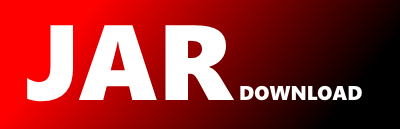
org.springframework.test.json.AbstractJsonValueAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-test Show documentation
Show all versions of spring-test Show documentation
Spring TestContext Framework
The newest version!
/*
* Copyright 2002-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.test.json;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.api.AbstractBooleanAssert;
import org.assertj.core.api.AbstractMapAssert;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.api.AbstractStringAssert;
import org.assertj.core.api.AssertFactory;
import org.assertj.core.api.Assertions;
import org.assertj.core.api.InstanceOfAssertFactories;
import org.assertj.core.api.ObjectArrayAssert;
import org.assertj.core.error.BasicErrorMessageFactory;
import org.assertj.core.internal.Failures;
import org.springframework.core.ResolvableType;
import org.springframework.http.converter.GenericHttpMessageConverter;
import org.springframework.lang.Nullable;
import org.springframework.test.http.HttpMessageContentConverter;
import org.springframework.util.ObjectUtils;
import org.springframework.util.StringUtils;
/**
* Base AssertJ {@linkplain org.assertj.core.api.Assert assertions} that can be
* applied to a JSON value.
*
* In JSON, values must be one of the following data types:
*
* - a {@linkplain #asString() string}
* - a {@linkplain #asNumber() number}
* - a {@linkplain #asBoolean() boolean}
* - an {@linkplain #asArray() array}
* - an {@linkplain #asMap() object} (JSON object)
* - {@linkplain #isNull() null}
*
* This base class offers direct access for each of those types as well as
* conversion methods based on an optional {@link GenericHttpMessageConverter}.
*
* @author Stephane Nicoll
* @since 6.2
* @param the type of assertions
*/
public abstract class AbstractJsonValueAssert>
extends AbstractObjectAssert {
private final Failures failures = Failures.instance();
@Nullable
private final HttpMessageContentConverter contentConverter;
protected AbstractJsonValueAssert(@Nullable Object actual, Class> selfType,
@Nullable HttpMessageContentConverter contentConverter) {
super(actual, selfType);
this.contentConverter = contentConverter;
}
/**
* Verify that the actual value is a non-{@code null} {@link String},
* and return a new {@linkplain AbstractStringAssert assertion} object that
* provides dedicated {@code String} assertions for it.
*/
@Override
public AbstractStringAssert> asString() {
return Assertions.assertThat(castTo(String.class, "a string"));
}
/**
* Verify that the actual value is a non-{@code null} {@link Number},
* usually an {@link Integer} or {@link Double}, and return a new
* {@linkplain AbstractObjectAssert assertion} object for it.
*/
public AbstractObjectAssert, Number> asNumber() {
return Assertions.assertThat(castTo(Number.class, "a number"));
}
/**
* Verify that the actual value is a non-{@code null} {@link Boolean},
* and return a new {@linkplain AbstractBooleanAssert assertion} object
* that provides dedicated {@code Boolean} assertions for it.
*/
public AbstractBooleanAssert> asBoolean() {
return Assertions.assertThat(castTo(Boolean.class, "a boolean"));
}
/**
* Verify that the actual value is a non-{@code null} array, and return a
* new {@linkplain ObjectArrayAssert assertion} object that provides dedicated
* array assertions for it.
*/
public ObjectArrayAssert
© 2015 - 2025 Weber Informatics LLC | Privacy Policy