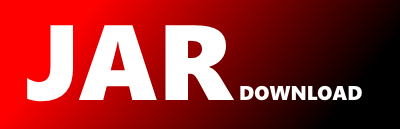
org.srplib.visitor.NodePath Maven / Gradle / Ivy
package org.srplib.visitor;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* Path structure.
*
* Structure is natively recursive: contains current node and a reference to parent path.
*
* Useful for recursive algorithms such as tree traversal.
*
* @author Anton Pechinsky
*/
public class NodePath implements Iterable {
private final NodePath parent;
private final T current;
private NodePath(NodePath parent, T node) {
this.parent = parent;
this.current = node;
}
public NodePath(T node) {
this(null, node);
}
public T getCurrent() {
return current;
}
public boolean hasParent() {
return parent != null;
}
public NodePath getParent() {
return parent;
}
public NodePath add(T node) {
return new NodePath<>(this, node);
}
public Iterator iterator() {
return new MyIterator<>(this);
}
public String format(String separator) {
List nodes = getNodes();
Collections.reverse(nodes);
StringBuilder sb = new StringBuilder();
for (Iterator iterator = nodes.iterator(); iterator.hasNext(); ) {
sb.append(iterator.next());
sb.append(iterator.hasNext() ? separator : "");
}
return sb.toString();
}
private List getNodes() {
List nodes = new ArrayList<>();
for (T node : this) {
nodes.add(node);
}
return nodes;
}
@Override
public String toString() {
return format(" -> ");
}
private static class MyIterator implements Iterator {
private NodePath path;
public MyIterator(NodePath path) {
this.path = path;
}
@Override
public boolean hasNext() {
return path != null;
}
@Override
public T next() {
T current = path.getCurrent();
path = path.getParent();
return current;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy