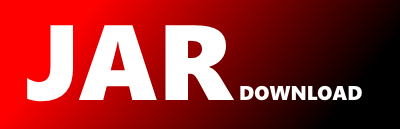
org.springframework.integration.jmx.DefaultMBeanObjectConverter Maven / Gradle / Ivy
/*
* Copyright 2013-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.integration.jmx;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.management.MBeanAttributeInfo;
import javax.management.MBeanInfo;
import javax.management.MBeanServerConnection;
import javax.management.ObjectInstance;
import javax.management.ObjectName;
import javax.management.RuntimeMBeanException;
import javax.management.openmbean.CompositeData;
import javax.management.openmbean.TabularData;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* @author Stuart Williams
* @since 3.0
*
*/
public class DefaultMBeanObjectConverter implements MBeanObjectConverter {
private static final Log log = LogFactory.getLog(DefaultMBeanObjectConverter.class);
private final MBeanAttributeFilter filter;
public DefaultMBeanObjectConverter() {
this(new DefaultMBeanAttributeFilter());
}
public DefaultMBeanObjectConverter(MBeanAttributeFilter filter) {
this.filter = filter;
}
@Override
public Object convert(MBeanServerConnection connection, ObjectInstance instance) {
Map attributeMap = new HashMap();
try {
ObjectName objName = instance.getObjectName();
if (!connection.isRegistered(objName)) {
return attributeMap;
}
MBeanInfo info = connection.getMBeanInfo(objName);
MBeanAttributeInfo[] attributeInfos = info.getAttributes();
for (MBeanAttributeInfo attrInfo : attributeInfos) {
// we don't need to repeat name of this as an attribute
if ("ObjectName".equals(attrInfo.getName()) || !this.filter.accept(objName, attrInfo.getName())) {
continue;
}
Object value;
try {
value = connection.getAttribute(objName, attrInfo.getName());
}
catch (RuntimeMBeanException e) {
// N.B. standard MemoryUsage MBeans will throw an exception when some
// measurement is unsupported. Logging at trace rather than debug to
// avoid confusion.
if (log.isTraceEnabled()) {
log.trace("Error getting attribute '" + attrInfo.getName() + "' on '" + objName + "'", e);
}
// try to unwrap the exception somewhat; not sure this is ideal
Throwable t = e;
while (t.getCause() != null) {
t = t.getCause();
}
value = String.format("%s[%s]", t.getClass().getName(), t.getMessage());
}
attributeMap.put(attrInfo.getName(), checkAndConvert(value));
}
}
catch (Exception e) {
throw new IllegalArgumentException(e);
}
return attributeMap;
}
/**
* @param input
* @return recursively mapped object
*/
private Object checkAndConvert(Object input) {
if (input == null) {
return input;
}
else if (input.getClass().isArray()) {
if (CompositeData.class.isAssignableFrom(input.getClass().getComponentType())) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy