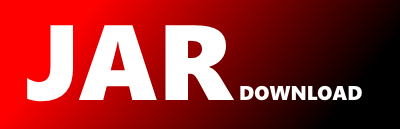
org.springframework.data.spel.Functions Maven / Gradle / Ivy
/*
* Copyright 2017-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.data.spel;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.springframework.core.convert.TypeDescriptor;
import org.springframework.data.spel.spi.Function;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
/**
* {@link MultiValueMap} like data structure to keep lists of
* {@link org.springframework.data.repository.query.spi.Function}s indexed by name and argument list length, where the
* value lists are actually unique with respect to the signature.
*
* @author Jens Schauder
* @author Oliver Gierke
* @since 2.1
*/
class Functions {
private static final String MESSAGE_TEMPLATE = "There are multiple matching methods of name '%s' for parameter types (%s), but no "
+ "exact match; Make sure to provide only one matching overload or one with exactly those types";
private final MultiValueMap functions = new LinkedMultiValueMap<>();
void addAll(Map newFunctions) {
newFunctions.forEach((n, f) -> {
List currentElements = get(n);
if (!contains(currentElements, f)) {
functions.add(n, f);
}
});
}
void addAll(MultiValueMap newFunctions) {
newFunctions.forEach((k, list) -> {
List currentElements = get(k);
list.stream() //
.filter(f -> !contains(currentElements, f)) //
.forEach(f -> functions.add(k, f));
});
}
List get(String name) {
return functions.getOrDefault(name, Collections.emptyList());
}
/**
* Gets the function that best matches the parameters given. The {@code name} must match, and the
* {@code argumentTypes} must be compatible with parameter list of the function. In order to resolve ambiguity it
* checks for a method with exactly matching parameter list.
*
* @param name the name of the method
* @param argumentTypes types of arguments that the method must be able to accept
* @return a {@code Function} if a unique on gets found. {@code Optional.empty} if none matches. Throws
* {@link IllegalStateException} if multiple functions match the parameters.
*/
Optional get(String name, List argumentTypes) {
Stream candidates = get(name).stream() //
.filter(f -> f.supports(argumentTypes));
List collect = candidates.collect(Collectors.toList());
return bestMatch(collect, argumentTypes);
}
private static boolean contains(List elements, Function f) {
return elements.stream().anyMatch(f::isSignatureEqual);
}
private static Optional bestMatch(List candidates, List argumentTypes) {
if (candidates.isEmpty()) {
return Optional.empty();
}
if (candidates.size() == 1) {
return Optional.of(candidates.get(0));
}
Optional exactMatch = candidates.stream().filter(f -> f.supportsExact(argumentTypes)).findFirst();
if (!exactMatch.isPresent()) {
throw new IllegalStateException(createErrorMessage(candidates, argumentTypes));
}
return exactMatch;
}
private static String createErrorMessage(List candidates, List argumentTypes) {
String argumentTypeString = argumentTypes.stream()//
.map(TypeDescriptor::getName)//
.collect(Collectors.joining(","));
return String.format(MESSAGE_TEMPLATE, candidates.get(0).getName(), argumentTypeString);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy