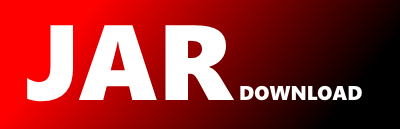
org.srplib.reflection.classgraph.ClassGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of srp-reflection-support Show documentation
Show all versions of srp-reflection-support Show documentation
Single Responsibility Principle (SRP) libraries collection
package org.srplib.reflection.classgraph;
import java.lang.reflect.Field;
import org.srplib.contract.Argument;
import org.srplib.reflection.ReflectionUtils;
import org.srplib.visitor.Element;
import org.srplib.support.Predicate;
import org.srplib.visitor.NodePath;
/**
* Encapsulates java class graph navigation algorithm.
*
* @author Anton Pechinsky
*/
public class ClassGraph> implements Element {
private Class root;
private Predicate filter;
/**
* Creates object graph with specified root class and node filter.
*
* @param root Object root object.
* @param filter Predicate node filter
*/
public ClassGraph(Class root, Predicate filter) {
Argument.checkNotNull(root, "root must not be null!");
Argument.checkNotNull(filter, "filter must not be null!");
this.root = root;
this.filter = filter;
}
/**
* Creates object graph with specified root class and default node filter.
*
* @param root Object root object.
*/
public ClassGraph(Class root) {
this(root, new TraversableNodesFilter());
}
@Override
public void accept(V visitor) {
N node = visitor.resolveNode(ClassGraphNode.create(root));
traverse(new NodePath<>(node), visitor);
}
private void traverse(NodePath path, V visitor) {
visitor.visit(path);
if (!isTraversable(path)) {
return;
}
ClassGraphNode node = path.getCurrent();
for (Field field : ReflectionUtils.getFieldsRecursively(node.getType())) {
N newNode = visitor.resolveNode(ClassGraphNode.create(field));
traverse(path.add(newNode), visitor);
}
}
private boolean isTraversable(NodePath path) {
return filter.test(path.getCurrent());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy