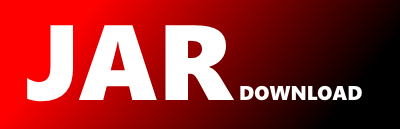
org.springmodules.validation.commons.DefaultValidatorFactory Maven / Gradle / Ivy
/*
* Copyright 2004-2005 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springmodules.validation.commons;
import java.io.IOException;
import java.io.InputStream;
import java.util.Locale;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.commons.validator.Form;
import org.apache.commons.validator.Validator;
import org.apache.commons.validator.ValidatorResources;
import org.springframework.beans.FatalBeanException;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.core.io.Resource;
import org.springframework.util.StringUtils;
import org.springframework.validation.Errors;
import org.xml.sax.SAXException;
/**
* @author Daniel Miller
* @author Rob Harrop
*/
public class DefaultValidatorFactory implements ValidatorFactory, InitializingBean {
/**
* Key used to store the Spring Errors
instance in the Validator
*/
public static final String ERRORS_KEY = "org.springframework.validation.Errors";
/**
* The Log
instance used by this class.
*/
private static Log log = LogFactory.getLog(DefaultValidatorFactory.class);
/**
* the Commons Validator ValidatorResources
used to load validation configuration.
*/
private ValidatorResources validatorResources;
/**
* Checks that the ValidatorResources
exists and has been configured with resources
* via a call to setValidationConfigLocations
.
*
* @throws org.springframework.beans.FatalBeanException if setValidationConfigLocations()
has not been called.
*/
public void afterPropertiesSet() throws Exception {
if (this.validatorResources == null) {
throw new FatalBeanException("Unable to locate validation configuration. Property [validationLocations] is required.");
}
}
/**
* Sets the locations of the validation configuration files from which to load validation rules. Creates an instance
* of ValidatorResources
from the specified configuration files.
*
* @see org.springframework.core.io.Resource
* @see ValidatorResources
*/
public void setValidationConfigLocations(Resource[] validationConfigLocations) {
if (DefaultValidatorFactory.log.isInfoEnabled()) {
DefaultValidatorFactory.log.info("Loading validation configurations from [" + StringUtils.arrayToCommaDelimitedString(validationConfigLocations) + "]");
}
try {
InputStream[] inputStreams = new InputStream[validationConfigLocations.length];
for (int i = 0; i < inputStreams.length; i++) {
inputStreams[i] = validationConfigLocations[i].getInputStream();
}
this.validatorResources = new ValidatorResources(inputStreams);
}
catch (IOException e) {
throw new FatalBeanException("Unable to read validation configuration due to IOException.", e);
}
catch (SAXException e) {
throw new FatalBeanException("Unable to parse validation configuration XML", e);
}
}
/**
* Gets a new instance of a org.apache.commons.validator.Validator
for the given bean.
*
* @param beanName The name of the bean for which this Validator
will be created
* @see org.apache.commons.validator.Validator
*/
public Validator getValidator(String beanName, Object bean, Errors errors) {
Validator validator = new Validator(validatorResources, beanName);
validator.setParameter(DefaultValidatorFactory.ERRORS_KEY, errors);
validator.setParameter(Validator.BEAN_PARAM, bean);
return validator;
}
/**
* Returns true if this validator factory can create a validator that
* supports the given beanName
and locale
.
*
* @param beanName name of the bean to be validated.
* @param locale Locale
to search under.
* @return true
if this validator factory can create a validator for
* the given bean name, false otherwise.
*/
public boolean hasRulesForBean(String beanName, Locale locale) {
Form form = validatorResources.getForm(locale, beanName);
return (form != null);
}
/**
* Gets the managed instance of ValidatorResources
.
*/
public ValidatorResources getValidatorResources() {
return validatorResources;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy