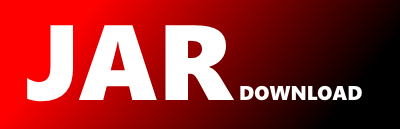
org.neo4j.rest.graphdb.index.RetrievedIndexInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-data-neo4j-rest Show documentation
Show all versions of spring-data-neo4j-rest Show documentation
pring Data Neo4j Wrapper for the Neo4j REST API, provides a Graph Database proxy for the remote invocation.
/**
* Copyright (c) 2002-2013 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.rest.graphdb.index;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import org.neo4j.rest.graphdb.RequestResult;
import com.sun.jersey.api.client.ClientResponse;
/**
* @author mh
* @since 22.09.11
*/
public class RetrievedIndexInfo implements IndexInfo {
private Map indexInfo;
private long expired;
public RetrievedIndexInfo(RequestResult response) {
if (response.statusIs(ClientResponse.Status.NO_CONTENT)) this.indexInfo = Collections.emptyMap();
else {
this.indexInfo = (Map) response.toMap();
this.expired = System.currentTimeMillis() + TimeUnit.MINUTES.toMillis(10);
}
}
@Override
public boolean checkConfig(String indexName, Map config) {
Map existingConfig = (Map) indexInfo.get(indexName);
if (config == null) {
return existingConfig != null;
} else {
if (existingConfig == null) {
return false;
} else {
if (existingConfig.entrySet().containsAll(config.entrySet())) {
return true;
} else {
throw new IllegalArgumentException("Index with the same name but different config exists!");
}
}
}
}
@Override
public String[] indexNames() {
Set keys = indexInfo.keySet();
return keys.toArray(new String[keys.size()]);
}
@Override
public boolean exists(String indexName) {
return indexInfo.containsKey(indexName);
}
@Override
public Map getConfig(String name) {
return (Map) indexInfo.get(name);
}
@Override
public boolean isExpired() {
return System.currentTimeMillis() > expired;
}
@Override
public void setExpired() {
this.expired = 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy