourceKey.sponge("bamboo_age"));
public static final DefaultedRegistryReference BAMBOO_STAGE = IntegerStateProperties.key(ResourceKey.sponge("bamboo_stage"));
public static final DefaultedRegistryReference BEEHIVE_HONEY_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("beehive_honey_level"));
public static final DefaultedRegistryReference BEETROOTS_AGE = IntegerStateProperties.key(ResourceKey.sponge("beetroots_age"));
public static final DefaultedRegistryReference BEE_NEST_HONEY_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("bee_nest_honey_level"));
public static final DefaultedRegistryReference BIRCH_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("birch_leaves_distance"));
public static final DefaultedRegistryReference BIRCH_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("birch_sapling_stage"));
public static final DefaultedRegistryReference BIRCH_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("birch_sign_rotation"));
public static final DefaultedRegistryReference BLACK_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("black_banner_rotation"));
public static final DefaultedRegistryReference BLUE_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("blue_banner_rotation"));
public static final DefaultedRegistryReference BROWN_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("brown_banner_rotation"));
public static final DefaultedRegistryReference CACTUS_AGE = IntegerStateProperties.key(ResourceKey.sponge("cactus_age"));
public static final DefaultedRegistryReference CAKE_BITES = IntegerStateProperties.key(ResourceKey.sponge("cake_bites"));
public static final DefaultedRegistryReference CARROTS_AGE = IntegerStateProperties.key(ResourceKey.sponge("carrots_age"));
public static final DefaultedRegistryReference CAULDRON_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("cauldron_level"));
public static final DefaultedRegistryReference CHORUS_FLOWER_AGE = IntegerStateProperties.key(ResourceKey.sponge("chorus_flower_age"));
public static final DefaultedRegistryReference COCOA_AGE = IntegerStateProperties.key(ResourceKey.sponge("cocoa_age"));
public static final DefaultedRegistryReference COMPOSTER_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("composter_level"));
public static final DefaultedRegistryReference CREEPER_HEAD_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("creeper_head_rotation"));
public static final DefaultedRegistryReference CRIMSON_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("crimson_sign_rotation"));
public static final DefaultedRegistryReference CYAN_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("cyan_banner_rotation"));
public static final DefaultedRegistryReference DARK_OAK_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("dark_oak_leaves_distance"));
public static final DefaultedRegistryReference DARK_OAK_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("dark_oak_sapling_stage"));
public static final DefaultedRegistryReference DARK_OAK_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("dark_oak_sign_rotation"));
public static final DefaultedRegistryReference DAYLIGHT_DETECTOR_POWER = IntegerStateProperties.key(ResourceKey.sponge("daylight_detector_power"));
public static final DefaultedRegistryReference DRAGON_HEAD_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("dragon_head_rotation"));
public static final DefaultedRegistryReference FARMLAND_MOISTURE = IntegerStateProperties.key(ResourceKey.sponge("farmland_moisture"));
public static final DefaultedRegistryReference FIRE_AGE = IntegerStateProperties.key(ResourceKey.sponge("fire_age"));
public static final DefaultedRegistryReference FROSTED_ICE_AGE = IntegerStateProperties.key(ResourceKey.sponge("frosted_ice_age"));
public static final DefaultedRegistryReference GRAY_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("gray_banner_rotation"));
public static final DefaultedRegistryReference GREEN_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("green_banner_rotation"));
public static final DefaultedRegistryReference HEAVY_WEIGHTED_PRESSURE_PLATE_POWER = IntegerStateProperties.key(ResourceKey.sponge("heavy_weighted_pressure_plate_power"));
public static final DefaultedRegistryReference JUNGLE_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("jungle_leaves_distance"));
public static final DefaultedRegistryReference JUNGLE_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("jungle_sapling_stage"));
public static final DefaultedRegistryReference JUNGLE_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("jungle_sign_rotation"));
public static final DefaultedRegistryReference KELP_AGE = IntegerStateProperties.key(ResourceKey.sponge("kelp_age"));
public static final DefaultedRegistryReference LAVA_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("lava_level"));
public static final DefaultedRegistryReference LIGHT_BLUE_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("light_blue_banner_rotation"));
public static final DefaultedRegistryReference LIGHT_GRAY_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("light_gray_banner_rotation"));
public static final DefaultedRegistryReference LIGHT_WEIGHTED_PRESSURE_PLATE_POWER = IntegerStateProperties.key(ResourceKey.sponge("light_weighted_pressure_plate_power"));
public static final DefaultedRegistryReference LIME_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("lime_banner_rotation"));
public static final DefaultedRegistryReference MAGENTA_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("magenta_banner_rotation"));
public static final DefaultedRegistryReference MELON_STEM_AGE = IntegerStateProperties.key(ResourceKey.sponge("melon_stem_age"));
public static final DefaultedRegistryReference NETHER_WART_AGE = IntegerStateProperties.key(ResourceKey.sponge("nether_wart_age"));
public static final DefaultedRegistryReference NOTE_BLOCK_NOTE = IntegerStateProperties.key(ResourceKey.sponge("note_block_note"));
public static final DefaultedRegistryReference OAK_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("oak_leaves_distance"));
public static final DefaultedRegistryReference OAK_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("oak_sapling_stage"));
public static final DefaultedRegistryReference OAK_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("oak_sign_rotation"));
public static final DefaultedRegistryReference ORANGE_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("orange_banner_rotation"));
public static final DefaultedRegistryReference PINK_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("pink_banner_rotation"));
public static final DefaultedRegistryReference PLAYER_HEAD_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("player_head_rotation"));
public static final DefaultedRegistryReference POTATOES_AGE = IntegerStateProperties.key(ResourceKey.sponge("potatoes_age"));
public static final DefaultedRegistryReference PUMPKIN_STEM_AGE = IntegerStateProperties.key(ResourceKey.sponge("pumpkin_stem_age"));
public static final DefaultedRegistryReference PURPLE_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("purple_banner_rotation"));
public static final DefaultedRegistryReference REDSTONE_WIRE_POWER = IntegerStateProperties.key(ResourceKey.sponge("redstone_wire_power"));
public static final DefaultedRegistryReference RED_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("red_banner_rotation"));
public static final DefaultedRegistryReference REPEATER_DELAY = IntegerStateProperties.key(ResourceKey.sponge("repeater_delay"));
public static final DefaultedRegistryReference RESPAWN_ANCHOR_CHARGES = IntegerStateProperties.key(ResourceKey.sponge("respawn_anchor_charges"));
public static final DefaultedRegistryReference SCAFFOLDING_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("scaffolding_distance"));
public static final DefaultedRegistryReference SEA_PICKLE_PICKLES = IntegerStateProperties.key(ResourceKey.sponge("sea_pickle_pickles"));
public static final DefaultedRegistryReference SKELETON_SKULL_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("skeleton_skull_rotation"));
public static final DefaultedRegistryReference SNOW_LAYERS = IntegerStateProperties.key(ResourceKey.sponge("snow_layers"));
public static final DefaultedRegistryReference SPRUCE_LEAVES_DISTANCE = IntegerStateProperties.key(ResourceKey.sponge("spruce_leaves_distance"));
public static final DefaultedRegistryReference SPRUCE_SAPLING_STAGE = IntegerStateProperties.key(ResourceKey.sponge("spruce_sapling_stage"));
public static final DefaultedRegistryReference SPRUCE_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("spruce_sign_rotation"));
public static final DefaultedRegistryReference SUGAR_CANE_AGE = IntegerStateProperties.key(ResourceKey.sponge("sugar_cane_age"));
public static final DefaultedRegistryReference SWEET_BERRY_BUSH_AGE = IntegerStateProperties.key(ResourceKey.sponge("sweet_berry_bush_age"));
public static final DefaultedRegistryReference TARGET_POWER = IntegerStateProperties.key(ResourceKey.sponge("target_power"));
public static final DefaultedRegistryReference TURTLE_EGG_EGGS = IntegerStateProperties.key(ResourceKey.sponge("turtle_egg_eggs"));
public static final DefaultedRegistryReference TURTLE_EGG_HATCH = IntegerStateProperties.key(ResourceKey.sponge("turtle_egg_hatch"));
public static final DefaultedRegistryReference TWISTING_VINES_AGE = IntegerStateProperties.key(ResourceKey.sponge("twisting_vines_age"));
public static final DefaultedRegistryReference WARPED_SIGN_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("warped_sign_rotation"));
public static final DefaultedRegistryReference WATER_LEVEL = IntegerStateProperties.key(ResourceKey.sponge("water_level"));
public static final DefaultedRegistryReference WEEPING_VINES_AGE = IntegerStateProperties.key(ResourceKey.sponge("weeping_vines_age"));
public static final DefaultedRegistryReference WHEAT_AGE = IntegerStateProperties.key(ResourceKey.sponge("wheat_age"));
public static final DefaultedRegistryReference WHITE_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("white_banner_rotation"));
public static final DefaultedRegistryReference WITHER_SKELETON_SKULL_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("wither_skeleton_skull_rotation"));
public static final DefaultedRegistryReference YELLOW_BANNER_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("yellow_banner_rotation"));
public static final DefaultedRegistryReference ZOMBIE_HEAD_ROTATION = IntegerStateProperties.key(ResourceKey.sponge("zombie_head_rotation"));
// @formatter:on
private IntegerStateProperties() {
}
public static Registry registry() {
return Sponge.game().registry(RegistryTypes.INTEGER_STATE_PROPERTY);
}
private static DefaultedRegistryReference key(final ResourceKey location) {
return RegistryKey.of(RegistryTypes.INTEGER_STATE_PROPERTY, location).asDefaultedReference(Sponge::game);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy