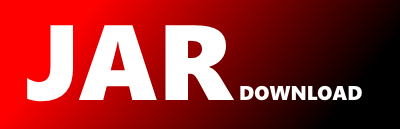
org.neo4j.rest.graphdb.util.QueryResultBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-data-neo4j-rest Show documentation
Show all versions of spring-data-neo4j-rest Show documentation
pring Data Neo4j Wrapper for the Neo4j REST API, provides a Graph Database proxy for the remote invocation.
The newest version!
/**
* Copyright (c) 2002-2013 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.rest.graphdb.util;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.index.IndexHits;
import org.neo4j.helpers.collection.ClosableIterable;
import org.neo4j.helpers.collection.IteratorUtil;
import org.neo4j.helpers.collection.IteratorWrapper;
import org.neo4j.rest.graphdb.query.CypherTransaction;
public class QueryResultBuilder implements QueryResult {
private Iterable result;
private final ResultConverter defaultConverter;
private final boolean isClosableIterable;
private boolean isClosed;
public QueryResultBuilder(Iterable result) {
this(result, new DefaultConverter());
}
public QueryResultBuilder(Iterable result, final ResultConverter defaultConverter) {
this.result = result;
this.isClosableIterable = result instanceof IndexHits || result instanceof ClosableIterable || result instanceof AutoCloseable;;
this.defaultConverter = defaultConverter;
}
@Override
public ConvertedResult to(Class type) {
return this.to(type, defaultConverter);
}
@Override
public ConvertedResult to(final Class type, final ResultConverter resultConverter) {
return new ConvertedResult() {
@Override
public R single() {
try {
final T value = IteratorUtil.single(QueryResultBuilder.this.result);
return resultConverter.convert(value, type);
} finally {
closeIfNeeded();
}
}
@Override
public R singleOrNull() {
try {
final T value = IteratorUtil.singleOrNull(QueryResultBuilder.this.result);
return resultConverter.convert(value, type);
} finally {
closeIfNeeded();
}
}
@Override
public void handle(Handler handler) {
try {
for (T value : result) {
handler.handle(resultConverter.convert(value, type));
}
} finally {
closeIfNeeded();
}
}
@Override
public Iterator iterator() {
return new IteratorWrapper(result.iterator()) {
protected R underlyingObjectToObject(T value) {
return resultConverter.convert(value, type);
}
};
}
};
}
@Override
public void handle(Handler handler) {
try {
for (T value : result) {
handler.handle(value);
}
} finally {
closeIfNeeded();
}
}
private void closeIfNeeded() {
if (isClosableIterable && !isClosed) {
if (result instanceof IndexHits) {
((IndexHits) result).close();
} else if (result instanceof ClosableIterable) {
((ClosableIterable) result).close();
} else if (result instanceof AutoCloseable) {
try {
((AutoCloseable)result).close();
} catch (Exception e) {
// ignore
}
}
isClosed=true;
}
}
@Override
public Iterator iterator() {
return result.iterator();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy