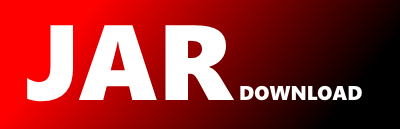
org.neo4j.rest.graphdb.RestAPIIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-data-neo4j-rest Show documentation
Show all versions of spring-data-neo4j-rest Show documentation
pring Data Neo4j Wrapper for the Neo4j REST API, provides a Graph Database proxy for the remote invocation.
The newest version!
package org.neo4j.rest.graphdb;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.Relationship;
import org.neo4j.graphdb.index.IndexHits;
import org.neo4j.rest.graphdb.entity.RestNode;
import org.neo4j.rest.graphdb.entity.RestRelationship;
import org.neo4j.rest.graphdb.index.IndexInfo;
import org.neo4j.rest.graphdb.index.RestIndex;
import org.neo4j.rest.graphdb.index.RestIndexManager;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
/**
* @author mh
* @since 21.09.14
*/
public interface RestAPIIndex {
RestIndexManager index();
@SuppressWarnings("unchecked")
RestIndex getIndex(String indexName);
@SuppressWarnings("unchecked")
void createIndex(String type, String indexName, Map config);
@SuppressWarnings("unchecked")
IndexHits getIndex(Class entityType, String indexName, String key, Object value);
IndexHits queryIndex(Class entityType, String indexName, String key, Object value);
IndexInfo indexInfo(String indexType);
void removeFromIndex(RestIndex index, T entity, String key, Object value);
void removeFromIndex(RestIndex index, T entity, String key);
void removeFromIndex(RestIndex index, T entity);
void addToIndex(T entity, RestIndex index, String key, Object value);
@SuppressWarnings("unchecked")
T putIfAbsent(T entity, RestIndex index, String key, Object value);
RestNode getOrCreateNode(RestIndex index, String key, Object value, Map properties, Collection labels);
RestRelationship getOrCreateRelationship(RestIndex index, String key, Object value, RestNode start, RestNode end, String type, Map properties);
@SuppressWarnings("unchecked")
RestIndex createIndex(Class type, String indexName, Map config);
boolean isAutoIndexingEnabled(Class extends PropertyContainer> clazz);
void setAutoIndexingEnabled(Class extends PropertyContainer> clazz, boolean enabled);
Set getAutoIndexedProperties(Class forClass);
void startAutoIndexingProperty(Class forClass, String s);
void stopAutoIndexingProperty(Class forClass, String s);
void delete(RestIndex index);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy