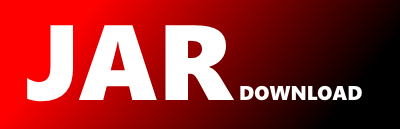
org.srplib.conversion.Converters Maven / Gradle / Ivy
Show all versions of srp-conversion-support Show documentation
package org.srplib.conversion;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
/**
* Factory class producing instances of {@link Converter} and {@link ConverterRegistry}
*
* @author Anton Pechinsky
*/
public class Converters {
/**
* Returns empty converter registry.
*
* @return ConverterRegistry converter registry without any converters.
*/
public static ConverterRegistry newRegistry() {
return new ConverterRegistry();
}
/**
* Returns default converter registry containing basic converter set.
*
* @return ConverterRegistry converter registry.
*/
public static ConverterRegistry newDefaultRegistry() {
ConverterRegistry converters = new ConverterRegistry();
converters.registerConverter(String.class, Double.class, new StringToDoubleConverter());
converters.registerConverter(String.class, double.class, new StringToDoubleConverter());
converters.registerConverter(String.class, Integer.class, new StringToIntegerConverter());
converters.registerConverter(String.class, int.class, new StringToIntegerConverter());
converters.registerConverter(String.class, Boolean.class, new StringToBooleanConverter());
converters.registerConverter(String.class, boolean.class, new StringToBooleanConverter());
converters.registerConverter(Boolean.class, boolean.class, EmptyConverter.instance());
converters.registerConverter(Integer.class, String.class, new IntegerToStringConverter());
converters.registerConverter(int.class, String.class, new IntegerToStringConverter());
converters.registerConverter(Integer.class, Long.class, new IntegerToLongConverter());
converters.registerConverter(Integer.class, long.class, new IntegerToLongConverter());
return converters;
}
/**
* Returns {@link EmptyConverter} which simply returns passed value back.
*
* @return EmptyConverter an empty converter
* @see EmptyConverter
*/
public static TwoWayConverter empty() {
return (TwoWayConverter)EmptyConverter.instance();
}
/**
* Returns string to enum converter.
*
*
* Call to this method is equivalent to new StringToEnumConverter(Sex.class)
*
*
* @param enumClass Class class of enum
* @return DataConverter
*/
public static Converter newStringToEnumConverter(Class enumClass) {
return new StringToEnumConverter(enumClass);
}
/**
* Creates converter from boolean value to specified alternatives.
*
* Converter may be considered as {@code IF (input) THEN trueValue ELSE falseValue}
*
* @param trueValue a value to return if input value is {@code true}
* @param falseValue a value to return if input value is {@code false}
* @return Converter
*/
public static Converter choice(T trueValue, T falseValue) {
return new IfConverter(trueValue, falseValue);
}
/**
* Creates converter which uses map for value conversion.
*
* Converter may be considered as {@code SWITCH (input) OF ... DEFAULT}
*
* @param map Map a map where input values are keys and values are output values.
* @param defaultValue Object default value if no matches found in map
* @return Converter
* @see SwitchConverter
*/
public static Converter choice(Map map, O defaultValue) {
return new SwitchConverter(map, defaultValue);
}
/**
* Creates converter which uses map for value conversion.
*
* Unlike {@link #choice(Map, Object)} this method doesn't accept default value and throws
* {@link IllegalStateException} if no mapping is found.
*
* @param map Map a map where input values are keys and values are output values.
* @return Converter
* @see SwitchConverter
*/
public static Converter choice(Map map) {
return new SwitchConverter(map);
}
/**
* Converts vararg array of converters into single converter.
*
* Method is useful when you have serveral converters but API expect single instance. So you can make a chain of
* converters.
*
* If converters is null or empty then {@link #empty} converter is returned (null-value)
*
* If converters.length == 1 then converters[0] is returned
*
* If converters.length > 1 then {@link ChainConverter} is returned.
*
* @param converters vararg of Converter
* @return Converter a converter encapsulating all converters.
*/
public static Converter chain(Converter... converters) {
if (converters == null) {
return empty();
}
return chain(Arrays.asList(converters));
}
/**
* Converts list of converters into single converter.
*
* Method is similar to {@link #chain(Converter[])}.
*
* @param converters vararg of Converter
* @return Converter a converter encapsulating all converters.
*/
public static Converter chain(List converters) {
Converter result;
if (converters == null || converters.isEmpty()) {
result = empty();
}
else if (converters.size() == 1) {
result = converters.get(0);
}
else {
result = new ChainConverter(converters);
}
return result;
}
/**
* Creates converter from object to boolean.
*
* Converter will return {@code true} if object is equal to specified object.
*
* @param object an object to compare to. Nulls are accepted.
* @return Converter from object to boolean.
*/
public static Converter equal(T object) {
return new EqualsConverter(object);
}
}