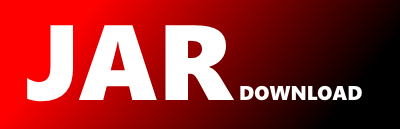
org.ssssssss.magicapi.cache.DefaultSqlCache Maven / Gradle / Ivy
package org.ssssssss.magicapi.cache;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class DefaultSqlCache extends LinkedHashMap> implements SqlCache {
private final String separator = ":";
private final int capacity;
private final long expire;
private final ReentrantReadWriteLock lock = new ReentrantReadWriteLock();
public DefaultSqlCache(int capacity, long expire) {
super((int) Math.ceil(capacity / 0.75) + 1, 0.75f, true);
// 容量
this.capacity = capacity;
// 固定过期时间
this.expire = expire;
}
@Override
public void put(String name, String key, Object value) {
// 封装成过期时间节点
put(name, key, value, this.expire);
}
@Override
public void put(String name, String key, Object value, long ttl) {
long expireTime = ttl > 0 ? (System.currentTimeMillis() + ttl) :
(this.expire > -1 ? System.currentTimeMillis() + this.expire : Long.MAX_VALUE);
lock.writeLock().lock();
try {
// 封装成过期时间节点
put(name + separator + key, new ExpireNode<>(expireTime, value));
} finally {
lock.writeLock().unlock();
}
}
@Override
public Object get(String name, String key) {
key = name + separator + key;
lock.readLock().lock();
ExpireNode
© 2015 - 2025 Weber Informatics LLC | Privacy Policy