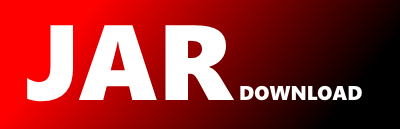
org.neo4j.rest.graphdb.traversal.SimplePath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-data-neo4j-rest Show documentation
Show all versions of spring-data-neo4j-rest Show documentation
pring Data Neo4j Wrapper for the Neo4j REST API, provides a Graph Database proxy for the remote invocation.
The newest version!
/**
* Copyright (c) 2002-2013 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.rest.graphdb.traversal;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.Path;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.Relationship;
import org.neo4j.helpers.collection.IteratorUtil;
/**
* @author Michael Hunger
* @since 03.02.11
*/
public class SimplePath implements Path {
Iterable relationships;
Iterable nodes;
private final Relationship lastRelationship;
private int length;
private Node startNode;
private Node endNode;
public SimplePath(Node startNode, Node endNode, Relationship lastRelationship, int length, Iterable nodes, Iterable relationships) {
this.startNode = startNode;
this.endNode = endNode;
this.lastRelationship = lastRelationship;
this.length = length;
this.nodes = nodes;
this.relationships = relationships;
}
public Node startNode() {
return startNode;
}
public Node endNode() {
return endNode;
}
public Relationship lastRelationship() {
return lastRelationship;
}
public Iterable relationships() {
return relationships;
}
public Iterable nodes() {
return nodes;
}
public int length() {
return length;
}
public Iterator iterator() {
return new Iterator()
{
Iterator extends PropertyContainer> current = nodes().iterator();
Iterator extends PropertyContainer> next = relationships().iterator();
public boolean hasNext()
{
return current.hasNext();
}
public PropertyContainer next()
{
try
{
return current.next();
}
finally
{
Iterator extends PropertyContainer> temp = current;
current = next;
next = temp;
}
}
public void remove()
{
next.remove();
}
};
}
public Iterable reverseRelationships() {
List reverseRels = IteratorUtil.addToCollection(relationships, new ArrayList());
Collections.reverse(reverseRels);
return reverseRels;
}
public Iterable reverseNodes() {
List reverseNodes = IteratorUtil.addToCollection(nodes, new ArrayList());
Collections.reverse(reverseNodes);
return reverseNodes;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy