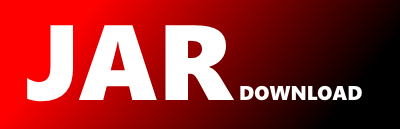
org.springframework.graphql.execution.ReactiveAdapterRegistryHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-graphql Show documentation
Show all versions of spring-graphql Show documentation
GraphQL Support for Spring Applications
/*
* Copyright 2002-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.graphql.execution;
import java.util.Collection;
import io.micrometer.context.Nullable;
import org.reactivestreams.Publisher;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import org.springframework.core.ReactiveAdapter;
import org.springframework.core.ReactiveAdapterRegistry;
import org.springframework.util.Assert;
/**
* Helper to adapt a result Object to {@link Mono} or {@link Flux} through
* {@link ReactiveAdapterRegistry}.
*
* @author Rossen Stoyanchev
* @since 1.3.1
*/
@SuppressWarnings({"ReactiveStreamsUnusedPublisher", "unchecked"})
public abstract class ReactiveAdapterRegistryHelper {
private static final ReactiveAdapterRegistry registry = ReactiveAdapterRegistry.getSharedInstance();
/**
* Return a {@link Mono} for the given Object by delegating to
* {@link #toMonoIfReactive}, and then applying {@link Mono#justOrEmpty}
* if necessary.
* @param result the result Object to adapt
* @param the type of element in the Mono to cast to
* @return a {@code Mono} that represents the result
*/
public static Mono toMono(@Nullable Object result) {
result = toMonoIfReactive(result);
return (Mono) ((result instanceof Mono> mono) ? mono : Mono.justOrEmpty(result));
}
/**
* Return a {@link Mono} for the given result Object if it can be adapted
* to a {@link Publisher} via {@link ReactiveAdapterRegistry}. Multivalued
* publishers are collected to a List.
* @param result the result Object to adapt
* @return the same instance or a {@code Mono} if the object is known to
* {@code ReactiveAdapterRegistry}
*/
@Nullable
public static Object toMonoIfReactive(@Nullable Object result) {
ReactiveAdapter adapter = ((result != null) ? registry.getAdapter(result.getClass()) : null);
if (adapter == null) {
return result;
}
Publisher> publisher = adapter.toPublisher(result);
return (adapter.isMultiValue() ? Flux.from(publisher).collectList() : Mono.from(publisher));
}
/**
* Adapt the given result Object to {@link Mono} or {@link Flux} if it can
* be adapted to a single or multi-value {@link Publisher} respectively
* via {@link ReactiveAdapterRegistry}.
* @param result the result Object to adapt
* @return the same instance, a {@code Mono}, or a {@code Flux}
*/
@Nullable
public static Object toMonoOrFluxIfReactive(@Nullable Object result) {
ReactiveAdapter adapter = ((result != null) ? registry.getAdapter(result.getClass()) : null);
if (adapter == null) {
return result;
}
Publisher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy