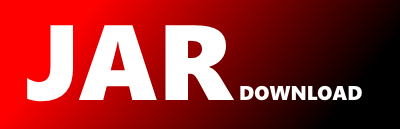
org.sqlproc.engine.SqlFeature Maven / Gradle / Ivy
Show all versions of sql-processor Show documentation
package org.sqlproc.engine;
/**
* The optional features are used to alter the behavior of the SQL Processor. They can be activated in the process of
* SQL Processor initialization.
*
*
* The features can be incorporated into the meta statements file in the form of name(OPT)=...;
.
*
*
* For example the SQL Processor supports the special searching feature based on text fragments. Lets have a table
* PERSON with two columns - ID and NAME.
* In the meta statements file statements.qry there's the next definition:
*
*
* LIKE_STRING(OPT)=like;
* WILDCARD_CHARACTER(OPT)=%;
* SURROUND_QUERY_LIKE_FULL(BOPT)=true;
* SURROUND_QUERY_MIN_LEN(IOPT)=2;
*
* LIKE_PEOPLE(QRY)=
* select p.ID @id, p.NAME @name
* from PERSON p
* {= where
* {& id=:id}
* {& UPPER(name) like :+name}
* }
* {#1 order by ID}
* {#2 order by NAME}
* ;
*
*
*
* The special searching capability is activated with the key SURROUND_QUERY_LIKE(BOPT)=true
. In this case
* every query with the like keyword (defined with the key LIKE_STRING
) is identified and any dynamic input
* value, which belongs to this query condition, is handled in a special way. The value for this input value is
* surrounded with wild-card character %
. This character is defined with the key
* WILDCARD_CHARACTER
. In the runtime to activate this feature, the input value has to have the minimal
* length = 2. This minimal length is defined with the key SURROUND_QUERY_MIN_LEN
.
*
* In the case of SQL Processor initialization
*
*
* JdbcEngineFactory sqlFactory = new JdbcEngineFactory();
* sqlFactory.setMetaFilesNames("statements.qry"); // the meta statements file
* SqlEngine sqlEngine = sqlFactory.getSqlEngine("LIKE_PEOPLE");
*
* // for the case it runs on the top of the JDBC stack
* Connection connection = DriverManager.getConnection("jdbc:hsqldb:mem:sqlproc", "sa", "");
* SqlSession session = new JdbcSimpleSession(connection);
*
*
* there's created an instance of SqlEngine with the name LIKE_PEOPLE
.
*
*
* Next there's an instance person of the class Person with the value an
for the attribute name. The
* invocation
*
*
* Person person = new Person();
* person.setName("jan");
* List<Person> list = sqlEngine.query(session, Person.class, person, SqlOrder.getDescOrder(2));
*
*
* produces the next SQL execution
*
*
* select p.ID id, p.NAME name from PERSON p where UPPER(name) like ? order by NAME DESC
*
*
* In the result list there are all table rows with name, which contains the text fragment jan
.
*
*
* For more info please see the Tutorials.
*
* @author Vladimir Hudec
*/
public enum SqlFeature {
/**
* The filter for Oracle devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader}.
*/
ORACLE,
/**
* The filter for HSQLDB devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader}.
*/
HSQLDB,
/**
* The filter for MySQL devoted optional features. It can be used for the construction of {@link SqlProcessorLoader}
* .
*/
MYSQL,
/**
* The filter for PostgreSQL devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader} .
*/
POSTGRESQL,
/**
* The filter for Informix devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader} .
*/
INFORMIX,
/**
* The filter for MS SQL Server devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader}.
*/
MSSQL,
/**
* The filter for DB2 Server devoted optional features. It can be used for the construction of
* {@link SqlProcessorLoader}.
*/
DB2,
/**
* The filter for H2 devoted optional features. It can be used for the construction of {@link SqlProcessorLoader}.
*/
H2,
/**
* WILDCARD_CHARACTER
is the key for the wildcard character for the SQL like
command.
*/
WILDCARD_CHARACTER,
/**
* SURROUND_QUERY_LIKE
is the key for the special SQL Processor behavior. In the case the value of this
* property is true
, the SQL Processor sets the wildcard character as a prefix and postfix for all
* string values related to the SQL command like
. These string values should have to have the minimal
* length greater or equal to SURROUND_QUERY_MIN_LEN
. The default value related to this key is
* false
.
*/
SURROUND_QUERY_LIKE_FULL,
/**
* SURROUND_QUERY_LIKE_PARTIAL
is the key for the special SQL Processor behavior. In the case the value
* of this property is true
, the SQL Processor sets the wildcard character as a postfix for all string
* values related to the SQL command like
. These string values should have to have the minimal length
* greater or equal to SURROUND_QUERY_MIN_LEN
. The default value related to this key is
* false
.
*/
SURROUND_QUERY_LIKE_PARTIAL,
/**
* SURROUND_QUERY_MIN_LEN
is the minimal length of the string input values for the SQL
* like
command to switch on the SQL Processor special behavior described above in the runtime.
*/
SURROUND_QUERY_MIN_LEN,
/**
* LIKE_STRING
is the key for the SQL command like
. For example for the Informix database
* it can be matches
.
*/
LIKE_STRING,
/**
* REPLACE_LIKE_STRING
is the key for the special SQL Processor behavior related to the SQL command
* like
. The supplied value is going to be used as the final LIKE_STRING.
*/
REPLACE_LIKE_STRING,
/**
* REPLACE_LIKE_CHARS
is the key for the special SQL Processor behavior related to the SQL command
* like
. The feature pattern is ['c1':'r1', 'c2':'r2', ...]
, where c1,c2,...
* is a set of characters to be replaced with the characters r1,r2,...
. For example to use the wildcard
* characters *
and ?
instead of %
_
, use
* ['*':'%', '?':'_']
.
*/
REPLACE_LIKE_CHARS,
/**
* METHODS_ENUM_IN
lists the methods used in the translation from a Java type to a JDBC datatype for
* enumerations based input values.
*/
METHODS_ENUM_IN,
/**
* METHODS_ENUM_OUT
lists the methods used in the translation from a JDBC datatype to a Java type for
* enumerations based output values.
*/
METHODS_ENUM_OUT,
/**
* ID
is the key for the identity columns. In the case it's values is defined, all columns with this
* name are implicitly treated as an identifier.
*/
ID,
/**
* IGNORE_INPROPER_IN
is the key for special handling of input/output values. In the case it's value is
* defined as true, in the case of any problems with input values the SqlRuntimeException is not thrown, only the
* related error is logged.
*/
IGNORE_INPROPER_IN,
/**
* IGNORE_INPROPER_OUT
is the key for special handling of input/output values. In the case it's value
* is defined as true, in the case of any problems with output values the SqlRuntimeException is not thrown, only
* the related error is logged.
*/
IGNORE_INPROPER_OUT,
/**
* JDBC
is the key for the raw JDBC stack usage. In this SQL Processor version the next stacks can be
* used:
*
* - raw JDBC
* - Hibernate
* - Spring DAO
*
* The default value related to this key is false
.
*/
JDBC,
/**
* LIMIT_FROM_TO
is the key for the SQL query pattern used to limit the query results. This pattern has
* to be combined with the original SQL query, the limit and the offset in the next way:
*
* - $S is the full original query
* - $s is the original query without the token
select
* - $F is 1-based from rowid (=offset)
* - $f is 0-based from rowid (offset)
* - $M is the max number of returned rows
* - $m is the max rowid of returned rows
*
*/
LIMIT_FROM_TO,
/**
* LIMIT_FROM_TO_ORDERED
is the key for the SQL query pattern used to limit the query results in the
* case the query output is sorted. Right now it's used only for HSQLDB.
*/
LIMIT_FROM_TO_ORDERED,
/**
* LIMIT_TO
is the key for the SQL query pattern used to limit the query results. This pattern has to
* be combined with the original SQL query and the limit in the next way:
*
* - $S is the full original query
* - $s is the original query without the token
select
* - $F is 1-based from rowid (=offset)
* - $f is 0-based from rowid (offset)
*
*/
LIMIT_TO,
/**
* LIMIT_TO_ORDERED
is the key for the SQL query pattern used to limit the query results in the case
* the query output is sorted. Right now it's used only for HSQLDB.
*/
LIMIT_TO_ORDERED,
/**
* SEQ
is the key for the SQL query pattern used for the sequences. This pattern can be combined with
* the sequence name used in the META SQL query in the next way:
*
* - $n is the name of the sequence from the META SQL query
*
*/
SEQ,
/**
* SEQ_NAME
is the key for the sequence name.
*/
SEQ_NAME,
/**
* IDSEL
is the key for the SQL query pattern used to obtain the value of identities after the INSERT
* command.
*/
IDSEL,
/**
* IDSEL_Long
is the key for the SQL query pattern used to obtain the value of identities after the
* INSERT command.
*/
IDSEL_Long,
/**
* IDSEL_Integer
is the key for the SQL query pattern used to obtain the value of identities after the
* INSERT command.
*/
IDSEL_Integer,
/**
* IDGEN
is the key for the SQL query pattern used to obtain the value of identities or sequences.
*/
IDGEN,
/**
* VERSION_COLUMN
is the key for the version column name.
*/
VERSION_COLUMN,
/**
* EMPTY_FOR_NULL
is indicator that the NULL values are always empty. It has meaning for the UPDATE
* statements, where the standard handling is to treat any values as not empty.
*/
EMPTY_FOR_NULL,
/**
* EMPTY_USE_METHOD_IS_NULL
is indicator that the non-emptiness depends the special isNull method. It
* has meaning for the UPDATE statements, where the standard handling is to treat any values as not empty.
*/
EMPTY_USE_METHOD_IS_NULL,
/**
* OPERATOR_ATTRIBUTE
is the operator atribute name suffix.
*/
OPERATOR_ATTRIBUTE,
/**
* OPERATOR_ATTRIBUTE_IN_MAP
is the map name of the operators name suffix.
*/
OPERATOR_ATTRIBUTE_IN_MAP,
/**
* LOG_SQL_COMMAND_FOR_EXCEPTION
is the indicator that in the case of an SQLException the related SQL
* command should be logged.
*/
LOG_SQL_COMMAND_FOR_EXCEPTION;
}